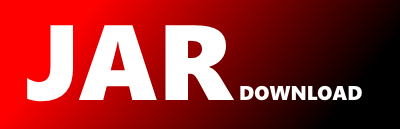
software.amazon.awssdk.services.sagemaker.model.Endpoint Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A hosted endpoint for real-time inference.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Endpoint implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ENDPOINT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointName").getter(getter(Endpoint::endpointName)).setter(setter(Builder::endpointName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointName").build()).build();
private static final SdkField ENDPOINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointArn").getter(getter(Endpoint::endpointArn)).setter(setter(Builder::endpointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointArn").build()).build();
private static final SdkField ENDPOINT_CONFIG_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointConfigName").getter(getter(Endpoint::endpointConfigName))
.setter(setter(Builder::endpointConfigName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointConfigName").build())
.build();
private static final SdkField> PRODUCTION_VARIANTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ProductionVariants")
.getter(getter(Endpoint::productionVariants))
.setter(setter(Builder::productionVariants))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductionVariants").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProductionVariantSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATA_CAPTURE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DataCaptureConfig")
.getter(getter(Endpoint::dataCaptureConfig)).setter(setter(Builder::dataCaptureConfig))
.constructor(DataCaptureConfigSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataCaptureConfig").build()).build();
private static final SdkField ENDPOINT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointStatus").getter(getter(Endpoint::endpointStatusAsString))
.setter(setter(Builder::endpointStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointStatus").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(Endpoint::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(Endpoint::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(Endpoint::lastModifiedTime)).setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField> MONITORING_SCHEDULES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MonitoringSchedules")
.getter(getter(Endpoint::monitoringSchedules))
.setter(setter(Builder::monitoringSchedules))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MonitoringSchedules").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MonitoringSchedule::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Endpoint::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SHADOW_PRODUCTION_VARIANTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ShadowProductionVariants")
.getter(getter(Endpoint::shadowProductionVariants))
.setter(setter(Builder::shadowProductionVariants))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShadowProductionVariants").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProductionVariantSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENDPOINT_NAME_FIELD,
ENDPOINT_ARN_FIELD, ENDPOINT_CONFIG_NAME_FIELD, PRODUCTION_VARIANTS_FIELD, DATA_CAPTURE_CONFIG_FIELD,
ENDPOINT_STATUS_FIELD, FAILURE_REASON_FIELD, CREATION_TIME_FIELD, LAST_MODIFIED_TIME_FIELD,
MONITORING_SCHEDULES_FIELD, TAGS_FIELD, SHADOW_PRODUCTION_VARIANTS_FIELD));
private static final long serialVersionUID = 1L;
private final String endpointName;
private final String endpointArn;
private final String endpointConfigName;
private final List productionVariants;
private final DataCaptureConfigSummary dataCaptureConfig;
private final String endpointStatus;
private final String failureReason;
private final Instant creationTime;
private final Instant lastModifiedTime;
private final List monitoringSchedules;
private final List tags;
private final List shadowProductionVariants;
private Endpoint(BuilderImpl builder) {
this.endpointName = builder.endpointName;
this.endpointArn = builder.endpointArn;
this.endpointConfigName = builder.endpointConfigName;
this.productionVariants = builder.productionVariants;
this.dataCaptureConfig = builder.dataCaptureConfig;
this.endpointStatus = builder.endpointStatus;
this.failureReason = builder.failureReason;
this.creationTime = builder.creationTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.monitoringSchedules = builder.monitoringSchedules;
this.tags = builder.tags;
this.shadowProductionVariants = builder.shadowProductionVariants;
}
/**
*
* The name of the endpoint.
*
*
* @return The name of the endpoint.
*/
public final String endpointName() {
return endpointName;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint.
*
*
* @return The Amazon Resource Name (ARN) of the endpoint.
*/
public final String endpointArn() {
return endpointArn;
}
/**
*
* The endpoint configuration associated with the endpoint.
*
*
* @return The endpoint configuration associated with the endpoint.
*/
public final String endpointConfigName() {
return endpointConfigName;
}
/**
* For responses, this returns true if the service returned a value for the ProductionVariants property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasProductionVariants() {
return productionVariants != null && !(productionVariants instanceof SdkAutoConstructList);
}
/**
*
* A list of the production variants hosted on the endpoint. Each production variant is a model.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasProductionVariants} method.
*
*
* @return A list of the production variants hosted on the endpoint. Each production variant is a model.
*/
public final List productionVariants() {
return productionVariants;
}
/**
* Returns the value of the DataCaptureConfig property for this object.
*
* @return The value of the DataCaptureConfig property for this object.
*/
public final DataCaptureConfigSummary dataCaptureConfig() {
return dataCaptureConfig;
}
/**
*
* The status of the endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointStatus}
* will return {@link EndpointStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #endpointStatusAsString}.
*
*
* @return The status of the endpoint.
* @see EndpointStatus
*/
public final EndpointStatus endpointStatus() {
return EndpointStatus.fromValue(endpointStatus);
}
/**
*
* The status of the endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointStatus}
* will return {@link EndpointStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #endpointStatusAsString}.
*
*
* @return The status of the endpoint.
* @see EndpointStatus
*/
public final String endpointStatusAsString() {
return endpointStatus;
}
/**
*
* If the endpoint failed, the reason it failed.
*
*
* @return If the endpoint failed, the reason it failed.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* The time that the endpoint was created.
*
*
* @return The time that the endpoint was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The last time the endpoint was modified.
*
*
* @return The last time the endpoint was modified.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
* For responses, this returns true if the service returned a value for the MonitoringSchedules property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMonitoringSchedules() {
return monitoringSchedules != null && !(monitoringSchedules instanceof SdkAutoConstructList);
}
/**
*
* A list of monitoring schedules for the endpoint. For information about model monitoring, see Amazon SageMaker Model Monitor.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMonitoringSchedules} method.
*
*
* @return A list of monitoring schedules for the endpoint. For information about model monitoring, see Amazon SageMaker Model
* Monitor.
*/
public final List monitoringSchedules() {
return monitoringSchedules;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of the tags associated with the endpoint. For more information, see Tagging Amazon Web Services resources
* in the Amazon Web Services General Reference Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of the tags associated with the endpoint. For more information, see Tagging Amazon Web Services
* resources in the Amazon Web Services General Reference Guide.
*/
public final List tags() {
return tags;
}
/**
* For responses, this returns true if the service returned a value for the ShadowProductionVariants property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasShadowProductionVariants() {
return shadowProductionVariants != null && !(shadowProductionVariants instanceof SdkAutoConstructList);
}
/**
*
* A list of the shadow variants hosted on the endpoint. Each shadow variant is a model in shadow mode with
* production traffic replicated from the production variant.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasShadowProductionVariants} method.
*
*
* @return A list of the shadow variants hosted on the endpoint. Each shadow variant is a model in shadow mode with
* production traffic replicated from the production variant.
*/
public final List shadowProductionVariants() {
return shadowProductionVariants;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(endpointName());
hashCode = 31 * hashCode + Objects.hashCode(endpointArn());
hashCode = 31 * hashCode + Objects.hashCode(endpointConfigName());
hashCode = 31 * hashCode + Objects.hashCode(hasProductionVariants() ? productionVariants() : null);
hashCode = 31 * hashCode + Objects.hashCode(dataCaptureConfig());
hashCode = 31 * hashCode + Objects.hashCode(endpointStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(hasMonitoringSchedules() ? monitoringSchedules() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasShadowProductionVariants() ? shadowProductionVariants() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Endpoint)) {
return false;
}
Endpoint other = (Endpoint) obj;
return Objects.equals(endpointName(), other.endpointName()) && Objects.equals(endpointArn(), other.endpointArn())
&& Objects.equals(endpointConfigName(), other.endpointConfigName())
&& hasProductionVariants() == other.hasProductionVariants()
&& Objects.equals(productionVariants(), other.productionVariants())
&& Objects.equals(dataCaptureConfig(), other.dataCaptureConfig())
&& Objects.equals(endpointStatusAsString(), other.endpointStatusAsString())
&& Objects.equals(failureReason(), other.failureReason()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& hasMonitoringSchedules() == other.hasMonitoringSchedules()
&& Objects.equals(monitoringSchedules(), other.monitoringSchedules()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && hasShadowProductionVariants() == other.hasShadowProductionVariants()
&& Objects.equals(shadowProductionVariants(), other.shadowProductionVariants());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Endpoint").add("EndpointName", endpointName()).add("EndpointArn", endpointArn())
.add("EndpointConfigName", endpointConfigName())
.add("ProductionVariants", hasProductionVariants() ? productionVariants() : null)
.add("DataCaptureConfig", dataCaptureConfig()).add("EndpointStatus", endpointStatusAsString())
.add("FailureReason", failureReason()).add("CreationTime", creationTime())
.add("LastModifiedTime", lastModifiedTime())
.add("MonitoringSchedules", hasMonitoringSchedules() ? monitoringSchedules() : null)
.add("Tags", hasTags() ? tags() : null)
.add("ShadowProductionVariants", hasShadowProductionVariants() ? shadowProductionVariants() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndpointName":
return Optional.ofNullable(clazz.cast(endpointName()));
case "EndpointArn":
return Optional.ofNullable(clazz.cast(endpointArn()));
case "EndpointConfigName":
return Optional.ofNullable(clazz.cast(endpointConfigName()));
case "ProductionVariants":
return Optional.ofNullable(clazz.cast(productionVariants()));
case "DataCaptureConfig":
return Optional.ofNullable(clazz.cast(dataCaptureConfig()));
case "EndpointStatus":
return Optional.ofNullable(clazz.cast(endpointStatusAsString()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "MonitoringSchedules":
return Optional.ofNullable(clazz.cast(monitoringSchedules()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ShadowProductionVariants":
return Optional.ofNullable(clazz.cast(shadowProductionVariants()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function