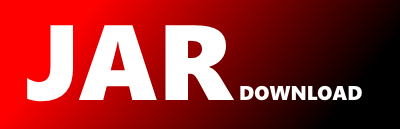
software.amazon.awssdk.services.sagemaker.model.Workteam Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides details about a labeling work team.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Workteam implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField WORKTEAM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkteamName").getter(getter(Workteam::workteamName)).setter(setter(Builder::workteamName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkteamName").build()).build();
private static final SdkField> MEMBER_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MemberDefinitions")
.getter(getter(Workteam::memberDefinitions))
.setter(setter(Builder::memberDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MemberDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField WORKTEAM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkteamArn").getter(getter(Workteam::workteamArn)).setter(setter(Builder::workteamArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkteamArn").build()).build();
private static final SdkField WORKFORCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkforceArn").getter(getter(Workteam::workforceArn)).setter(setter(Builder::workforceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkforceArn").build()).build();
private static final SdkField> PRODUCT_LISTING_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ProductListingIds")
.getter(getter(Workteam::productListingIds))
.setter(setter(Builder::productListingIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductListingIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Workteam::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField SUB_DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubDomain").getter(getter(Workteam::subDomain)).setter(setter(Builder::subDomain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubDomain").build()).build();
private static final SdkField CREATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateDate").getter(getter(Workteam::createDate)).setter(setter(Builder::createDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateDate").build()).build();
private static final SdkField LAST_UPDATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdatedDate").getter(getter(Workteam::lastUpdatedDate)).setter(setter(Builder::lastUpdatedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedDate").build()).build();
private static final SdkField NOTIFICATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("NotificationConfiguration")
.getter(getter(Workteam::notificationConfiguration)).setter(setter(Builder::notificationConfiguration))
.constructor(NotificationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKTEAM_NAME_FIELD,
MEMBER_DEFINITIONS_FIELD, WORKTEAM_ARN_FIELD, WORKFORCE_ARN_FIELD, PRODUCT_LISTING_IDS_FIELD, DESCRIPTION_FIELD,
SUB_DOMAIN_FIELD, CREATE_DATE_FIELD, LAST_UPDATED_DATE_FIELD, NOTIFICATION_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String workteamName;
private final List memberDefinitions;
private final String workteamArn;
private final String workforceArn;
private final List productListingIds;
private final String description;
private final String subDomain;
private final Instant createDate;
private final Instant lastUpdatedDate;
private final NotificationConfiguration notificationConfiguration;
private Workteam(BuilderImpl builder) {
this.workteamName = builder.workteamName;
this.memberDefinitions = builder.memberDefinitions;
this.workteamArn = builder.workteamArn;
this.workforceArn = builder.workforceArn;
this.productListingIds = builder.productListingIds;
this.description = builder.description;
this.subDomain = builder.subDomain;
this.createDate = builder.createDate;
this.lastUpdatedDate = builder.lastUpdatedDate;
this.notificationConfiguration = builder.notificationConfiguration;
}
/**
*
* The name of the work team.
*
*
* @return The name of the work team.
*/
public final String workteamName() {
return workteamName;
}
/**
* For responses, this returns true if the service returned a value for the MemberDefinitions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMemberDefinitions() {
return memberDefinitions != null && !(memberDefinitions instanceof SdkAutoConstructList);
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMemberDefinitions} method.
*
*
* @return A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
.
*/
public final List memberDefinitions() {
return memberDefinitions;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the work team.
*
*
* @return The Amazon Resource Name (ARN) that identifies the work team.
*/
public final String workteamArn() {
return workteamArn;
}
/**
*
* The Amazon Resource Name (ARN) of the workforce.
*
*
* @return The Amazon Resource Name (ARN) of the workforce.
*/
public final String workforceArn() {
return workforceArn;
}
/**
* For responses, this returns true if the service returned a value for the ProductListingIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasProductListingIds() {
return productListingIds != null && !(productListingIds instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Marketplace identifier for a vendor's work team.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasProductListingIds} method.
*
*
* @return The Amazon Marketplace identifier for a vendor's work team.
*/
public final List productListingIds() {
return productListingIds;
}
/**
*
* A description of the work team.
*
*
* @return A description of the work team.
*/
public final String description() {
return description;
}
/**
*
* The URI of the labeling job's user interface. Workers open this URI to start labeling your data objects.
*
*
* @return The URI of the labeling job's user interface. Workers open this URI to start labeling your data objects.
*/
public final String subDomain() {
return subDomain;
}
/**
*
* The date and time that the work team was created (timestamp).
*
*
* @return The date and time that the work team was created (timestamp).
*/
public final Instant createDate() {
return createDate;
}
/**
*
* The date and time that the work team was last updated (timestamp).
*
*
* @return The date and time that the work team was last updated (timestamp).
*/
public final Instant lastUpdatedDate() {
return lastUpdatedDate;
}
/**
*
* Configures SNS notifications of available or expiring work items for work teams.
*
*
* @return Configures SNS notifications of available or expiring work items for work teams.
*/
public final NotificationConfiguration notificationConfiguration() {
return notificationConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(workteamName());
hashCode = 31 * hashCode + Objects.hashCode(hasMemberDefinitions() ? memberDefinitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(workteamArn());
hashCode = 31 * hashCode + Objects.hashCode(workforceArn());
hashCode = 31 * hashCode + Objects.hashCode(hasProductListingIds() ? productListingIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(subDomain());
hashCode = 31 * hashCode + Objects.hashCode(createDate());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedDate());
hashCode = 31 * hashCode + Objects.hashCode(notificationConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Workteam)) {
return false;
}
Workteam other = (Workteam) obj;
return Objects.equals(workteamName(), other.workteamName()) && hasMemberDefinitions() == other.hasMemberDefinitions()
&& Objects.equals(memberDefinitions(), other.memberDefinitions())
&& Objects.equals(workteamArn(), other.workteamArn()) && Objects.equals(workforceArn(), other.workforceArn())
&& hasProductListingIds() == other.hasProductListingIds()
&& Objects.equals(productListingIds(), other.productListingIds())
&& Objects.equals(description(), other.description()) && Objects.equals(subDomain(), other.subDomain())
&& Objects.equals(createDate(), other.createDate()) && Objects.equals(lastUpdatedDate(), other.lastUpdatedDate())
&& Objects.equals(notificationConfiguration(), other.notificationConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Workteam").add("WorkteamName", workteamName())
.add("MemberDefinitions", hasMemberDefinitions() ? memberDefinitions() : null).add("WorkteamArn", workteamArn())
.add("WorkforceArn", workforceArn())
.add("ProductListingIds", hasProductListingIds() ? productListingIds() : null).add("Description", description())
.add("SubDomain", subDomain()).add("CreateDate", createDate()).add("LastUpdatedDate", lastUpdatedDate())
.add("NotificationConfiguration", notificationConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "WorkteamName":
return Optional.ofNullable(clazz.cast(workteamName()));
case "MemberDefinitions":
return Optional.ofNullable(clazz.cast(memberDefinitions()));
case "WorkteamArn":
return Optional.ofNullable(clazz.cast(workteamArn()));
case "WorkforceArn":
return Optional.ofNullable(clazz.cast(workforceArn()));
case "ProductListingIds":
return Optional.ofNullable(clazz.cast(productListingIds()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "SubDomain":
return Optional.ofNullable(clazz.cast(subDomain()));
case "CreateDate":
return Optional.ofNullable(clazz.cast(createDate()));
case "LastUpdatedDate":
return Optional.ofNullable(clazz.cast(lastUpdatedDate()));
case "NotificationConfiguration":
return Optional.ofNullable(clazz.cast(notificationConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces
* created using your own OIDC identity provider (IdP) use OidcMemberDefinition
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder memberDefinitions(Collection memberDefinitions);
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up
* the work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
.
*
*
* @param memberDefinitions
* A list of MemberDefinition
objects that contains objects that identify the workers that
* make up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces
* created using your own OIDC identity provider (IdP) use OidcMemberDefinition
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder memberDefinitions(MemberDefinition... memberDefinitions);
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up
* the work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #memberDefinitions(List)}.
*
* @param memberDefinitions
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #memberDefinitions(List)
*/
Builder memberDefinitions(Consumer... memberDefinitions);
/**
*
* The Amazon Resource Name (ARN) that identifies the work team.
*
*
* @param workteamArn
* The Amazon Resource Name (ARN) that identifies the work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder workteamArn(String workteamArn);
/**
*
* The Amazon Resource Name (ARN) of the workforce.
*
*
* @param workforceArn
* The Amazon Resource Name (ARN) of the workforce.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder workforceArn(String workforceArn);
/**
*
* The Amazon Marketplace identifier for a vendor's work team.
*
*
* @param productListingIds
* The Amazon Marketplace identifier for a vendor's work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder productListingIds(Collection productListingIds);
/**
*
* The Amazon Marketplace identifier for a vendor's work team.
*
*
* @param productListingIds
* The Amazon Marketplace identifier for a vendor's work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder productListingIds(String... productListingIds);
/**
*
* A description of the work team.
*
*
* @param description
* A description of the work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
*
* The URI of the labeling job's user interface. Workers open this URI to start labeling your data objects.
*
*
* @param subDomain
* The URI of the labeling job's user interface. Workers open this URI to start labeling your data
* objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder subDomain(String subDomain);
/**
*
* The date and time that the work team was created (timestamp).
*
*
* @param createDate
* The date and time that the work team was created (timestamp).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder createDate(Instant createDate);
/**
*
* The date and time that the work team was last updated (timestamp).
*
*
* @param lastUpdatedDate
* The date and time that the work team was last updated (timestamp).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastUpdatedDate(Instant lastUpdatedDate);
/**
*
* Configures SNS notifications of available or expiring work items for work teams.
*
*
* @param notificationConfiguration
* Configures SNS notifications of available or expiring work items for work teams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationConfiguration(NotificationConfiguration notificationConfiguration);
/**
*
* Configures SNS notifications of available or expiring work items for work teams.
*
* This is a convenience that creates an instance of the {@link NotificationConfiguration.Builder} avoiding the
* need to create one manually via {@link NotificationConfiguration#builder()}.
*
* When the {@link Consumer} completes, {@link NotificationConfiguration.Builder#build()} is called immediately
* and its result is passed to {@link #notificationConfiguration(NotificationConfiguration)}.
*
* @param notificationConfiguration
* a consumer that will call methods on {@link NotificationConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #notificationConfiguration(NotificationConfiguration)
*/
default Builder notificationConfiguration(Consumer notificationConfiguration) {
return notificationConfiguration(NotificationConfiguration.builder().applyMutation(notificationConfiguration).build());
}
}
static final class BuilderImpl implements Builder {
private String workteamName;
private List memberDefinitions = DefaultSdkAutoConstructList.getInstance();
private String workteamArn;
private String workforceArn;
private List productListingIds = DefaultSdkAutoConstructList.getInstance();
private String description;
private String subDomain;
private Instant createDate;
private Instant lastUpdatedDate;
private NotificationConfiguration notificationConfiguration;
private BuilderImpl() {
}
private BuilderImpl(Workteam model) {
workteamName(model.workteamName);
memberDefinitions(model.memberDefinitions);
workteamArn(model.workteamArn);
workforceArn(model.workforceArn);
productListingIds(model.productListingIds);
description(model.description);
subDomain(model.subDomain);
createDate(model.createDate);
lastUpdatedDate(model.lastUpdatedDate);
notificationConfiguration(model.notificationConfiguration);
}
public final String getWorkteamName() {
return workteamName;
}
public final void setWorkteamName(String workteamName) {
this.workteamName = workteamName;
}
@Override
@Transient
public final Builder workteamName(String workteamName) {
this.workteamName = workteamName;
return this;
}
public final List getMemberDefinitions() {
List result = MemberDefinitionsCopier.copyToBuilder(this.memberDefinitions);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setMemberDefinitions(Collection memberDefinitions) {
this.memberDefinitions = MemberDefinitionsCopier.copyFromBuilder(memberDefinitions);
}
@Override
@Transient
public final Builder memberDefinitions(Collection memberDefinitions) {
this.memberDefinitions = MemberDefinitionsCopier.copy(memberDefinitions);
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder memberDefinitions(MemberDefinition... memberDefinitions) {
memberDefinitions(Arrays.asList(memberDefinitions));
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder memberDefinitions(Consumer... memberDefinitions) {
memberDefinitions(Stream.of(memberDefinitions).map(c -> MemberDefinition.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getWorkteamArn() {
return workteamArn;
}
public final void setWorkteamArn(String workteamArn) {
this.workteamArn = workteamArn;
}
@Override
@Transient
public final Builder workteamArn(String workteamArn) {
this.workteamArn = workteamArn;
return this;
}
public final String getWorkforceArn() {
return workforceArn;
}
public final void setWorkforceArn(String workforceArn) {
this.workforceArn = workforceArn;
}
@Override
@Transient
public final Builder workforceArn(String workforceArn) {
this.workforceArn = workforceArn;
return this;
}
public final Collection getProductListingIds() {
if (productListingIds instanceof SdkAutoConstructList) {
return null;
}
return productListingIds;
}
public final void setProductListingIds(Collection productListingIds) {
this.productListingIds = ProductListingsCopier.copy(productListingIds);
}
@Override
@Transient
public final Builder productListingIds(Collection productListingIds) {
this.productListingIds = ProductListingsCopier.copy(productListingIds);
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder productListingIds(String... productListingIds) {
productListingIds(Arrays.asList(productListingIds));
return this;
}
public final String getDescription() {
return description;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
@Transient
public final Builder description(String description) {
this.description = description;
return this;
}
public final String getSubDomain() {
return subDomain;
}
public final void setSubDomain(String subDomain) {
this.subDomain = subDomain;
}
@Override
@Transient
public final Builder subDomain(String subDomain) {
this.subDomain = subDomain;
return this;
}
public final Instant getCreateDate() {
return createDate;
}
public final void setCreateDate(Instant createDate) {
this.createDate = createDate;
}
@Override
@Transient
public final Builder createDate(Instant createDate) {
this.createDate = createDate;
return this;
}
public final Instant getLastUpdatedDate() {
return lastUpdatedDate;
}
public final void setLastUpdatedDate(Instant lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
@Override
@Transient
public final Builder lastUpdatedDate(Instant lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
public final NotificationConfiguration.Builder getNotificationConfiguration() {
return notificationConfiguration != null ? notificationConfiguration.toBuilder() : null;
}
public final void setNotificationConfiguration(NotificationConfiguration.BuilderImpl notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration != null ? notificationConfiguration.build() : null;
}
@Override
@Transient
public final Builder notificationConfiguration(NotificationConfiguration notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration;
return this;
}
@Override
public Workteam build() {
return new Workteam(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}