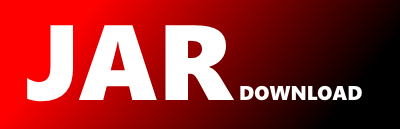
software.amazon.awssdk.services.sagemaker.model.DescribeLabelingJobResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeLabelingJobResponse extends SageMakerResponse implements
ToCopyableBuilder {
private static final SdkField LABELING_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LabelingJobStatus").getter(getter(DescribeLabelingJobResponse::labelingJobStatusAsString))
.setter(setter(Builder::labelingJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelingJobStatus").build()).build();
private static final SdkField LABEL_COUNTERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LabelCounters")
.getter(getter(DescribeLabelingJobResponse::labelCounters)).setter(setter(Builder::labelCounters))
.constructor(LabelCounters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelCounters").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(DescribeLabelingJobResponse::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(DescribeLabelingJobResponse::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(DescribeLabelingJobResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField JOB_REFERENCE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobReferenceCode").getter(getter(DescribeLabelingJobResponse::jobReferenceCode))
.setter(setter(Builder::jobReferenceCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobReferenceCode").build()).build();
private static final SdkField LABELING_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LabelingJobName").getter(getter(DescribeLabelingJobResponse::labelingJobName))
.setter(setter(Builder::labelingJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelingJobName").build()).build();
private static final SdkField LABELING_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LabelingJobArn").getter(getter(DescribeLabelingJobResponse::labelingJobArn))
.setter(setter(Builder::labelingJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelingJobArn").build()).build();
private static final SdkField LABEL_ATTRIBUTE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LabelAttributeName").getter(getter(DescribeLabelingJobResponse::labelAttributeName))
.setter(setter(Builder::labelAttributeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelAttributeName").build())
.build();
private static final SdkField INPUT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputConfig")
.getter(getter(DescribeLabelingJobResponse::inputConfig)).setter(setter(Builder::inputConfig))
.constructor(LabelingJobInputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputConfig").build()).build();
private static final SdkField OUTPUT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OutputConfig")
.getter(getter(DescribeLabelingJobResponse::outputConfig)).setter(setter(Builder::outputConfig))
.constructor(LabelingJobOutputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputConfig").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(DescribeLabelingJobResponse::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField LABEL_CATEGORY_CONFIG_S3_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LabelCategoryConfigS3Uri").getter(getter(DescribeLabelingJobResponse::labelCategoryConfigS3Uri))
.setter(setter(Builder::labelCategoryConfigS3Uri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelCategoryConfigS3Uri").build())
.build();
private static final SdkField STOPPING_CONDITIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StoppingConditions")
.getter(getter(DescribeLabelingJobResponse::stoppingConditions)).setter(setter(Builder::stoppingConditions))
.constructor(LabelingJobStoppingConditions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StoppingConditions").build())
.build();
private static final SdkField LABELING_JOB_ALGORITHMS_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LabelingJobAlgorithmsConfig")
.getter(getter(DescribeLabelingJobResponse::labelingJobAlgorithmsConfig))
.setter(setter(Builder::labelingJobAlgorithmsConfig))
.constructor(LabelingJobAlgorithmsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelingJobAlgorithmsConfig")
.build()).build();
private static final SdkField HUMAN_TASK_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HumanTaskConfig")
.getter(getter(DescribeLabelingJobResponse::humanTaskConfig)).setter(setter(Builder::humanTaskConfig))
.constructor(HumanTaskConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HumanTaskConfig").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(DescribeLabelingJobResponse::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LABELING_JOB_OUTPUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LabelingJobOutput")
.getter(getter(DescribeLabelingJobResponse::labelingJobOutput)).setter(setter(Builder::labelingJobOutput))
.constructor(LabelingJobOutput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelingJobOutput").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LABELING_JOB_STATUS_FIELD,
LABEL_COUNTERS_FIELD, FAILURE_REASON_FIELD, CREATION_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, JOB_REFERENCE_CODE_FIELD,
LABELING_JOB_NAME_FIELD, LABELING_JOB_ARN_FIELD, LABEL_ATTRIBUTE_NAME_FIELD, INPUT_CONFIG_FIELD, OUTPUT_CONFIG_FIELD,
ROLE_ARN_FIELD, LABEL_CATEGORY_CONFIG_S3_URI_FIELD, STOPPING_CONDITIONS_FIELD, LABELING_JOB_ALGORITHMS_CONFIG_FIELD,
HUMAN_TASK_CONFIG_FIELD, TAGS_FIELD, LABELING_JOB_OUTPUT_FIELD));
private final String labelingJobStatus;
private final LabelCounters labelCounters;
private final String failureReason;
private final Instant creationTime;
private final Instant lastModifiedTime;
private final String jobReferenceCode;
private final String labelingJobName;
private final String labelingJobArn;
private final String labelAttributeName;
private final LabelingJobInputConfig inputConfig;
private final LabelingJobOutputConfig outputConfig;
private final String roleArn;
private final String labelCategoryConfigS3Uri;
private final LabelingJobStoppingConditions stoppingConditions;
private final LabelingJobAlgorithmsConfig labelingJobAlgorithmsConfig;
private final HumanTaskConfig humanTaskConfig;
private final List tags;
private final LabelingJobOutput labelingJobOutput;
private DescribeLabelingJobResponse(BuilderImpl builder) {
super(builder);
this.labelingJobStatus = builder.labelingJobStatus;
this.labelCounters = builder.labelCounters;
this.failureReason = builder.failureReason;
this.creationTime = builder.creationTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.jobReferenceCode = builder.jobReferenceCode;
this.labelingJobName = builder.labelingJobName;
this.labelingJobArn = builder.labelingJobArn;
this.labelAttributeName = builder.labelAttributeName;
this.inputConfig = builder.inputConfig;
this.outputConfig = builder.outputConfig;
this.roleArn = builder.roleArn;
this.labelCategoryConfigS3Uri = builder.labelCategoryConfigS3Uri;
this.stoppingConditions = builder.stoppingConditions;
this.labelingJobAlgorithmsConfig = builder.labelingJobAlgorithmsConfig;
this.humanTaskConfig = builder.humanTaskConfig;
this.tags = builder.tags;
this.labelingJobOutput = builder.labelingJobOutput;
}
/**
*
* The processing status of the labeling job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #labelingJobStatus}
* will return {@link LabelingJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #labelingJobStatusAsString}.
*
*
* @return The processing status of the labeling job.
* @see LabelingJobStatus
*/
public final LabelingJobStatus labelingJobStatus() {
return LabelingJobStatus.fromValue(labelingJobStatus);
}
/**
*
* The processing status of the labeling job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #labelingJobStatus}
* will return {@link LabelingJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #labelingJobStatusAsString}.
*
*
* @return The processing status of the labeling job.
* @see LabelingJobStatus
*/
public final String labelingJobStatusAsString() {
return labelingJobStatus;
}
/**
*
* Provides a breakdown of the number of data objects labeled by humans, the number of objects labeled by machine,
* the number of objects than couldn't be labeled, and the total number of objects labeled.
*
*
* @return Provides a breakdown of the number of data objects labeled by humans, the number of objects labeled by
* machine, the number of objects than couldn't be labeled, and the total number of objects labeled.
*/
public final LabelCounters labelCounters() {
return labelCounters;
}
/**
*
* If the job failed, the reason that it failed.
*
*
* @return If the job failed, the reason that it failed.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* The date and time that the labeling job was created.
*
*
* @return The date and time that the labeling job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The date and time that the labeling job was last updated.
*
*
* @return The date and time that the labeling job was last updated.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* A unique identifier for work done as part of a labeling job.
*
*
* @return A unique identifier for work done as part of a labeling job.
*/
public final String jobReferenceCode() {
return jobReferenceCode;
}
/**
*
* The name assigned to the labeling job when it was created.
*
*
* @return The name assigned to the labeling job when it was created.
*/
public final String labelingJobName() {
return labelingJobName;
}
/**
*
* The Amazon Resource Name (ARN) of the labeling job.
*
*
* @return The Amazon Resource Name (ARN) of the labeling job.
*/
public final String labelingJobArn() {
return labelingJobArn;
}
/**
*
* The attribute used as the label in the output manifest file.
*
*
* @return The attribute used as the label in the output manifest file.
*/
public final String labelAttributeName() {
return labelAttributeName;
}
/**
*
* Input configuration information for the labeling job, such as the Amazon S3 location of the data objects and the
* location of the manifest file that describes the data objects.
*
*
* @return Input configuration information for the labeling job, such as the Amazon S3 location of the data objects
* and the location of the manifest file that describes the data objects.
*/
public final LabelingJobInputConfig inputConfig() {
return inputConfig;
}
/**
*
* The location of the job's output data and the Amazon Web Services Key Management Service key ID for the key used
* to encrypt the output data, if any.
*
*
* @return The location of the job's output data and the Amazon Web Services Key Management Service key ID for the
* key used to encrypt the output data, if any.
*/
public final LabelingJobOutputConfig outputConfig() {
return outputConfig;
}
/**
*
* The Amazon Resource Name (ARN) that SageMaker assumes to perform tasks on your behalf during data labeling.
*
*
* @return The Amazon Resource Name (ARN) that SageMaker assumes to perform tasks on your behalf during data
* labeling.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The S3 location of the JSON file that defines the categories used to label data objects. Please note the
* following label-category limits:
*
*
* -
*
* Semantic segmentation labeling jobs using automated labeling: 20 labels
*
*
* -
*
* Box bounding labeling jobs (all): 10 labels
*
*
*
*
* The file is a JSON structure in the following format:
*
*
* {
*
*
* "document-version": "2018-11-28"
*
*
* "labels": [
*
*
* {
*
*
* "label": "label 1"
*
*
* },
*
*
* {
*
*
* "label": "label 2"
*
*
* },
*
*
* ...
*
*
* {
*
*
* "label": "label n"
*
*
* }
*
*
* ]
*
*
* }
*
*
* @return The S3 location of the JSON file that defines the categories used to label data objects. Please note the
* following label-category limits:
*
* -
*
* Semantic segmentation labeling jobs using automated labeling: 20 labels
*
*
* -
*
* Box bounding labeling jobs (all): 10 labels
*
*
*
*
* The file is a JSON structure in the following format:
*
*
* {
*
*
* "document-version": "2018-11-28"
*
*
* "labels": [
*
*
* {
*
*
* "label": "label 1"
*
*
* },
*
*
* {
*
*
* "label": "label 2"
*
*
* },
*
*
* ...
*
*
* {
*
*
* "label": "label n"
*
*
* }
*
*
* ]
*
*
* }
*/
public final String labelCategoryConfigS3Uri() {
return labelCategoryConfigS3Uri;
}
/**
*
* A set of conditions for stopping a labeling job. If any of the conditions are met, the job is automatically
* stopped.
*
*
* @return A set of conditions for stopping a labeling job. If any of the conditions are met, the job is
* automatically stopped.
*/
public final LabelingJobStoppingConditions stoppingConditions() {
return stoppingConditions;
}
/**
*
* Configuration information for automated data labeling.
*
*
* @return Configuration information for automated data labeling.
*/
public final LabelingJobAlgorithmsConfig labelingJobAlgorithmsConfig() {
return labelingJobAlgorithmsConfig;
}
/**
*
* Configuration information required for human workers to complete a labeling task.
*
*
* @return Configuration information required for human workers to complete a labeling task.
*/
public final HumanTaskConfig humanTaskConfig() {
return humanTaskConfig;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public final List tags() {
return tags;
}
/**
*
* The location of the output produced by the labeling job.
*
*
* @return The location of the output produced by the labeling job.
*/
public final LabelingJobOutput labelingJobOutput() {
return labelingJobOutput;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(labelingJobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(labelCounters());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(jobReferenceCode());
hashCode = 31 * hashCode + Objects.hashCode(labelingJobName());
hashCode = 31 * hashCode + Objects.hashCode(labelingJobArn());
hashCode = 31 * hashCode + Objects.hashCode(labelAttributeName());
hashCode = 31 * hashCode + Objects.hashCode(inputConfig());
hashCode = 31 * hashCode + Objects.hashCode(outputConfig());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(labelCategoryConfigS3Uri());
hashCode = 31 * hashCode + Objects.hashCode(stoppingConditions());
hashCode = 31 * hashCode + Objects.hashCode(labelingJobAlgorithmsConfig());
hashCode = 31 * hashCode + Objects.hashCode(humanTaskConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(labelingJobOutput());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeLabelingJobResponse)) {
return false;
}
DescribeLabelingJobResponse other = (DescribeLabelingJobResponse) obj;
return Objects.equals(labelingJobStatusAsString(), other.labelingJobStatusAsString())
&& Objects.equals(labelCounters(), other.labelCounters())
&& Objects.equals(failureReason(), other.failureReason()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(jobReferenceCode(), other.jobReferenceCode())
&& Objects.equals(labelingJobName(), other.labelingJobName())
&& Objects.equals(labelingJobArn(), other.labelingJobArn())
&& Objects.equals(labelAttributeName(), other.labelAttributeName())
&& Objects.equals(inputConfig(), other.inputConfig()) && Objects.equals(outputConfig(), other.outputConfig())
&& Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(labelCategoryConfigS3Uri(), other.labelCategoryConfigS3Uri())
&& Objects.equals(stoppingConditions(), other.stoppingConditions())
&& Objects.equals(labelingJobAlgorithmsConfig(), other.labelingJobAlgorithmsConfig())
&& Objects.equals(humanTaskConfig(), other.humanTaskConfig()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(labelingJobOutput(), other.labelingJobOutput());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeLabelingJobResponse").add("LabelingJobStatus", labelingJobStatusAsString())
.add("LabelCounters", labelCounters()).add("FailureReason", failureReason()).add("CreationTime", creationTime())
.add("LastModifiedTime", lastModifiedTime()).add("JobReferenceCode", jobReferenceCode())
.add("LabelingJobName", labelingJobName()).add("LabelingJobArn", labelingJobArn())
.add("LabelAttributeName", labelAttributeName()).add("InputConfig", inputConfig())
.add("OutputConfig", outputConfig()).add("RoleArn", roleArn())
.add("LabelCategoryConfigS3Uri", labelCategoryConfigS3Uri()).add("StoppingConditions", stoppingConditions())
.add("LabelingJobAlgorithmsConfig", labelingJobAlgorithmsConfig()).add("HumanTaskConfig", humanTaskConfig())
.add("Tags", hasTags() ? tags() : null).add("LabelingJobOutput", labelingJobOutput()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LabelingJobStatus":
return Optional.ofNullable(clazz.cast(labelingJobStatusAsString()));
case "LabelCounters":
return Optional.ofNullable(clazz.cast(labelCounters()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "JobReferenceCode":
return Optional.ofNullable(clazz.cast(jobReferenceCode()));
case "LabelingJobName":
return Optional.ofNullable(clazz.cast(labelingJobName()));
case "LabelingJobArn":
return Optional.ofNullable(clazz.cast(labelingJobArn()));
case "LabelAttributeName":
return Optional.ofNullable(clazz.cast(labelAttributeName()));
case "InputConfig":
return Optional.ofNullable(clazz.cast(inputConfig()));
case "OutputConfig":
return Optional.ofNullable(clazz.cast(outputConfig()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "LabelCategoryConfigS3Uri":
return Optional.ofNullable(clazz.cast(labelCategoryConfigS3Uri()));
case "StoppingConditions":
return Optional.ofNullable(clazz.cast(stoppingConditions()));
case "LabelingJobAlgorithmsConfig":
return Optional.ofNullable(clazz.cast(labelingJobAlgorithmsConfig()));
case "HumanTaskConfig":
return Optional.ofNullable(clazz.cast(humanTaskConfig()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "LabelingJobOutput":
return Optional.ofNullable(clazz.cast(labelingJobOutput()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function