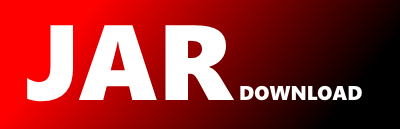
software.amazon.awssdk.services.sagemaker.model.DescribeInferenceRecommendationsJobResponse Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeInferenceRecommendationsJobResponse extends SageMakerResponse implements
ToCopyableBuilder {
private static final SdkField JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobName").getter(getter(DescribeInferenceRecommendationsJobResponse::jobName))
.setter(setter(Builder::jobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobName").build()).build();
private static final SdkField JOB_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobDescription").getter(getter(DescribeInferenceRecommendationsJobResponse::jobDescription))
.setter(setter(Builder::jobDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobDescription").build()).build();
private static final SdkField JOB_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobType").getter(getter(DescribeInferenceRecommendationsJobResponse::jobTypeAsString))
.setter(setter(Builder::jobType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobType").build()).build();
private static final SdkField JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("JobArn")
.getter(getter(DescribeInferenceRecommendationsJobResponse::jobArn)).setter(setter(Builder::jobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobArn").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(DescribeInferenceRecommendationsJobResponse::roleArn))
.setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DescribeInferenceRecommendationsJobResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(DescribeInferenceRecommendationsJobResponse::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField COMPLETION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompletionTime").getter(getter(DescribeInferenceRecommendationsJobResponse::completionTime))
.setter(setter(Builder::completionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(DescribeInferenceRecommendationsJobResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(DescribeInferenceRecommendationsJobResponse::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField INPUT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputConfig")
.getter(getter(DescribeInferenceRecommendationsJobResponse::inputConfig)).setter(setter(Builder::inputConfig))
.constructor(RecommendationJobInputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputConfig").build()).build();
private static final SdkField STOPPING_CONDITIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StoppingConditions")
.getter(getter(DescribeInferenceRecommendationsJobResponse::stoppingConditions))
.setter(setter(Builder::stoppingConditions)).constructor(RecommendationJobStoppingConditions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StoppingConditions").build())
.build();
private static final SdkField> INFERENCE_RECOMMENDATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InferenceRecommendations")
.getter(getter(DescribeInferenceRecommendationsJobResponse::inferenceRecommendations))
.setter(setter(Builder::inferenceRecommendations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceRecommendations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InferenceRecommendation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ENDPOINT_PERFORMANCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EndpointPerformances")
.getter(getter(DescribeInferenceRecommendationsJobResponse::endpointPerformances))
.setter(setter(Builder::endpointPerformances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointPerformances").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EndpointPerformance::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_NAME_FIELD,
JOB_DESCRIPTION_FIELD, JOB_TYPE_FIELD, JOB_ARN_FIELD, ROLE_ARN_FIELD, STATUS_FIELD, CREATION_TIME_FIELD,
COMPLETION_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, FAILURE_REASON_FIELD, INPUT_CONFIG_FIELD, STOPPING_CONDITIONS_FIELD,
INFERENCE_RECOMMENDATIONS_FIELD, ENDPOINT_PERFORMANCES_FIELD));
private final String jobName;
private final String jobDescription;
private final String jobType;
private final String jobArn;
private final String roleArn;
private final String status;
private final Instant creationTime;
private final Instant completionTime;
private final Instant lastModifiedTime;
private final String failureReason;
private final RecommendationJobInputConfig inputConfig;
private final RecommendationJobStoppingConditions stoppingConditions;
private final List inferenceRecommendations;
private final List endpointPerformances;
private DescribeInferenceRecommendationsJobResponse(BuilderImpl builder) {
super(builder);
this.jobName = builder.jobName;
this.jobDescription = builder.jobDescription;
this.jobType = builder.jobType;
this.jobArn = builder.jobArn;
this.roleArn = builder.roleArn;
this.status = builder.status;
this.creationTime = builder.creationTime;
this.completionTime = builder.completionTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.failureReason = builder.failureReason;
this.inputConfig = builder.inputConfig;
this.stoppingConditions = builder.stoppingConditions;
this.inferenceRecommendations = builder.inferenceRecommendations;
this.endpointPerformances = builder.endpointPerformances;
}
/**
*
* The name of the job. The name must be unique within an Amazon Web Services Region in the Amazon Web Services
* account.
*
*
* @return The name of the job. The name must be unique within an Amazon Web Services Region in the Amazon Web
* Services account.
*/
public final String jobName() {
return jobName;
}
/**
*
* The job description that you provided when you initiated the job.
*
*
* @return The job description that you provided when you initiated the job.
*/
public final String jobDescription() {
return jobDescription;
}
/**
*
* The job type that you provided when you initiated the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link RecommendationJobType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #jobTypeAsString}.
*
*
* @return The job type that you provided when you initiated the job.
* @see RecommendationJobType
*/
public final RecommendationJobType jobType() {
return RecommendationJobType.fromValue(jobType);
}
/**
*
* The job type that you provided when you initiated the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link RecommendationJobType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #jobTypeAsString}.
*
*
* @return The job type that you provided when you initiated the job.
* @see RecommendationJobType
*/
public final String jobTypeAsString() {
return jobType;
}
/**
*
* The Amazon Resource Name (ARN) of the job.
*
*
* @return The Amazon Resource Name (ARN) of the job.
*/
public final String jobArn() {
return jobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Web Services Identity and Access Management (IAM) role you provided
* when you initiated the job.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon Web Services Identity and Access Management (IAM) role you
* provided when you initiated the job.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RecommendationJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the job.
* @see RecommendationJobStatus
*/
public final RecommendationJobStatus status() {
return RecommendationJobStatus.fromValue(status);
}
/**
*
* The status of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RecommendationJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the job.
* @see RecommendationJobStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* A timestamp that shows when the job was created.
*
*
* @return A timestamp that shows when the job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* A timestamp that shows when the job completed.
*
*
* @return A timestamp that shows when the job completed.
*/
public final Instant completionTime() {
return completionTime;
}
/**
*
* A timestamp that shows when the job was last modified.
*
*
* @return A timestamp that shows when the job was last modified.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* If the job fails, provides information why the job failed.
*
*
* @return If the job fails, provides information why the job failed.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* Returns information about the versioned model package Amazon Resource Name (ARN), the traffic pattern, and
* endpoint configurations you provided when you initiated the job.
*
*
* @return Returns information about the versioned model package Amazon Resource Name (ARN), the traffic pattern,
* and endpoint configurations you provided when you initiated the job.
*/
public final RecommendationJobInputConfig inputConfig() {
return inputConfig;
}
/**
*
* The stopping conditions that you provided when you initiated the job.
*
*
* @return The stopping conditions that you provided when you initiated the job.
*/
public final RecommendationJobStoppingConditions stoppingConditions() {
return stoppingConditions;
}
/**
* For responses, this returns true if the service returned a value for the InferenceRecommendations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInferenceRecommendations() {
return inferenceRecommendations != null && !(inferenceRecommendations instanceof SdkAutoConstructList);
}
/**
*
* The recommendations made by Inference Recommender.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInferenceRecommendations} method.
*
*
* @return The recommendations made by Inference Recommender.
*/
public final List inferenceRecommendations() {
return inferenceRecommendations;
}
/**
* For responses, this returns true if the service returned a value for the EndpointPerformances property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEndpointPerformances() {
return endpointPerformances != null && !(endpointPerformances instanceof SdkAutoConstructList);
}
/**
*
* The performance results from running an Inference Recommender job on an existing endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEndpointPerformances} method.
*
*
* @return The performance results from running an Inference Recommender job on an existing endpoint.
*/
public final List endpointPerformances() {
return endpointPerformances;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(jobName());
hashCode = 31 * hashCode + Objects.hashCode(jobDescription());
hashCode = 31 * hashCode + Objects.hashCode(jobTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(jobArn());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(completionTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(inputConfig());
hashCode = 31 * hashCode + Objects.hashCode(stoppingConditions());
hashCode = 31 * hashCode + Objects.hashCode(hasInferenceRecommendations() ? inferenceRecommendations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasEndpointPerformances() ? endpointPerformances() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeInferenceRecommendationsJobResponse)) {
return false;
}
DescribeInferenceRecommendationsJobResponse other = (DescribeInferenceRecommendationsJobResponse) obj;
return Objects.equals(jobName(), other.jobName()) && Objects.equals(jobDescription(), other.jobDescription())
&& Objects.equals(jobTypeAsString(), other.jobTypeAsString()) && Objects.equals(jobArn(), other.jobArn())
&& Objects.equals(roleArn(), other.roleArn()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(completionTime(), other.completionTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(failureReason(), other.failureReason()) && Objects.equals(inputConfig(), other.inputConfig())
&& Objects.equals(stoppingConditions(), other.stoppingConditions())
&& hasInferenceRecommendations() == other.hasInferenceRecommendations()
&& Objects.equals(inferenceRecommendations(), other.inferenceRecommendations())
&& hasEndpointPerformances() == other.hasEndpointPerformances()
&& Objects.equals(endpointPerformances(), other.endpointPerformances());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeInferenceRecommendationsJobResponse").add("JobName", jobName())
.add("JobDescription", jobDescription()).add("JobType", jobTypeAsString()).add("JobArn", jobArn())
.add("RoleArn", roleArn()).add("Status", statusAsString()).add("CreationTime", creationTime())
.add("CompletionTime", completionTime()).add("LastModifiedTime", lastModifiedTime())
.add("FailureReason", failureReason()).add("InputConfig", inputConfig())
.add("StoppingConditions", stoppingConditions())
.add("InferenceRecommendations", hasInferenceRecommendations() ? inferenceRecommendations() : null)
.add("EndpointPerformances", hasEndpointPerformances() ? endpointPerformances() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "JobName":
return Optional.ofNullable(clazz.cast(jobName()));
case "JobDescription":
return Optional.ofNullable(clazz.cast(jobDescription()));
case "JobType":
return Optional.ofNullable(clazz.cast(jobTypeAsString()));
case "JobArn":
return Optional.ofNullable(clazz.cast(jobArn()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "CompletionTime":
return Optional.ofNullable(clazz.cast(completionTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "InputConfig":
return Optional.ofNullable(clazz.cast(inputConfig()));
case "StoppingConditions":
return Optional.ofNullable(clazz.cast(stoppingConditions()));
case "InferenceRecommendations":
return Optional.ofNullable(clazz.cast(inferenceRecommendations()));
case "EndpointPerformances":
return Optional.ofNullable(clazz.cast(endpointPerformances()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function