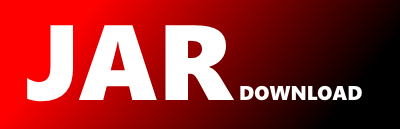
software.amazon.awssdk.services.sagemaker.model.CreateEndpointConfigRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateEndpointConfigRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField ENDPOINT_CONFIG_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointConfigName").getter(getter(CreateEndpointConfigRequest::endpointConfigName))
.setter(setter(Builder::endpointConfigName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointConfigName").build())
.build();
private static final SdkField> PRODUCTION_VARIANTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ProductionVariants")
.getter(getter(CreateEndpointConfigRequest::productionVariants))
.setter(setter(Builder::productionVariants))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductionVariants").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProductionVariant::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATA_CAPTURE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DataCaptureConfig")
.getter(getter(CreateEndpointConfigRequest::dataCaptureConfig)).setter(setter(Builder::dataCaptureConfig))
.constructor(DataCaptureConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataCaptureConfig").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateEndpointConfigRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(CreateEndpointConfigRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField ASYNC_INFERENCE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AsyncInferenceConfig")
.getter(getter(CreateEndpointConfigRequest::asyncInferenceConfig)).setter(setter(Builder::asyncInferenceConfig))
.constructor(AsyncInferenceConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AsyncInferenceConfig").build())
.build();
private static final SdkField EXPLAINER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ExplainerConfig")
.getter(getter(CreateEndpointConfigRequest::explainerConfig)).setter(setter(Builder::explainerConfig))
.constructor(ExplainerConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExplainerConfig").build()).build();
private static final SdkField> SHADOW_PRODUCTION_VARIANTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ShadowProductionVariants")
.getter(getter(CreateEndpointConfigRequest::shadowProductionVariants))
.setter(setter(Builder::shadowProductionVariants))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShadowProductionVariants").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProductionVariant::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EXECUTION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExecutionRoleArn").getter(getter(CreateEndpointConfigRequest::executionRoleArn))
.setter(setter(Builder::executionRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionRoleArn").build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("VpcConfig").getter(getter(CreateEndpointConfigRequest::vpcConfig)).setter(setter(Builder::vpcConfig))
.constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcConfig").build()).build();
private static final SdkField ENABLE_NETWORK_ISOLATION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnableNetworkIsolation").getter(getter(CreateEndpointConfigRequest::enableNetworkIsolation))
.setter(setter(Builder::enableNetworkIsolation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableNetworkIsolation").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENDPOINT_CONFIG_NAME_FIELD,
PRODUCTION_VARIANTS_FIELD, DATA_CAPTURE_CONFIG_FIELD, TAGS_FIELD, KMS_KEY_ID_FIELD, ASYNC_INFERENCE_CONFIG_FIELD,
EXPLAINER_CONFIG_FIELD, SHADOW_PRODUCTION_VARIANTS_FIELD, EXECUTION_ROLE_ARN_FIELD, VPC_CONFIG_FIELD,
ENABLE_NETWORK_ISOLATION_FIELD));
private final String endpointConfigName;
private final List productionVariants;
private final DataCaptureConfig dataCaptureConfig;
private final List tags;
private final String kmsKeyId;
private final AsyncInferenceConfig asyncInferenceConfig;
private final ExplainerConfig explainerConfig;
private final List shadowProductionVariants;
private final String executionRoleArn;
private final VpcConfig vpcConfig;
private final Boolean enableNetworkIsolation;
private CreateEndpointConfigRequest(BuilderImpl builder) {
super(builder);
this.endpointConfigName = builder.endpointConfigName;
this.productionVariants = builder.productionVariants;
this.dataCaptureConfig = builder.dataCaptureConfig;
this.tags = builder.tags;
this.kmsKeyId = builder.kmsKeyId;
this.asyncInferenceConfig = builder.asyncInferenceConfig;
this.explainerConfig = builder.explainerConfig;
this.shadowProductionVariants = builder.shadowProductionVariants;
this.executionRoleArn = builder.executionRoleArn;
this.vpcConfig = builder.vpcConfig;
this.enableNetworkIsolation = builder.enableNetworkIsolation;
}
/**
*
* The name of the endpoint configuration. You specify this name in a CreateEndpoint
* request.
*
*
* @return The name of the endpoint configuration. You specify this name in a CreateEndpoint request.
*/
public final String endpointConfigName() {
return endpointConfigName;
}
/**
* For responses, this returns true if the service returned a value for the ProductionVariants property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasProductionVariants() {
return productionVariants != null && !(productionVariants instanceof SdkAutoConstructList);
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasProductionVariants} method.
*
*
* @return An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint.
*/
public final List productionVariants() {
return productionVariants;
}
/**
* Returns the value of the DataCaptureConfig property for this object.
*
* @return The value of the DataCaptureConfig property for this object.
*/
public final DataCaptureConfig dataCaptureConfig() {
return dataCaptureConfig;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public final List tags() {
return tags;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your CreateEndpoint
,
* UpdateEndpoint
requests. For more information, refer to the Amazon Web Services Key Management
* Service section Using Key
* Policies in Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a KmsKeyId
when using an
* instance type with local storage. If any of the models that you specify in the ProductionVariants
* parameter use nitro-based instances with local storage, do not specify a value for the KmsKeyId
* parameter. If you specify a value for KmsKeyId
when using any nitro-based instances with local
* storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* @return The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateEndpoint
, UpdateEndpoint
requests. For more information, refer to the
* Amazon Web Services Key Management Service section Using Key Policies in
* Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage
* volumes are encrypted using a hardware module on the instance. You can't request a KmsKeyId
* when using an instance type with local storage. If any of the models that you specify in the
* ProductionVariants
parameter use nitro-based instances with local storage, do not specify a
* value for the KmsKeyId
parameter. If you specify a value for KmsKeyId
when
* using any nitro-based instances with local storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in order
* for your Endpoint to be invoked using InvokeEndpointAsync.
*
*
* @return Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in
* order for your Endpoint to be invoked using InvokeEndpointAsync.
*/
public final AsyncInferenceConfig asyncInferenceConfig() {
return asyncInferenceConfig;
}
/**
*
* A member of CreateEndpointConfig
that enables explainers.
*
*
* @return A member of CreateEndpointConfig
that enables explainers.
*/
public final ExplainerConfig explainerConfig() {
return explainerConfig;
}
/**
* For responses, this returns true if the service returned a value for the ShadowProductionVariants property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasShadowProductionVariants() {
return shadowProductionVariants != null && !(shadowProductionVariants instanceof SdkAutoConstructList);
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasShadowProductionVariants} method.
*
*
* @return An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint in shadow mode with production traffic replicated from the model specified on
* ProductionVariants
. If you use this field, you can only specify one variant for
* ProductionVariants
and one variant for ShadowProductionVariants
.
*/
public final List shadowProductionVariants() {
return shadowProductionVariants;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your behalf.
* For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your
* behalf. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*/
public final String executionRoleArn() {
return executionRoleArn;
}
/**
* Returns the value of the VpcConfig property for this object.
*
* @return The value of the VpcConfig property for this object.
*/
public final VpcConfig vpcConfig() {
return vpcConfig;
}
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*
* @return Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or
* outbound network calls can be made to or from the model containers.
*/
public final Boolean enableNetworkIsolation() {
return enableNetworkIsolation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(endpointConfigName());
hashCode = 31 * hashCode + Objects.hashCode(hasProductionVariants() ? productionVariants() : null);
hashCode = 31 * hashCode + Objects.hashCode(dataCaptureConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(asyncInferenceConfig());
hashCode = 31 * hashCode + Objects.hashCode(explainerConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasShadowProductionVariants() ? shadowProductionVariants() : null);
hashCode = 31 * hashCode + Objects.hashCode(executionRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
hashCode = 31 * hashCode + Objects.hashCode(enableNetworkIsolation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateEndpointConfigRequest)) {
return false;
}
CreateEndpointConfigRequest other = (CreateEndpointConfigRequest) obj;
return Objects.equals(endpointConfigName(), other.endpointConfigName())
&& hasProductionVariants() == other.hasProductionVariants()
&& Objects.equals(productionVariants(), other.productionVariants())
&& Objects.equals(dataCaptureConfig(), other.dataCaptureConfig()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(asyncInferenceConfig(), other.asyncInferenceConfig())
&& Objects.equals(explainerConfig(), other.explainerConfig())
&& hasShadowProductionVariants() == other.hasShadowProductionVariants()
&& Objects.equals(shadowProductionVariants(), other.shadowProductionVariants())
&& Objects.equals(executionRoleArn(), other.executionRoleArn()) && Objects.equals(vpcConfig(), other.vpcConfig())
&& Objects.equals(enableNetworkIsolation(), other.enableNetworkIsolation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateEndpointConfigRequest").add("EndpointConfigName", endpointConfigName())
.add("ProductionVariants", hasProductionVariants() ? productionVariants() : null)
.add("DataCaptureConfig", dataCaptureConfig()).add("Tags", hasTags() ? tags() : null).add("KmsKeyId", kmsKeyId())
.add("AsyncInferenceConfig", asyncInferenceConfig()).add("ExplainerConfig", explainerConfig())
.add("ShadowProductionVariants", hasShadowProductionVariants() ? shadowProductionVariants() : null)
.add("ExecutionRoleArn", executionRoleArn()).add("VpcConfig", vpcConfig())
.add("EnableNetworkIsolation", enableNetworkIsolation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndpointConfigName":
return Optional.ofNullable(clazz.cast(endpointConfigName()));
case "ProductionVariants":
return Optional.ofNullable(clazz.cast(productionVariants()));
case "DataCaptureConfig":
return Optional.ofNullable(clazz.cast(dataCaptureConfig()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "AsyncInferenceConfig":
return Optional.ofNullable(clazz.cast(asyncInferenceConfig()));
case "ExplainerConfig":
return Optional.ofNullable(clazz.cast(explainerConfig()));
case "ShadowProductionVariants":
return Optional.ofNullable(clazz.cast(shadowProductionVariants()));
case "ExecutionRoleArn":
return Optional.ofNullable(clazz.cast(executionRoleArn()));
case "VpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
case "EnableNetworkIsolation":
return Optional.ofNullable(clazz.cast(enableNetworkIsolation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function