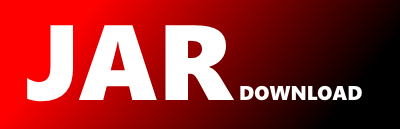
software.amazon.awssdk.services.sagemaker.model.DescribeCodeRepositoryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemaker Show documentation
Show all versions of sagemaker Show documentation
The AWS Java SDK for Amazon SageMaker module holds the client classes that are used for communicating
with Amazon SageMaker Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeCodeRepositoryResponse extends SageMakerResponse implements
ToCopyableBuilder {
private static final SdkField CODE_REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CodeRepositoryName").getter(getter(DescribeCodeRepositoryResponse::codeRepositoryName))
.setter(setter(Builder::codeRepositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeRepositoryName").build())
.build();
private static final SdkField CODE_REPOSITORY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CodeRepositoryArn").getter(getter(DescribeCodeRepositoryResponse::codeRepositoryArn))
.setter(setter(Builder::codeRepositoryArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeRepositoryArn").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(DescribeCodeRepositoryResponse::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(DescribeCodeRepositoryResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField GIT_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("GitConfig").getter(getter(DescribeCodeRepositoryResponse::gitConfig)).setter(setter(Builder::gitConfig))
.constructor(GitConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GitConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CODE_REPOSITORY_NAME_FIELD,
CODE_REPOSITORY_ARN_FIELD, CREATION_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, GIT_CONFIG_FIELD));
private final String codeRepositoryName;
private final String codeRepositoryArn;
private final Instant creationTime;
private final Instant lastModifiedTime;
private final GitConfig gitConfig;
private DescribeCodeRepositoryResponse(BuilderImpl builder) {
super(builder);
this.codeRepositoryName = builder.codeRepositoryName;
this.codeRepositoryArn = builder.codeRepositoryArn;
this.creationTime = builder.creationTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.gitConfig = builder.gitConfig;
}
/**
*
* The name of the Git repository.
*
*
* @return The name of the Git repository.
*/
public final String codeRepositoryName() {
return codeRepositoryName;
}
/**
*
* The Amazon Resource Name (ARN) of the Git repository.
*
*
* @return The Amazon Resource Name (ARN) of the Git repository.
*/
public final String codeRepositoryArn() {
return codeRepositoryArn;
}
/**
*
* The date and time that the repository was created.
*
*
* @return The date and time that the repository was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The date and time that the repository was last changed.
*
*
* @return The date and time that the repository was last changed.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* Configuration details about the repository, including the URL where the repository is located, the default
* branch, and the Amazon Resource Name (ARN) of the Amazon Web Services Secrets Manager secret that contains the
* credentials used to access the repository.
*
*
* @return Configuration details about the repository, including the URL where the repository is located, the
* default branch, and the Amazon Resource Name (ARN) of the Amazon Web Services Secrets Manager secret that
* contains the credentials used to access the repository.
*/
public final GitConfig gitConfig() {
return gitConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(codeRepositoryName());
hashCode = 31 * hashCode + Objects.hashCode(codeRepositoryArn());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(gitConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeCodeRepositoryResponse)) {
return false;
}
DescribeCodeRepositoryResponse other = (DescribeCodeRepositoryResponse) obj;
return Objects.equals(codeRepositoryName(), other.codeRepositoryName())
&& Objects.equals(codeRepositoryArn(), other.codeRepositoryArn())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime()) && Objects.equals(gitConfig(), other.gitConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeCodeRepositoryResponse").add("CodeRepositoryName", codeRepositoryName())
.add("CodeRepositoryArn", codeRepositoryArn()).add("CreationTime", creationTime())
.add("LastModifiedTime", lastModifiedTime()).add("GitConfig", gitConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CodeRepositoryName":
return Optional.ofNullable(clazz.cast(codeRepositoryName()));
case "CodeRepositoryArn":
return Optional.ofNullable(clazz.cast(codeRepositoryArn()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "GitConfig":
return Optional.ofNullable(clazz.cast(gitConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy