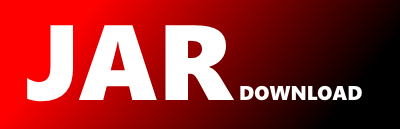
software.amazon.awssdk.services.sagemaker.model.CreateWorkteamRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateWorkteamRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField WORKTEAM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkteamName").getter(getter(CreateWorkteamRequest::workteamName)).setter(setter(Builder::workteamName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkteamName").build()).build();
private static final SdkField WORKFORCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkforceName").getter(getter(CreateWorkteamRequest::workforceName))
.setter(setter(Builder::workforceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkforceName").build()).build();
private static final SdkField> MEMBER_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MemberDefinitions")
.getter(getter(CreateWorkteamRequest::memberDefinitions))
.setter(setter(Builder::memberDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MemberDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateWorkteamRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField NOTIFICATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("NotificationConfiguration")
.getter(getter(CreateWorkteamRequest::notificationConfiguration)).setter(setter(Builder::notificationConfiguration))
.constructor(NotificationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationConfiguration").build())
.build();
private static final SdkField WORKER_ACCESS_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("WorkerAccessConfiguration")
.getter(getter(CreateWorkteamRequest::workerAccessConfiguration)).setter(setter(Builder::workerAccessConfiguration))
.constructor(WorkerAccessConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkerAccessConfiguration").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateWorkteamRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKTEAM_NAME_FIELD,
WORKFORCE_NAME_FIELD, MEMBER_DEFINITIONS_FIELD, DESCRIPTION_FIELD, NOTIFICATION_CONFIGURATION_FIELD,
WORKER_ACCESS_CONFIGURATION_FIELD, TAGS_FIELD));
private final String workteamName;
private final String workforceName;
private final List memberDefinitions;
private final String description;
private final NotificationConfiguration notificationConfiguration;
private final WorkerAccessConfiguration workerAccessConfiguration;
private final List tags;
private CreateWorkteamRequest(BuilderImpl builder) {
super(builder);
this.workteamName = builder.workteamName;
this.workforceName = builder.workforceName;
this.memberDefinitions = builder.memberDefinitions;
this.description = builder.description;
this.notificationConfiguration = builder.notificationConfiguration;
this.workerAccessConfiguration = builder.workerAccessConfiguration;
this.tags = builder.tags;
}
/**
*
* The name of the work team. Use this name to identify the work team.
*
*
* @return The name of the work team. Use this name to identify the work team.
*/
public final String workteamName() {
return workteamName;
}
/**
*
* The name of the workforce.
*
*
* @return The name of the workforce.
*/
public final String workforceName() {
return workforceName;
}
/**
* For responses, this returns true if the service returned a value for the MemberDefinitions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMemberDefinitions() {
return memberDefinitions != null && !(memberDefinitions instanceof SdkAutoConstructList);
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMemberDefinitions} method.
*
*
* @return A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input
* for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an existing
* worker pool, see Adding groups to a User Pool. For more information about user pools, see
*
* Amazon Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
*/
public final List memberDefinitions() {
return memberDefinitions;
}
/**
*
* A description of the work team.
*
*
* @return A description of the work team.
*/
public final String description() {
return description;
}
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*
* @return Configures notification of workers regarding available or expiring work items.
*/
public final NotificationConfiguration notificationConfiguration() {
return notificationConfiguration;
}
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using supported
* IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon S3 presigned
* URL.
*
*
* @return Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a
* Amazon S3 presigned URL.
*/
public final WorkerAccessConfiguration workerAccessConfiguration() {
return workerAccessConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return An array of key-value pairs.
*
* For more information, see Resource Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(workteamName());
hashCode = 31 * hashCode + Objects.hashCode(workforceName());
hashCode = 31 * hashCode + Objects.hashCode(hasMemberDefinitions() ? memberDefinitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(notificationConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(workerAccessConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateWorkteamRequest)) {
return false;
}
CreateWorkteamRequest other = (CreateWorkteamRequest) obj;
return Objects.equals(workteamName(), other.workteamName()) && Objects.equals(workforceName(), other.workforceName())
&& hasMemberDefinitions() == other.hasMemberDefinitions()
&& Objects.equals(memberDefinitions(), other.memberDefinitions())
&& Objects.equals(description(), other.description())
&& Objects.equals(notificationConfiguration(), other.notificationConfiguration())
&& Objects.equals(workerAccessConfiguration(), other.workerAccessConfiguration()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateWorkteamRequest").add("WorkteamName", workteamName())
.add("WorkforceName", workforceName())
.add("MemberDefinitions", hasMemberDefinitions() ? memberDefinitions() : null).add("Description", description())
.add("NotificationConfiguration", notificationConfiguration())
.add("WorkerAccessConfiguration", workerAccessConfiguration()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "WorkteamName":
return Optional.ofNullable(clazz.cast(workteamName()));
case "WorkforceName":
return Optional.ofNullable(clazz.cast(workforceName()));
case "MemberDefinitions":
return Optional.ofNullable(clazz.cast(memberDefinitions()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "NotificationConfiguration":
return Optional.ofNullable(clazz.cast(notificationConfiguration()));
case "WorkerAccessConfiguration":
return Optional.ofNullable(clazz.cast(workerAccessConfiguration()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces
* created using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not
* provide input for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an
* existing worker pool, see Adding groups to a User Pool. For more information about user
* pools, see Amazon Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in
* your private work team in OidcMemberDefinition
by listing those groups in
* Groups
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder memberDefinitions(Collection memberDefinitions);
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up
* the work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input for
* both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the CognitoMemberDefinition
* objects that make up the member definition must have the same ClientId
and UserPool
* values. To add a Amazon Cognito user group to an existing worker pool, see Adding groups to a User
* Pool. For more information about user pools, see Amazon
* Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* @param memberDefinitions
* A list of MemberDefinition
objects that contains objects that identify the workers that
* make up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces
* created using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not
* provide input for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an
* existing worker pool, see Adding groups to a User Pool. For more information about user
* pools, see Amazon Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in
* your private work team in OidcMemberDefinition
by listing those groups in
* Groups
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder memberDefinitions(MemberDefinition... memberDefinitions);
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up
* the work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input for
* both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the CognitoMemberDefinition
* objects that make up the member definition must have the same ClientId
and UserPool
* values. To add a Amazon Cognito user group to an existing worker pool, see Adding groups to a User
* Pool. For more information about user pools, see Amazon
* Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.sagemaker.model.MemberDefinition.Builder} avoiding the need to create
* one manually via {@link software.amazon.awssdk.services.sagemaker.model.MemberDefinition#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.sagemaker.model.MemberDefinition.Builder#build()} is called
* immediately and its result is passed to {@link #memberDefinitions(List)}.
*
* @param memberDefinitions
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.sagemaker.model.MemberDefinition.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #memberDefinitions(java.util.Collection)
*/
Builder memberDefinitions(Consumer... memberDefinitions);
/**
*
* A description of the work team.
*
*
* @param description
* A description of the work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*
* @param notificationConfiguration
* Configures notification of workers regarding available or expiring work items.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationConfiguration(NotificationConfiguration notificationConfiguration);
/**
*
* Configures notification of workers regarding available or expiring work items.
*
* This is a convenience method that creates an instance of the {@link NotificationConfiguration.Builder}
* avoiding the need to create one manually via {@link NotificationConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link NotificationConfiguration.Builder#build()} is called immediately
* and its result is passed to {@link #notificationConfiguration(NotificationConfiguration)}.
*
* @param notificationConfiguration
* a consumer that will call methods on {@link NotificationConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #notificationConfiguration(NotificationConfiguration)
*/
default Builder notificationConfiguration(Consumer notificationConfiguration) {
return notificationConfiguration(NotificationConfiguration.builder().applyMutation(notificationConfiguration).build());
}
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon
* S3 presigned URL.
*
*
* @param workerAccessConfiguration
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a
* Amazon S3 presigned URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder workerAccessConfiguration(WorkerAccessConfiguration workerAccessConfiguration);
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon
* S3 presigned URL.
*
* This is a convenience method that creates an instance of the {@link WorkerAccessConfiguration.Builder}
* avoiding the need to create one manually via {@link WorkerAccessConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link WorkerAccessConfiguration.Builder#build()} is called immediately
* and its result is passed to {@link #workerAccessConfiguration(WorkerAccessConfiguration)}.
*
* @param workerAccessConfiguration
* a consumer that will call methods on {@link WorkerAccessConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #workerAccessConfiguration(WorkerAccessConfiguration)
*/
default Builder workerAccessConfiguration(Consumer workerAccessConfiguration) {
return workerAccessConfiguration(WorkerAccessConfiguration.builder().applyMutation(workerAccessConfiguration).build());
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* @param tags
* An array of key-value pairs.
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User
* Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* @param tags
* An array of key-value pairs.
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User
* Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.sagemaker.model.Tag.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.sagemaker.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.sagemaker.model.Tag.Builder#build()} is called immediately and its
* result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.sagemaker.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends SageMakerRequest.BuilderImpl implements Builder {
private String workteamName;
private String workforceName;
private List memberDefinitions = DefaultSdkAutoConstructList.getInstance();
private String description;
private NotificationConfiguration notificationConfiguration;
private WorkerAccessConfiguration workerAccessConfiguration;
private List tags = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(CreateWorkteamRequest model) {
super(model);
workteamName(model.workteamName);
workforceName(model.workforceName);
memberDefinitions(model.memberDefinitions);
description(model.description);
notificationConfiguration(model.notificationConfiguration);
workerAccessConfiguration(model.workerAccessConfiguration);
tags(model.tags);
}
public final String getWorkteamName() {
return workteamName;
}
public final void setWorkteamName(String workteamName) {
this.workteamName = workteamName;
}
@Override
public final Builder workteamName(String workteamName) {
this.workteamName = workteamName;
return this;
}
public final String getWorkforceName() {
return workforceName;
}
public final void setWorkforceName(String workforceName) {
this.workforceName = workforceName;
}
@Override
public final Builder workforceName(String workforceName) {
this.workforceName = workforceName;
return this;
}
public final List getMemberDefinitions() {
List result = MemberDefinitionsCopier.copyToBuilder(this.memberDefinitions);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setMemberDefinitions(Collection memberDefinitions) {
this.memberDefinitions = MemberDefinitionsCopier.copyFromBuilder(memberDefinitions);
}
@Override
public final Builder memberDefinitions(Collection memberDefinitions) {
this.memberDefinitions = MemberDefinitionsCopier.copy(memberDefinitions);
return this;
}
@Override
@SafeVarargs
public final Builder memberDefinitions(MemberDefinition... memberDefinitions) {
memberDefinitions(Arrays.asList(memberDefinitions));
return this;
}
@Override
@SafeVarargs
public final Builder memberDefinitions(Consumer... memberDefinitions) {
memberDefinitions(Stream.of(memberDefinitions).map(c -> MemberDefinition.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getDescription() {
return description;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final NotificationConfiguration.Builder getNotificationConfiguration() {
return notificationConfiguration != null ? notificationConfiguration.toBuilder() : null;
}
public final void setNotificationConfiguration(NotificationConfiguration.BuilderImpl notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration != null ? notificationConfiguration.build() : null;
}
@Override
public final Builder notificationConfiguration(NotificationConfiguration notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration;
return this;
}
public final WorkerAccessConfiguration.Builder getWorkerAccessConfiguration() {
return workerAccessConfiguration != null ? workerAccessConfiguration.toBuilder() : null;
}
public final void setWorkerAccessConfiguration(WorkerAccessConfiguration.BuilderImpl workerAccessConfiguration) {
this.workerAccessConfiguration = workerAccessConfiguration != null ? workerAccessConfiguration.build() : null;
}
@Override
public final Builder workerAccessConfiguration(WorkerAccessConfiguration workerAccessConfiguration) {
this.workerAccessConfiguration = workerAccessConfiguration;
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateWorkteamRequest build() {
return new CreateWorkteamRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}