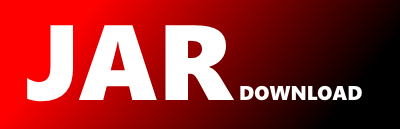
software.amazon.awssdk.services.sagemaker.model.TrialComponentMetricSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A summary of the metrics of a trial component.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TrialComponentMetricSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricName").getter(getter(TrialComponentMetricSummary::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField SOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceArn").getter(getter(TrialComponentMetricSummary::sourceArn)).setter(setter(Builder::sourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceArn").build()).build();
private static final SdkField TIME_STAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("TimeStamp").getter(getter(TrialComponentMetricSummary::timeStamp)).setter(setter(Builder::timeStamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeStamp").build()).build();
private static final SdkField MAX_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Max")
.getter(getter(TrialComponentMetricSummary::max)).setter(setter(Builder::max))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Max").build()).build();
private static final SdkField MIN_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Min")
.getter(getter(TrialComponentMetricSummary::min)).setter(setter(Builder::min))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Min").build()).build();
private static final SdkField LAST_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Last")
.getter(getter(TrialComponentMetricSummary::last)).setter(setter(Builder::last))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Last").build()).build();
private static final SdkField COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Count")
.getter(getter(TrialComponentMetricSummary::count)).setter(setter(Builder::count))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Count").build()).build();
private static final SdkField AVG_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Avg")
.getter(getter(TrialComponentMetricSummary::avg)).setter(setter(Builder::avg))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Avg").build()).build();
private static final SdkField STD_DEV_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("StdDev")
.getter(getter(TrialComponentMetricSummary::stdDev)).setter(setter(Builder::stdDev))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StdDev").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(METRIC_NAME_FIELD,
SOURCE_ARN_FIELD, TIME_STAMP_FIELD, MAX_FIELD, MIN_FIELD, LAST_FIELD, COUNT_FIELD, AVG_FIELD, STD_DEV_FIELD));
private static final long serialVersionUID = 1L;
private final String metricName;
private final String sourceArn;
private final Instant timeStamp;
private final Double max;
private final Double min;
private final Double last;
private final Integer count;
private final Double avg;
private final Double stdDev;
private TrialComponentMetricSummary(BuilderImpl builder) {
this.metricName = builder.metricName;
this.sourceArn = builder.sourceArn;
this.timeStamp = builder.timeStamp;
this.max = builder.max;
this.min = builder.min;
this.last = builder.last;
this.count = builder.count;
this.avg = builder.avg;
this.stdDev = builder.stdDev;
}
/**
*
* The name of the metric.
*
*
* @return The name of the metric.
*/
public final String metricName() {
return metricName;
}
/**
*
* The Amazon Resource Name (ARN) of the source.
*
*
* @return The Amazon Resource Name (ARN) of the source.
*/
public final String sourceArn() {
return sourceArn;
}
/**
*
* When the metric was last updated.
*
*
* @return When the metric was last updated.
*/
public final Instant timeStamp() {
return timeStamp;
}
/**
*
* The maximum value of the metric.
*
*
* @return The maximum value of the metric.
*/
public final Double max() {
return max;
}
/**
*
* The minimum value of the metric.
*
*
* @return The minimum value of the metric.
*/
public final Double min() {
return min;
}
/**
*
* The most recent value of the metric.
*
*
* @return The most recent value of the metric.
*/
public final Double last() {
return last;
}
/**
*
* The number of samples used to generate the metric.
*
*
* @return The number of samples used to generate the metric.
*/
public final Integer count() {
return count;
}
/**
*
* The average value of the metric.
*
*
* @return The average value of the metric.
*/
public final Double avg() {
return avg;
}
/**
*
* The standard deviation of the metric.
*
*
* @return The standard deviation of the metric.
*/
public final Double stdDev() {
return stdDev;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(sourceArn());
hashCode = 31 * hashCode + Objects.hashCode(timeStamp());
hashCode = 31 * hashCode + Objects.hashCode(max());
hashCode = 31 * hashCode + Objects.hashCode(min());
hashCode = 31 * hashCode + Objects.hashCode(last());
hashCode = 31 * hashCode + Objects.hashCode(count());
hashCode = 31 * hashCode + Objects.hashCode(avg());
hashCode = 31 * hashCode + Objects.hashCode(stdDev());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TrialComponentMetricSummary)) {
return false;
}
TrialComponentMetricSummary other = (TrialComponentMetricSummary) obj;
return Objects.equals(metricName(), other.metricName()) && Objects.equals(sourceArn(), other.sourceArn())
&& Objects.equals(timeStamp(), other.timeStamp()) && Objects.equals(max(), other.max())
&& Objects.equals(min(), other.min()) && Objects.equals(last(), other.last())
&& Objects.equals(count(), other.count()) && Objects.equals(avg(), other.avg())
&& Objects.equals(stdDev(), other.stdDev());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TrialComponentMetricSummary").add("MetricName", metricName()).add("SourceArn", sourceArn())
.add("TimeStamp", timeStamp()).add("Max", max()).add("Min", min()).add("Last", last()).add("Count", count())
.add("Avg", avg()).add("StdDev", stdDev()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "SourceArn":
return Optional.ofNullable(clazz.cast(sourceArn()));
case "TimeStamp":
return Optional.ofNullable(clazz.cast(timeStamp()));
case "Max":
return Optional.ofNullable(clazz.cast(max()));
case "Min":
return Optional.ofNullable(clazz.cast(min()));
case "Last":
return Optional.ofNullable(clazz.cast(last()));
case "Count":
return Optional.ofNullable(clazz.cast(count()));
case "Avg":
return Optional.ofNullable(clazz.cast(avg()));
case "StdDev":
return Optional.ofNullable(clazz.cast(stdDev()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy