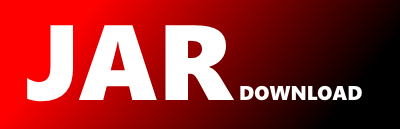
software.amazon.awssdk.services.sagemaker.model.SecondaryStatusTransition Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An array element of DescribeTrainingJobResponse$SecondaryStatusTransitions. It provides additional details
* about a status that the training job has transitioned through. A training job can be in one of several states, for
* example, starting, downloading, training, or uploading. Within each state, there are a number of intermediate states.
* For example, within the starting state, Amazon SageMaker could be starting the training job or launching the ML
* instances. These transitional states are referred to as the job's secondary status.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SecondaryStatusTransition implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(SecondaryStatusTransition::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(SecondaryStatusTransition::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(SecondaryStatusTransition::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusMessage").getter(getter(SecondaryStatusTransition::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STATUS_FIELD,
START_TIME_FIELD, END_TIME_FIELD, STATUS_MESSAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String status;
private final Instant startTime;
private final Instant endTime;
private final String statusMessage;
private SecondaryStatusTransition(BuilderImpl builder) {
this.status = builder.status;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.statusMessage = builder.statusMessage;
}
/**
*
* Contains a secondary status information from a training job.
*
*
* Status might be one of the following secondary statuses:
*
*
* - InProgress
* -
*
* -
*
* Starting
- Starting the training job.
*
*
* -
*
* Downloading
- An optional stage for algorithms that support File
training input mode.
* It indicates that data is being downloaded to the ML storage volumes.
*
*
* -
*
* Training
- Training is in progress.
*
*
* -
*
* Uploading
- Training is complete and the model artifacts are being uploaded to the S3 location.
*
*
*
*
* - Completed
* -
*
* -
*
* Completed
- The training job has completed.
*
*
*
*
* - Failed
* -
*
* -
*
* Failed
- The training job has failed. The reason for the failure is returned in the
* FailureReason
field of DescribeTrainingJobResponse
.
*
*
*
*
* - Stopped
* -
*
* -
*
* MaxRuntimeExceeded
- The job stopped because it exceeded the maximum allowed runtime.
*
*
* -
*
* Stopped
- The training job has stopped.
*
*
*
*
* - Stopping
* -
*
* -
*
* Stopping
- Stopping the training job.
*
*
*
*
*
*
* We no longer support the following secondary statuses:
*
*
* -
*
* LaunchingMLInstances
*
*
* -
*
* PreparingTrainingStack
*
*
* -
*
* DownloadingTrainingImage
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link SecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Contains a secondary status information from a training job.
*
* Status might be one of the following secondary statuses:
*
*
* - InProgress
* -
*
* -
*
* Starting
- Starting the training job.
*
*
* -
*
* Downloading
- An optional stage for algorithms that support File
training input
* mode. It indicates that data is being downloaded to the ML storage volumes.
*
*
* -
*
* Training
- Training is in progress.
*
*
* -
*
* Uploading
- Training is complete and the model artifacts are being uploaded to the S3
* location.
*
*
*
*
* - Completed
* -
*
* -
*
* Completed
- The training job has completed.
*
*
*
*
* - Failed
* -
*
* -
*
* Failed
- The training job has failed. The reason for the failure is returned in the
* FailureReason
field of DescribeTrainingJobResponse
.
*
*
*
*
* - Stopped
* -
*
* -
*
* MaxRuntimeExceeded
- The job stopped because it exceeded the maximum allowed runtime.
*
*
* -
*
* Stopped
- The training job has stopped.
*
*
*
*
* - Stopping
* -
*
* -
*
* Stopping
- Stopping the training job.
*
*
*
*
*
*
* We no longer support the following secondary statuses:
*
*
* -
*
* LaunchingMLInstances
*
*
* -
*
* PreparingTrainingStack
*
*
* -
*
* DownloadingTrainingImage
*
*
* @see SecondaryStatus
*/
public final SecondaryStatus status() {
return SecondaryStatus.fromValue(status);
}
/**
*
* Contains a secondary status information from a training job.
*
*
* Status might be one of the following secondary statuses:
*
*
* - InProgress
* -
*
* -
*
* Starting
- Starting the training job.
*
*
* -
*
* Downloading
- An optional stage for algorithms that support File
training input mode.
* It indicates that data is being downloaded to the ML storage volumes.
*
*
* -
*
* Training
- Training is in progress.
*
*
* -
*
* Uploading
- Training is complete and the model artifacts are being uploaded to the S3 location.
*
*
*
*
* - Completed
* -
*
* -
*
* Completed
- The training job has completed.
*
*
*
*
* - Failed
* -
*
* -
*
* Failed
- The training job has failed. The reason for the failure is returned in the
* FailureReason
field of DescribeTrainingJobResponse
.
*
*
*
*
* - Stopped
* -
*
* -
*
* MaxRuntimeExceeded
- The job stopped because it exceeded the maximum allowed runtime.
*
*
* -
*
* Stopped
- The training job has stopped.
*
*
*
*
* - Stopping
* -
*
* -
*
* Stopping
- Stopping the training job.
*
*
*
*
*
*
* We no longer support the following secondary statuses:
*
*
* -
*
* LaunchingMLInstances
*
*
* -
*
* PreparingTrainingStack
*
*
* -
*
* DownloadingTrainingImage
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link SecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Contains a secondary status information from a training job.
*
* Status might be one of the following secondary statuses:
*
*
* - InProgress
* -
*
* -
*
* Starting
- Starting the training job.
*
*
* -
*
* Downloading
- An optional stage for algorithms that support File
training input
* mode. It indicates that data is being downloaded to the ML storage volumes.
*
*
* -
*
* Training
- Training is in progress.
*
*
* -
*
* Uploading
- Training is complete and the model artifacts are being uploaded to the S3
* location.
*
*
*
*
* - Completed
* -
*
* -
*
* Completed
- The training job has completed.
*
*
*
*
* - Failed
* -
*
* -
*
* Failed
- The training job has failed. The reason for the failure is returned in the
* FailureReason
field of DescribeTrainingJobResponse
.
*
*
*
*
* - Stopped
* -
*
* -
*
* MaxRuntimeExceeded
- The job stopped because it exceeded the maximum allowed runtime.
*
*
* -
*
* Stopped
- The training job has stopped.
*
*
*
*
* - Stopping
* -
*
* -
*
* Stopping
- Stopping the training job.
*
*
*
*
*
*
* We no longer support the following secondary statuses:
*
*
* -
*
* LaunchingMLInstances
*
*
* -
*
* PreparingTrainingStack
*
*
* -
*
* DownloadingTrainingImage
*
*
* @see SecondaryStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* A timestamp that shows when the training job transitioned to the current secondary status state.
*
*
* @return A timestamp that shows when the training job transitioned to the current secondary status state.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* A timestamp that shows when the training job transitioned out of this secondary status state into another
* secondary status state or when the training job has ended.
*
*
* @return A timestamp that shows when the training job transitioned out of this secondary status state into another
* secondary status state or when the training job has ended.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* A detailed description of the progress within a secondary status.
*
*
* Amazon SageMaker provides secondary statuses and status messages that apply to each of them:
*
*
* - Starting
* -
*
* -
*
* Starting the training job.
*
*
* -
*
* Launching requested ML instances.
*
*
* -
*
* Insufficient capacity error from EC2 while launching instances, retrying!
*
*
* -
*
* Launched instance was unhealthy, replacing it!
*
*
* -
*
* Preparing the instances for training.
*
*
*
*
* - Training
* -
*
* -
*
* Downloading the training image.
*
*
* -
*
* Training image download completed. Training in progress.
*
*
*
*
*
*
*
* Status messages are subject to change. Therefore, we recommend not including them in code that programmatically
* initiates actions. For examples, don't use status messages in if statements.
*
*
*
* To have an overview of your training job's progress, view TrainingJobStatus
and
* SecondaryStatus
in DescribeTrainingJob, and StatusMessage
together. For example,
* at the start of a training job, you might see the following:
*
*
* -
*
* TrainingJobStatus
- InProgress
*
*
* -
*
* SecondaryStatus
- Training
*
*
* -
*
* StatusMessage
- Downloading the training image
*
*
*
*
* @return A detailed description of the progress within a secondary status.
*
* Amazon SageMaker provides secondary statuses and status messages that apply to each of them:
*
*
* - Starting
* -
*
* -
*
* Starting the training job.
*
*
* -
*
* Launching requested ML instances.
*
*
* -
*
* Insufficient capacity error from EC2 while launching instances, retrying!
*
*
* -
*
* Launched instance was unhealthy, replacing it!
*
*
* -
*
* Preparing the instances for training.
*
*
*
*
* - Training
* -
*
* -
*
* Downloading the training image.
*
*
* -
*
* Training image download completed. Training in progress.
*
*
*
*
*
*
*
* Status messages are subject to change. Therefore, we recommend not including them in code that
* programmatically initiates actions. For examples, don't use status messages in if statements.
*
*
*
* To have an overview of your training job's progress, view TrainingJobStatus
and
* SecondaryStatus
in DescribeTrainingJob, and StatusMessage
together. For
* example, at the start of a training job, you might see the following:
*
*
* -
*
* TrainingJobStatus
- InProgress
*
*
* -
*
* SecondaryStatus
- Training
*
*
* -
*
* StatusMessage
- Downloading the training image
*
*
*/
public final String statusMessage() {
return statusMessage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SecondaryStatusTransition)) {
return false;
}
SecondaryStatusTransition other = (SecondaryStatusTransition) obj;
return Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(statusMessage(), other.statusMessage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SecondaryStatusTransition").add("Status", statusAsString()).add("StartTime", startTime())
.add("EndTime", endTime()).add("StatusMessage", statusMessage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function