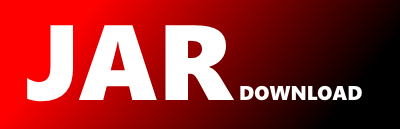
software.amazon.awssdk.services.sagemaker.model.CreateDomainRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateDomainRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField DOMAIN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DomainName").getter(getter(CreateDomainRequest::domainName)).setter(setter(Builder::domainName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainName").build()).build();
private static final SdkField AUTH_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthMode").getter(getter(CreateDomainRequest::authModeAsString)).setter(setter(Builder::authMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthMode").build()).build();
private static final SdkField DEFAULT_USER_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DefaultUserSettings")
.getter(getter(CreateDomainRequest::defaultUserSettings)).setter(setter(Builder::defaultUserSettings))
.constructor(UserSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultUserSettings").build())
.build();
private static final SdkField DOMAIN_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DomainSettings")
.getter(getter(CreateDomainRequest::domainSettings)).setter(setter(Builder::domainSettings))
.constructor(DomainSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainSettings").build()).build();
private static final SdkField> SUBNET_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SubnetIds")
.getter(getter(CreateDomainRequest::subnetIds))
.setter(setter(Builder::subnetIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(CreateDomainRequest::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateDomainRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField APP_NETWORK_ACCESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AppNetworkAccessType").getter(getter(CreateDomainRequest::appNetworkAccessTypeAsString))
.setter(setter(Builder::appNetworkAccessType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppNetworkAccessType").build())
.build();
private static final SdkField HOME_EFS_FILE_SYSTEM_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING).memberName("HomeEfsFileSystemKmsKeyId")
.getter(getter(CreateDomainRequest::homeEfsFileSystemKmsKeyId)).setter(setter(Builder::homeEfsFileSystemKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HomeEfsFileSystemKmsKeyId").build())
.build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(CreateDomainRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField APP_SECURITY_GROUP_MANAGEMENT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AppSecurityGroupManagement")
.getter(getter(CreateDomainRequest::appSecurityGroupManagementAsString))
.setter(setter(Builder::appSecurityGroupManagement))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppSecurityGroupManagement").build())
.build();
private static final SdkField TAG_PROPAGATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TagPropagation").getter(getter(CreateDomainRequest::tagPropagationAsString))
.setter(setter(Builder::tagPropagation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagPropagation").build()).build();
private static final SdkField DEFAULT_SPACE_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DefaultSpaceSettings")
.getter(getter(CreateDomainRequest::defaultSpaceSettings)).setter(setter(Builder::defaultSpaceSettings))
.constructor(DefaultSpaceSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultSpaceSettings").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_NAME_FIELD,
AUTH_MODE_FIELD, DEFAULT_USER_SETTINGS_FIELD, DOMAIN_SETTINGS_FIELD, SUBNET_IDS_FIELD, VPC_ID_FIELD, TAGS_FIELD,
APP_NETWORK_ACCESS_TYPE_FIELD, HOME_EFS_FILE_SYSTEM_KMS_KEY_ID_FIELD, KMS_KEY_ID_FIELD,
APP_SECURITY_GROUP_MANAGEMENT_FIELD, TAG_PROPAGATION_FIELD, DEFAULT_SPACE_SETTINGS_FIELD));
private final String domainName;
private final String authMode;
private final UserSettings defaultUserSettings;
private final DomainSettings domainSettings;
private final List subnetIds;
private final String vpcId;
private final List tags;
private final String appNetworkAccessType;
private final String homeEfsFileSystemKmsKeyId;
private final String kmsKeyId;
private final String appSecurityGroupManagement;
private final String tagPropagation;
private final DefaultSpaceSettings defaultSpaceSettings;
private CreateDomainRequest(BuilderImpl builder) {
super(builder);
this.domainName = builder.domainName;
this.authMode = builder.authMode;
this.defaultUserSettings = builder.defaultUserSettings;
this.domainSettings = builder.domainSettings;
this.subnetIds = builder.subnetIds;
this.vpcId = builder.vpcId;
this.tags = builder.tags;
this.appNetworkAccessType = builder.appNetworkAccessType;
this.homeEfsFileSystemKmsKeyId = builder.homeEfsFileSystemKmsKeyId;
this.kmsKeyId = builder.kmsKeyId;
this.appSecurityGroupManagement = builder.appSecurityGroupManagement;
this.tagPropagation = builder.tagPropagation;
this.defaultSpaceSettings = builder.defaultSpaceSettings;
}
/**
*
* A name for the domain.
*
*
* @return A name for the domain.
*/
public final String domainName() {
return domainName;
}
/**
*
* The mode of authentication that members use to access the domain.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authMode} will
* return {@link AuthMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #authModeAsString}.
*
*
* @return The mode of authentication that members use to access the domain.
* @see AuthMode
*/
public final AuthMode authMode() {
return AuthMode.fromValue(authMode);
}
/**
*
* The mode of authentication that members use to access the domain.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authMode} will
* return {@link AuthMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #authModeAsString}.
*
*
* @return The mode of authentication that members use to access the domain.
* @see AuthMode
*/
public final String authModeAsString() {
return authMode;
}
/**
*
* The default settings to use to create a user profile when UserSettings
isn't specified in the call
* to the CreateUserProfile
API.
*
*
* SecurityGroups
is aggregated when specified in both calls. For all other settings in
* UserSettings
, the values specified in CreateUserProfile
take precedence over those
* specified in CreateDomain
.
*
*
* @return The default settings to use to create a user profile when UserSettings
isn't specified in
* the call to the CreateUserProfile
API.
*
* SecurityGroups
is aggregated when specified in both calls. For all other settings in
* UserSettings
, the values specified in CreateUserProfile
take precedence over
* those specified in CreateDomain
.
*/
public final UserSettings defaultUserSettings() {
return defaultUserSettings;
}
/**
*
* A collection of Domain
settings.
*
*
* @return A collection of Domain
settings.
*/
public final DomainSettings domainSettings() {
return domainSettings;
}
/**
* For responses, this returns true if the service returned a value for the SubnetIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSubnetIds() {
return subnetIds != null && !(subnetIds instanceof SdkAutoConstructList);
}
/**
*
* The VPC subnets that the domain uses for communication.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSubnetIds} method.
*
*
* @return The VPC subnets that the domain uses for communication.
*/
public final List subnetIds() {
return subnetIds;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* @return The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*/
public final String vpcId() {
return vpcId;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Tags to associated with the Domain. Each tag consists of a key and an optional value. Tag keys must be unique per
* resource. Tags are searchable using the Search
API.
*
*
* Tags that you specify for the Domain are also added to all Apps that the Domain launches.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Tags to associated with the Domain. Each tag consists of a key and an optional value. Tag keys must be
* unique per resource. Tags are searchable using the Search
API.
*
* Tags that you specify for the Domain are also added to all Apps that the Domain launches.
*/
public final List tags() {
return tags;
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #appNetworkAccessType} will return {@link AppNetworkAccessType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #appNetworkAccessTypeAsString}.
*
*
* @return Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @see AppNetworkAccessType
*/
public final AppNetworkAccessType appNetworkAccessType() {
return AppNetworkAccessType.fromValue(appNetworkAccessType);
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #appNetworkAccessType} will return {@link AppNetworkAccessType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #appNetworkAccessTypeAsString}.
*
*
* @return Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @see AppNetworkAccessType
*/
public final String appNetworkAccessTypeAsString() {
return appNetworkAccessType;
}
/**
*
* Use KmsKeyId
.
*
*
* @return Use KmsKeyId
.
* @deprecated This property is deprecated, use KmsKeyId instead.
*/
@Deprecated
public final String homeEfsFileSystemKmsKeyId() {
return homeEfsFileSystemKmsKeyId;
}
/**
*
* SageMaker uses Amazon Web Services KMS to encrypt EFS and EBS volumes attached to the domain with an Amazon Web
* Services managed key by default. For more control, specify a customer managed key.
*
*
* @return SageMaker uses Amazon Web Services KMS to encrypt EFS and EBS volumes attached to the domain with an
* Amazon Web Services managed key by default. For more control, specify a customer managed key.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided. If setting up
* the domain for use with RStudio, this value must be set to Service
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #appSecurityGroupManagement} will return {@link AppSecurityGroupManagement#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #appSecurityGroupManagementAsString}.
*
*
* @return The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and
* DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided. If setting
* up the domain for use with RStudio, this value must be set to Service
.
* @see AppSecurityGroupManagement
*/
public final AppSecurityGroupManagement appSecurityGroupManagement() {
return AppSecurityGroupManagement.fromValue(appSecurityGroupManagement);
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided. If setting up
* the domain for use with RStudio, this value must be set to Service
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #appSecurityGroupManagement} will return {@link AppSecurityGroupManagement#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #appSecurityGroupManagementAsString}.
*
*
* @return The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and
* DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided. If setting
* up the domain for use with RStudio, this value must be set to Service
.
* @see AppSecurityGroupManagement
*/
public final String appSecurityGroupManagementAsString() {
return appSecurityGroupManagement;
}
/**
*
* Indicates whether custom tag propagation is supported for the domain. Defaults to DISABLED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tagPropagation}
* will return {@link TagPropagation#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #tagPropagationAsString}.
*
*
* @return Indicates whether custom tag propagation is supported for the domain. Defaults to DISABLED
.
* @see TagPropagation
*/
public final TagPropagation tagPropagation() {
return TagPropagation.fromValue(tagPropagation);
}
/**
*
* Indicates whether custom tag propagation is supported for the domain. Defaults to DISABLED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tagPropagation}
* will return {@link TagPropagation#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #tagPropagationAsString}.
*
*
* @return Indicates whether custom tag propagation is supported for the domain. Defaults to DISABLED
.
* @see TagPropagation
*/
public final String tagPropagationAsString() {
return tagPropagation;
}
/**
*
* The default settings used to create a space.
*
*
* @return The default settings used to create a space.
*/
public final DefaultSpaceSettings defaultSpaceSettings() {
return defaultSpaceSettings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(domainName());
hashCode = 31 * hashCode + Objects.hashCode(authModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(defaultUserSettings());
hashCode = 31 * hashCode + Objects.hashCode(domainSettings());
hashCode = 31 * hashCode + Objects.hashCode(hasSubnetIds() ? subnetIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(appNetworkAccessTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(homeEfsFileSystemKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(appSecurityGroupManagementAsString());
hashCode = 31 * hashCode + Objects.hashCode(tagPropagationAsString());
hashCode = 31 * hashCode + Objects.hashCode(defaultSpaceSettings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateDomainRequest)) {
return false;
}
CreateDomainRequest other = (CreateDomainRequest) obj;
return Objects.equals(domainName(), other.domainName()) && Objects.equals(authModeAsString(), other.authModeAsString())
&& Objects.equals(defaultUserSettings(), other.defaultUserSettings())
&& Objects.equals(domainSettings(), other.domainSettings()) && hasSubnetIds() == other.hasSubnetIds()
&& Objects.equals(subnetIds(), other.subnetIds()) && Objects.equals(vpcId(), other.vpcId())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(appNetworkAccessTypeAsString(), other.appNetworkAccessTypeAsString())
&& Objects.equals(homeEfsFileSystemKmsKeyId(), other.homeEfsFileSystemKmsKeyId())
&& Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(appSecurityGroupManagementAsString(), other.appSecurityGroupManagementAsString())
&& Objects.equals(tagPropagationAsString(), other.tagPropagationAsString())
&& Objects.equals(defaultSpaceSettings(), other.defaultSpaceSettings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateDomainRequest").add("DomainName", domainName()).add("AuthMode", authModeAsString())
.add("DefaultUserSettings", defaultUserSettings()).add("DomainSettings", domainSettings())
.add("SubnetIds", hasSubnetIds() ? subnetIds() : null).add("VpcId", vpcId())
.add("Tags", hasTags() ? tags() : null).add("AppNetworkAccessType", appNetworkAccessTypeAsString())
.add("HomeEfsFileSystemKmsKeyId", homeEfsFileSystemKmsKeyId()).add("KmsKeyId", kmsKeyId())
.add("AppSecurityGroupManagement", appSecurityGroupManagementAsString())
.add("TagPropagation", tagPropagationAsString()).add("DefaultSpaceSettings", defaultSpaceSettings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DomainName":
return Optional.ofNullable(clazz.cast(domainName()));
case "AuthMode":
return Optional.ofNullable(clazz.cast(authModeAsString()));
case "DefaultUserSettings":
return Optional.ofNullable(clazz.cast(defaultUserSettings()));
case "DomainSettings":
return Optional.ofNullable(clazz.cast(domainSettings()));
case "SubnetIds":
return Optional.ofNullable(clazz.cast(subnetIds()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "AppNetworkAccessType":
return Optional.ofNullable(clazz.cast(appNetworkAccessTypeAsString()));
case "HomeEfsFileSystemKmsKeyId":
return Optional.ofNullable(clazz.cast(homeEfsFileSystemKmsKeyId()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "AppSecurityGroupManagement":
return Optional.ofNullable(clazz.cast(appSecurityGroupManagementAsString()));
case "TagPropagation":
return Optional.ofNullable(clazz.cast(tagPropagationAsString()));
case "DefaultSpaceSettings":
return Optional.ofNullable(clazz.cast(defaultSpaceSettings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function