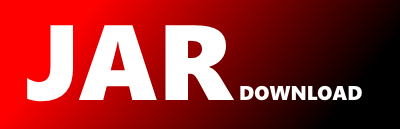
software.amazon.awssdk.services.sagemaker.model.HumanTaskConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information required for human workers to complete a labeling task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class HumanTaskConfig implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField WORKTEAM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkteamArn").getter(getter(HumanTaskConfig::workteamArn)).setter(setter(Builder::workteamArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkteamArn").build()).build();
private static final SdkField UI_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("UiConfig").getter(getter(HumanTaskConfig::uiConfig)).setter(setter(Builder::uiConfig))
.constructor(UiConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UiConfig").build()).build();
private static final SdkField PRE_HUMAN_TASK_LAMBDA_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreHumanTaskLambdaArn").getter(getter(HumanTaskConfig::preHumanTaskLambdaArn))
.setter(setter(Builder::preHumanTaskLambdaArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreHumanTaskLambdaArn").build())
.build();
private static final SdkField> TASK_KEYWORDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TaskKeywords")
.getter(getter(HumanTaskConfig::taskKeywords))
.setter(setter(Builder::taskKeywords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskKeywords").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TASK_TITLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskTitle").getter(getter(HumanTaskConfig::taskTitle)).setter(setter(Builder::taskTitle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskTitle").build()).build();
private static final SdkField TASK_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskDescription").getter(getter(HumanTaskConfig::taskDescription))
.setter(setter(Builder::taskDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskDescription").build()).build();
private static final SdkField NUMBER_OF_HUMAN_WORKERS_PER_DATA_OBJECT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("NumberOfHumanWorkersPerDataObject")
.getter(getter(HumanTaskConfig::numberOfHumanWorkersPerDataObject))
.setter(setter(Builder::numberOfHumanWorkersPerDataObject))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfHumanWorkersPerDataObject")
.build()).build();
private static final SdkField TASK_TIME_LIMIT_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TaskTimeLimitInSeconds").getter(getter(HumanTaskConfig::taskTimeLimitInSeconds))
.setter(setter(Builder::taskTimeLimitInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskTimeLimitInSeconds").build())
.build();
private static final SdkField TASK_AVAILABILITY_LIFETIME_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("TaskAvailabilityLifetimeInSeconds")
.getter(getter(HumanTaskConfig::taskAvailabilityLifetimeInSeconds))
.setter(setter(Builder::taskAvailabilityLifetimeInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskAvailabilityLifetimeInSeconds")
.build()).build();
private static final SdkField MAX_CONCURRENT_TASK_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxConcurrentTaskCount").getter(getter(HumanTaskConfig::maxConcurrentTaskCount))
.setter(setter(Builder::maxConcurrentTaskCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxConcurrentTaskCount").build())
.build();
private static final SdkField ANNOTATION_CONSOLIDATION_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("AnnotationConsolidationConfig")
.getter(getter(HumanTaskConfig::annotationConsolidationConfig))
.setter(setter(Builder::annotationConsolidationConfig))
.constructor(AnnotationConsolidationConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnnotationConsolidationConfig")
.build()).build();
private static final SdkField PUBLIC_WORKFORCE_TASK_PRICE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PublicWorkforceTaskPrice")
.getter(getter(HumanTaskConfig::publicWorkforceTaskPrice)).setter(setter(Builder::publicWorkforceTaskPrice))
.constructor(PublicWorkforceTaskPrice::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicWorkforceTaskPrice").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKTEAM_ARN_FIELD,
UI_CONFIG_FIELD, PRE_HUMAN_TASK_LAMBDA_ARN_FIELD, TASK_KEYWORDS_FIELD, TASK_TITLE_FIELD, TASK_DESCRIPTION_FIELD,
NUMBER_OF_HUMAN_WORKERS_PER_DATA_OBJECT_FIELD, TASK_TIME_LIMIT_IN_SECONDS_FIELD,
TASK_AVAILABILITY_LIFETIME_IN_SECONDS_FIELD, MAX_CONCURRENT_TASK_COUNT_FIELD, ANNOTATION_CONSOLIDATION_CONFIG_FIELD,
PUBLIC_WORKFORCE_TASK_PRICE_FIELD));
private static final long serialVersionUID = 1L;
private final String workteamArn;
private final UiConfig uiConfig;
private final String preHumanTaskLambdaArn;
private final List taskKeywords;
private final String taskTitle;
private final String taskDescription;
private final Integer numberOfHumanWorkersPerDataObject;
private final Integer taskTimeLimitInSeconds;
private final Integer taskAvailabilityLifetimeInSeconds;
private final Integer maxConcurrentTaskCount;
private final AnnotationConsolidationConfig annotationConsolidationConfig;
private final PublicWorkforceTaskPrice publicWorkforceTaskPrice;
private HumanTaskConfig(BuilderImpl builder) {
this.workteamArn = builder.workteamArn;
this.uiConfig = builder.uiConfig;
this.preHumanTaskLambdaArn = builder.preHumanTaskLambdaArn;
this.taskKeywords = builder.taskKeywords;
this.taskTitle = builder.taskTitle;
this.taskDescription = builder.taskDescription;
this.numberOfHumanWorkersPerDataObject = builder.numberOfHumanWorkersPerDataObject;
this.taskTimeLimitInSeconds = builder.taskTimeLimitInSeconds;
this.taskAvailabilityLifetimeInSeconds = builder.taskAvailabilityLifetimeInSeconds;
this.maxConcurrentTaskCount = builder.maxConcurrentTaskCount;
this.annotationConsolidationConfig = builder.annotationConsolidationConfig;
this.publicWorkforceTaskPrice = builder.publicWorkforceTaskPrice;
}
/**
*
* The Amazon Resource Name (ARN) of the work team assigned to complete the tasks.
*
*
* @return The Amazon Resource Name (ARN) of the work team assigned to complete the tasks.
*/
public final String workteamArn() {
return workteamArn;
}
/**
*
* Information about the user interface that workers use to complete the labeling task.
*
*
* @return Information about the user interface that workers use to complete the labeling task.
*/
public final UiConfig uiConfig() {
return uiConfig;
}
/**
*
* The Amazon Resource Name (ARN) of a Lambda function that is run before a data object is sent to a human worker.
* Use this function to provide input to a custom labeling job.
*
*
* For built-in task types, use
* one of the following Amazon SageMaker Ground Truth Lambda function ARNs for PreHumanTaskLambdaArn
.
* For custom labeling workflows, see Pre-annotation Lambda.
*
*
* Bounding box - Finds the most similar boxes from different workers based on the Jaccard index of the
* boxes.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-BoundingBox
*
*
*
*
* Image classification - Uses a variant of the Expectation Maximization approach to estimate the true class
* of an image based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-ImageMultiClass
*
*
*
*
* Multi-label image classification - Uses a variant of the Expectation Maximization approach to estimate the
* true classes of an image based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-ImageMultiClassMultiLabel
*
*
*
*
* Semantic segmentation - Treats each pixel in an image as a multi-class classification and treats pixel
* annotations from workers as "votes" for the correct label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-SemanticSegmentation
*
*
*
*
* Text classification - Uses a variant of the Expectation Maximization approach to estimate the true class
* of text based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-TextMultiClass
*
*
*
*
* Multi-label text classification - Uses a variant of the Expectation Maximization approach to estimate the
* true classes of text based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-TextMultiClassMultiLabel
*
*
*
*
* Named entity recognition - Groups similar selections and calculates aggregate boundaries, resolving to
* most-assigned label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-NamedEntityRecognition
*
*
*
*
* Video Classification - Use this task type when you need workers to classify videos using predefined labels
* that you specify. Workers are shown videos and are asked to choose one label for each video.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoMultiClass
*
*
*
*
* Video Frame Object Detection - Use this task type to have workers identify and locate objects in a
* sequence of video frames (images extracted from a video) using bounding boxes. For example, you can use this task
* to ask workers to identify and localize various objects in a series of video frames, such as cars, bikes, and
* pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoObjectDetection
*
*
*
*
* Video Frame Object Tracking - Use this task type to have workers track the movement of objects in a
* sequence of video frames (images extracted from a video) using bounding boxes. For example, you can use this task
* to ask workers to track the movement of objects, such as cars, bikes, and pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoObjectTracking
*
*
*
*
* 3D Point Cloud Modalities
*
*
* Use the following pre-annotation lambdas for 3D point cloud labeling modality tasks. See 3D Point Cloud Task types
* to learn more.
*
*
* 3D Point Cloud Object Detection - Use this task type when you want workers to classify objects in a 3D
* point cloud by drawing 3D cuboids around objects. For example, you can use this task type to ask workers to
* identify different types of objects in a point cloud, such as cars, bikes, and pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudObjectDetection
*
*
*
*
* 3D Point Cloud Object Tracking - Use this task type when you want workers to draw 3D cuboids around
* objects that appear in a sequence of 3D point cloud frames. For example, you can use this task type to ask
* workers to track the movement of vehicles across multiple point cloud frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudObjectTracking
*
*
*
*
* 3D Point Cloud Semantic Segmentation - Use this task type when you want workers to create a point-level
* semantic segmentation masks by painting objects in a 3D point cloud using different colors where each color is
* assigned to one of the classes you specify.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudSemanticSegmentation
*
*
*
*
* Use the following ARNs for Label Verification and Adjustment Jobs
*
*
* Use label verification and adjustment jobs to review and adjust labels. To learn more, see Verify and Adjust Labels .
*
*
* Bounding box verification - Uses a variant of the Expectation Maximization approach to estimate the true
* class of verification judgement for bounding box labels based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VerificationBoundingBox
*
*
*
*
* Bounding box adjustment - Finds the most similar boxes from different workers based on the Jaccard index
* of the adjusted annotations.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentBoundingBox
*
*
*
*
* Semantic segmentation verification - Uses a variant of the Expectation Maximization approach to estimate
* the true class of verification judgment for semantic segmentation labels based on annotations from individual
* workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VerificationSemanticSegmentation
*
*
*
*
* Semantic segmentation adjustment - Treats each pixel in an image as a multi-class classification and
* treats pixel adjusted annotations from workers as "votes" for the correct label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentSemanticSegmentation
*
*
*
*
* Video Frame Object Detection Adjustment - Use this task type when you want workers to adjust bounding
* boxes that workers have added to video frames to classify and localize objects in a sequence of video frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentVideoObjectDetection
*
*
*
*
* Video Frame Object Tracking Adjustment - Use this task type when you want workers to adjust bounding boxes
* that workers have added to video frames to track object movement across a sequence of video frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentVideoObjectTracking
*
*
*
*
* 3D point cloud object detection adjustment - Adjust 3D cuboids in a point cloud frame.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
*
*
* 3D point cloud object tracking adjustment - Adjust 3D cuboids across a sequence of point cloud frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
*
*
* 3D point cloud semantic segmentation adjustment - Adjust semantic segmentation masks in a 3D point cloud.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
*
*
* @return The Amazon Resource Name (ARN) of a Lambda function that is run before a data object is sent to a human
* worker. Use this function to provide input to a custom labeling job.
*
* For built-in task
* types, use one of the following Amazon SageMaker Ground Truth Lambda function ARNs for
* PreHumanTaskLambdaArn
. For custom labeling workflows, see Pre-annotation Lambda.
*
*
* Bounding box - Finds the most similar boxes from different workers based on the Jaccard index of
* the boxes.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-BoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-BoundingBox
*
*
*
*
* Image classification - Uses a variant of the Expectation Maximization approach to estimate the
* true class of an image based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-ImageMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-ImageMultiClass
*
*
*
*
* Multi-label image classification - Uses a variant of the Expectation Maximization approach to
* estimate the true classes of an image based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-ImageMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-ImageMultiClassMultiLabel
*
*
*
*
* Semantic segmentation - Treats each pixel in an image as a multi-class classification and treats
* pixel annotations from workers as "votes" for the correct label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-SemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-SemanticSegmentation
*
*
*
*
* Text classification - Uses a variant of the Expectation Maximization approach to estimate the true
* class of text based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-TextMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-TextMultiClass
*
*
*
*
* Multi-label text classification - Uses a variant of the Expectation Maximization approach to
* estimate the true classes of text based on annotations from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-TextMultiClassMultiLabel
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-TextMultiClassMultiLabel
*
*
*
*
* Named entity recognition - Groups similar selections and calculates aggregate boundaries,
* resolving to most-assigned label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-NamedEntityRecognition
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-NamedEntityRecognition
*
*
*
*
* Video Classification - Use this task type when you need workers to classify videos using
* predefined labels that you specify. Workers are shown videos and are asked to choose one label for each
* video.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoMultiClass
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoMultiClass
*
*
*
*
* Video Frame Object Detection - Use this task type to have workers identify and locate objects in a
* sequence of video frames (images extracted from a video) using bounding boxes. For example, you can use
* this task to ask workers to identify and localize various objects in a series of video frames, such as
* cars, bikes, and pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoObjectDetection
*
*
*
*
* Video Frame Object Tracking - Use this task type to have workers track the movement of objects in
* a sequence of video frames (images extracted from a video) using bounding boxes. For example, you can use
* this task to ask workers to track the movement of objects, such as cars, bikes, and pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VideoObjectTracking
*
*
*
*
* 3D Point Cloud Modalities
*
*
* Use the following pre-annotation lambdas for 3D point cloud labeling modality tasks. See 3D Point Cloud
* Task types to learn more.
*
*
* 3D Point Cloud Object Detection - Use this task type when you want workers to classify objects in
* a 3D point cloud by drawing 3D cuboids around objects. For example, you can use this task type to ask
* workers to identify different types of objects in a point cloud, such as cars, bikes, and pedestrians.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudObjectDetection
*
*
*
*
* 3D Point Cloud Object Tracking - Use this task type when you want workers to draw 3D cuboids
* around objects that appear in a sequence of 3D point cloud frames. For example, you can use this task
* type to ask workers to track the movement of vehicles across multiple point cloud frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudObjectTracking
*
*
*
*
* 3D Point Cloud Semantic Segmentation - Use this task type when you want workers to create a
* point-level semantic segmentation masks by painting objects in a 3D point cloud using different colors
* where each color is assigned to one of the classes you specify.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-3DPointCloudSemanticSegmentation
*
*
*
*
* Use the following ARNs for Label Verification and Adjustment Jobs
*
*
* Use label verification and adjustment jobs to review and adjust labels. To learn more, see Verify and Adjust
* Labels .
*
*
* Bounding box verification - Uses a variant of the Expectation Maximization approach to estimate
* the true class of verification judgement for bounding box labels based on annotations from individual
* workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VerificationBoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VerificationBoundingBox
*
*
*
*
* Bounding box adjustment - Finds the most similar boxes from different workers based on the Jaccard
* index of the adjusted annotations.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentBoundingBox
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentBoundingBox
*
*
*
*
* Semantic segmentation verification - Uses a variant of the Expectation Maximization approach to
* estimate the true class of verification judgment for semantic segmentation labels based on annotations
* from individual workers.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-VerificationSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-VerificationSemanticSegmentation
*
*
*
*
* Semantic segmentation adjustment - Treats each pixel in an image as a multi-class classification
* and treats pixel adjusted annotations from workers as "votes" for the correct label.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentSemanticSegmentation
*
*
*
*
* Video Frame Object Detection Adjustment - Use this task type when you want workers to adjust
* bounding boxes that workers have added to video frames to classify and localize objects in a sequence of
* video frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentVideoObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentVideoObjectDetection
*
*
*
*
* Video Frame Object Tracking Adjustment - Use this task type when you want workers to adjust
* bounding boxes that workers have added to video frames to track object movement across a sequence of
* video frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-AdjustmentVideoObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-AdjustmentVideoObjectTracking
*
*
*
*
* 3D point cloud object detection adjustment - Adjust 3D cuboids in a point cloud frame.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudObjectDetection
*
*
*
*
* 3D point cloud object tracking adjustment - Adjust 3D cuboids across a sequence of point cloud
* frames.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudObjectTracking
*
*
*
*
* 3D point cloud semantic segmentation adjustment - Adjust semantic segmentation masks in a 3D point
* cloud.
*
*
* -
*
* arn:aws:lambda:us-east-1:432418664414:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-east-2:266458841044:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:us-west-2:081040173940:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-1:568282634449:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-1:477331159723:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-2:454466003867:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-south-1:565803892007:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-central-1:203001061592:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-northeast-2:845288260483:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:eu-west-2:487402164563:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ap-southeast-1:377565633583:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
* -
*
* arn:aws:lambda:ca-central-1:918755190332:function:PRE-Adjustment3DPointCloudSemanticSegmentation
*
*
*/
public final String preHumanTaskLambdaArn() {
return preHumanTaskLambdaArn;
}
/**
* For responses, this returns true if the service returned a value for the TaskKeywords property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTaskKeywords() {
return taskKeywords != null && !(taskKeywords instanceof SdkAutoConstructList);
}
/**
*
* Keywords used to describe the task so that workers on Amazon Mechanical Turk can discover the task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTaskKeywords} method.
*
*
* @return Keywords used to describe the task so that workers on Amazon Mechanical Turk can discover the task.
*/
public final List taskKeywords() {
return taskKeywords;
}
/**
*
* A title for the task for your human workers.
*
*
* @return A title for the task for your human workers.
*/
public final String taskTitle() {
return taskTitle;
}
/**
*
* A description of the task for your human workers.
*
*
* @return A description of the task for your human workers.
*/
public final String taskDescription() {
return taskDescription;
}
/**
*
* The number of human workers that will label an object.
*
*
* @return The number of human workers that will label an object.
*/
public final Integer numberOfHumanWorkersPerDataObject() {
return numberOfHumanWorkersPerDataObject;
}
/**
*
* The amount of time that a worker has to complete a task.
*
*
* If you create a custom labeling job, the maximum value for this parameter is 8 hours (28,800 seconds).
*
*
* If you create a labeling job using a built-in task type the maximum for
* this parameter depends on the task type you use:
*
*
* -
*
* For image and text labeling jobs, the maximum is
* 8 hours (28,800 seconds).
*
*
* -
*
* For 3D point cloud and video frame labeling jobs, the maximum
* is 30 days (2952,000 seconds) for non-AL mode. For most users, the maximum is also 30 days. If you want to change
* these limits, contact Amazon Web Services Support.
*
*
*
*
* @return The amount of time that a worker has to complete a task.
*
* If you create a custom labeling job, the maximum value for this parameter is 8 hours (28,800 seconds).
*
*
* If you create a labeling job using a built-in task type the
* maximum for this parameter depends on the task type you use:
*
*
* -
*
* For image and text labeling jobs, the
* maximum is 8 hours (28,800 seconds).
*
*
* -
*
* For 3D point cloud and
* video frame labeling jobs,
* the maximum is 30 days (2952,000 seconds) for non-AL mode. For most users, the maximum is also 30 days.
* If you want to change these limits, contact Amazon Web Services Support.
*
*
*/
public final Integer taskTimeLimitInSeconds() {
return taskTimeLimitInSeconds;
}
/**
*
* The length of time that a task remains available for labeling by human workers. The default and maximum values
* for this parameter depend on the type of workforce you use.
*
*
* -
*
* If you choose the Amazon Mechanical Turk workforce, the maximum is 12 hours (43,200 seconds). The default is 6
* hours (21,600 seconds).
*
*
* -
*
* If you choose a private or vendor workforce, the default value is 30 days (2592,000 seconds) for non-AL mode. For
* most users, the maximum is also 30 days. If you want to change this limit, contact Amazon Web Services Support.
*
*
*
*
* @return The length of time that a task remains available for labeling by human workers. The default and maximum
* values for this parameter depend on the type of workforce you use.
*
* -
*
* If you choose the Amazon Mechanical Turk workforce, the maximum is 12 hours (43,200 seconds). The default
* is 6 hours (21,600 seconds).
*
*
* -
*
* If you choose a private or vendor workforce, the default value is 30 days (2592,000 seconds) for non-AL
* mode. For most users, the maximum is also 30 days. If you want to change this limit, contact Amazon Web
* Services Support.
*
*
*/
public final Integer taskAvailabilityLifetimeInSeconds() {
return taskAvailabilityLifetimeInSeconds;
}
/**
*
* Defines the maximum number of data objects that can be labeled by human workers at the same time. Also referred
* to as batch size. Each object may have more than one worker at one time. The default value is 1000 objects.
*
*
* @return Defines the maximum number of data objects that can be labeled by human workers at the same time. Also
* referred to as batch size. Each object may have more than one worker at one time. The default value is
* 1000 objects.
*/
public final Integer maxConcurrentTaskCount() {
return maxConcurrentTaskCount;
}
/**
*
* Configures how labels are consolidated across human workers.
*
*
* @return Configures how labels are consolidated across human workers.
*/
public final AnnotationConsolidationConfig annotationConsolidationConfig() {
return annotationConsolidationConfig;
}
/**
*
* The price that you pay for each task performed by an Amazon Mechanical Turk worker.
*
*
* @return The price that you pay for each task performed by an Amazon Mechanical Turk worker.
*/
public final PublicWorkforceTaskPrice publicWorkforceTaskPrice() {
return publicWorkforceTaskPrice;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(workteamArn());
hashCode = 31 * hashCode + Objects.hashCode(uiConfig());
hashCode = 31 * hashCode + Objects.hashCode(preHumanTaskLambdaArn());
hashCode = 31 * hashCode + Objects.hashCode(hasTaskKeywords() ? taskKeywords() : null);
hashCode = 31 * hashCode + Objects.hashCode(taskTitle());
hashCode = 31 * hashCode + Objects.hashCode(taskDescription());
hashCode = 31 * hashCode + Objects.hashCode(numberOfHumanWorkersPerDataObject());
hashCode = 31 * hashCode + Objects.hashCode(taskTimeLimitInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(taskAvailabilityLifetimeInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(maxConcurrentTaskCount());
hashCode = 31 * hashCode + Objects.hashCode(annotationConsolidationConfig());
hashCode = 31 * hashCode + Objects.hashCode(publicWorkforceTaskPrice());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof HumanTaskConfig)) {
return false;
}
HumanTaskConfig other = (HumanTaskConfig) obj;
return Objects.equals(workteamArn(), other.workteamArn()) && Objects.equals(uiConfig(), other.uiConfig())
&& Objects.equals(preHumanTaskLambdaArn(), other.preHumanTaskLambdaArn())
&& hasTaskKeywords() == other.hasTaskKeywords() && Objects.equals(taskKeywords(), other.taskKeywords())
&& Objects.equals(taskTitle(), other.taskTitle()) && Objects.equals(taskDescription(), other.taskDescription())
&& Objects.equals(numberOfHumanWorkersPerDataObject(), other.numberOfHumanWorkersPerDataObject())
&& Objects.equals(taskTimeLimitInSeconds(), other.taskTimeLimitInSeconds())
&& Objects.equals(taskAvailabilityLifetimeInSeconds(), other.taskAvailabilityLifetimeInSeconds())
&& Objects.equals(maxConcurrentTaskCount(), other.maxConcurrentTaskCount())
&& Objects.equals(annotationConsolidationConfig(), other.annotationConsolidationConfig())
&& Objects.equals(publicWorkforceTaskPrice(), other.publicWorkforceTaskPrice());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("HumanTaskConfig").add("WorkteamArn", workteamArn()).add("UiConfig", uiConfig())
.add("PreHumanTaskLambdaArn", preHumanTaskLambdaArn())
.add("TaskKeywords", hasTaskKeywords() ? taskKeywords() : null).add("TaskTitle", taskTitle())
.add("TaskDescription", taskDescription())
.add("NumberOfHumanWorkersPerDataObject", numberOfHumanWorkersPerDataObject())
.add("TaskTimeLimitInSeconds", taskTimeLimitInSeconds())
.add("TaskAvailabilityLifetimeInSeconds", taskAvailabilityLifetimeInSeconds())
.add("MaxConcurrentTaskCount", maxConcurrentTaskCount())
.add("AnnotationConsolidationConfig", annotationConsolidationConfig())
.add("PublicWorkforceTaskPrice", publicWorkforceTaskPrice()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "WorkteamArn":
return Optional.ofNullable(clazz.cast(workteamArn()));
case "UiConfig":
return Optional.ofNullable(clazz.cast(uiConfig()));
case "PreHumanTaskLambdaArn":
return Optional.ofNullable(clazz.cast(preHumanTaskLambdaArn()));
case "TaskKeywords":
return Optional.ofNullable(clazz.cast(taskKeywords()));
case "TaskTitle":
return Optional.ofNullable(clazz.cast(taskTitle()));
case "TaskDescription":
return Optional.ofNullable(clazz.cast(taskDescription()));
case "NumberOfHumanWorkersPerDataObject":
return Optional.ofNullable(clazz.cast(numberOfHumanWorkersPerDataObject()));
case "TaskTimeLimitInSeconds":
return Optional.ofNullable(clazz.cast(taskTimeLimitInSeconds()));
case "TaskAvailabilityLifetimeInSeconds":
return Optional.ofNullable(clazz.cast(taskAvailabilityLifetimeInSeconds()));
case "MaxConcurrentTaskCount":
return Optional.ofNullable(clazz.cast(maxConcurrentTaskCount()));
case "AnnotationConsolidationConfig":
return Optional.ofNullable(clazz.cast(annotationConsolidationConfig()));
case "PublicWorkforceTaskPrice":
return Optional.ofNullable(clazz.cast(publicWorkforceTaskPrice()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function