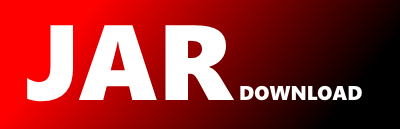
software.amazon.awssdk.services.sagemaker.model.ListHubContentVersionsRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListHubContentVersionsRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField HUB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HubName").getter(getter(ListHubContentVersionsRequest::hubName)).setter(setter(Builder::hubName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HubName").build()).build();
private static final SdkField HUB_CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HubContentType").getter(getter(ListHubContentVersionsRequest::hubContentTypeAsString))
.setter(setter(Builder::hubContentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HubContentType").build()).build();
private static final SdkField HUB_CONTENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HubContentName").getter(getter(ListHubContentVersionsRequest::hubContentName))
.setter(setter(Builder::hubContentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HubContentName").build()).build();
private static final SdkField MIN_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MinVersion").getter(getter(ListHubContentVersionsRequest::minVersion))
.setter(setter(Builder::minVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinVersion").build()).build();
private static final SdkField MAX_SCHEMA_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaxSchemaVersion").getter(getter(ListHubContentVersionsRequest::maxSchemaVersion))
.setter(setter(Builder::maxSchemaVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxSchemaVersion").build()).build();
private static final SdkField CREATION_TIME_BEFORE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTimeBefore").getter(getter(ListHubContentVersionsRequest::creationTimeBefore))
.setter(setter(Builder::creationTimeBefore))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTimeBefore").build())
.build();
private static final SdkField CREATION_TIME_AFTER_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTimeAfter").getter(getter(ListHubContentVersionsRequest::creationTimeAfter))
.setter(setter(Builder::creationTimeAfter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTimeAfter").build()).build();
private static final SdkField SORT_BY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("SortBy")
.getter(getter(ListHubContentVersionsRequest::sortByAsString)).setter(setter(Builder::sortBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SortBy").build()).build();
private static final SdkField SORT_ORDER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SortOrder").getter(getter(ListHubContentVersionsRequest::sortOrderAsString))
.setter(setter(Builder::sortOrder))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SortOrder").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxResults").getter(getter(ListHubContentVersionsRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxResults").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(ListHubContentVersionsRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(HUB_NAME_FIELD,
HUB_CONTENT_TYPE_FIELD, HUB_CONTENT_NAME_FIELD, MIN_VERSION_FIELD, MAX_SCHEMA_VERSION_FIELD,
CREATION_TIME_BEFORE_FIELD, CREATION_TIME_AFTER_FIELD, SORT_BY_FIELD, SORT_ORDER_FIELD, MAX_RESULTS_FIELD,
NEXT_TOKEN_FIELD));
private final String hubName;
private final String hubContentType;
private final String hubContentName;
private final String minVersion;
private final String maxSchemaVersion;
private final Instant creationTimeBefore;
private final Instant creationTimeAfter;
private final String sortBy;
private final String sortOrder;
private final Integer maxResults;
private final String nextToken;
private ListHubContentVersionsRequest(BuilderImpl builder) {
super(builder);
this.hubName = builder.hubName;
this.hubContentType = builder.hubContentType;
this.hubContentName = builder.hubContentName;
this.minVersion = builder.minVersion;
this.maxSchemaVersion = builder.maxSchemaVersion;
this.creationTimeBefore = builder.creationTimeBefore;
this.creationTimeAfter = builder.creationTimeAfter;
this.sortBy = builder.sortBy;
this.sortOrder = builder.sortOrder;
this.maxResults = builder.maxResults;
this.nextToken = builder.nextToken;
}
/**
*
* The name of the hub to list the content versions of.
*
*
* @return The name of the hub to list the content versions of.
*/
public final String hubName() {
return hubName;
}
/**
*
* The type of hub content to list versions of.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hubContentType}
* will return {@link HubContentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #hubContentTypeAsString}.
*
*
* @return The type of hub content to list versions of.
* @see HubContentType
*/
public final HubContentType hubContentType() {
return HubContentType.fromValue(hubContentType);
}
/**
*
* The type of hub content to list versions of.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hubContentType}
* will return {@link HubContentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #hubContentTypeAsString}.
*
*
* @return The type of hub content to list versions of.
* @see HubContentType
*/
public final String hubContentTypeAsString() {
return hubContentType;
}
/**
*
* The name of the hub content.
*
*
* @return The name of the hub content.
*/
public final String hubContentName() {
return hubContentName;
}
/**
*
* The lower bound of the hub content versions to list.
*
*
* @return The lower bound of the hub content versions to list.
*/
public final String minVersion() {
return minVersion;
}
/**
*
* The upper bound of the hub content schema version.
*
*
* @return The upper bound of the hub content schema version.
*/
public final String maxSchemaVersion() {
return maxSchemaVersion;
}
/**
*
* Only list hub content versions that were created before the time specified.
*
*
* @return Only list hub content versions that were created before the time specified.
*/
public final Instant creationTimeBefore() {
return creationTimeBefore;
}
/**
*
* Only list hub content versions that were created after the time specified.
*
*
* @return Only list hub content versions that were created after the time specified.
*/
public final Instant creationTimeAfter() {
return creationTimeAfter;
}
/**
*
* Sort hub content versions by either name or creation time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sortBy} will
* return {@link HubContentSortBy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sortByAsString}.
*
*
* @return Sort hub content versions by either name or creation time.
* @see HubContentSortBy
*/
public final HubContentSortBy sortBy() {
return HubContentSortBy.fromValue(sortBy);
}
/**
*
* Sort hub content versions by either name or creation time.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sortBy} will
* return {@link HubContentSortBy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sortByAsString}.
*
*
* @return Sort hub content versions by either name or creation time.
* @see HubContentSortBy
*/
public final String sortByAsString() {
return sortBy;
}
/**
*
* Sort hub content versions by ascending or descending order.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sortOrder} will
* return {@link SortOrder#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sortOrderAsString}.
*
*
* @return Sort hub content versions by ascending or descending order.
* @see SortOrder
*/
public final SortOrder sortOrder() {
return SortOrder.fromValue(sortOrder);
}
/**
*
* Sort hub content versions by ascending or descending order.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sortOrder} will
* return {@link SortOrder#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sortOrderAsString}.
*
*
* @return Sort hub content versions by ascending or descending order.
* @see SortOrder
*/
public final String sortOrderAsString() {
return sortOrder;
}
/**
*
* The maximum number of hub content versions to list.
*
*
* @return The maximum number of hub content versions to list.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* If the response to a previous ListHubContentVersions
request was truncated, the response includes a
* NextToken
. To retrieve the next set of hub content versions, use the token in the next request.
*
*
* @return If the response to a previous ListHubContentVersions
request was truncated, the response
* includes a NextToken
. To retrieve the next set of hub content versions, use the token in the
* next request.
*/
public final String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hubName());
hashCode = 31 * hashCode + Objects.hashCode(hubContentTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hubContentName());
hashCode = 31 * hashCode + Objects.hashCode(minVersion());
hashCode = 31 * hashCode + Objects.hashCode(maxSchemaVersion());
hashCode = 31 * hashCode + Objects.hashCode(creationTimeBefore());
hashCode = 31 * hashCode + Objects.hashCode(creationTimeAfter());
hashCode = 31 * hashCode + Objects.hashCode(sortByAsString());
hashCode = 31 * hashCode + Objects.hashCode(sortOrderAsString());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListHubContentVersionsRequest)) {
return false;
}
ListHubContentVersionsRequest other = (ListHubContentVersionsRequest) obj;
return Objects.equals(hubName(), other.hubName())
&& Objects.equals(hubContentTypeAsString(), other.hubContentTypeAsString())
&& Objects.equals(hubContentName(), other.hubContentName()) && Objects.equals(minVersion(), other.minVersion())
&& Objects.equals(maxSchemaVersion(), other.maxSchemaVersion())
&& Objects.equals(creationTimeBefore(), other.creationTimeBefore())
&& Objects.equals(creationTimeAfter(), other.creationTimeAfter())
&& Objects.equals(sortByAsString(), other.sortByAsString())
&& Objects.equals(sortOrderAsString(), other.sortOrderAsString())
&& Objects.equals(maxResults(), other.maxResults()) && Objects.equals(nextToken(), other.nextToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListHubContentVersionsRequest").add("HubName", hubName())
.add("HubContentType", hubContentTypeAsString()).add("HubContentName", hubContentName())
.add("MinVersion", minVersion()).add("MaxSchemaVersion", maxSchemaVersion())
.add("CreationTimeBefore", creationTimeBefore()).add("CreationTimeAfter", creationTimeAfter())
.add("SortBy", sortByAsString()).add("SortOrder", sortOrderAsString()).add("MaxResults", maxResults())
.add("NextToken", nextToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "HubName":
return Optional.ofNullable(clazz.cast(hubName()));
case "HubContentType":
return Optional.ofNullable(clazz.cast(hubContentTypeAsString()));
case "HubContentName":
return Optional.ofNullable(clazz.cast(hubContentName()));
case "MinVersion":
return Optional.ofNullable(clazz.cast(minVersion()));
case "MaxSchemaVersion":
return Optional.ofNullable(clazz.cast(maxSchemaVersion()));
case "CreationTimeBefore":
return Optional.ofNullable(clazz.cast(creationTimeBefore()));
case "CreationTimeAfter":
return Optional.ofNullable(clazz.cast(creationTimeAfter()));
case "SortBy":
return Optional.ofNullable(clazz.cast(sortByAsString()));
case "SortOrder":
return Optional.ofNullable(clazz.cast(sortOrderAsString()));
case "MaxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function