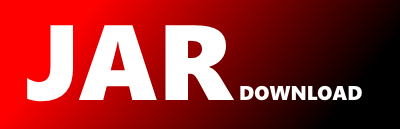
software.amazon.awssdk.services.s3outposts.model.CreateEndpointRequest Maven / Gradle / Ivy
Show all versions of s3outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.s3outposts.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateEndpointRequest extends S3OutpostsRequest implements
ToCopyableBuilder {
private static final SdkField OUTPOST_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutpostId").getter(getter(CreateEndpointRequest::outpostId)).setter(setter(Builder::outpostId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutpostId").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetId").getter(getter(CreateEndpointRequest::subnetId)).setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId").build()).build();
private static final SdkField SECURITY_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecurityGroupId").getter(getter(CreateEndpointRequest::securityGroupId))
.setter(setter(Builder::securityGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupId").build()).build();
private static final SdkField ACCESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccessType").getter(getter(CreateEndpointRequest::accessTypeAsString))
.setter(setter(Builder::accessType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccessType").build()).build();
private static final SdkField CUSTOMER_OWNED_IPV4_POOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomerOwnedIpv4Pool").getter(getter(CreateEndpointRequest::customerOwnedIpv4Pool))
.setter(setter(Builder::customerOwnedIpv4Pool))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomerOwnedIpv4Pool").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OUTPOST_ID_FIELD,
SUBNET_ID_FIELD, SECURITY_GROUP_ID_FIELD, ACCESS_TYPE_FIELD, CUSTOMER_OWNED_IPV4_POOL_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("OutpostId", OUTPOST_ID_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("SecurityGroupId", SECURITY_GROUP_ID_FIELD);
put("AccessType", ACCESS_TYPE_FIELD);
put("CustomerOwnedIpv4Pool", CUSTOMER_OWNED_IPV4_POOL_FIELD);
}
});
private final String outpostId;
private final String subnetId;
private final String securityGroupId;
private final String accessType;
private final String customerOwnedIpv4Pool;
private CreateEndpointRequest(BuilderImpl builder) {
super(builder);
this.outpostId = builder.outpostId;
this.subnetId = builder.subnetId;
this.securityGroupId = builder.securityGroupId;
this.accessType = builder.accessType;
this.customerOwnedIpv4Pool = builder.customerOwnedIpv4Pool;
}
/**
*
* The ID of the Outposts.
*
*
* @return The ID of the Outposts.
*/
public final String outpostId() {
return outpostId;
}
/**
*
* The ID of the subnet in the selected VPC. The endpoint subnet must belong to the Outpost that has Amazon S3 on
* Outposts provisioned.
*
*
* @return The ID of the subnet in the selected VPC. The endpoint subnet must belong to the Outpost that has Amazon
* S3 on Outposts provisioned.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The ID of the security group to use with the endpoint.
*
*
* @return The ID of the security group to use with the endpoint.
*/
public final String securityGroupId() {
return securityGroupId;
}
/**
*
* The type of access for the network connectivity for the Amazon S3 on Outposts endpoint. To use the Amazon Web
* Services VPC, choose Private
. To use the endpoint with an on-premises network, choose
* CustomerOwnedIp
. If you choose CustomerOwnedIp
, you must also provide the
* customer-owned IP address pool (CoIP pool).
*
*
*
* Private
is the default access type value.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accessType} will
* return {@link EndpointAccessType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #accessTypeAsString}.
*
*
* @return The type of access for the network connectivity for the Amazon S3 on Outposts endpoint. To use the Amazon
* Web Services VPC, choose Private
. To use the endpoint with an on-premises network, choose
* CustomerOwnedIp
. If you choose CustomerOwnedIp
, you must also provide the
* customer-owned IP address pool (CoIP pool).
*
* Private
is the default access type value.
*
* @see EndpointAccessType
*/
public final EndpointAccessType accessType() {
return EndpointAccessType.fromValue(accessType);
}
/**
*
* The type of access for the network connectivity for the Amazon S3 on Outposts endpoint. To use the Amazon Web
* Services VPC, choose Private
. To use the endpoint with an on-premises network, choose
* CustomerOwnedIp
. If you choose CustomerOwnedIp
, you must also provide the
* customer-owned IP address pool (CoIP pool).
*
*
*
* Private
is the default access type value.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accessType} will
* return {@link EndpointAccessType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #accessTypeAsString}.
*
*
* @return The type of access for the network connectivity for the Amazon S3 on Outposts endpoint. To use the Amazon
* Web Services VPC, choose Private
. To use the endpoint with an on-premises network, choose
* CustomerOwnedIp
. If you choose CustomerOwnedIp
, you must also provide the
* customer-owned IP address pool (CoIP pool).
*
* Private
is the default access type value.
*
* @see EndpointAccessType
*/
public final String accessTypeAsString() {
return accessType;
}
/**
*
* The ID of the customer-owned IPv4 address pool (CoIP pool) for the endpoint. IP addresses are allocated from this
* pool for the endpoint.
*
*
* @return The ID of the customer-owned IPv4 address pool (CoIP pool) for the endpoint. IP addresses are allocated
* from this pool for the endpoint.
*/
public final String customerOwnedIpv4Pool() {
return customerOwnedIpv4Pool;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(outpostId());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(securityGroupId());
hashCode = 31 * hashCode + Objects.hashCode(accessTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(customerOwnedIpv4Pool());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateEndpointRequest)) {
return false;
}
CreateEndpointRequest other = (CreateEndpointRequest) obj;
return Objects.equals(outpostId(), other.outpostId()) && Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(securityGroupId(), other.securityGroupId())
&& Objects.equals(accessTypeAsString(), other.accessTypeAsString())
&& Objects.equals(customerOwnedIpv4Pool(), other.customerOwnedIpv4Pool());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateEndpointRequest").add("OutpostId", outpostId()).add("SubnetId", subnetId())
.add("SecurityGroupId", securityGroupId()).add("AccessType", accessTypeAsString())
.add("CustomerOwnedIpv4Pool", customerOwnedIpv4Pool()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OutpostId":
return Optional.ofNullable(clazz.cast(outpostId()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "SecurityGroupId":
return Optional.ofNullable(clazz.cast(securityGroupId()));
case "AccessType":
return Optional.ofNullable(clazz.cast(accessTypeAsString()));
case "CustomerOwnedIpv4Pool":
return Optional.ofNullable(clazz.cast(customerOwnedIpv4Pool()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function