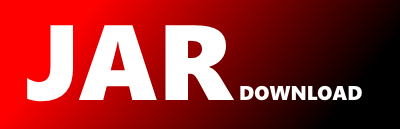
software.amazon.awssdk.services.sagemaker.model.ModelCardExportJobSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The summary of the Amazon SageMaker Model Card export job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModelCardExportJobSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MODEL_CARD_EXPORT_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelCardExportJobName").getter(getter(ModelCardExportJobSummary::modelCardExportJobName))
.setter(setter(Builder::modelCardExportJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelCardExportJobName").build())
.build();
private static final SdkField MODEL_CARD_EXPORT_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelCardExportJobArn").getter(getter(ModelCardExportJobSummary::modelCardExportJobArn))
.setter(setter(Builder::modelCardExportJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelCardExportJobArn").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ModelCardExportJobSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField MODEL_CARD_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelCardName").getter(getter(ModelCardExportJobSummary::modelCardName))
.setter(setter(Builder::modelCardName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelCardName").build()).build();
private static final SdkField MODEL_CARD_VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ModelCardVersion").getter(getter(ModelCardExportJobSummary::modelCardVersion))
.setter(setter(Builder::modelCardVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelCardVersion").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(ModelCardExportJobSummary::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField LAST_MODIFIED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedAt").getter(getter(ModelCardExportJobSummary::lastModifiedAt))
.setter(setter(Builder::lastModifiedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
MODEL_CARD_EXPORT_JOB_NAME_FIELD, MODEL_CARD_EXPORT_JOB_ARN_FIELD, STATUS_FIELD, MODEL_CARD_NAME_FIELD,
MODEL_CARD_VERSION_FIELD, CREATED_AT_FIELD, LAST_MODIFIED_AT_FIELD));
private static final long serialVersionUID = 1L;
private final String modelCardExportJobName;
private final String modelCardExportJobArn;
private final String status;
private final String modelCardName;
private final Integer modelCardVersion;
private final Instant createdAt;
private final Instant lastModifiedAt;
private ModelCardExportJobSummary(BuilderImpl builder) {
this.modelCardExportJobName = builder.modelCardExportJobName;
this.modelCardExportJobArn = builder.modelCardExportJobArn;
this.status = builder.status;
this.modelCardName = builder.modelCardName;
this.modelCardVersion = builder.modelCardVersion;
this.createdAt = builder.createdAt;
this.lastModifiedAt = builder.lastModifiedAt;
}
/**
*
* The name of the model card export job.
*
*
* @return The name of the model card export job.
*/
public final String modelCardExportJobName() {
return modelCardExportJobName;
}
/**
*
* The Amazon Resource Name (ARN) of the model card export job.
*
*
* @return The Amazon Resource Name (ARN) of the model card export job.
*/
public final String modelCardExportJobArn() {
return modelCardExportJobArn;
}
/**
*
* The completion status of the model card export job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelCardExportJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The completion status of the model card export job.
* @see ModelCardExportJobStatus
*/
public final ModelCardExportJobStatus status() {
return ModelCardExportJobStatus.fromValue(status);
}
/**
*
* The completion status of the model card export job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ModelCardExportJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The completion status of the model card export job.
* @see ModelCardExportJobStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The name of the model card that the export job exports.
*
*
* @return The name of the model card that the export job exports.
*/
public final String modelCardName() {
return modelCardName;
}
/**
*
* The version of the model card that the export job exports.
*
*
* @return The version of the model card that the export job exports.
*/
public final Integer modelCardVersion() {
return modelCardVersion;
}
/**
*
* The date and time that the model card export job was created.
*
*
* @return The date and time that the model card export job was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The date and time that the model card export job was last modified..
*
*
* @return The date and time that the model card export job was last modified..
*/
public final Instant lastModifiedAt() {
return lastModifiedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(modelCardExportJobName());
hashCode = 31 * hashCode + Objects.hashCode(modelCardExportJobArn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(modelCardName());
hashCode = 31 * hashCode + Objects.hashCode(modelCardVersion());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModelCardExportJobSummary)) {
return false;
}
ModelCardExportJobSummary other = (ModelCardExportJobSummary) obj;
return Objects.equals(modelCardExportJobName(), other.modelCardExportJobName())
&& Objects.equals(modelCardExportJobArn(), other.modelCardExportJobArn())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(modelCardName(), other.modelCardName())
&& Objects.equals(modelCardVersion(), other.modelCardVersion()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(lastModifiedAt(), other.lastModifiedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModelCardExportJobSummary").add("ModelCardExportJobName", modelCardExportJobName())
.add("ModelCardExportJobArn", modelCardExportJobArn()).add("Status", statusAsString())
.add("ModelCardName", modelCardName()).add("ModelCardVersion", modelCardVersion()).add("CreatedAt", createdAt())
.add("LastModifiedAt", lastModifiedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ModelCardExportJobName":
return Optional.ofNullable(clazz.cast(modelCardExportJobName()));
case "ModelCardExportJobArn":
return Optional.ofNullable(clazz.cast(modelCardExportJobArn()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "ModelCardName":
return Optional.ofNullable(clazz.cast(modelCardName()));
case "ModelCardVersion":
return Optional.ofNullable(clazz.cast(modelCardVersion()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "LastModifiedAt":
return Optional.ofNullable(clazz.cast(lastModifiedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function