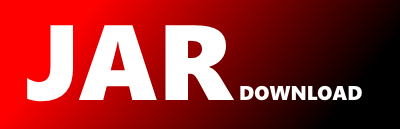
software.amazon.awssdk.services.sagemaker.model.DescribeAutoMlJobV2Response Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeAutoMlJobV2Response extends SageMakerResponse implements
ToCopyableBuilder {
private static final SdkField AUTO_ML_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobName").getter(getter(DescribeAutoMlJobV2Response::autoMLJobName))
.setter(setter(Builder::autoMLJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobName").build()).build();
private static final SdkField AUTO_ML_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobArn").getter(getter(DescribeAutoMlJobV2Response::autoMLJobArn))
.setter(setter(Builder::autoMLJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobArn").build()).build();
private static final SdkField> AUTO_ML_JOB_INPUT_DATA_CONFIG_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AutoMLJobInputDataConfig")
.getter(getter(DescribeAutoMlJobV2Response::autoMLJobInputDataConfig))
.setter(setter(Builder::autoMLJobInputDataConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobInputDataConfig").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoMLJobChannel::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OUTPUT_DATA_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OutputDataConfig")
.getter(getter(DescribeAutoMlJobV2Response::outputDataConfig)).setter(setter(Builder::outputDataConfig))
.constructor(AutoMLOutputDataConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputDataConfig").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(DescribeAutoMlJobV2Response::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField AUTO_ML_JOB_OBJECTIVE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLJobObjective")
.getter(getter(DescribeAutoMlJobV2Response::autoMLJobObjective)).setter(setter(Builder::autoMLJobObjective))
.constructor(AutoMLJobObjective::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobObjective").build())
.build();
private static final SdkField AUTO_ML_PROBLEM_TYPE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLProblemTypeConfig")
.getter(getter(DescribeAutoMlJobV2Response::autoMLProblemTypeConfig))
.setter(setter(Builder::autoMLProblemTypeConfig)).constructor(AutoMLProblemTypeConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLProblemTypeConfig").build())
.build();
private static final SdkField AUTO_ML_PROBLEM_TYPE_CONFIG_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AutoMLProblemTypeConfigName")
.getter(getter(DescribeAutoMlJobV2Response::autoMLProblemTypeConfigNameAsString))
.setter(setter(Builder::autoMLProblemTypeConfigName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLProblemTypeConfigName")
.build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(DescribeAutoMlJobV2Response::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(DescribeAutoMlJobV2Response::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(DescribeAutoMlJobV2Response::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(DescribeAutoMlJobV2Response::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField> PARTIAL_FAILURE_REASONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PartialFailureReasons")
.getter(getter(DescribeAutoMlJobV2Response::partialFailureReasons))
.setter(setter(Builder::partialFailureReasons))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PartialFailureReasons").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoMLPartialFailureReason::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField BEST_CANDIDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("BestCandidate")
.getter(getter(DescribeAutoMlJobV2Response::bestCandidate)).setter(setter(Builder::bestCandidate))
.constructor(AutoMLCandidate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BestCandidate").build()).build();
private static final SdkField AUTO_ML_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobStatus").getter(getter(DescribeAutoMlJobV2Response::autoMLJobStatusAsString))
.setter(setter(Builder::autoMLJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobStatus").build()).build();
private static final SdkField AUTO_ML_JOB_SECONDARY_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobSecondaryStatus").getter(getter(DescribeAutoMlJobV2Response::autoMLJobSecondaryStatusAsString))
.setter(setter(Builder::autoMLJobSecondaryStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobSecondaryStatus").build())
.build();
private static final SdkField AUTO_ML_JOB_ARTIFACTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLJobArtifacts")
.getter(getter(DescribeAutoMlJobV2Response::autoMLJobArtifacts)).setter(setter(Builder::autoMLJobArtifacts))
.constructor(AutoMLJobArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobArtifacts").build())
.build();
private static final SdkField RESOLVED_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ResolvedAttributes")
.getter(getter(DescribeAutoMlJobV2Response::resolvedAttributes)).setter(setter(Builder::resolvedAttributes))
.constructor(AutoMLResolvedAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResolvedAttributes").build())
.build();
private static final SdkField MODEL_DEPLOY_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModelDeployConfig")
.getter(getter(DescribeAutoMlJobV2Response::modelDeployConfig)).setter(setter(Builder::modelDeployConfig))
.constructor(ModelDeployConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelDeployConfig").build()).build();
private static final SdkField MODEL_DEPLOY_RESULT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModelDeployResult")
.getter(getter(DescribeAutoMlJobV2Response::modelDeployResult)).setter(setter(Builder::modelDeployResult))
.constructor(ModelDeployResult::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelDeployResult").build()).build();
private static final SdkField DATA_SPLIT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DataSplitConfig")
.getter(getter(DescribeAutoMlJobV2Response::dataSplitConfig)).setter(setter(Builder::dataSplitConfig))
.constructor(AutoMLDataSplitConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSplitConfig").build()).build();
private static final SdkField SECURITY_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SecurityConfig")
.getter(getter(DescribeAutoMlJobV2Response::securityConfig)).setter(setter(Builder::securityConfig))
.constructor(AutoMLSecurityConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityConfig").build()).build();
private static final SdkField AUTO_ML_COMPUTE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLComputeConfig")
.getter(getter(DescribeAutoMlJobV2Response::autoMLComputeConfig)).setter(setter(Builder::autoMLComputeConfig))
.constructor(AutoMLComputeConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLComputeConfig").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTO_ML_JOB_NAME_FIELD,
AUTO_ML_JOB_ARN_FIELD, AUTO_ML_JOB_INPUT_DATA_CONFIG_FIELD, OUTPUT_DATA_CONFIG_FIELD, ROLE_ARN_FIELD,
AUTO_ML_JOB_OBJECTIVE_FIELD, AUTO_ML_PROBLEM_TYPE_CONFIG_FIELD, AUTO_ML_PROBLEM_TYPE_CONFIG_NAME_FIELD,
CREATION_TIME_FIELD, END_TIME_FIELD, LAST_MODIFIED_TIME_FIELD, FAILURE_REASON_FIELD, PARTIAL_FAILURE_REASONS_FIELD,
BEST_CANDIDATE_FIELD, AUTO_ML_JOB_STATUS_FIELD, AUTO_ML_JOB_SECONDARY_STATUS_FIELD, AUTO_ML_JOB_ARTIFACTS_FIELD,
RESOLVED_ATTRIBUTES_FIELD, MODEL_DEPLOY_CONFIG_FIELD, MODEL_DEPLOY_RESULT_FIELD, DATA_SPLIT_CONFIG_FIELD,
SECURITY_CONFIG_FIELD, AUTO_ML_COMPUTE_CONFIG_FIELD));
private final String autoMLJobName;
private final String autoMLJobArn;
private final List autoMLJobInputDataConfig;
private final AutoMLOutputDataConfig outputDataConfig;
private final String roleArn;
private final AutoMLJobObjective autoMLJobObjective;
private final AutoMLProblemTypeConfig autoMLProblemTypeConfig;
private final String autoMLProblemTypeConfigName;
private final Instant creationTime;
private final Instant endTime;
private final Instant lastModifiedTime;
private final String failureReason;
private final List partialFailureReasons;
private final AutoMLCandidate bestCandidate;
private final String autoMLJobStatus;
private final String autoMLJobSecondaryStatus;
private final AutoMLJobArtifacts autoMLJobArtifacts;
private final AutoMLResolvedAttributes resolvedAttributes;
private final ModelDeployConfig modelDeployConfig;
private final ModelDeployResult modelDeployResult;
private final AutoMLDataSplitConfig dataSplitConfig;
private final AutoMLSecurityConfig securityConfig;
private final AutoMLComputeConfig autoMLComputeConfig;
private DescribeAutoMlJobV2Response(BuilderImpl builder) {
super(builder);
this.autoMLJobName = builder.autoMLJobName;
this.autoMLJobArn = builder.autoMLJobArn;
this.autoMLJobInputDataConfig = builder.autoMLJobInputDataConfig;
this.outputDataConfig = builder.outputDataConfig;
this.roleArn = builder.roleArn;
this.autoMLJobObjective = builder.autoMLJobObjective;
this.autoMLProblemTypeConfig = builder.autoMLProblemTypeConfig;
this.autoMLProblemTypeConfigName = builder.autoMLProblemTypeConfigName;
this.creationTime = builder.creationTime;
this.endTime = builder.endTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.failureReason = builder.failureReason;
this.partialFailureReasons = builder.partialFailureReasons;
this.bestCandidate = builder.bestCandidate;
this.autoMLJobStatus = builder.autoMLJobStatus;
this.autoMLJobSecondaryStatus = builder.autoMLJobSecondaryStatus;
this.autoMLJobArtifacts = builder.autoMLJobArtifacts;
this.resolvedAttributes = builder.resolvedAttributes;
this.modelDeployConfig = builder.modelDeployConfig;
this.modelDeployResult = builder.modelDeployResult;
this.dataSplitConfig = builder.dataSplitConfig;
this.securityConfig = builder.securityConfig;
this.autoMLComputeConfig = builder.autoMLComputeConfig;
}
/**
*
* Returns the name of the AutoML job V2.
*
*
* @return Returns the name of the AutoML job V2.
*/
public final String autoMLJobName() {
return autoMLJobName;
}
/**
*
* Returns the Amazon Resource Name (ARN) of the AutoML job V2.
*
*
* @return Returns the Amazon Resource Name (ARN) of the AutoML job V2.
*/
public final String autoMLJobArn() {
return autoMLJobArn;
}
/**
* For responses, this returns true if the service returned a value for the AutoMLJobInputDataConfig property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAutoMLJobInputDataConfig() {
return autoMLJobInputDataConfig != null && !(autoMLJobInputDataConfig instanceof SdkAutoConstructList);
}
/**
*
* Returns an array of channel objects describing the input data and their location.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAutoMLJobInputDataConfig} method.
*
*
* @return Returns an array of channel objects describing the input data and their location.
*/
public final List autoMLJobInputDataConfig() {
return autoMLJobInputDataConfig;
}
/**
*
* Returns the job's output data config.
*
*
* @return Returns the job's output data config.
*/
public final AutoMLOutputDataConfig outputDataConfig() {
return outputDataConfig;
}
/**
*
* The ARN of the IAM role that has read permission to the input data location and write permission to the output
* data location in Amazon S3.
*
*
* @return The ARN of the IAM role that has read permission to the input data location and write permission to the
* output data location in Amazon S3.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* Returns the job's objective.
*
*
* @return Returns the job's objective.
*/
public final AutoMLJobObjective autoMLJobObjective() {
return autoMLJobObjective;
}
/**
*
* Returns the configuration settings of the problem type set for the AutoML job V2.
*
*
* @return Returns the configuration settings of the problem type set for the AutoML job V2.
*/
public final AutoMLProblemTypeConfig autoMLProblemTypeConfig() {
return autoMLProblemTypeConfig;
}
/**
*
* Returns the name of the problem type configuration set for the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLProblemTypeConfigName} will return {@link AutoMLProblemTypeConfigName#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #autoMLProblemTypeConfigNameAsString}.
*
*
* @return Returns the name of the problem type configuration set for the AutoML job V2.
* @see AutoMLProblemTypeConfigName
*/
public final AutoMLProblemTypeConfigName autoMLProblemTypeConfigName() {
return AutoMLProblemTypeConfigName.fromValue(autoMLProblemTypeConfigName);
}
/**
*
* Returns the name of the problem type configuration set for the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLProblemTypeConfigName} will return {@link AutoMLProblemTypeConfigName#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #autoMLProblemTypeConfigNameAsString}.
*
*
* @return Returns the name of the problem type configuration set for the AutoML job V2.
* @see AutoMLProblemTypeConfigName
*/
public final String autoMLProblemTypeConfigNameAsString() {
return autoMLProblemTypeConfigName;
}
/**
*
* Returns the creation time of the AutoML job V2.
*
*
* @return Returns the creation time of the AutoML job V2.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* Returns the end time of the AutoML job V2.
*
*
* @return Returns the end time of the AutoML job V2.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* Returns the job's last modified time.
*
*
* @return Returns the job's last modified time.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* Returns the reason for the failure of the AutoML job V2, when applicable.
*
*
* @return Returns the reason for the failure of the AutoML job V2, when applicable.
*/
public final String failureReason() {
return failureReason;
}
/**
* For responses, this returns true if the service returned a value for the PartialFailureReasons property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasPartialFailureReasons() {
return partialFailureReasons != null && !(partialFailureReasons instanceof SdkAutoConstructList);
}
/**
*
* Returns a list of reasons for partial failures within an AutoML job V2.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPartialFailureReasons} method.
*
*
* @return Returns a list of reasons for partial failures within an AutoML job V2.
*/
public final List partialFailureReasons() {
return partialFailureReasons;
}
/**
*
* Information about the candidate produced by an AutoML training job V2, including its status, steps, and other
* properties.
*
*
* @return Information about the candidate produced by an AutoML training job V2, including its status, steps, and
* other properties.
*/
public final AutoMLCandidate bestCandidate() {
return bestCandidate;
}
/**
*
* Returns the status of the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoMLJobStatus}
* will return {@link AutoMLJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoMLJobStatusAsString}.
*
*
* @return Returns the status of the AutoML job V2.
* @see AutoMLJobStatus
*/
public final AutoMLJobStatus autoMLJobStatus() {
return AutoMLJobStatus.fromValue(autoMLJobStatus);
}
/**
*
* Returns the status of the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoMLJobStatus}
* will return {@link AutoMLJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoMLJobStatusAsString}.
*
*
* @return Returns the status of the AutoML job V2.
* @see AutoMLJobStatus
*/
public final String autoMLJobStatusAsString() {
return autoMLJobStatus;
}
/**
*
* Returns the secondary status of the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLJobSecondaryStatus} will return {@link AutoMLJobSecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #autoMLJobSecondaryStatusAsString}.
*
*
* @return Returns the secondary status of the AutoML job V2.
* @see AutoMLJobSecondaryStatus
*/
public final AutoMLJobSecondaryStatus autoMLJobSecondaryStatus() {
return AutoMLJobSecondaryStatus.fromValue(autoMLJobSecondaryStatus);
}
/**
*
* Returns the secondary status of the AutoML job V2.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoMLJobSecondaryStatus} will return {@link AutoMLJobSecondaryStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #autoMLJobSecondaryStatusAsString}.
*
*
* @return Returns the secondary status of the AutoML job V2.
* @see AutoMLJobSecondaryStatus
*/
public final String autoMLJobSecondaryStatusAsString() {
return autoMLJobSecondaryStatus;
}
/**
* Returns the value of the AutoMLJobArtifacts property for this object.
*
* @return The value of the AutoMLJobArtifacts property for this object.
*/
public final AutoMLJobArtifacts autoMLJobArtifacts() {
return autoMLJobArtifacts;
}
/**
*
* Returns the resolved attributes used by the AutoML job V2.
*
*
* @return Returns the resolved attributes used by the AutoML job V2.
*/
public final AutoMLResolvedAttributes resolvedAttributes() {
return resolvedAttributes;
}
/**
*
* Indicates whether the model was deployed automatically to an endpoint and the name of that endpoint if deployed
* automatically.
*
*
* @return Indicates whether the model was deployed automatically to an endpoint and the name of that endpoint if
* deployed automatically.
*/
public final ModelDeployConfig modelDeployConfig() {
return modelDeployConfig;
}
/**
*
* Provides information about endpoint for the model deployment.
*
*
* @return Provides information about endpoint for the model deployment.
*/
public final ModelDeployResult modelDeployResult() {
return modelDeployResult;
}
/**
*
* Returns the configuration settings of how the data are split into train and validation datasets.
*
*
* @return Returns the configuration settings of how the data are split into train and validation datasets.
*/
public final AutoMLDataSplitConfig dataSplitConfig() {
return dataSplitConfig;
}
/**
*
* Returns the security configuration for traffic encryption or Amazon VPC settings.
*
*
* @return Returns the security configuration for traffic encryption or Amazon VPC settings.
*/
public final AutoMLSecurityConfig securityConfig() {
return securityConfig;
}
/**
*
* The compute configuration used for the AutoML job V2.
*
*
* @return The compute configuration used for the AutoML job V2.
*/
public final AutoMLComputeConfig autoMLComputeConfig() {
return autoMLComputeConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobName());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobArn());
hashCode = 31 * hashCode + Objects.hashCode(hasAutoMLJobInputDataConfig() ? autoMLJobInputDataConfig() : null);
hashCode = 31 * hashCode + Objects.hashCode(outputDataConfig());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobObjective());
hashCode = 31 * hashCode + Objects.hashCode(autoMLProblemTypeConfig());
hashCode = 31 * hashCode + Objects.hashCode(autoMLProblemTypeConfigNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(hasPartialFailureReasons() ? partialFailureReasons() : null);
hashCode = 31 * hashCode + Objects.hashCode(bestCandidate());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobSecondaryStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobArtifacts());
hashCode = 31 * hashCode + Objects.hashCode(resolvedAttributes());
hashCode = 31 * hashCode + Objects.hashCode(modelDeployConfig());
hashCode = 31 * hashCode + Objects.hashCode(modelDeployResult());
hashCode = 31 * hashCode + Objects.hashCode(dataSplitConfig());
hashCode = 31 * hashCode + Objects.hashCode(securityConfig());
hashCode = 31 * hashCode + Objects.hashCode(autoMLComputeConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeAutoMlJobV2Response)) {
return false;
}
DescribeAutoMlJobV2Response other = (DescribeAutoMlJobV2Response) obj;
return Objects.equals(autoMLJobName(), other.autoMLJobName()) && Objects.equals(autoMLJobArn(), other.autoMLJobArn())
&& hasAutoMLJobInputDataConfig() == other.hasAutoMLJobInputDataConfig()
&& Objects.equals(autoMLJobInputDataConfig(), other.autoMLJobInputDataConfig())
&& Objects.equals(outputDataConfig(), other.outputDataConfig()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(autoMLJobObjective(), other.autoMLJobObjective())
&& Objects.equals(autoMLProblemTypeConfig(), other.autoMLProblemTypeConfig())
&& Objects.equals(autoMLProblemTypeConfigNameAsString(), other.autoMLProblemTypeConfigNameAsString())
&& Objects.equals(creationTime(), other.creationTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(failureReason(), other.failureReason())
&& hasPartialFailureReasons() == other.hasPartialFailureReasons()
&& Objects.equals(partialFailureReasons(), other.partialFailureReasons())
&& Objects.equals(bestCandidate(), other.bestCandidate())
&& Objects.equals(autoMLJobStatusAsString(), other.autoMLJobStatusAsString())
&& Objects.equals(autoMLJobSecondaryStatusAsString(), other.autoMLJobSecondaryStatusAsString())
&& Objects.equals(autoMLJobArtifacts(), other.autoMLJobArtifacts())
&& Objects.equals(resolvedAttributes(), other.resolvedAttributes())
&& Objects.equals(modelDeployConfig(), other.modelDeployConfig())
&& Objects.equals(modelDeployResult(), other.modelDeployResult())
&& Objects.equals(dataSplitConfig(), other.dataSplitConfig())
&& Objects.equals(securityConfig(), other.securityConfig())
&& Objects.equals(autoMLComputeConfig(), other.autoMLComputeConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeAutoMlJobV2Response").add("AutoMLJobName", autoMLJobName())
.add("AutoMLJobArn", autoMLJobArn())
.add("AutoMLJobInputDataConfig", hasAutoMLJobInputDataConfig() ? autoMLJobInputDataConfig() : null)
.add("OutputDataConfig", outputDataConfig()).add("RoleArn", roleArn())
.add("AutoMLJobObjective", autoMLJobObjective()).add("AutoMLProblemTypeConfig", autoMLProblemTypeConfig())
.add("AutoMLProblemTypeConfigName", autoMLProblemTypeConfigNameAsString()).add("CreationTime", creationTime())
.add("EndTime", endTime()).add("LastModifiedTime", lastModifiedTime()).add("FailureReason", failureReason())
.add("PartialFailureReasons", hasPartialFailureReasons() ? partialFailureReasons() : null)
.add("BestCandidate", bestCandidate()).add("AutoMLJobStatus", autoMLJobStatusAsString())
.add("AutoMLJobSecondaryStatus", autoMLJobSecondaryStatusAsString())
.add("AutoMLJobArtifacts", autoMLJobArtifacts()).add("ResolvedAttributes", resolvedAttributes())
.add("ModelDeployConfig", modelDeployConfig()).add("ModelDeployResult", modelDeployResult())
.add("DataSplitConfig", dataSplitConfig()).add("SecurityConfig", securityConfig())
.add("AutoMLComputeConfig", autoMLComputeConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AutoMLJobName":
return Optional.ofNullable(clazz.cast(autoMLJobName()));
case "AutoMLJobArn":
return Optional.ofNullable(clazz.cast(autoMLJobArn()));
case "AutoMLJobInputDataConfig":
return Optional.ofNullable(clazz.cast(autoMLJobInputDataConfig()));
case "OutputDataConfig":
return Optional.ofNullable(clazz.cast(outputDataConfig()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "AutoMLJobObjective":
return Optional.ofNullable(clazz.cast(autoMLJobObjective()));
case "AutoMLProblemTypeConfig":
return Optional.ofNullable(clazz.cast(autoMLProblemTypeConfig()));
case "AutoMLProblemTypeConfigName":
return Optional.ofNullable(clazz.cast(autoMLProblemTypeConfigNameAsString()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "PartialFailureReasons":
return Optional.ofNullable(clazz.cast(partialFailureReasons()));
case "BestCandidate":
return Optional.ofNullable(clazz.cast(bestCandidate()));
case "AutoMLJobStatus":
return Optional.ofNullable(clazz.cast(autoMLJobStatusAsString()));
case "AutoMLJobSecondaryStatus":
return Optional.ofNullable(clazz.cast(autoMLJobSecondaryStatusAsString()));
case "AutoMLJobArtifacts":
return Optional.ofNullable(clazz.cast(autoMLJobArtifacts()));
case "ResolvedAttributes":
return Optional.ofNullable(clazz.cast(resolvedAttributes()));
case "ModelDeployConfig":
return Optional.ofNullable(clazz.cast(modelDeployConfig()));
case "ModelDeployResult":
return Optional.ofNullable(clazz.cast(modelDeployResult()));
case "DataSplitConfig":
return Optional.ofNullable(clazz.cast(dataSplitConfig()));
case "SecurityConfig":
return Optional.ofNullable(clazz.cast(securityConfig()));
case "AutoMLComputeConfig":
return Optional.ofNullable(clazz.cast(autoMLComputeConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function