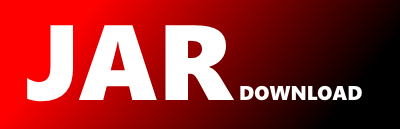
software.amazon.awssdk.services.sagemaker.model.CreateFeatureGroupRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateFeatureGroupRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField FEATURE_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FeatureGroupName").getter(getter(CreateFeatureGroupRequest::featureGroupName))
.setter(setter(Builder::featureGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FeatureGroupName").build()).build();
private static final SdkField RECORD_IDENTIFIER_FEATURE_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RecordIdentifierFeatureName")
.getter(getter(CreateFeatureGroupRequest::recordIdentifierFeatureName))
.setter(setter(Builder::recordIdentifierFeatureName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecordIdentifierFeatureName")
.build()).build();
private static final SdkField EVENT_TIME_FEATURE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EventTimeFeatureName").getter(getter(CreateFeatureGroupRequest::eventTimeFeatureName))
.setter(setter(Builder::eventTimeFeatureName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventTimeFeatureName").build())
.build();
private static final SdkField> FEATURE_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("FeatureDefinitions")
.getter(getter(CreateFeatureGroupRequest::featureDefinitions))
.setter(setter(Builder::featureDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FeatureDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(FeatureDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ONLINE_STORE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OnlineStoreConfig")
.getter(getter(CreateFeatureGroupRequest::onlineStoreConfig)).setter(setter(Builder::onlineStoreConfig))
.constructor(OnlineStoreConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnlineStoreConfig").build()).build();
private static final SdkField OFFLINE_STORE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OfflineStoreConfig")
.getter(getter(CreateFeatureGroupRequest::offlineStoreConfig)).setter(setter(Builder::offlineStoreConfig))
.constructor(OfflineStoreConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OfflineStoreConfig").build())
.build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(CreateFeatureGroupRequest::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateFeatureGroupRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateFeatureGroupRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FEATURE_GROUP_NAME_FIELD,
RECORD_IDENTIFIER_FEATURE_NAME_FIELD, EVENT_TIME_FEATURE_NAME_FIELD, FEATURE_DEFINITIONS_FIELD,
ONLINE_STORE_CONFIG_FIELD, OFFLINE_STORE_CONFIG_FIELD, ROLE_ARN_FIELD, DESCRIPTION_FIELD, TAGS_FIELD));
private final String featureGroupName;
private final String recordIdentifierFeatureName;
private final String eventTimeFeatureName;
private final List featureDefinitions;
private final OnlineStoreConfig onlineStoreConfig;
private final OfflineStoreConfig offlineStoreConfig;
private final String roleArn;
private final String description;
private final List tags;
private CreateFeatureGroupRequest(BuilderImpl builder) {
super(builder);
this.featureGroupName = builder.featureGroupName;
this.recordIdentifierFeatureName = builder.recordIdentifierFeatureName;
this.eventTimeFeatureName = builder.eventTimeFeatureName;
this.featureDefinitions = builder.featureDefinitions;
this.onlineStoreConfig = builder.onlineStoreConfig;
this.offlineStoreConfig = builder.offlineStoreConfig;
this.roleArn = builder.roleArn;
this.description = builder.description;
this.tags = builder.tags;
}
/**
*
* The name of the FeatureGroup
. The name must be unique within an AWS Region in an AWS account. The
* name:
*
*
* -
*
* Must start and end with an alphanumeric character.
*
*
* -
*
* Can only contain alphanumeric character and hyphens. Spaces are not allowed.
*
*
*
*
* @return The name of the FeatureGroup
. The name must be unique within an AWS Region in an AWS
* account. The name:
*
* -
*
* Must start and end with an alphanumeric character.
*
*
* -
*
* Can only contain alphanumeric character and hyphens. Spaces are not allowed.
*
*
*/
public final String featureGroupName() {
return featureGroupName;
}
/**
*
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions' names.
*
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start and end with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*
*
* @return The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions'
* names.
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start and end with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*/
public final String recordIdentifierFeatureName() {
return recordIdentifierFeatureName;
}
/**
*
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or update
* of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and yyyy-MM-dd'T'HH:mm:ss.SSSZ
* where yyyy
, MM
, and dd
represent the year, month, and day respectively and
* HH
, mm
, ss
, and if applicable, SSS
represent the hour, month,
* second and milliseconds respsectively. 'T'
and Z
are constants.
*
*
*
*
* @return The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or
* update of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and
* yyyy-MM-dd'T'HH:mm:ss.SSSZ
where yyyy
, MM
, and dd
* represent the year, month, and day respectively and HH
, mm
, ss
,
* and if applicable, SSS
represent the hour, month, second and milliseconds respsectively.
* 'T'
and Z
are constants.
*
*
*/
public final String eventTimeFeatureName() {
return eventTimeFeatureName;
}
/**
* Returns true if the FeatureDefinitions property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasFeatureDefinitions() {
return featureDefinitions != null && !(featureDefinitions instanceof SdkAutoConstructList);
}
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasFeatureDefinitions()} to see if a value was sent in this field.
*
*
* @return A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
,
* write_time
, api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*/
public final List featureDefinitions() {
return featureDefinitions;
}
/**
*
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
; the default value is False
.
*
*
* You can also include an AWS KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*
*
* @return You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
; the default value is
* False
.
*
* You can also include an AWS KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*/
public final OnlineStoreConfig onlineStoreConfig() {
return onlineStoreConfig;
}
/**
*
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an AWS Glue or AWS Hive data cataolgue.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*
*
* @return Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an AWS Glue or AWS Hive data cataolgue.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*/
public final OfflineStoreConfig offlineStoreConfig() {
return offlineStoreConfig;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the OfflineStore
* if an OfflineStoreConfig
is provided.
*
*
* @return The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the
* OfflineStore
if an OfflineStoreConfig
is provided.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* A free-form description of a FeatureGroup
.
*
*
* @return A free-form description of a FeatureGroup
.
*/
public final String description() {
return description;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return Tags used to identify Features
in each FeatureGroup
.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(featureGroupName());
hashCode = 31 * hashCode + Objects.hashCode(recordIdentifierFeatureName());
hashCode = 31 * hashCode + Objects.hashCode(eventTimeFeatureName());
hashCode = 31 * hashCode + Objects.hashCode(hasFeatureDefinitions() ? featureDefinitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(onlineStoreConfig());
hashCode = 31 * hashCode + Objects.hashCode(offlineStoreConfig());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateFeatureGroupRequest)) {
return false;
}
CreateFeatureGroupRequest other = (CreateFeatureGroupRequest) obj;
return Objects.equals(featureGroupName(), other.featureGroupName())
&& Objects.equals(recordIdentifierFeatureName(), other.recordIdentifierFeatureName())
&& Objects.equals(eventTimeFeatureName(), other.eventTimeFeatureName())
&& hasFeatureDefinitions() == other.hasFeatureDefinitions()
&& Objects.equals(featureDefinitions(), other.featureDefinitions())
&& Objects.equals(onlineStoreConfig(), other.onlineStoreConfig())
&& Objects.equals(offlineStoreConfig(), other.offlineStoreConfig()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(description(), other.description()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateFeatureGroupRequest").add("FeatureGroupName", featureGroupName())
.add("RecordIdentifierFeatureName", recordIdentifierFeatureName())
.add("EventTimeFeatureName", eventTimeFeatureName())
.add("FeatureDefinitions", hasFeatureDefinitions() ? featureDefinitions() : null)
.add("OnlineStoreConfig", onlineStoreConfig()).add("OfflineStoreConfig", offlineStoreConfig())
.add("RoleArn", roleArn()).add("Description", description()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "FeatureGroupName":
return Optional.ofNullable(clazz.cast(featureGroupName()));
case "RecordIdentifierFeatureName":
return Optional.ofNullable(clazz.cast(recordIdentifierFeatureName()));
case "EventTimeFeatureName":
return Optional.ofNullable(clazz.cast(eventTimeFeatureName()));
case "FeatureDefinitions":
return Optional.ofNullable(clazz.cast(featureDefinitions()));
case "OnlineStoreConfig":
return Optional.ofNullable(clazz.cast(onlineStoreConfig()));
case "OfflineStoreConfig":
return Optional.ofNullable(clazz.cast(offlineStoreConfig()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function