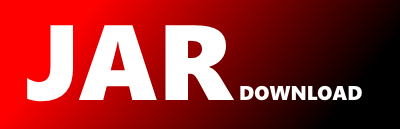
software.amazon.awssdk.services.sagemaker.model.DomainSettingsForUpdate Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A collection of Domain
configuration settings to update.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DomainSettingsForUpdate implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField R_STUDIO_SERVER_PRO_DOMAIN_SETTINGS_FOR_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("RStudioServerProDomainSettingsForUpdate")
.getter(getter(DomainSettingsForUpdate::rStudioServerProDomainSettingsForUpdate))
.setter(setter(Builder::rStudioServerProDomainSettingsForUpdate))
.constructor(RStudioServerProDomainSettingsForUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("RStudioServerProDomainSettingsForUpdate").build()).build();
private static final SdkField EXECUTION_ROLE_IDENTITY_CONFIG_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExecutionRoleIdentityConfig")
.getter(getter(DomainSettingsForUpdate::executionRoleIdentityConfigAsString))
.setter(setter(Builder::executionRoleIdentityConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionRoleIdentityConfig")
.build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(DomainSettingsForUpdate::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DOCKER_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DockerSettings")
.getter(getter(DomainSettingsForUpdate::dockerSettings)).setter(setter(Builder::dockerSettings))
.constructor(DockerSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DockerSettings").build()).build();
private static final SdkField AMAZON_Q_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AmazonQSettings")
.getter(getter(DomainSettingsForUpdate::amazonQSettings)).setter(setter(Builder::amazonQSettings))
.constructor(AmazonQSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmazonQSettings").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
R_STUDIO_SERVER_PRO_DOMAIN_SETTINGS_FOR_UPDATE_FIELD, EXECUTION_ROLE_IDENTITY_CONFIG_FIELD, SECURITY_GROUP_IDS_FIELD,
DOCKER_SETTINGS_FIELD, AMAZON_Q_SETTINGS_FIELD));
private static final long serialVersionUID = 1L;
private final RStudioServerProDomainSettingsForUpdate rStudioServerProDomainSettingsForUpdate;
private final String executionRoleIdentityConfig;
private final List securityGroupIds;
private final DockerSettings dockerSettings;
private final AmazonQSettings amazonQSettings;
private DomainSettingsForUpdate(BuilderImpl builder) {
this.rStudioServerProDomainSettingsForUpdate = builder.rStudioServerProDomainSettingsForUpdate;
this.executionRoleIdentityConfig = builder.executionRoleIdentityConfig;
this.securityGroupIds = builder.securityGroupIds;
this.dockerSettings = builder.dockerSettings;
this.amazonQSettings = builder.amazonQSettings;
}
/**
*
* A collection of RStudioServerPro
Domain-level app settings to update. A single
* RStudioServerPro
application is created for a domain.
*
*
* @return A collection of RStudioServerPro
Domain-level app settings to update. A single
* RStudioServerPro
application is created for a domain.
*/
public final RStudioServerProDomainSettingsForUpdate rStudioServerProDomainSettingsForUpdate() {
return rStudioServerProDomainSettingsForUpdate;
}
/**
*
* The configuration for attaching a SageMaker user profile name to the execution role as a sts:SourceIdentity key. This configuration can only be modified if there are no apps in the
* InService
or Pending
state.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #executionRoleIdentityConfig} will return {@link ExecutionRoleIdentityConfig#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #executionRoleIdentityConfigAsString}.
*
*
* @return The configuration for attaching a SageMaker user profile name to the execution role as a sts:SourceIdentity key. This configuration can only be modified if there are no apps in the
* InService
or Pending
state.
* @see ExecutionRoleIdentityConfig
*/
public final ExecutionRoleIdentityConfig executionRoleIdentityConfig() {
return ExecutionRoleIdentityConfig.fromValue(executionRoleIdentityConfig);
}
/**
*
* The configuration for attaching a SageMaker user profile name to the execution role as a sts:SourceIdentity key. This configuration can only be modified if there are no apps in the
* InService
or Pending
state.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #executionRoleIdentityConfig} will return {@link ExecutionRoleIdentityConfig#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #executionRoleIdentityConfigAsString}.
*
*
* @return The configuration for attaching a SageMaker user profile name to the execution role as a sts:SourceIdentity key. This configuration can only be modified if there are no apps in the
* InService
or Pending
state.
* @see ExecutionRoleIdentityConfig
*/
public final String executionRoleIdentityConfigAsString() {
return executionRoleIdentityConfig;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* The security groups for the Amazon Virtual Private Cloud that the Domain
uses for communication
* between Domain-level apps and user apps.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return The security groups for the Amazon Virtual Private Cloud that the Domain
uses for
* communication between Domain-level apps and user apps.
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
*
* A collection of settings that configure the domain's Docker interaction.
*
*
* @return A collection of settings that configure the domain's Docker interaction.
*/
public final DockerSettings dockerSettings() {
return dockerSettings;
}
/**
*
* A collection of settings that configure the Amazon Q experience within the domain.
*
*
* @return A collection of settings that configure the Amazon Q experience within the domain.
*/
public final AmazonQSettings amazonQSettings() {
return amazonQSettings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(rStudioServerProDomainSettingsForUpdate());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleIdentityConfigAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(dockerSettings());
hashCode = 31 * hashCode + Objects.hashCode(amazonQSettings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DomainSettingsForUpdate)) {
return false;
}
DomainSettingsForUpdate other = (DomainSettingsForUpdate) obj;
return Objects.equals(rStudioServerProDomainSettingsForUpdate(), other.rStudioServerProDomainSettingsForUpdate())
&& Objects.equals(executionRoleIdentityConfigAsString(), other.executionRoleIdentityConfigAsString())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(dockerSettings(), other.dockerSettings())
&& Objects.equals(amazonQSettings(), other.amazonQSettings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DomainSettingsForUpdate")
.add("RStudioServerProDomainSettingsForUpdate", rStudioServerProDomainSettingsForUpdate())
.add("ExecutionRoleIdentityConfig", executionRoleIdentityConfigAsString())
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("DockerSettings", dockerSettings()).add("AmazonQSettings", amazonQSettings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RStudioServerProDomainSettingsForUpdate":
return Optional.ofNullable(clazz.cast(rStudioServerProDomainSettingsForUpdate()));
case "ExecutionRoleIdentityConfig":
return Optional.ofNullable(clazz.cast(executionRoleIdentityConfigAsString()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "DockerSettings":
return Optional.ofNullable(clazz.cast(dockerSettings()));
case "AmazonQSettings":
return Optional.ofNullable(clazz.cast(amazonQSettings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function