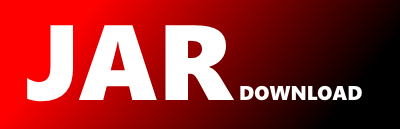
software.amazon.awssdk.services.sagemaker.model.AutoMLJobConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A collection of settings used for an AutoML job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AutoMLJobConfig implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField COMPLETION_CRITERIA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CompletionCriteria")
.getter(getter(AutoMLJobConfig::completionCriteria)).setter(setter(Builder::completionCriteria))
.constructor(AutoMLJobCompletionCriteria::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionCriteria").build())
.build();
private static final SdkField SECURITY_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SecurityConfig")
.getter(getter(AutoMLJobConfig::securityConfig)).setter(setter(Builder::securityConfig))
.constructor(AutoMLSecurityConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityConfig").build()).build();
private static final SdkField CANDIDATE_GENERATION_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CandidateGenerationConfig")
.getter(getter(AutoMLJobConfig::candidateGenerationConfig)).setter(setter(Builder::candidateGenerationConfig))
.constructor(AutoMLCandidateGenerationConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CandidateGenerationConfig").build())
.build();
private static final SdkField DATA_SPLIT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DataSplitConfig")
.getter(getter(AutoMLJobConfig::dataSplitConfig)).setter(setter(Builder::dataSplitConfig))
.constructor(AutoMLDataSplitConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSplitConfig").build()).build();
private static final SdkField MODE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Mode")
.getter(getter(AutoMLJobConfig::modeAsString)).setter(setter(Builder::mode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Mode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPLETION_CRITERIA_FIELD,
SECURITY_CONFIG_FIELD, CANDIDATE_GENERATION_CONFIG_FIELD, DATA_SPLIT_CONFIG_FIELD, MODE_FIELD));
private static final long serialVersionUID = 1L;
private final AutoMLJobCompletionCriteria completionCriteria;
private final AutoMLSecurityConfig securityConfig;
private final AutoMLCandidateGenerationConfig candidateGenerationConfig;
private final AutoMLDataSplitConfig dataSplitConfig;
private final String mode;
private AutoMLJobConfig(BuilderImpl builder) {
this.completionCriteria = builder.completionCriteria;
this.securityConfig = builder.securityConfig;
this.candidateGenerationConfig = builder.candidateGenerationConfig;
this.dataSplitConfig = builder.dataSplitConfig;
this.mode = builder.mode;
}
/**
*
* How long an AutoML job is allowed to run, or how many candidates a job is allowed to generate.
*
*
* @return How long an AutoML job is allowed to run, or how many candidates a job is allowed to generate.
*/
public final AutoMLJobCompletionCriteria completionCriteria() {
return completionCriteria;
}
/**
*
* The security configuration for traffic encryption or Amazon VPC settings.
*
*
* @return The security configuration for traffic encryption or Amazon VPC settings.
*/
public final AutoMLSecurityConfig securityConfig() {
return securityConfig;
}
/**
*
* The configuration for generating a candidate for an AutoML job (optional).
*
*
* @return The configuration for generating a candidate for an AutoML job (optional).
*/
public final AutoMLCandidateGenerationConfig candidateGenerationConfig() {
return candidateGenerationConfig;
}
/**
*
* The configuration for splitting the input training dataset.
*
*
* Type: AutoMLDataSplitConfig
*
*
* @return The configuration for splitting the input training dataset.
*
* Type: AutoMLDataSplitConfig
*/
public final AutoMLDataSplitConfig dataSplitConfig() {
return dataSplitConfig;
}
/**
*
* The method that Autopilot uses to train the data. You can either specify the mode manually or let Autopilot
* choose for you based on the dataset size by selecting AUTO
. In AUTO
mode, Autopilot
* chooses ENSEMBLING
for datasets smaller than 100 MB, and HYPERPARAMETER_TUNING
for
* larger ones.
*
*
* The ENSEMBLING
mode uses a multi-stack ensemble model to predict classification and regression tasks
* directly from your dataset. This machine learning mode combines several base models to produce an optimal
* predictive model. It then uses a stacking ensemble method to combine predictions from contributing members. A
* multi-stack ensemble model can provide better performance over a single model by combining the predictive
* capabilities of multiple models. See Autopilot algorithm support for a list of algorithms supported by ENSEMBLING
mode.
*
*
* The HYPERPARAMETER_TUNING
(HPO) mode uses the best hyperparameters to train the best version of a
* model. HPO automatically selects an algorithm for the type of problem you want to solve. Then HPO finds the best
* hyperparameters according to your objective metric. See Autopilot algorithm support for a list of algorithms supported by HYPERPARAMETER_TUNING
mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link AutoMLMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The method that Autopilot uses to train the data. You can either specify the mode manually or let
* Autopilot choose for you based on the dataset size by selecting AUTO
. In AUTO
* mode, Autopilot chooses ENSEMBLING
for datasets smaller than 100 MB, and
* HYPERPARAMETER_TUNING
for larger ones.
*
* The ENSEMBLING
mode uses a multi-stack ensemble model to predict classification and
* regression tasks directly from your dataset. This machine learning mode combines several base models to
* produce an optimal predictive model. It then uses a stacking ensemble method to combine predictions from
* contributing members. A multi-stack ensemble model can provide better performance over a single model by
* combining the predictive capabilities of multiple models. See Autopilot algorithm support for a list of algorithms supported by ENSEMBLING
mode.
*
*
* The HYPERPARAMETER_TUNING
(HPO) mode uses the best hyperparameters to train the best version
* of a model. HPO automatically selects an algorithm for the type of problem you want to solve. Then HPO
* finds the best hyperparameters according to your objective metric. See Autopilot algorithm support for a list of algorithms supported by HYPERPARAMETER_TUNING
* mode.
* @see AutoMLMode
*/
public final AutoMLMode mode() {
return AutoMLMode.fromValue(mode);
}
/**
*
* The method that Autopilot uses to train the data. You can either specify the mode manually or let Autopilot
* choose for you based on the dataset size by selecting AUTO
. In AUTO
mode, Autopilot
* chooses ENSEMBLING
for datasets smaller than 100 MB, and HYPERPARAMETER_TUNING
for
* larger ones.
*
*
* The ENSEMBLING
mode uses a multi-stack ensemble model to predict classification and regression tasks
* directly from your dataset. This machine learning mode combines several base models to produce an optimal
* predictive model. It then uses a stacking ensemble method to combine predictions from contributing members. A
* multi-stack ensemble model can provide better performance over a single model by combining the predictive
* capabilities of multiple models. See Autopilot algorithm support for a list of algorithms supported by ENSEMBLING
mode.
*
*
* The HYPERPARAMETER_TUNING
(HPO) mode uses the best hyperparameters to train the best version of a
* model. HPO automatically selects an algorithm for the type of problem you want to solve. Then HPO finds the best
* hyperparameters according to your objective metric. See Autopilot algorithm support for a list of algorithms supported by HYPERPARAMETER_TUNING
mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link AutoMLMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The method that Autopilot uses to train the data. You can either specify the mode manually or let
* Autopilot choose for you based on the dataset size by selecting AUTO
. In AUTO
* mode, Autopilot chooses ENSEMBLING
for datasets smaller than 100 MB, and
* HYPERPARAMETER_TUNING
for larger ones.
*
* The ENSEMBLING
mode uses a multi-stack ensemble model to predict classification and
* regression tasks directly from your dataset. This machine learning mode combines several base models to
* produce an optimal predictive model. It then uses a stacking ensemble method to combine predictions from
* contributing members. A multi-stack ensemble model can provide better performance over a single model by
* combining the predictive capabilities of multiple models. See Autopilot algorithm support for a list of algorithms supported by ENSEMBLING
mode.
*
*
* The HYPERPARAMETER_TUNING
(HPO) mode uses the best hyperparameters to train the best version
* of a model. HPO automatically selects an algorithm for the type of problem you want to solve. Then HPO
* finds the best hyperparameters according to your objective metric. See Autopilot algorithm support for a list of algorithms supported by HYPERPARAMETER_TUNING
* mode.
* @see AutoMLMode
*/
public final String modeAsString() {
return mode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(completionCriteria());
hashCode = 31 * hashCode + Objects.hashCode(securityConfig());
hashCode = 31 * hashCode + Objects.hashCode(candidateGenerationConfig());
hashCode = 31 * hashCode + Objects.hashCode(dataSplitConfig());
hashCode = 31 * hashCode + Objects.hashCode(modeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AutoMLJobConfig)) {
return false;
}
AutoMLJobConfig other = (AutoMLJobConfig) obj;
return Objects.equals(completionCriteria(), other.completionCriteria())
&& Objects.equals(securityConfig(), other.securityConfig())
&& Objects.equals(candidateGenerationConfig(), other.candidateGenerationConfig())
&& Objects.equals(dataSplitConfig(), other.dataSplitConfig())
&& Objects.equals(modeAsString(), other.modeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AutoMLJobConfig").add("CompletionCriteria", completionCriteria())
.add("SecurityConfig", securityConfig()).add("CandidateGenerationConfig", candidateGenerationConfig())
.add("DataSplitConfig", dataSplitConfig()).add("Mode", modeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CompletionCriteria":
return Optional.ofNullable(clazz.cast(completionCriteria()));
case "SecurityConfig":
return Optional.ofNullable(clazz.cast(securityConfig()));
case "CandidateGenerationConfig":
return Optional.ofNullable(clazz.cast(candidateGenerationConfig()));
case "DataSplitConfig":
return Optional.ofNullable(clazz.cast(dataSplitConfig()));
case "Mode":
return Optional.ofNullable(clazz.cast(modeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function