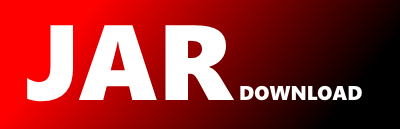
software.amazon.awssdk.services.sagemaker.model.EndpointInput Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Input object for the endpoint
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EndpointInput implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ENDPOINT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointName").getter(getter(EndpointInput::endpointName)).setter(setter(Builder::endpointName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointName").build()).build();
private static final SdkField LOCAL_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LocalPath").getter(getter(EndpointInput::localPath)).setter(setter(Builder::localPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalPath").build()).build();
private static final SdkField S3_INPUT_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3InputMode").getter(getter(EndpointInput::s3InputModeAsString)).setter(setter(Builder::s3InputMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3InputMode").build()).build();
private static final SdkField S3_DATA_DISTRIBUTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3DataDistributionType").getter(getter(EndpointInput::s3DataDistributionTypeAsString))
.setter(setter(Builder::s3DataDistributionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3DataDistributionType").build())
.build();
private static final SdkField FEATURES_ATTRIBUTE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FeaturesAttribute").getter(getter(EndpointInput::featuresAttribute))
.setter(setter(Builder::featuresAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FeaturesAttribute").build()).build();
private static final SdkField INFERENCE_ATTRIBUTE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InferenceAttribute").getter(getter(EndpointInput::inferenceAttribute))
.setter(setter(Builder::inferenceAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceAttribute").build())
.build();
private static final SdkField PROBABILITY_ATTRIBUTE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProbabilityAttribute").getter(getter(EndpointInput::probabilityAttribute))
.setter(setter(Builder::probabilityAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProbabilityAttribute").build())
.build();
private static final SdkField PROBABILITY_THRESHOLD_ATTRIBUTE_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("ProbabilityThresholdAttribute")
.getter(getter(EndpointInput::probabilityThresholdAttribute))
.setter(setter(Builder::probabilityThresholdAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProbabilityThresholdAttribute")
.build()).build();
private static final SdkField START_TIME_OFFSET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StartTimeOffset").getter(getter(EndpointInput::startTimeOffset))
.setter(setter(Builder::startTimeOffset))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTimeOffset").build()).build();
private static final SdkField END_TIME_OFFSET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndTimeOffset").getter(getter(EndpointInput::endTimeOffset)).setter(setter(Builder::endTimeOffset))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTimeOffset").build()).build();
private static final SdkField EXCLUDE_FEATURES_ATTRIBUTE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExcludeFeaturesAttribute").getter(getter(EndpointInput::excludeFeaturesAttribute))
.setter(setter(Builder::excludeFeaturesAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcludeFeaturesAttribute").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENDPOINT_NAME_FIELD,
LOCAL_PATH_FIELD, S3_INPUT_MODE_FIELD, S3_DATA_DISTRIBUTION_TYPE_FIELD, FEATURES_ATTRIBUTE_FIELD,
INFERENCE_ATTRIBUTE_FIELD, PROBABILITY_ATTRIBUTE_FIELD, PROBABILITY_THRESHOLD_ATTRIBUTE_FIELD,
START_TIME_OFFSET_FIELD, END_TIME_OFFSET_FIELD, EXCLUDE_FEATURES_ATTRIBUTE_FIELD));
private static final long serialVersionUID = 1L;
private final String endpointName;
private final String localPath;
private final String s3InputMode;
private final String s3DataDistributionType;
private final String featuresAttribute;
private final String inferenceAttribute;
private final String probabilityAttribute;
private final Double probabilityThresholdAttribute;
private final String startTimeOffset;
private final String endTimeOffset;
private final String excludeFeaturesAttribute;
private EndpointInput(BuilderImpl builder) {
this.endpointName = builder.endpointName;
this.localPath = builder.localPath;
this.s3InputMode = builder.s3InputMode;
this.s3DataDistributionType = builder.s3DataDistributionType;
this.featuresAttribute = builder.featuresAttribute;
this.inferenceAttribute = builder.inferenceAttribute;
this.probabilityAttribute = builder.probabilityAttribute;
this.probabilityThresholdAttribute = builder.probabilityThresholdAttribute;
this.startTimeOffset = builder.startTimeOffset;
this.endTimeOffset = builder.endTimeOffset;
this.excludeFeaturesAttribute = builder.excludeFeaturesAttribute;
}
/**
*
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*
*
* @return An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*/
public final String endpointName() {
return endpointName;
}
/**
*
* Path to the filesystem where the endpoint data is available to the container.
*
*
* @return Path to the filesystem where the endpoint data is available to the container.
*/
public final String localPath() {
return localPath;
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #s3InputMode} will
* return {@link ProcessingS3InputMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #s3InputModeAsString}.
*
*
* @return Whether the Pipe
or File
is used as the input mode for transferring data for
* the monitoring job. Pipe
mode is recommended for large datasets. File
mode is
* useful for small files that fit in memory. Defaults to File
.
* @see ProcessingS3InputMode
*/
public final ProcessingS3InputMode s3InputMode() {
return ProcessingS3InputMode.fromValue(s3InputMode);
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #s3InputMode} will
* return {@link ProcessingS3InputMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #s3InputModeAsString}.
*
*
* @return Whether the Pipe
or File
is used as the input mode for transferring data for
* the monitoring job. Pipe
mode is recommended for large datasets. File
mode is
* useful for small files that fit in memory. Defaults to File
.
* @see ProcessingS3InputMode
*/
public final String s3InputModeAsString() {
return s3InputMode;
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #s3DataDistributionType} will return {@link ProcessingS3DataDistributionType#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #s3DataDistributionTypeAsString}.
*
*
* @return Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @see ProcessingS3DataDistributionType
*/
public final ProcessingS3DataDistributionType s3DataDistributionType() {
return ProcessingS3DataDistributionType.fromValue(s3DataDistributionType);
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #s3DataDistributionType} will return {@link ProcessingS3DataDistributionType#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #s3DataDistributionTypeAsString}.
*
*
* @return Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @see ProcessingS3DataDistributionType
*/
public final String s3DataDistributionTypeAsString() {
return s3DataDistributionType;
}
/**
*
* The attributes of the input data that are the input features.
*
*
* @return The attributes of the input data that are the input features.
*/
public final String featuresAttribute() {
return featuresAttribute;
}
/**
*
* The attribute of the input data that represents the ground truth label.
*
*
* @return The attribute of the input data that represents the ground truth label.
*/
public final String inferenceAttribute() {
return inferenceAttribute;
}
/**
*
* In a classification problem, the attribute that represents the class probability.
*
*
* @return In a classification problem, the attribute that represents the class probability.
*/
public final String probabilityAttribute() {
return probabilityAttribute;
}
/**
*
* The threshold for the class probability to be evaluated as a positive result.
*
*
* @return The threshold for the class probability to be evaluated as a positive result.
*/
public final Double probabilityThresholdAttribute() {
return probabilityThresholdAttribute;
}
/**
*
* If specified, monitoring jobs substract this time from the start time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @return If specified, monitoring jobs substract this time from the start time. For information about using
* offsets for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public final String startTimeOffset() {
return startTimeOffset;
}
/**
*
* If specified, monitoring jobs substract this time from the end time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @return If specified, monitoring jobs substract this time from the end time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public final String endTimeOffset() {
return endTimeOffset;
}
/**
*
* The attributes of the input data to exclude from the analysis.
*
*
* @return The attributes of the input data to exclude from the analysis.
*/
public final String excludeFeaturesAttribute() {
return excludeFeaturesAttribute;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(endpointName());
hashCode = 31 * hashCode + Objects.hashCode(localPath());
hashCode = 31 * hashCode + Objects.hashCode(s3InputModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(s3DataDistributionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(featuresAttribute());
hashCode = 31 * hashCode + Objects.hashCode(inferenceAttribute());
hashCode = 31 * hashCode + Objects.hashCode(probabilityAttribute());
hashCode = 31 * hashCode + Objects.hashCode(probabilityThresholdAttribute());
hashCode = 31 * hashCode + Objects.hashCode(startTimeOffset());
hashCode = 31 * hashCode + Objects.hashCode(endTimeOffset());
hashCode = 31 * hashCode + Objects.hashCode(excludeFeaturesAttribute());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EndpointInput)) {
return false;
}
EndpointInput other = (EndpointInput) obj;
return Objects.equals(endpointName(), other.endpointName()) && Objects.equals(localPath(), other.localPath())
&& Objects.equals(s3InputModeAsString(), other.s3InputModeAsString())
&& Objects.equals(s3DataDistributionTypeAsString(), other.s3DataDistributionTypeAsString())
&& Objects.equals(featuresAttribute(), other.featuresAttribute())
&& Objects.equals(inferenceAttribute(), other.inferenceAttribute())
&& Objects.equals(probabilityAttribute(), other.probabilityAttribute())
&& Objects.equals(probabilityThresholdAttribute(), other.probabilityThresholdAttribute())
&& Objects.equals(startTimeOffset(), other.startTimeOffset())
&& Objects.equals(endTimeOffset(), other.endTimeOffset())
&& Objects.equals(excludeFeaturesAttribute(), other.excludeFeaturesAttribute());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EndpointInput").add("EndpointName", endpointName()).add("LocalPath", localPath())
.add("S3InputMode", s3InputModeAsString()).add("S3DataDistributionType", s3DataDistributionTypeAsString())
.add("FeaturesAttribute", featuresAttribute()).add("InferenceAttribute", inferenceAttribute())
.add("ProbabilityAttribute", probabilityAttribute())
.add("ProbabilityThresholdAttribute", probabilityThresholdAttribute()).add("StartTimeOffset", startTimeOffset())
.add("EndTimeOffset", endTimeOffset()).add("ExcludeFeaturesAttribute", excludeFeaturesAttribute()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndpointName":
return Optional.ofNullable(clazz.cast(endpointName()));
case "LocalPath":
return Optional.ofNullable(clazz.cast(localPath()));
case "S3InputMode":
return Optional.ofNullable(clazz.cast(s3InputModeAsString()));
case "S3DataDistributionType":
return Optional.ofNullable(clazz.cast(s3DataDistributionTypeAsString()));
case "FeaturesAttribute":
return Optional.ofNullable(clazz.cast(featuresAttribute()));
case "InferenceAttribute":
return Optional.ofNullable(clazz.cast(inferenceAttribute()));
case "ProbabilityAttribute":
return Optional.ofNullable(clazz.cast(probabilityAttribute()));
case "ProbabilityThresholdAttribute":
return Optional.ofNullable(clazz.cast(probabilityThresholdAttribute()));
case "StartTimeOffset":
return Optional.ofNullable(clazz.cast(startTimeOffset()));
case "EndTimeOffset":
return Optional.ofNullable(clazz.cast(endTimeOffset()));
case "ExcludeFeaturesAttribute":
return Optional.ofNullable(clazz.cast(excludeFeaturesAttribute()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function