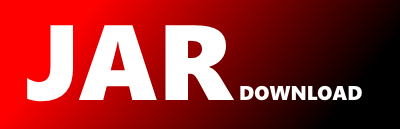
software.amazon.awssdk.services.sagemaker.model.ClarifyCheckStepMetadata Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The container for the metadata for the ClarifyCheck step. For more information, see the topic on ClarifyCheck step in the Amazon SageMaker Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ClarifyCheckStepMetadata implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CHECK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CheckType").getter(getter(ClarifyCheckStepMetadata::checkType)).setter(setter(Builder::checkType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CheckType").build()).build();
private static final SdkField BASELINE_USED_FOR_DRIFT_CHECK_CONSTRAINTS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("BaselineUsedForDriftCheckConstraints")
.getter(getter(ClarifyCheckStepMetadata::baselineUsedForDriftCheckConstraints))
.setter(setter(Builder::baselineUsedForDriftCheckConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("BaselineUsedForDriftCheckConstraints").build()).build();
private static final SdkField CALCULATED_BASELINE_CONSTRAINTS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CalculatedBaselineConstraints")
.getter(getter(ClarifyCheckStepMetadata::calculatedBaselineConstraints))
.setter(setter(Builder::calculatedBaselineConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CalculatedBaselineConstraints")
.build()).build();
private static final SdkField MODEL_PACKAGE_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelPackageGroupName").getter(getter(ClarifyCheckStepMetadata::modelPackageGroupName))
.setter(setter(Builder::modelPackageGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelPackageGroupName").build())
.build();
private static final SdkField VIOLATION_REPORT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ViolationReport").getter(getter(ClarifyCheckStepMetadata::violationReport))
.setter(setter(Builder::violationReport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViolationReport").build()).build();
private static final SdkField CHECK_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CheckJobArn").getter(getter(ClarifyCheckStepMetadata::checkJobArn)).setter(setter(Builder::checkJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CheckJobArn").build()).build();
private static final SdkField SKIP_CHECK_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("SkipCheck").getter(getter(ClarifyCheckStepMetadata::skipCheck)).setter(setter(Builder::skipCheck))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SkipCheck").build()).build();
private static final SdkField REGISTER_NEW_BASELINE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RegisterNewBaseline").getter(getter(ClarifyCheckStepMetadata::registerNewBaseline))
.setter(setter(Builder::registerNewBaseline))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegisterNewBaseline").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHECK_TYPE_FIELD,
BASELINE_USED_FOR_DRIFT_CHECK_CONSTRAINTS_FIELD, CALCULATED_BASELINE_CONSTRAINTS_FIELD,
MODEL_PACKAGE_GROUP_NAME_FIELD, VIOLATION_REPORT_FIELD, CHECK_JOB_ARN_FIELD, SKIP_CHECK_FIELD,
REGISTER_NEW_BASELINE_FIELD));
private static final long serialVersionUID = 1L;
private final String checkType;
private final String baselineUsedForDriftCheckConstraints;
private final String calculatedBaselineConstraints;
private final String modelPackageGroupName;
private final String violationReport;
private final String checkJobArn;
private final Boolean skipCheck;
private final Boolean registerNewBaseline;
private ClarifyCheckStepMetadata(BuilderImpl builder) {
this.checkType = builder.checkType;
this.baselineUsedForDriftCheckConstraints = builder.baselineUsedForDriftCheckConstraints;
this.calculatedBaselineConstraints = builder.calculatedBaselineConstraints;
this.modelPackageGroupName = builder.modelPackageGroupName;
this.violationReport = builder.violationReport;
this.checkJobArn = builder.checkJobArn;
this.skipCheck = builder.skipCheck;
this.registerNewBaseline = builder.registerNewBaseline;
}
/**
*
* The type of the Clarify Check step
*
*
* @return The type of the Clarify Check step
*/
public final String checkType() {
return checkType;
}
/**
*
* The Amazon S3 URI of baseline constraints file to be used for the drift check.
*
*
* @return The Amazon S3 URI of baseline constraints file to be used for the drift check.
*/
public final String baselineUsedForDriftCheckConstraints() {
return baselineUsedForDriftCheckConstraints;
}
/**
*
* The Amazon S3 URI of the newly calculated baseline constraints file.
*
*
* @return The Amazon S3 URI of the newly calculated baseline constraints file.
*/
public final String calculatedBaselineConstraints() {
return calculatedBaselineConstraints;
}
/**
*
* The model package group name.
*
*
* @return The model package group name.
*/
public final String modelPackageGroupName() {
return modelPackageGroupName;
}
/**
*
* The Amazon S3 URI of the violation report if violations are detected.
*
*
* @return The Amazon S3 URI of the violation report if violations are detected.
*/
public final String violationReport() {
return violationReport;
}
/**
*
* The Amazon Resource Name (ARN) of the check processing job that was run by this step's execution.
*
*
* @return The Amazon Resource Name (ARN) of the check processing job that was run by this step's execution.
*/
public final String checkJobArn() {
return checkJobArn;
}
/**
*
* This flag indicates if the drift check against the previous baseline will be skipped or not. If it is set to
* False
, the previous baseline of the configured check type must be available.
*
*
* @return This flag indicates if the drift check against the previous baseline will be skipped or not. If it is set
* to False
, the previous baseline of the configured check type must be available.
*/
public final Boolean skipCheck() {
return skipCheck;
}
/**
*
* This flag indicates if a newly calculated baseline can be accessed through step properties
* BaselineUsedForDriftCheckConstraints
and BaselineUsedForDriftCheckStatistics
. If it is
* set to False
, the previous baseline of the configured check type must also be available. These can
* be accessed through the BaselineUsedForDriftCheckConstraints
property.
*
*
* @return This flag indicates if a newly calculated baseline can be accessed through step properties
* BaselineUsedForDriftCheckConstraints
and BaselineUsedForDriftCheckStatistics
.
* If it is set to False
, the previous baseline of the configured check type must also be
* available. These can be accessed through the BaselineUsedForDriftCheckConstraints
property.
*/
public final Boolean registerNewBaseline() {
return registerNewBaseline;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(checkType());
hashCode = 31 * hashCode + Objects.hashCode(baselineUsedForDriftCheckConstraints());
hashCode = 31 * hashCode + Objects.hashCode(calculatedBaselineConstraints());
hashCode = 31 * hashCode + Objects.hashCode(modelPackageGroupName());
hashCode = 31 * hashCode + Objects.hashCode(violationReport());
hashCode = 31 * hashCode + Objects.hashCode(checkJobArn());
hashCode = 31 * hashCode + Objects.hashCode(skipCheck());
hashCode = 31 * hashCode + Objects.hashCode(registerNewBaseline());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ClarifyCheckStepMetadata)) {
return false;
}
ClarifyCheckStepMetadata other = (ClarifyCheckStepMetadata) obj;
return Objects.equals(checkType(), other.checkType())
&& Objects.equals(baselineUsedForDriftCheckConstraints(), other.baselineUsedForDriftCheckConstraints())
&& Objects.equals(calculatedBaselineConstraints(), other.calculatedBaselineConstraints())
&& Objects.equals(modelPackageGroupName(), other.modelPackageGroupName())
&& Objects.equals(violationReport(), other.violationReport())
&& Objects.equals(checkJobArn(), other.checkJobArn()) && Objects.equals(skipCheck(), other.skipCheck())
&& Objects.equals(registerNewBaseline(), other.registerNewBaseline());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ClarifyCheckStepMetadata").add("CheckType", checkType())
.add("BaselineUsedForDriftCheckConstraints", baselineUsedForDriftCheckConstraints())
.add("CalculatedBaselineConstraints", calculatedBaselineConstraints())
.add("ModelPackageGroupName", modelPackageGroupName()).add("ViolationReport", violationReport())
.add("CheckJobArn", checkJobArn()).add("SkipCheck", skipCheck())
.add("RegisterNewBaseline", registerNewBaseline()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CheckType":
return Optional.ofNullable(clazz.cast(checkType()));
case "BaselineUsedForDriftCheckConstraints":
return Optional.ofNullable(clazz.cast(baselineUsedForDriftCheckConstraints()));
case "CalculatedBaselineConstraints":
return Optional.ofNullable(clazz.cast(calculatedBaselineConstraints()));
case "ModelPackageGroupName":
return Optional.ofNullable(clazz.cast(modelPackageGroupName()));
case "ViolationReport":
return Optional.ofNullable(clazz.cast(violationReport()));
case "CheckJobArn":
return Optional.ofNullable(clazz.cast(checkJobArn()));
case "SkipCheck":
return Optional.ofNullable(clazz.cast(skipCheck()));
case "RegisterNewBaseline":
return Optional.ofNullable(clazz.cast(registerNewBaseline()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function