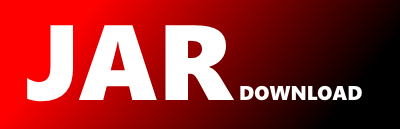
software.amazon.awssdk.services.sagemaker.model.DescribeInferenceComponentResponse Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeInferenceComponentResponse extends SageMakerResponse implements
ToCopyableBuilder {
private static final SdkField INFERENCE_COMPONENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InferenceComponentName").getter(getter(DescribeInferenceComponentResponse::inferenceComponentName))
.setter(setter(Builder::inferenceComponentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceComponentName").build())
.build();
private static final SdkField INFERENCE_COMPONENT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InferenceComponentArn").getter(getter(DescribeInferenceComponentResponse::inferenceComponentArn))
.setter(setter(Builder::inferenceComponentArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceComponentArn").build())
.build();
private static final SdkField ENDPOINT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointName").getter(getter(DescribeInferenceComponentResponse::endpointName))
.setter(setter(Builder::endpointName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointName").build()).build();
private static final SdkField ENDPOINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointArn").getter(getter(DescribeInferenceComponentResponse::endpointArn))
.setter(setter(Builder::endpointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointArn").build()).build();
private static final SdkField VARIANT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VariantName").getter(getter(DescribeInferenceComponentResponse::variantName))
.setter(setter(Builder::variantName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VariantName").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(DescribeInferenceComponentResponse::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Specification")
.getter(getter(DescribeInferenceComponentResponse::specification)).setter(setter(Builder::specification))
.constructor(InferenceComponentSpecificationSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Specification").build()).build();
private static final SdkField RUNTIME_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RuntimeConfig")
.getter(getter(DescribeInferenceComponentResponse::runtimeConfig)).setter(setter(Builder::runtimeConfig))
.constructor(InferenceComponentRuntimeConfigSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuntimeConfig").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(DescribeInferenceComponentResponse::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(DescribeInferenceComponentResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField INFERENCE_COMPONENT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InferenceComponentStatus")
.getter(getter(DescribeInferenceComponentResponse::inferenceComponentStatusAsString))
.setter(setter(Builder::inferenceComponentStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceComponentStatus").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
INFERENCE_COMPONENT_NAME_FIELD, INFERENCE_COMPONENT_ARN_FIELD, ENDPOINT_NAME_FIELD, ENDPOINT_ARN_FIELD,
VARIANT_NAME_FIELD, FAILURE_REASON_FIELD, SPECIFICATION_FIELD, RUNTIME_CONFIG_FIELD, CREATION_TIME_FIELD,
LAST_MODIFIED_TIME_FIELD, INFERENCE_COMPONENT_STATUS_FIELD));
private final String inferenceComponentName;
private final String inferenceComponentArn;
private final String endpointName;
private final String endpointArn;
private final String variantName;
private final String failureReason;
private final InferenceComponentSpecificationSummary specification;
private final InferenceComponentRuntimeConfigSummary runtimeConfig;
private final Instant creationTime;
private final Instant lastModifiedTime;
private final String inferenceComponentStatus;
private DescribeInferenceComponentResponse(BuilderImpl builder) {
super(builder);
this.inferenceComponentName = builder.inferenceComponentName;
this.inferenceComponentArn = builder.inferenceComponentArn;
this.endpointName = builder.endpointName;
this.endpointArn = builder.endpointArn;
this.variantName = builder.variantName;
this.failureReason = builder.failureReason;
this.specification = builder.specification;
this.runtimeConfig = builder.runtimeConfig;
this.creationTime = builder.creationTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.inferenceComponentStatus = builder.inferenceComponentStatus;
}
/**
*
* The name of the inference component.
*
*
* @return The name of the inference component.
*/
public final String inferenceComponentName() {
return inferenceComponentName;
}
/**
*
* The Amazon Resource Name (ARN) of the inference component.
*
*
* @return The Amazon Resource Name (ARN) of the inference component.
*/
public final String inferenceComponentArn() {
return inferenceComponentArn;
}
/**
*
* The name of the endpoint that hosts the inference component.
*
*
* @return The name of the endpoint that hosts the inference component.
*/
public final String endpointName() {
return endpointName;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint that hosts the inference component.
*
*
* @return The Amazon Resource Name (ARN) of the endpoint that hosts the inference component.
*/
public final String endpointArn() {
return endpointArn;
}
/**
*
* The name of the production variant that hosts the inference component.
*
*
* @return The name of the production variant that hosts the inference component.
*/
public final String variantName() {
return variantName;
}
/**
*
* If the inference component status is Failed
, the reason for the failure.
*
*
* @return If the inference component status is Failed
, the reason for the failure.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* Details about the resources that are deployed with this inference component.
*
*
* @return Details about the resources that are deployed with this inference component.
*/
public final InferenceComponentSpecificationSummary specification() {
return specification;
}
/**
*
* Details about the runtime settings for the model that is deployed with the inference component.
*
*
* @return Details about the runtime settings for the model that is deployed with the inference component.
*/
public final InferenceComponentRuntimeConfigSummary runtimeConfig() {
return runtimeConfig;
}
/**
*
* The time when the inference component was created.
*
*
* @return The time when the inference component was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The time when the inference component was last updated.
*
*
* @return The time when the inference component was last updated.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* The status of the inference component.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferenceComponentStatus} will return {@link InferenceComponentStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #inferenceComponentStatusAsString}.
*
*
* @return The status of the inference component.
* @see InferenceComponentStatus
*/
public final InferenceComponentStatus inferenceComponentStatus() {
return InferenceComponentStatus.fromValue(inferenceComponentStatus);
}
/**
*
* The status of the inference component.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferenceComponentStatus} will return {@link InferenceComponentStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #inferenceComponentStatusAsString}.
*
*
* @return The status of the inference component.
* @see InferenceComponentStatus
*/
public final String inferenceComponentStatusAsString() {
return inferenceComponentStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(inferenceComponentName());
hashCode = 31 * hashCode + Objects.hashCode(inferenceComponentArn());
hashCode = 31 * hashCode + Objects.hashCode(endpointName());
hashCode = 31 * hashCode + Objects.hashCode(endpointArn());
hashCode = 31 * hashCode + Objects.hashCode(variantName());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(specification());
hashCode = 31 * hashCode + Objects.hashCode(runtimeConfig());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(inferenceComponentStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeInferenceComponentResponse)) {
return false;
}
DescribeInferenceComponentResponse other = (DescribeInferenceComponentResponse) obj;
return Objects.equals(inferenceComponentName(), other.inferenceComponentName())
&& Objects.equals(inferenceComponentArn(), other.inferenceComponentArn())
&& Objects.equals(endpointName(), other.endpointName()) && Objects.equals(endpointArn(), other.endpointArn())
&& Objects.equals(variantName(), other.variantName()) && Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(specification(), other.specification())
&& Objects.equals(runtimeConfig(), other.runtimeConfig()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(inferenceComponentStatusAsString(), other.inferenceComponentStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeInferenceComponentResponse").add("InferenceComponentName", inferenceComponentName())
.add("InferenceComponentArn", inferenceComponentArn()).add("EndpointName", endpointName())
.add("EndpointArn", endpointArn()).add("VariantName", variantName()).add("FailureReason", failureReason())
.add("Specification", specification()).add("RuntimeConfig", runtimeConfig()).add("CreationTime", creationTime())
.add("LastModifiedTime", lastModifiedTime()).add("InferenceComponentStatus", inferenceComponentStatusAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InferenceComponentName":
return Optional.ofNullable(clazz.cast(inferenceComponentName()));
case "InferenceComponentArn":
return Optional.ofNullable(clazz.cast(inferenceComponentArn()));
case "EndpointName":
return Optional.ofNullable(clazz.cast(endpointName()));
case "EndpointArn":
return Optional.ofNullable(clazz.cast(endpointArn()));
case "VariantName":
return Optional.ofNullable(clazz.cast(variantName()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "Specification":
return Optional.ofNullable(clazz.cast(specification()));
case "RuntimeConfig":
return Optional.ofNullable(clazz.cast(runtimeConfig()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "InferenceComponentStatus":
return Optional.ofNullable(clazz.cast(inferenceComponentStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function