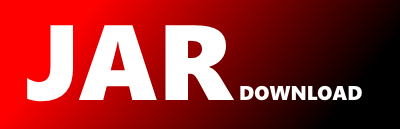
software.amazon.awssdk.services.sagemaker.model.JupyterLabAppSettings Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The settings for the JupyterLab application.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JupyterLabAppSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DEFAULT_RESOURCE_SPEC_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DefaultResourceSpec")
.getter(getter(JupyterLabAppSettings::defaultResourceSpec)).setter(setter(Builder::defaultResourceSpec))
.constructor(ResourceSpec::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultResourceSpec").build())
.build();
private static final SdkField> CUSTOM_IMAGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CustomImages")
.getter(getter(JupyterLabAppSettings::customImages))
.setter(setter(Builder::customImages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomImages").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CustomImage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LIFECYCLE_CONFIG_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LifecycleConfigArns")
.getter(getter(JupyterLabAppSettings::lifecycleConfigArns))
.setter(setter(Builder::lifecycleConfigArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LifecycleConfigArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CODE_REPOSITORIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CodeRepositories")
.getter(getter(JupyterLabAppSettings::codeRepositories))
.setter(setter(Builder::codeRepositories))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeRepositories").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CodeRepository::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField APP_LIFECYCLE_MANAGEMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AppLifecycleManagement")
.getter(getter(JupyterLabAppSettings::appLifecycleManagement)).setter(setter(Builder::appLifecycleManagement))
.constructor(AppLifecycleManagement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppLifecycleManagement").build())
.build();
private static final SdkField EMR_SETTINGS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("EmrSettings").getter(getter(JupyterLabAppSettings::emrSettings)).setter(setter(Builder::emrSettings))
.constructor(EmrSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmrSettings").build()).build();
private static final SdkField BUILT_IN_LIFECYCLE_CONFIG_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BuiltInLifecycleConfigArn").getter(getter(JupyterLabAppSettings::builtInLifecycleConfigArn))
.setter(setter(Builder::builtInLifecycleConfigArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BuiltInLifecycleConfigArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DEFAULT_RESOURCE_SPEC_FIELD,
CUSTOM_IMAGES_FIELD, LIFECYCLE_CONFIG_ARNS_FIELD, CODE_REPOSITORIES_FIELD, APP_LIFECYCLE_MANAGEMENT_FIELD,
EMR_SETTINGS_FIELD, BUILT_IN_LIFECYCLE_CONFIG_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final ResourceSpec defaultResourceSpec;
private final List customImages;
private final List lifecycleConfigArns;
private final List codeRepositories;
private final AppLifecycleManagement appLifecycleManagement;
private final EmrSettings emrSettings;
private final String builtInLifecycleConfigArn;
private JupyterLabAppSettings(BuilderImpl builder) {
this.defaultResourceSpec = builder.defaultResourceSpec;
this.customImages = builder.customImages;
this.lifecycleConfigArns = builder.lifecycleConfigArns;
this.codeRepositories = builder.codeRepositories;
this.appLifecycleManagement = builder.appLifecycleManagement;
this.emrSettings = builder.emrSettings;
this.builtInLifecycleConfigArn = builder.builtInLifecycleConfigArn;
}
/**
* Returns the value of the DefaultResourceSpec property for this object.
*
* @return The value of the DefaultResourceSpec property for this object.
*/
public final ResourceSpec defaultResourceSpec() {
return defaultResourceSpec;
}
/**
* For responses, this returns true if the service returned a value for the CustomImages property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCustomImages() {
return customImages != null && !(customImages instanceof SdkAutoConstructList);
}
/**
*
* A list of custom SageMaker images that are configured to run as a JupyterLab app.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCustomImages} method.
*
*
* @return A list of custom SageMaker images that are configured to run as a JupyterLab app.
*/
public final List customImages() {
return customImages;
}
/**
* For responses, this returns true if the service returned a value for the LifecycleConfigArns property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLifecycleConfigArns() {
return lifecycleConfigArns != null && !(lifecycleConfigArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Name (ARN) of the lifecycle configurations attached to the user profile or domain. To remove
* a lifecycle config, you must set LifecycleConfigArns
to an empty list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLifecycleConfigArns} method.
*
*
* @return The Amazon Resource Name (ARN) of the lifecycle configurations attached to the user profile or domain. To
* remove a lifecycle config, you must set LifecycleConfigArns
to an empty list.
*/
public final List lifecycleConfigArns() {
return lifecycleConfigArns;
}
/**
* For responses, this returns true if the service returned a value for the CodeRepositories property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCodeRepositories() {
return codeRepositories != null && !(codeRepositories instanceof SdkAutoConstructList);
}
/**
*
* A list of Git repositories that SageMaker automatically displays to users for cloning in the JupyterLab
* application.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCodeRepositories} method.
*
*
* @return A list of Git repositories that SageMaker automatically displays to users for cloning in the JupyterLab
* application.
*/
public final List codeRepositories() {
return codeRepositories;
}
/**
*
* Indicates whether idle shutdown is activated for JupyterLab applications.
*
*
* @return Indicates whether idle shutdown is activated for JupyterLab applications.
*/
public final AppLifecycleManagement appLifecycleManagement() {
return appLifecycleManagement;
}
/**
*
* The configuration parameters that specify the IAM roles assumed by the execution role of SageMaker (assumable
* roles) and the cluster instances or job execution environments (execution roles or runtime roles) to manage and
* access resources required for running Amazon EMR clusters or Amazon EMR Serverless applications.
*
*
* @return The configuration parameters that specify the IAM roles assumed by the execution role of SageMaker
* (assumable roles) and the cluster instances or job execution environments (execution roles or runtime
* roles) to manage and access resources required for running Amazon EMR clusters or Amazon EMR Serverless
* applications.
*/
public final EmrSettings emrSettings() {
return emrSettings;
}
/**
*
* The lifecycle configuration that runs before the default lifecycle configuration. It can override changes made in
* the default lifecycle configuration.
*
*
* @return The lifecycle configuration that runs before the default lifecycle configuration. It can override changes
* made in the default lifecycle configuration.
*/
public final String builtInLifecycleConfigArn() {
return builtInLifecycleConfigArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(defaultResourceSpec());
hashCode = 31 * hashCode + Objects.hashCode(hasCustomImages() ? customImages() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLifecycleConfigArns() ? lifecycleConfigArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCodeRepositories() ? codeRepositories() : null);
hashCode = 31 * hashCode + Objects.hashCode(appLifecycleManagement());
hashCode = 31 * hashCode + Objects.hashCode(emrSettings());
hashCode = 31 * hashCode + Objects.hashCode(builtInLifecycleConfigArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JupyterLabAppSettings)) {
return false;
}
JupyterLabAppSettings other = (JupyterLabAppSettings) obj;
return Objects.equals(defaultResourceSpec(), other.defaultResourceSpec()) && hasCustomImages() == other.hasCustomImages()
&& Objects.equals(customImages(), other.customImages())
&& hasLifecycleConfigArns() == other.hasLifecycleConfigArns()
&& Objects.equals(lifecycleConfigArns(), other.lifecycleConfigArns())
&& hasCodeRepositories() == other.hasCodeRepositories()
&& Objects.equals(codeRepositories(), other.codeRepositories())
&& Objects.equals(appLifecycleManagement(), other.appLifecycleManagement())
&& Objects.equals(emrSettings(), other.emrSettings())
&& Objects.equals(builtInLifecycleConfigArn(), other.builtInLifecycleConfigArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JupyterLabAppSettings").add("DefaultResourceSpec", defaultResourceSpec())
.add("CustomImages", hasCustomImages() ? customImages() : null)
.add("LifecycleConfigArns", hasLifecycleConfigArns() ? lifecycleConfigArns() : null)
.add("CodeRepositories", hasCodeRepositories() ? codeRepositories() : null)
.add("AppLifecycleManagement", appLifecycleManagement()).add("EmrSettings", emrSettings())
.add("BuiltInLifecycleConfigArn", builtInLifecycleConfigArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DefaultResourceSpec":
return Optional.ofNullable(clazz.cast(defaultResourceSpec()));
case "CustomImages":
return Optional.ofNullable(clazz.cast(customImages()));
case "LifecycleConfigArns":
return Optional.ofNullable(clazz.cast(lifecycleConfigArns()));
case "CodeRepositories":
return Optional.ofNullable(clazz.cast(codeRepositories()));
case "AppLifecycleManagement":
return Optional.ofNullable(clazz.cast(appLifecycleManagement()));
case "EmrSettings":
return Optional.ofNullable(clazz.cast(emrSettings()));
case "BuiltInLifecycleConfigArn":
return Optional.ofNullable(clazz.cast(builtInLifecycleConfigArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function