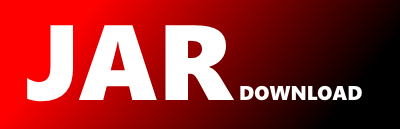
software.amazon.awssdk.services.sagemaker.model.UiConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provided configuration information for the worker UI for a labeling job. Provide either HumanTaskUiArn
* or UiTemplateS3Uri
.
*
*
* For named entity recognition, 3D point cloud and video frame labeling jobs, use HumanTaskUiArn
.
*
*
* For all other Ground Truth built-in task types and custom task types, use UiTemplateS3Uri
to specify the
* location of a worker task template in Amazon S3.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UiConfig implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UI_TEMPLATE_S3_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UiTemplateS3Uri").getter(getter(UiConfig::uiTemplateS3Uri)).setter(setter(Builder::uiTemplateS3Uri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UiTemplateS3Uri").build()).build();
private static final SdkField HUMAN_TASK_UI_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HumanTaskUiArn").getter(getter(UiConfig::humanTaskUiArn)).setter(setter(Builder::humanTaskUiArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HumanTaskUiArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UI_TEMPLATE_S3_URI_FIELD,
HUMAN_TASK_UI_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String uiTemplateS3Uri;
private final String humanTaskUiArn;
private UiConfig(BuilderImpl builder) {
this.uiTemplateS3Uri = builder.uiTemplateS3Uri;
this.humanTaskUiArn = builder.humanTaskUiArn;
}
/**
*
* The Amazon S3 bucket location of the UI template, or worker task template. This is the template used to render
* the worker UI and tools for labeling job tasks. For more information about the contents of a UI template, see Creating Your Custom
* Labeling Task Template.
*
*
* @return The Amazon S3 bucket location of the UI template, or worker task template. This is the template used to
* render the worker UI and tools for labeling job tasks. For more information about the contents of a UI
* template, see
* Creating Your Custom Labeling Task Template.
*/
public final String uiTemplateS3Uri() {
return uiTemplateS3Uri;
}
/**
*
* The ARN of the worker task template used to render the worker UI and tools for labeling job tasks.
*
*
* Use this parameter when you are creating a labeling job for named entity recognition, 3D point cloud and video
* frame labeling jobs. Use your labeling job task type to select one of the following ARNs and use it with this
* parameter when you create a labeling job. Replace aws-region
with the Amazon Web Services Region you
* are creating your labeling job in. For example, replace aws-region
with us-west-1
if
* you create a labeling job in US West (N. California).
*
*
* Named Entity Recognition
*
*
* Use the following HumanTaskUiArn
for named entity recognition labeling jobs:
*
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/NamedEntityRecognition
*
*
* 3D Point Cloud HumanTaskUiArns
*
*
* Use this HumanTaskUiArn
for 3D point cloud object detection and 3D point cloud object detection
* adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudObjectDetection
*
*
*
*
* Use this HumanTaskUiArn
for 3D point cloud object tracking and 3D point cloud object tracking
* adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudObjectTracking
*
*
*
*
* Use this HumanTaskUiArn
for 3D point cloud semantic segmentation and 3D point cloud semantic
* segmentation adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudSemanticSegmentation
*
*
*
*
* Video Frame HumanTaskUiArns
*
*
* Use this HumanTaskUiArn
for video frame object detection and video frame object detection adjustment
* labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:region:394669845002:human-task-ui/VideoObjectDetection
*
*
*
*
* Use this HumanTaskUiArn
for video frame object tracking and video frame object tracking adjustment
* labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/VideoObjectTracking
*
*
*
*
* @return The ARN of the worker task template used to render the worker UI and tools for labeling job tasks.
*
* Use this parameter when you are creating a labeling job for named entity recognition, 3D point cloud and
* video frame labeling jobs. Use your labeling job task type to select one of the following ARNs and use it
* with this parameter when you create a labeling job. Replace aws-region
with the Amazon Web
* Services Region you are creating your labeling job in. For example, replace aws-region
with
* us-west-1
if you create a labeling job in US West (N. California).
*
*
* Named Entity Recognition
*
*
* Use the following HumanTaskUiArn
for named entity recognition labeling jobs:
*
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/NamedEntityRecognition
*
*
* 3D Point Cloud HumanTaskUiArns
*
*
* Use this HumanTaskUiArn
for 3D point cloud object detection and 3D point cloud object
* detection adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudObjectDetection
*
*
*
*
* Use this HumanTaskUiArn
for 3D point cloud object tracking and 3D point cloud object
* tracking adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudObjectTracking
*
*
*
*
* Use this HumanTaskUiArn
for 3D point cloud semantic segmentation and 3D point cloud semantic
* segmentation adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/PointCloudSemanticSegmentation
*
*
*
*
* Video Frame HumanTaskUiArns
*
*
* Use this HumanTaskUiArn
for video frame object detection and video frame object detection
* adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:region:394669845002:human-task-ui/VideoObjectDetection
*
*
*
*
* Use this HumanTaskUiArn
for video frame object tracking and video frame object tracking
* adjustment labeling jobs.
*
*
* -
*
* arn:aws:sagemaker:aws-region:394669845002:human-task-ui/VideoObjectTracking
*
*
*/
public final String humanTaskUiArn() {
return humanTaskUiArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(uiTemplateS3Uri());
hashCode = 31 * hashCode + Objects.hashCode(humanTaskUiArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UiConfig)) {
return false;
}
UiConfig other = (UiConfig) obj;
return Objects.equals(uiTemplateS3Uri(), other.uiTemplateS3Uri())
&& Objects.equals(humanTaskUiArn(), other.humanTaskUiArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UiConfig").add("UiTemplateS3Uri", uiTemplateS3Uri()).add("HumanTaskUiArn", humanTaskUiArn())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UiTemplateS3Uri":
return Optional.ofNullable(clazz.cast(uiTemplateS3Uri()));
case "HumanTaskUiArn":
return Optional.ofNullable(clazz.cast(humanTaskUiArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function