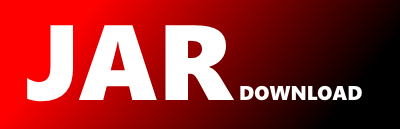
software.amazon.awssdk.services.sagemaker.model.PipelineExecutionStepMetadata Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Metadata for a step execution.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PipelineExecutionStepMetadata implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TRAINING_JOB_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TrainingJob")
.getter(getter(PipelineExecutionStepMetadata::trainingJob)).setter(setter(Builder::trainingJob))
.constructor(TrainingJobStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrainingJob").build()).build();
private static final SdkField PROCESSING_JOB_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ProcessingJob")
.getter(getter(PipelineExecutionStepMetadata::processingJob)).setter(setter(Builder::processingJob))
.constructor(ProcessingJobStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProcessingJob").build()).build();
private static final SdkField TRANSFORM_JOB_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TransformJob")
.getter(getter(PipelineExecutionStepMetadata::transformJob)).setter(setter(Builder::transformJob))
.constructor(TransformJobStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransformJob").build()).build();
private static final SdkField TUNING_JOB_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TuningJob")
.getter(getter(PipelineExecutionStepMetadata::tuningJob)).setter(setter(Builder::tuningJob))
.constructor(TuningJobStepMetaData::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TuningJob").build()).build();
private static final SdkField MODEL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Model").getter(getter(PipelineExecutionStepMetadata::model)).setter(setter(Builder::model))
.constructor(ModelStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Model").build()).build();
private static final SdkField REGISTER_MODEL_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RegisterModel")
.getter(getter(PipelineExecutionStepMetadata::registerModel)).setter(setter(Builder::registerModel))
.constructor(RegisterModelStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegisterModel").build()).build();
private static final SdkField CONDITION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Condition")
.getter(getter(PipelineExecutionStepMetadata::condition)).setter(setter(Builder::condition))
.constructor(ConditionStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Condition").build()).build();
private static final SdkField CALLBACK_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Callback")
.getter(getter(PipelineExecutionStepMetadata::callback)).setter(setter(Builder::callback))
.constructor(CallbackStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Callback").build()).build();
private static final SdkField LAMBDA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Lambda")
.getter(getter(PipelineExecutionStepMetadata::lambda)).setter(setter(Builder::lambda))
.constructor(LambdaStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Lambda").build()).build();
private static final SdkField QUALITY_CHECK_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("QualityCheck")
.getter(getter(PipelineExecutionStepMetadata::qualityCheck)).setter(setter(Builder::qualityCheck))
.constructor(QualityCheckStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QualityCheck").build()).build();
private static final SdkField CLARIFY_CHECK_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ClarifyCheck")
.getter(getter(PipelineExecutionStepMetadata::clarifyCheck)).setter(setter(Builder::clarifyCheck))
.constructor(ClarifyCheckStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClarifyCheck").build()).build();
private static final SdkField EMR_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("EMR").getter(getter(PipelineExecutionStepMetadata::emr)).setter(setter(Builder::emr))
.constructor(EMRStepMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EMR").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TRAINING_JOB_FIELD,
PROCESSING_JOB_FIELD, TRANSFORM_JOB_FIELD, TUNING_JOB_FIELD, MODEL_FIELD, REGISTER_MODEL_FIELD, CONDITION_FIELD,
CALLBACK_FIELD, LAMBDA_FIELD, QUALITY_CHECK_FIELD, CLARIFY_CHECK_FIELD, EMR_FIELD));
private static final long serialVersionUID = 1L;
private final TrainingJobStepMetadata trainingJob;
private final ProcessingJobStepMetadata processingJob;
private final TransformJobStepMetadata transformJob;
private final TuningJobStepMetaData tuningJob;
private final ModelStepMetadata model;
private final RegisterModelStepMetadata registerModel;
private final ConditionStepMetadata condition;
private final CallbackStepMetadata callback;
private final LambdaStepMetadata lambda;
private final QualityCheckStepMetadata qualityCheck;
private final ClarifyCheckStepMetadata clarifyCheck;
private final EMRStepMetadata emr;
private PipelineExecutionStepMetadata(BuilderImpl builder) {
this.trainingJob = builder.trainingJob;
this.processingJob = builder.processingJob;
this.transformJob = builder.transformJob;
this.tuningJob = builder.tuningJob;
this.model = builder.model;
this.registerModel = builder.registerModel;
this.condition = builder.condition;
this.callback = builder.callback;
this.lambda = builder.lambda;
this.qualityCheck = builder.qualityCheck;
this.clarifyCheck = builder.clarifyCheck;
this.emr = builder.emr;
}
/**
*
* The Amazon Resource Name (ARN) of the training job that was run by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the training job that was run by this step execution.
*/
public final TrainingJobStepMetadata trainingJob() {
return trainingJob;
}
/**
*
* The Amazon Resource Name (ARN) of the processing job that was run by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the processing job that was run by this step execution.
*/
public final ProcessingJobStepMetadata processingJob() {
return processingJob;
}
/**
*
* The Amazon Resource Name (ARN) of the transform job that was run by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the transform job that was run by this step execution.
*/
public final TransformJobStepMetadata transformJob() {
return transformJob;
}
/**
*
* The Amazon Resource Name (ARN) of the tuning job that was run by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the tuning job that was run by this step execution.
*/
public final TuningJobStepMetaData tuningJob() {
return tuningJob;
}
/**
*
* The Amazon Resource Name (ARN) of the model that was created by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the model that was created by this step execution.
*/
public final ModelStepMetadata model() {
return model;
}
/**
*
* The Amazon Resource Name (ARN) of the model package the model was registered to by this step execution.
*
*
* @return The Amazon Resource Name (ARN) of the model package the model was registered to by this step execution.
*/
public final RegisterModelStepMetadata registerModel() {
return registerModel;
}
/**
*
* The outcome of the condition evaluation that was run by this step execution.
*
*
* @return The outcome of the condition evaluation that was run by this step execution.
*/
public final ConditionStepMetadata condition() {
return condition;
}
/**
*
* The URL of the Amazon SQS queue used by this step execution, the pipeline generated token, and a list of output
* parameters.
*
*
* @return The URL of the Amazon SQS queue used by this step execution, the pipeline generated token, and a list of
* output parameters.
*/
public final CallbackStepMetadata callback() {
return callback;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda function that was run by this step execution and a list of output
* parameters.
*
*
* @return The Amazon Resource Name (ARN) of the Lambda function that was run by this step execution and a list of
* output parameters.
*/
public final LambdaStepMetadata lambda() {
return lambda;
}
/**
*
* The configurations and outcomes of the check step execution. This includes:
*
*
* -
*
* The type of the check conducted,
*
*
* -
*
* The Amazon S3 URIs of baseline constraints and statistics files to be used for the drift check.
*
*
* -
*
* The Amazon S3 URIs of newly calculated baseline constraints and statistics.
*
*
* -
*
* The model package group name provided.
*
*
* -
*
* The Amazon S3 URI of the violation report if violations detected.
*
*
* -
*
* The Amazon Resource Name (ARN) of check processing job initiated by the step execution.
*
*
* -
*
* The boolean flags indicating if the drift check is skipped.
*
*
* -
*
* If step property BaselineUsedForDriftCheck
is set the same as CalculatedBaseline
.
*
*
*
*
* @return The configurations and outcomes of the check step execution. This includes:
*
* -
*
* The type of the check conducted,
*
*
* -
*
* The Amazon S3 URIs of baseline constraints and statistics files to be used for the drift check.
*
*
* -
*
* The Amazon S3 URIs of newly calculated baseline constraints and statistics.
*
*
* -
*
* The model package group name provided.
*
*
* -
*
* The Amazon S3 URI of the violation report if violations detected.
*
*
* -
*
* The Amazon Resource Name (ARN) of check processing job initiated by the step execution.
*
*
* -
*
* The boolean flags indicating if the drift check is skipped.
*
*
* -
*
* If step property BaselineUsedForDriftCheck
is set the same as
* CalculatedBaseline
.
*
*
*/
public final QualityCheckStepMetadata qualityCheck() {
return qualityCheck;
}
/**
*
* Container for the metadata for a Clarify check step. The configurations and outcomes of the check step execution.
* This includes:
*
*
* -
*
* The type of the check conducted,
*
*
* -
*
* The Amazon S3 URIs of baseline constraints and statistics files to be used for the drift check.
*
*
* -
*
* The Amazon S3 URIs of newly calculated baseline constraints and statistics.
*
*
* -
*
* The model package group name provided.
*
*
* -
*
* The Amazon S3 URI of the violation report if violations detected.
*
*
* -
*
* The Amazon Resource Name (ARN) of check processing job initiated by the step execution.
*
*
* -
*
* The boolean flags indicating if the drift check is skipped.
*
*
* -
*
* If step property BaselineUsedForDriftCheck
is set the same as CalculatedBaseline
.
*
*
*
*
* @return Container for the metadata for a Clarify check step. The configurations and outcomes of the check step
* execution. This includes:
*
* -
*
* The type of the check conducted,
*
*
* -
*
* The Amazon S3 URIs of baseline constraints and statistics files to be used for the drift check.
*
*
* -
*
* The Amazon S3 URIs of newly calculated baseline constraints and statistics.
*
*
* -
*
* The model package group name provided.
*
*
* -
*
* The Amazon S3 URI of the violation report if violations detected.
*
*
* -
*
* The Amazon Resource Name (ARN) of check processing job initiated by the step execution.
*
*
* -
*
* The boolean flags indicating if the drift check is skipped.
*
*
* -
*
* If step property BaselineUsedForDriftCheck
is set the same as
* CalculatedBaseline
.
*
*
*/
public final ClarifyCheckStepMetadata clarifyCheck() {
return clarifyCheck;
}
/**
*
* The configurations and outcomes of an EMR step execution.
*
*
* @return The configurations and outcomes of an EMR step execution.
*/
public final EMRStepMetadata emr() {
return emr;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(trainingJob());
hashCode = 31 * hashCode + Objects.hashCode(processingJob());
hashCode = 31 * hashCode + Objects.hashCode(transformJob());
hashCode = 31 * hashCode + Objects.hashCode(tuningJob());
hashCode = 31 * hashCode + Objects.hashCode(model());
hashCode = 31 * hashCode + Objects.hashCode(registerModel());
hashCode = 31 * hashCode + Objects.hashCode(condition());
hashCode = 31 * hashCode + Objects.hashCode(callback());
hashCode = 31 * hashCode + Objects.hashCode(lambda());
hashCode = 31 * hashCode + Objects.hashCode(qualityCheck());
hashCode = 31 * hashCode + Objects.hashCode(clarifyCheck());
hashCode = 31 * hashCode + Objects.hashCode(emr());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PipelineExecutionStepMetadata)) {
return false;
}
PipelineExecutionStepMetadata other = (PipelineExecutionStepMetadata) obj;
return Objects.equals(trainingJob(), other.trainingJob()) && Objects.equals(processingJob(), other.processingJob())
&& Objects.equals(transformJob(), other.transformJob()) && Objects.equals(tuningJob(), other.tuningJob())
&& Objects.equals(model(), other.model()) && Objects.equals(registerModel(), other.registerModel())
&& Objects.equals(condition(), other.condition()) && Objects.equals(callback(), other.callback())
&& Objects.equals(lambda(), other.lambda()) && Objects.equals(qualityCheck(), other.qualityCheck())
&& Objects.equals(clarifyCheck(), other.clarifyCheck()) && Objects.equals(emr(), other.emr());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PipelineExecutionStepMetadata").add("TrainingJob", trainingJob())
.add("ProcessingJob", processingJob()).add("TransformJob", transformJob()).add("TuningJob", tuningJob())
.add("Model", model()).add("RegisterModel", registerModel()).add("Condition", condition())
.add("Callback", callback()).add("Lambda", lambda()).add("QualityCheck", qualityCheck())
.add("ClarifyCheck", clarifyCheck()).add("EMR", emr()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TrainingJob":
return Optional.ofNullable(clazz.cast(trainingJob()));
case "ProcessingJob":
return Optional.ofNullable(clazz.cast(processingJob()));
case "TransformJob":
return Optional.ofNullable(clazz.cast(transformJob()));
case "TuningJob":
return Optional.ofNullable(clazz.cast(tuningJob()));
case "Model":
return Optional.ofNullable(clazz.cast(model()));
case "RegisterModel":
return Optional.ofNullable(clazz.cast(registerModel()));
case "Condition":
return Optional.ofNullable(clazz.cast(condition()));
case "Callback":
return Optional.ofNullable(clazz.cast(callback()));
case "Lambda":
return Optional.ofNullable(clazz.cast(lambda()));
case "QualityCheck":
return Optional.ofNullable(clazz.cast(qualityCheck()));
case "ClarifyCheck":
return Optional.ofNullable(clazz.cast(clarifyCheck()));
case "EMR":
return Optional.ofNullable(clazz.cast(emr()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function