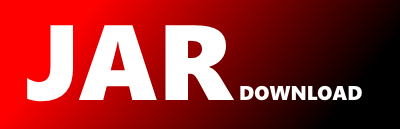
software.amazon.awssdk.services.sagemaker.model.AutoMLJobSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides a summary about an AutoML job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AutoMLJobSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AUTO_ML_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobName").getter(getter(AutoMLJobSummary::autoMLJobName)).setter(setter(Builder::autoMLJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobName").build()).build();
private static final SdkField AUTO_ML_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobArn").getter(getter(AutoMLJobSummary::autoMLJobArn)).setter(setter(Builder::autoMLJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobArn").build()).build();
private static final SdkField AUTO_ML_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobStatus").getter(getter(AutoMLJobSummary::autoMLJobStatusAsString))
.setter(setter(Builder::autoMLJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobStatus").build()).build();
private static final SdkField AUTO_ML_JOB_SECONDARY_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobSecondaryStatus").getter(getter(AutoMLJobSummary::autoMLJobSecondaryStatusAsString))
.setter(setter(Builder::autoMLJobSecondaryStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobSecondaryStatus").build())
.build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(AutoMLJobSummary::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(AutoMLJobSummary::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(AutoMLJobSummary::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(AutoMLJobSummary::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField> PARTIAL_FAILURE_REASONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PartialFailureReasons")
.getter(getter(AutoMLJobSummary::partialFailureReasons))
.setter(setter(Builder::partialFailureReasons))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PartialFailureReasons").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoMLPartialFailureReason::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTO_ML_JOB_NAME_FIELD,
AUTO_ML_JOB_ARN_FIELD, AUTO_ML_JOB_STATUS_FIELD, AUTO_ML_JOB_SECONDARY_STATUS_FIELD, CREATION_TIME_FIELD,
END_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, FAILURE_REASON_FIELD, PARTIAL_FAILURE_REASONS_FIELD));
private static final long serialVersionUID = 1L;
private final String autoMLJobName;
private final String autoMLJobArn;
private final String autoMLJobStatus;
private final String autoMLJobSecondaryStatus;
private final Instant creationTime;
private final Instant endTime;
private final Instant lastModifiedTime;
private final String failureReason;
private final List partialFailureReasons;
private AutoMLJobSummary(BuilderImpl builder) {
this.autoMLJobName = builder.autoMLJobName;
this.autoMLJobArn = builder.autoMLJobArn;
this.autoMLJobStatus = builder.autoMLJobStatus;
this.autoMLJobSecondaryStatus = builder.autoMLJobSecondaryStatus;
this.creationTime = builder.creationTime;
this.endTime = builder.endTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.failureReason = builder.failureReason;
this.partialFailureReasons = builder.partialFailureReasons;
}
/**
*
* The name of the AutoML job you are requesting.
*
*
* @return The name of the AutoML job you are requesting.
*/
public final String autoMLJobName() {
return autoMLJobName;
}
/**
*
* The ARN of the AutoML job.
*
*
* @return The ARN of the AutoML job.
*/
public final String autoMLJobArn() {
return autoMLJobArn;
}
/**
*
* The status of the AutoML job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoMLJobStatus}
* will return {@link AutoMLJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoMLJobStatusAsString}.
*
*
* @return The status of the AutoML job.
* @see AutoMLJobStatus
*/
public final AutoMLJobStatus autoMLJobStatus() {
return AutoMLJobStatus.fromValue(autoMLJobStatus);
}
/**
*
* The status of the AutoML job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoMLJobStatus}
* will return {@link AutoMLJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoMLJobStatusAsString}.
*
*
* @return The status of the AutoML job.
* @see AutoMLJobStatus
*/
public final String autoMLJobStatusAsString() {
return autoMLJobStatus;
}
/**
*
* The secondary status of the AutoML job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLJobSecondaryStatus} will return {@link AutoMLJobSecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #autoMLJobSecondaryStatusAsString}.
*
*
* @return The secondary status of the AutoML job.
* @see AutoMLJobSecondaryStatus
*/
public final AutoMLJobSecondaryStatus autoMLJobSecondaryStatus() {
return AutoMLJobSecondaryStatus.fromValue(autoMLJobSecondaryStatus);
}
/**
*
* The secondary status of the AutoML job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLJobSecondaryStatus} will return {@link AutoMLJobSecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #autoMLJobSecondaryStatusAsString}.
*
*
* @return The secondary status of the AutoML job.
* @see AutoMLJobSecondaryStatus
*/
public final String autoMLJobSecondaryStatusAsString() {
return autoMLJobSecondaryStatus;
}
/**
*
* When the AutoML job was created.
*
*
* @return When the AutoML job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The end time of an AutoML job.
*
*
* @return The end time of an AutoML job.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* When the AutoML job was last modified.
*
*
* @return When the AutoML job was last modified.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* The failure reason of an AutoML job.
*
*
* @return The failure reason of an AutoML job.
*/
public final String failureReason() {
return failureReason;
}
/**
* For responses, this returns true if the service returned a value for the PartialFailureReasons property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasPartialFailureReasons() {
return partialFailureReasons != null && !(partialFailureReasons instanceof SdkAutoConstructList);
}
/**
*
* The list of reasons for partial failures within an AutoML job.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPartialFailureReasons} method.
*
*
* @return The list of reasons for partial failures within an AutoML job.
*/
public final List partialFailureReasons() {
return partialFailureReasons;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobName());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobArn());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobSecondaryStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(hasPartialFailureReasons() ? partialFailureReasons() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AutoMLJobSummary)) {
return false;
}
AutoMLJobSummary other = (AutoMLJobSummary) obj;
return Objects.equals(autoMLJobName(), other.autoMLJobName()) && Objects.equals(autoMLJobArn(), other.autoMLJobArn())
&& Objects.equals(autoMLJobStatusAsString(), other.autoMLJobStatusAsString())
&& Objects.equals(autoMLJobSecondaryStatusAsString(), other.autoMLJobSecondaryStatusAsString())
&& Objects.equals(creationTime(), other.creationTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(failureReason(), other.failureReason())
&& hasPartialFailureReasons() == other.hasPartialFailureReasons()
&& Objects.equals(partialFailureReasons(), other.partialFailureReasons());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AutoMLJobSummary").add("AutoMLJobName", autoMLJobName()).add("AutoMLJobArn", autoMLJobArn())
.add("AutoMLJobStatus", autoMLJobStatusAsString())
.add("AutoMLJobSecondaryStatus", autoMLJobSecondaryStatusAsString()).add("CreationTime", creationTime())
.add("EndTime", endTime()).add("LastModifiedTime", lastModifiedTime()).add("FailureReason", failureReason())
.add("PartialFailureReasons", hasPartialFailureReasons() ? partialFailureReasons() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AutoMLJobName":
return Optional.ofNullable(clazz.cast(autoMLJobName()));
case "AutoMLJobArn":
return Optional.ofNullable(clazz.cast(autoMLJobArn()));
case "AutoMLJobStatus":
return Optional.ofNullable(clazz.cast(autoMLJobStatusAsString()));
case "AutoMLJobSecondaryStatus":
return Optional.ofNullable(clazz.cast(autoMLJobSecondaryStatusAsString()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "PartialFailureReasons":
return Optional.ofNullable(clazz.cast(partialFailureReasons()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function