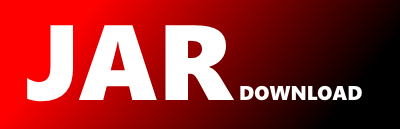
software.amazon.awssdk.services.sagemaker.model.ParameterRanges Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies ranges of integer, continuous, and categorical hyperparameters that a hyperparameter tuning job searches.
* The hyperparameter tuning job launches training jobs with hyperparameter values within these ranges to find the
* combination of values that result in the training job with the best performance as measured by the objective metric
* of the hyperparameter tuning job.
*
*
*
* You can specify a maximum of 20 hyperparameters that a hyperparameter tuning job can search over. Every possible
* value of a categorical parameter range counts against this limit.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ParameterRanges implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> INTEGER_PARAMETER_RANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IntegerParameterRanges")
.getter(getter(ParameterRanges::integerParameterRanges))
.setter(setter(Builder::integerParameterRanges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IntegerParameterRanges").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(IntegerParameterRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CONTINUOUS_PARAMETER_RANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ContinuousParameterRanges")
.getter(getter(ParameterRanges::continuousParameterRanges))
.setter(setter(Builder::continuousParameterRanges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContinuousParameterRanges").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContinuousParameterRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CATEGORICAL_PARAMETER_RANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CategoricalParameterRanges")
.getter(getter(ParameterRanges::categoricalParameterRanges))
.setter(setter(Builder::categoricalParameterRanges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CategoricalParameterRanges").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CategoricalParameterRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
INTEGER_PARAMETER_RANGES_FIELD, CONTINUOUS_PARAMETER_RANGES_FIELD, CATEGORICAL_PARAMETER_RANGES_FIELD));
private static final long serialVersionUID = 1L;
private final List integerParameterRanges;
private final List continuousParameterRanges;
private final List categoricalParameterRanges;
private ParameterRanges(BuilderImpl builder) {
this.integerParameterRanges = builder.integerParameterRanges;
this.continuousParameterRanges = builder.continuousParameterRanges;
this.categoricalParameterRanges = builder.categoricalParameterRanges;
}
/**
* For responses, this returns true if the service returned a value for the IntegerParameterRanges property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasIntegerParameterRanges() {
return integerParameterRanges != null && !(integerParameterRanges instanceof SdkAutoConstructList);
}
/**
*
* The array of IntegerParameterRange objects that specify ranges of integer hyperparameters that a
* hyperparameter tuning job searches.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIntegerParameterRanges} method.
*
*
* @return The array of IntegerParameterRange objects that specify ranges of integer hyperparameters that a
* hyperparameter tuning job searches.
*/
public final List integerParameterRanges() {
return integerParameterRanges;
}
/**
* For responses, this returns true if the service returned a value for the ContinuousParameterRanges property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasContinuousParameterRanges() {
return continuousParameterRanges != null && !(continuousParameterRanges instanceof SdkAutoConstructList);
}
/**
*
* The array of ContinuousParameterRange objects that specify ranges of continuous hyperparameters that a
* hyperparameter tuning job searches.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContinuousParameterRanges} method.
*
*
* @return The array of ContinuousParameterRange objects that specify ranges of continuous hyperparameters
* that a hyperparameter tuning job searches.
*/
public final List continuousParameterRanges() {
return continuousParameterRanges;
}
/**
* For responses, this returns true if the service returned a value for the CategoricalParameterRanges property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCategoricalParameterRanges() {
return categoricalParameterRanges != null && !(categoricalParameterRanges instanceof SdkAutoConstructList);
}
/**
*
* The array of CategoricalParameterRange objects that specify ranges of categorical hyperparameters that a
* hyperparameter tuning job searches.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCategoricalParameterRanges} method.
*
*
* @return The array of CategoricalParameterRange objects that specify ranges of categorical hyperparameters
* that a hyperparameter tuning job searches.
*/
public final List categoricalParameterRanges() {
return categoricalParameterRanges;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasIntegerParameterRanges() ? integerParameterRanges() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasContinuousParameterRanges() ? continuousParameterRanges() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCategoricalParameterRanges() ? categoricalParameterRanges() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ParameterRanges)) {
return false;
}
ParameterRanges other = (ParameterRanges) obj;
return hasIntegerParameterRanges() == other.hasIntegerParameterRanges()
&& Objects.equals(integerParameterRanges(), other.integerParameterRanges())
&& hasContinuousParameterRanges() == other.hasContinuousParameterRanges()
&& Objects.equals(continuousParameterRanges(), other.continuousParameterRanges())
&& hasCategoricalParameterRanges() == other.hasCategoricalParameterRanges()
&& Objects.equals(categoricalParameterRanges(), other.categoricalParameterRanges());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ParameterRanges")
.add("IntegerParameterRanges", hasIntegerParameterRanges() ? integerParameterRanges() : null)
.add("ContinuousParameterRanges", hasContinuousParameterRanges() ? continuousParameterRanges() : null)
.add("CategoricalParameterRanges", hasCategoricalParameterRanges() ? categoricalParameterRanges() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IntegerParameterRanges":
return Optional.ofNullable(clazz.cast(integerParameterRanges()));
case "ContinuousParameterRanges":
return Optional.ofNullable(clazz.cast(continuousParameterRanges()));
case "CategoricalParameterRanges":
return Optional.ofNullable(clazz.cast(categoricalParameterRanges()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function