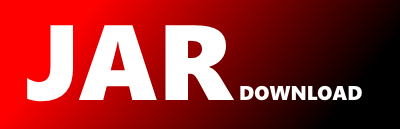
software.amazon.awssdk.services.sagemaker.model.ProductionVariant Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Identifies a model that you want to host and the resources chosen to deploy for hosting it. If you are deploying
* multiple models, tell SageMaker how to distribute traffic among the models by specifying variant weights. For more
* information on production variants, check Production variants.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProductionVariant implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField VARIANT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VariantName").getter(getter(ProductionVariant::variantName)).setter(setter(Builder::variantName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VariantName").build()).build();
private static final SdkField MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelName").getter(getter(ProductionVariant::modelName)).setter(setter(Builder::modelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelName").build()).build();
private static final SdkField INITIAL_INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("InitialInstanceCount").getter(getter(ProductionVariant::initialInstanceCount))
.setter(setter(Builder::initialInstanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitialInstanceCount").build())
.build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(ProductionVariant::instanceTypeAsString))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField INITIAL_VARIANT_WEIGHT_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("InitialVariantWeight").getter(getter(ProductionVariant::initialVariantWeight))
.setter(setter(Builder::initialVariantWeight))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitialVariantWeight").build())
.build();
private static final SdkField ACCELERATOR_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AcceleratorType").getter(getter(ProductionVariant::acceleratorTypeAsString))
.setter(setter(Builder::acceleratorType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AcceleratorType").build()).build();
private static final SdkField CORE_DUMP_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CoreDumpConfig")
.getter(getter(ProductionVariant::coreDumpConfig)).setter(setter(Builder::coreDumpConfig))
.constructor(ProductionVariantCoreDumpConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoreDumpConfig").build()).build();
private static final SdkField SERVERLESS_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ServerlessConfig")
.getter(getter(ProductionVariant::serverlessConfig)).setter(setter(Builder::serverlessConfig))
.constructor(ProductionVariantServerlessConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerlessConfig").build()).build();
private static final SdkField VOLUME_SIZE_IN_GB_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("VolumeSizeInGB").getter(getter(ProductionVariant::volumeSizeInGB))
.setter(setter(Builder::volumeSizeInGB))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeSizeInGB").build()).build();
private static final SdkField MODEL_DATA_DOWNLOAD_TIMEOUT_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ModelDataDownloadTimeoutInSeconds")
.getter(getter(ProductionVariant::modelDataDownloadTimeoutInSeconds))
.setter(setter(Builder::modelDataDownloadTimeoutInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelDataDownloadTimeoutInSeconds")
.build()).build();
private static final SdkField CONTAINER_STARTUP_HEALTH_CHECK_TIMEOUT_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ContainerStartupHealthCheckTimeoutInSeconds")
.getter(getter(ProductionVariant::containerStartupHealthCheckTimeoutInSeconds))
.setter(setter(Builder::containerStartupHealthCheckTimeoutInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ContainerStartupHealthCheckTimeoutInSeconds").build()).build();
private static final SdkField ENABLE_SSM_ACCESS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnableSSMAccess").getter(getter(ProductionVariant::enableSSMAccess))
.setter(setter(Builder::enableSSMAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableSSMAccess").build()).build();
private static final SdkField MANAGED_INSTANCE_SCALING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ManagedInstanceScaling")
.getter(getter(ProductionVariant::managedInstanceScaling)).setter(setter(Builder::managedInstanceScaling))
.constructor(ProductionVariantManagedInstanceScaling::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManagedInstanceScaling").build())
.build();
private static final SdkField ROUTING_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RoutingConfig")
.getter(getter(ProductionVariant::routingConfig)).setter(setter(Builder::routingConfig))
.constructor(ProductionVariantRoutingConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoutingConfig").build()).build();
private static final SdkField INFERENCE_AMI_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InferenceAmiVersion").getter(getter(ProductionVariant::inferenceAmiVersionAsString))
.setter(setter(Builder::inferenceAmiVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InferenceAmiVersion").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VARIANT_NAME_FIELD,
MODEL_NAME_FIELD, INITIAL_INSTANCE_COUNT_FIELD, INSTANCE_TYPE_FIELD, INITIAL_VARIANT_WEIGHT_FIELD,
ACCELERATOR_TYPE_FIELD, CORE_DUMP_CONFIG_FIELD, SERVERLESS_CONFIG_FIELD, VOLUME_SIZE_IN_GB_FIELD,
MODEL_DATA_DOWNLOAD_TIMEOUT_IN_SECONDS_FIELD, CONTAINER_STARTUP_HEALTH_CHECK_TIMEOUT_IN_SECONDS_FIELD,
ENABLE_SSM_ACCESS_FIELD, MANAGED_INSTANCE_SCALING_FIELD, ROUTING_CONFIG_FIELD, INFERENCE_AMI_VERSION_FIELD));
private static final long serialVersionUID = 1L;
private final String variantName;
private final String modelName;
private final Integer initialInstanceCount;
private final String instanceType;
private final Float initialVariantWeight;
private final String acceleratorType;
private final ProductionVariantCoreDumpConfig coreDumpConfig;
private final ProductionVariantServerlessConfig serverlessConfig;
private final Integer volumeSizeInGB;
private final Integer modelDataDownloadTimeoutInSeconds;
private final Integer containerStartupHealthCheckTimeoutInSeconds;
private final Boolean enableSSMAccess;
private final ProductionVariantManagedInstanceScaling managedInstanceScaling;
private final ProductionVariantRoutingConfig routingConfig;
private final String inferenceAmiVersion;
private ProductionVariant(BuilderImpl builder) {
this.variantName = builder.variantName;
this.modelName = builder.modelName;
this.initialInstanceCount = builder.initialInstanceCount;
this.instanceType = builder.instanceType;
this.initialVariantWeight = builder.initialVariantWeight;
this.acceleratorType = builder.acceleratorType;
this.coreDumpConfig = builder.coreDumpConfig;
this.serverlessConfig = builder.serverlessConfig;
this.volumeSizeInGB = builder.volumeSizeInGB;
this.modelDataDownloadTimeoutInSeconds = builder.modelDataDownloadTimeoutInSeconds;
this.containerStartupHealthCheckTimeoutInSeconds = builder.containerStartupHealthCheckTimeoutInSeconds;
this.enableSSMAccess = builder.enableSSMAccess;
this.managedInstanceScaling = builder.managedInstanceScaling;
this.routingConfig = builder.routingConfig;
this.inferenceAmiVersion = builder.inferenceAmiVersion;
}
/**
*
* The name of the production variant.
*
*
* @return The name of the production variant.
*/
public final String variantName() {
return variantName;
}
/**
*
* The name of the model that you want to host. This is the name that you specified when creating the model.
*
*
* @return The name of the model that you want to host. This is the name that you specified when creating the model.
*/
public final String modelName() {
return modelName;
}
/**
*
* Number of instances to launch initially.
*
*
* @return Number of instances to launch initially.
*/
public final Integer initialInstanceCount() {
return initialInstanceCount;
}
/**
*
* The ML compute instance type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link ProductionVariantInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #instanceTypeAsString}.
*
*
* @return The ML compute instance type.
* @see ProductionVariantInstanceType
*/
public final ProductionVariantInstanceType instanceType() {
return ProductionVariantInstanceType.fromValue(instanceType);
}
/**
*
* The ML compute instance type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link ProductionVariantInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #instanceTypeAsString}.
*
*
* @return The ML compute instance type.
* @see ProductionVariantInstanceType
*/
public final String instanceTypeAsString() {
return instanceType;
}
/**
*
* Determines initial traffic distribution among all of the models that you specify in the endpoint configuration.
* The traffic to a production variant is determined by the ratio of the VariantWeight
to the sum of
* all VariantWeight
values across all ProductionVariants. If unspecified, it defaults to 1.0.
*
*
* @return Determines initial traffic distribution among all of the models that you specify in the endpoint
* configuration. The traffic to a production variant is determined by the ratio of the
* VariantWeight
to the sum of all VariantWeight
values across all
* ProductionVariants. If unspecified, it defaults to 1.0.
*/
public final Float initialVariantWeight() {
return initialVariantWeight;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acceleratorType}
* will return {@link ProductionVariantAcceleratorType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #acceleratorTypeAsString}.
*
*
* @return The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public final ProductionVariantAcceleratorType acceleratorType() {
return ProductionVariantAcceleratorType.fromValue(acceleratorType);
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #acceleratorType}
* will return {@link ProductionVariantAcceleratorType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #acceleratorTypeAsString}.
*
*
* @return The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public final String acceleratorTypeAsString() {
return acceleratorType;
}
/**
*
* Specifies configuration for a core dump from the model container when the process crashes.
*
*
* @return Specifies configuration for a core dump from the model container when the process crashes.
*/
public final ProductionVariantCoreDumpConfig coreDumpConfig() {
return coreDumpConfig;
}
/**
*
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*
*
* @return The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*/
public final ProductionVariantServerlessConfig serverlessConfig() {
return serverlessConfig;
}
/**
*
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*
*
* @return The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*/
public final Integer volumeSizeInGB() {
return volumeSizeInGB;
}
/**
*
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to the
* individual inference instance associated with this production variant.
*
*
* @return The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to
* the individual inference instance associated with this production variant.
*/
public final Integer modelDataDownloadTimeoutInSeconds() {
return modelDataDownloadTimeoutInSeconds;
}
/**
*
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For more
* information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*
*
* @return The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting.
* For more information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*/
public final Integer containerStartupHealthCheckTimeoutInSeconds() {
return containerStartupHealthCheckTimeoutInSeconds;
}
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*
* @return You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a
* production variant behind an endpoint. By default, SSM access is disabled for all production variants
* behind an endpoint. You can turn on or turn off SSM access for a production variant behind an existing
* endpoint by creating a new endpoint configuration and calling UpdateEndpoint
.
*/
public final Boolean enableSSMAccess() {
return enableSSMAccess;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @return Settings that control the range in the number of instances that the endpoint provisions as it scales up
* or down to accommodate traffic.
*/
public final ProductionVariantManagedInstanceScaling managedInstanceScaling() {
return managedInstanceScaling;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @return Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*/
public final ProductionVariantRoutingConfig routingConfig() {
return routingConfig;
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* The AMI version names, and their configurations, are the following:
*
*
* - al2-ami-sagemaker-inference-gpu-2
* -
*
* -
*
* Accelerator: GPU
*
*
* -
*
* NVIDIA driver version: 535.54.03
*
*
* -
*
* CUDA driver version: 12.2
*
*
* -
*
* Supported instance types: ml.g4dn.*, ml.g5.*, ml.g6.*, ml.p3.*, ml.p4d.*, ml.p4de.*, ml.p5.*
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferenceAmiVersion} will return {@link ProductionVariantInferenceAmiVersion#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #inferenceAmiVersionAsString}.
*
*
* @return Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
*
*
* The AMI version names, and their configurations, are the following:
*
*
* - al2-ami-sagemaker-inference-gpu-2
* -
*
* -
*
* Accelerator: GPU
*
*
* -
*
* NVIDIA driver version: 535.54.03
*
*
* -
*
* CUDA driver version: 12.2
*
*
* -
*
* Supported instance types: ml.g4dn.*, ml.g5.*, ml.g6.*, ml.p3.*, ml.p4d.*, ml.p4de.*, ml.p5.*
*
*
*
*
* @see ProductionVariantInferenceAmiVersion
*/
public final ProductionVariantInferenceAmiVersion inferenceAmiVersion() {
return ProductionVariantInferenceAmiVersion.fromValue(inferenceAmiVersion);
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* The AMI version names, and their configurations, are the following:
*
*
* - al2-ami-sagemaker-inference-gpu-2
* -
*
* -
*
* Accelerator: GPU
*
*
* -
*
* NVIDIA driver version: 535.54.03
*
*
* -
*
* CUDA driver version: 12.2
*
*
* -
*
* Supported instance types: ml.g4dn.*, ml.g5.*, ml.g6.*, ml.p3.*, ml.p4d.*, ml.p4de.*, ml.p5.*
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferenceAmiVersion} will return {@link ProductionVariantInferenceAmiVersion#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #inferenceAmiVersionAsString}.
*
*
* @return Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
*
*
* The AMI version names, and their configurations, are the following:
*
*
* - al2-ami-sagemaker-inference-gpu-2
* -
*
* -
*
* Accelerator: GPU
*
*
* -
*
* NVIDIA driver version: 535.54.03
*
*
* -
*
* CUDA driver version: 12.2
*
*
* -
*
* Supported instance types: ml.g4dn.*, ml.g5.*, ml.g6.*, ml.p3.*, ml.p4d.*, ml.p4de.*, ml.p5.*
*
*
*
*
* @see ProductionVariantInferenceAmiVersion
*/
public final String inferenceAmiVersionAsString() {
return inferenceAmiVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(variantName());
hashCode = 31 * hashCode + Objects.hashCode(modelName());
hashCode = 31 * hashCode + Objects.hashCode(initialInstanceCount());
hashCode = 31 * hashCode + Objects.hashCode(instanceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(initialVariantWeight());
hashCode = 31 * hashCode + Objects.hashCode(acceleratorTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(coreDumpConfig());
hashCode = 31 * hashCode + Objects.hashCode(serverlessConfig());
hashCode = 31 * hashCode + Objects.hashCode(volumeSizeInGB());
hashCode = 31 * hashCode + Objects.hashCode(modelDataDownloadTimeoutInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(containerStartupHealthCheckTimeoutInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(enableSSMAccess());
hashCode = 31 * hashCode + Objects.hashCode(managedInstanceScaling());
hashCode = 31 * hashCode + Objects.hashCode(routingConfig());
hashCode = 31 * hashCode + Objects.hashCode(inferenceAmiVersionAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProductionVariant)) {
return false;
}
ProductionVariant other = (ProductionVariant) obj;
return Objects.equals(variantName(), other.variantName())
&& Objects.equals(modelName(), other.modelName())
&& Objects.equals(initialInstanceCount(), other.initialInstanceCount())
&& Objects.equals(instanceTypeAsString(), other.instanceTypeAsString())
&& Objects.equals(initialVariantWeight(), other.initialVariantWeight())
&& Objects.equals(acceleratorTypeAsString(), other.acceleratorTypeAsString())
&& Objects.equals(coreDumpConfig(), other.coreDumpConfig())
&& Objects.equals(serverlessConfig(), other.serverlessConfig())
&& Objects.equals(volumeSizeInGB(), other.volumeSizeInGB())
&& Objects.equals(modelDataDownloadTimeoutInSeconds(), other.modelDataDownloadTimeoutInSeconds())
&& Objects.equals(containerStartupHealthCheckTimeoutInSeconds(),
other.containerStartupHealthCheckTimeoutInSeconds())
&& Objects.equals(enableSSMAccess(), other.enableSSMAccess())
&& Objects.equals(managedInstanceScaling(), other.managedInstanceScaling())
&& Objects.equals(routingConfig(), other.routingConfig())
&& Objects.equals(inferenceAmiVersionAsString(), other.inferenceAmiVersionAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProductionVariant").add("VariantName", variantName()).add("ModelName", modelName())
.add("InitialInstanceCount", initialInstanceCount()).add("InstanceType", instanceTypeAsString())
.add("InitialVariantWeight", initialVariantWeight()).add("AcceleratorType", acceleratorTypeAsString())
.add("CoreDumpConfig", coreDumpConfig()).add("ServerlessConfig", serverlessConfig())
.add("VolumeSizeInGB", volumeSizeInGB())
.add("ModelDataDownloadTimeoutInSeconds", modelDataDownloadTimeoutInSeconds())
.add("ContainerStartupHealthCheckTimeoutInSeconds", containerStartupHealthCheckTimeoutInSeconds())
.add("EnableSSMAccess", enableSSMAccess()).add("ManagedInstanceScaling", managedInstanceScaling())
.add("RoutingConfig", routingConfig()).add("InferenceAmiVersion", inferenceAmiVersionAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "VariantName":
return Optional.ofNullable(clazz.cast(variantName()));
case "ModelName":
return Optional.ofNullable(clazz.cast(modelName()));
case "InitialInstanceCount":
return Optional.ofNullable(clazz.cast(initialInstanceCount()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceTypeAsString()));
case "InitialVariantWeight":
return Optional.ofNullable(clazz.cast(initialVariantWeight()));
case "AcceleratorType":
return Optional.ofNullable(clazz.cast(acceleratorTypeAsString()));
case "CoreDumpConfig":
return Optional.ofNullable(clazz.cast(coreDumpConfig()));
case "ServerlessConfig":
return Optional.ofNullable(clazz.cast(serverlessConfig()));
case "VolumeSizeInGB":
return Optional.ofNullable(clazz.cast(volumeSizeInGB()));
case "ModelDataDownloadTimeoutInSeconds":
return Optional.ofNullable(clazz.cast(modelDataDownloadTimeoutInSeconds()));
case "ContainerStartupHealthCheckTimeoutInSeconds":
return Optional.ofNullable(clazz.cast(containerStartupHealthCheckTimeoutInSeconds()));
case "EnableSSMAccess":
return Optional.ofNullable(clazz.cast(enableSSMAccess()));
case "ManagedInstanceScaling":
return Optional.ofNullable(clazz.cast(managedInstanceScaling()));
case "RoutingConfig":
return Optional.ofNullable(clazz.cast(routingConfig()));
case "InferenceAmiVersion":
return Optional.ofNullable(clazz.cast(inferenceAmiVersionAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function