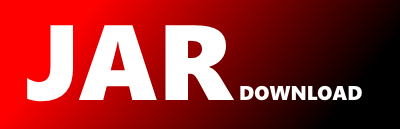
software.amazon.awssdk.services.sagemaker.model.CreateCompilationJobRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateCompilationJobRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField COMPILATION_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompilationJobName").getter(getter(CreateCompilationJobRequest::compilationJobName))
.setter(setter(Builder::compilationJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationJobName").build())
.build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(CreateCompilationJobRequest::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField INPUT_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("InputConfig").getter(getter(CreateCompilationJobRequest::inputConfig))
.setter(setter(Builder::inputConfig)).constructor(InputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputConfig").build()).build();
private static final SdkField OUTPUT_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("OutputConfig").getter(getter(CreateCompilationJobRequest::outputConfig))
.setter(setter(Builder::outputConfig)).constructor(OutputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputConfig").build()).build();
private static final SdkField STOPPING_CONDITION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StoppingCondition")
.getter(getter(CreateCompilationJobRequest::stoppingCondition)).setter(setter(Builder::stoppingCondition))
.constructor(StoppingCondition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StoppingCondition").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateCompilationJobRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPILATION_JOB_NAME_FIELD,
ROLE_ARN_FIELD, INPUT_CONFIG_FIELD, OUTPUT_CONFIG_FIELD, STOPPING_CONDITION_FIELD, TAGS_FIELD));
private final String compilationJobName;
private final String roleArn;
private final InputConfig inputConfig;
private final OutputConfig outputConfig;
private final StoppingCondition stoppingCondition;
private final List tags;
private CreateCompilationJobRequest(BuilderImpl builder) {
super(builder);
this.compilationJobName = builder.compilationJobName;
this.roleArn = builder.roleArn;
this.inputConfig = builder.inputConfig;
this.outputConfig = builder.outputConfig;
this.stoppingCondition = builder.stoppingCondition;
this.tags = builder.tags;
}
/**
*
* A name for the model compilation job. The name must be unique within the AWS Region and within your AWS account.
*
*
* @return A name for the model compilation job. The name must be unique within the AWS Region and within your AWS
* account.
*/
public final String compilationJobName() {
return compilationJobName;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the caller of
* this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your
* behalf.
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the
* caller of this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* Provides information about the location of input model artifacts, the name and shape of the expected data inputs,
* and the framework in which the model was trained.
*
*
* @return Provides information about the location of input model artifacts, the name and shape of the expected data
* inputs, and the framework in which the model was trained.
*/
public final InputConfig inputConfig() {
return inputConfig;
}
/**
*
* Provides information about the output location for the compiled model and the target device the model runs on.
*
*
* @return Provides information about the output location for the compiled model and the target device the model
* runs on.
*/
public final OutputConfig outputConfig() {
return outputConfig;
}
/**
*
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*
*
* @return Specifies a limit to how long a model compilation job can run. When the job reaches the time limit,
* Amazon SageMaker ends the compilation job. Use this API to cap model training costs.
*/
public final StoppingCondition stoppingCondition() {
return stoppingCondition;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your AWS resources in different ways, for example, by
* purpose, owner, or environment. For more information, see Tagging AWS Resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return An array of key-value pairs. You can use tags to categorize your AWS resources in different ways, for
* example, by purpose, owner, or environment. For more information, see Tagging AWS Resources.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(compilationJobName());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(inputConfig());
hashCode = 31 * hashCode + Objects.hashCode(outputConfig());
hashCode = 31 * hashCode + Objects.hashCode(stoppingCondition());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateCompilationJobRequest)) {
return false;
}
CreateCompilationJobRequest other = (CreateCompilationJobRequest) obj;
return Objects.equals(compilationJobName(), other.compilationJobName()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(inputConfig(), other.inputConfig()) && Objects.equals(outputConfig(), other.outputConfig())
&& Objects.equals(stoppingCondition(), other.stoppingCondition()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateCompilationJobRequest").add("CompilationJobName", compilationJobName())
.add("RoleArn", roleArn()).add("InputConfig", inputConfig()).add("OutputConfig", outputConfig())
.add("StoppingCondition", stoppingCondition()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CompilationJobName":
return Optional.ofNullable(clazz.cast(compilationJobName()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "InputConfig":
return Optional.ofNullable(clazz.cast(inputConfig()));
case "OutputConfig":
return Optional.ofNullable(clazz.cast(outputConfig()));
case "StoppingCondition":
return Optional.ofNullable(clazz.cast(stoppingCondition()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function