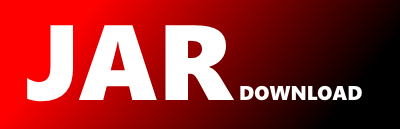
software.amazon.awssdk.services.sagemaker.model.AthenaDatasetDefinition Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Configuration for Athena Dataset Definition input.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AthenaDatasetDefinition implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CATALOG_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Catalog")
.getter(getter(AthenaDatasetDefinition::catalog)).setter(setter(Builder::catalog))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Catalog").build()).build();
private static final SdkField DATABASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Database").getter(getter(AthenaDatasetDefinition::database)).setter(setter(Builder::database))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Database").build()).build();
private static final SdkField QUERY_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QueryString").getter(getter(AthenaDatasetDefinition::queryString)).setter(setter(Builder::queryString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueryString").build()).build();
private static final SdkField WORK_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkGroup").getter(getter(AthenaDatasetDefinition::workGroup)).setter(setter(Builder::workGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkGroup").build()).build();
private static final SdkField OUTPUT_S3_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputS3Uri").getter(getter(AthenaDatasetDefinition::outputS3Uri)).setter(setter(Builder::outputS3Uri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputS3Uri").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(AthenaDatasetDefinition::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField OUTPUT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputFormat").getter(getter(AthenaDatasetDefinition::outputFormatAsString))
.setter(setter(Builder::outputFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputFormat").build()).build();
private static final SdkField OUTPUT_COMPRESSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputCompression").getter(getter(AthenaDatasetDefinition::outputCompressionAsString))
.setter(setter(Builder::outputCompression))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputCompression").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CATALOG_FIELD, DATABASE_FIELD,
QUERY_STRING_FIELD, WORK_GROUP_FIELD, OUTPUT_S3_URI_FIELD, KMS_KEY_ID_FIELD, OUTPUT_FORMAT_FIELD,
OUTPUT_COMPRESSION_FIELD));
private static final long serialVersionUID = 1L;
private final String catalog;
private final String database;
private final String queryString;
private final String workGroup;
private final String outputS3Uri;
private final String kmsKeyId;
private final String outputFormat;
private final String outputCompression;
private AthenaDatasetDefinition(BuilderImpl builder) {
this.catalog = builder.catalog;
this.database = builder.database;
this.queryString = builder.queryString;
this.workGroup = builder.workGroup;
this.outputS3Uri = builder.outputS3Uri;
this.kmsKeyId = builder.kmsKeyId;
this.outputFormat = builder.outputFormat;
this.outputCompression = builder.outputCompression;
}
/**
* Returns the value of the Catalog property for this object.
*
* @return The value of the Catalog property for this object.
*/
public final String catalog() {
return catalog;
}
/**
* Returns the value of the Database property for this object.
*
* @return The value of the Database property for this object.
*/
public final String database() {
return database;
}
/**
* Returns the value of the QueryString property for this object.
*
* @return The value of the QueryString property for this object.
*/
public final String queryString() {
return queryString;
}
/**
* Returns the value of the WorkGroup property for this object.
*
* @return The value of the WorkGroup property for this object.
*/
public final String workGroup() {
return workGroup;
}
/**
*
* The location in Amazon S3 where Athena query results are stored.
*
*
* @return The location in Amazon S3 where Athena query results are stored.
*/
public final String outputS3Uri() {
return outputS3Uri;
}
/**
*
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to
* encrypt data generated from an Athena query execution.
*
*
* @return The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses
* to encrypt data generated from an Athena query execution.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
* Returns the value of the OutputFormat property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link AthenaResultFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #outputFormatAsString}.
*
*
* @return The value of the OutputFormat property for this object.
* @see AthenaResultFormat
*/
public final AthenaResultFormat outputFormat() {
return AthenaResultFormat.fromValue(outputFormat);
}
/**
* Returns the value of the OutputFormat property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link AthenaResultFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #outputFormatAsString}.
*
*
* @return The value of the OutputFormat property for this object.
* @see AthenaResultFormat
*/
public final String outputFormatAsString() {
return outputFormat;
}
/**
* Returns the value of the OutputCompression property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputCompression}
* will return {@link AthenaResultCompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputCompressionAsString}.
*
*
* @return The value of the OutputCompression property for this object.
* @see AthenaResultCompressionType
*/
public final AthenaResultCompressionType outputCompression() {
return AthenaResultCompressionType.fromValue(outputCompression);
}
/**
* Returns the value of the OutputCompression property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputCompression}
* will return {@link AthenaResultCompressionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputCompressionAsString}.
*
*
* @return The value of the OutputCompression property for this object.
* @see AthenaResultCompressionType
*/
public final String outputCompressionAsString() {
return outputCompression;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(catalog());
hashCode = 31 * hashCode + Objects.hashCode(database());
hashCode = 31 * hashCode + Objects.hashCode(queryString());
hashCode = 31 * hashCode + Objects.hashCode(workGroup());
hashCode = 31 * hashCode + Objects.hashCode(outputS3Uri());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(outputFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(outputCompressionAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AthenaDatasetDefinition)) {
return false;
}
AthenaDatasetDefinition other = (AthenaDatasetDefinition) obj;
return Objects.equals(catalog(), other.catalog()) && Objects.equals(database(), other.database())
&& Objects.equals(queryString(), other.queryString()) && Objects.equals(workGroup(), other.workGroup())
&& Objects.equals(outputS3Uri(), other.outputS3Uri()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(outputFormatAsString(), other.outputFormatAsString())
&& Objects.equals(outputCompressionAsString(), other.outputCompressionAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AthenaDatasetDefinition").add("Catalog", catalog()).add("Database", database())
.add("QueryString", queryString()).add("WorkGroup", workGroup()).add("OutputS3Uri", outputS3Uri())
.add("KmsKeyId", kmsKeyId()).add("OutputFormat", outputFormatAsString())
.add("OutputCompression", outputCompressionAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Catalog":
return Optional.ofNullable(clazz.cast(catalog()));
case "Database":
return Optional.ofNullable(clazz.cast(database()));
case "QueryString":
return Optional.ofNullable(clazz.cast(queryString()));
case "WorkGroup":
return Optional.ofNullable(clazz.cast(workGroup()));
case "OutputS3Uri":
return Optional.ofNullable(clazz.cast(outputS3Uri()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "OutputFormat":
return Optional.ofNullable(clazz.cast(outputFormatAsString()));
case "OutputCompression":
return Optional.ofNullable(clazz.cast(outputCompressionAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function