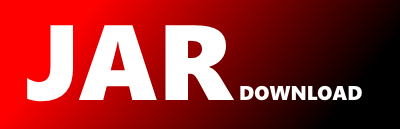
software.amazon.awssdk.services.sagemaker.model.CompilationJobSummary Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A summary of a model compilation job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CompilationJobSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField COMPILATION_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompilationJobName").getter(getter(CompilationJobSummary::compilationJobName))
.setter(setter(Builder::compilationJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationJobName").build())
.build();
private static final SdkField COMPILATION_JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompilationJobArn").getter(getter(CompilationJobSummary::compilationJobArn))
.setter(setter(Builder::compilationJobArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationJobArn").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(CompilationJobSummary::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField COMPILATION_START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompilationStartTime").getter(getter(CompilationJobSummary::compilationStartTime))
.setter(setter(Builder::compilationStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationStartTime").build())
.build();
private static final SdkField COMPILATION_END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompilationEndTime").getter(getter(CompilationJobSummary::compilationEndTime))
.setter(setter(Builder::compilationEndTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationEndTime").build())
.build();
private static final SdkField COMPILATION_TARGET_DEVICE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompilationTargetDevice").getter(getter(CompilationJobSummary::compilationTargetDeviceAsString))
.setter(setter(Builder::compilationTargetDevice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationTargetDevice").build())
.build();
private static final SdkField COMPILATION_TARGET_PLATFORM_OS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CompilationTargetPlatformOs")
.getter(getter(CompilationJobSummary::compilationTargetPlatformOsAsString))
.setter(setter(Builder::compilationTargetPlatformOs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationTargetPlatformOs")
.build()).build();
private static final SdkField COMPILATION_TARGET_PLATFORM_ARCH_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CompilationTargetPlatformArch")
.getter(getter(CompilationJobSummary::compilationTargetPlatformArchAsString))
.setter(setter(Builder::compilationTargetPlatformArch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationTargetPlatformArch")
.build()).build();
private static final SdkField COMPILATION_TARGET_PLATFORM_ACCELERATOR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CompilationTargetPlatformAccelerator")
.getter(getter(CompilationJobSummary::compilationTargetPlatformAcceleratorAsString))
.setter(setter(Builder::compilationTargetPlatformAccelerator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CompilationTargetPlatformAccelerator").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedTime").getter(getter(CompilationJobSummary::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedTime").build()).build();
private static final SdkField COMPILATION_JOB_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompilationJobStatus").getter(getter(CompilationJobSummary::compilationJobStatusAsString))
.setter(setter(Builder::compilationJobStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompilationJobStatus").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPILATION_JOB_NAME_FIELD,
COMPILATION_JOB_ARN_FIELD, CREATION_TIME_FIELD, COMPILATION_START_TIME_FIELD, COMPILATION_END_TIME_FIELD,
COMPILATION_TARGET_DEVICE_FIELD, COMPILATION_TARGET_PLATFORM_OS_FIELD, COMPILATION_TARGET_PLATFORM_ARCH_FIELD,
COMPILATION_TARGET_PLATFORM_ACCELERATOR_FIELD, LAST_MODIFIED_TIME_FIELD, COMPILATION_JOB_STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String compilationJobName;
private final String compilationJobArn;
private final Instant creationTime;
private final Instant compilationStartTime;
private final Instant compilationEndTime;
private final String compilationTargetDevice;
private final String compilationTargetPlatformOs;
private final String compilationTargetPlatformArch;
private final String compilationTargetPlatformAccelerator;
private final Instant lastModifiedTime;
private final String compilationJobStatus;
private CompilationJobSummary(BuilderImpl builder) {
this.compilationJobName = builder.compilationJobName;
this.compilationJobArn = builder.compilationJobArn;
this.creationTime = builder.creationTime;
this.compilationStartTime = builder.compilationStartTime;
this.compilationEndTime = builder.compilationEndTime;
this.compilationTargetDevice = builder.compilationTargetDevice;
this.compilationTargetPlatformOs = builder.compilationTargetPlatformOs;
this.compilationTargetPlatformArch = builder.compilationTargetPlatformArch;
this.compilationTargetPlatformAccelerator = builder.compilationTargetPlatformAccelerator;
this.lastModifiedTime = builder.lastModifiedTime;
this.compilationJobStatus = builder.compilationJobStatus;
}
/**
*
* The name of the model compilation job that you want a summary for.
*
*
* @return The name of the model compilation job that you want a summary for.
*/
public final String compilationJobName() {
return compilationJobName;
}
/**
*
* The Amazon Resource Name (ARN) of the model compilation job.
*
*
* @return The Amazon Resource Name (ARN) of the model compilation job.
*/
public final String compilationJobArn() {
return compilationJobArn;
}
/**
*
* The time when the model compilation job was created.
*
*
* @return The time when the model compilation job was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The time when the model compilation job started.
*
*
* @return The time when the model compilation job started.
*/
public final Instant compilationStartTime() {
return compilationStartTime;
}
/**
*
* The time when the model compilation job completed.
*
*
* @return The time when the model compilation job completed.
*/
public final Instant compilationEndTime() {
return compilationEndTime;
}
/**
*
* The type of device that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetDevice} will return {@link TargetDevice#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #compilationTargetDeviceAsString}.
*
*
* @return The type of device that the model will run on after the compilation job has completed.
* @see TargetDevice
*/
public final TargetDevice compilationTargetDevice() {
return TargetDevice.fromValue(compilationTargetDevice);
}
/**
*
* The type of device that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetDevice} will return {@link TargetDevice#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #compilationTargetDeviceAsString}.
*
*
* @return The type of device that the model will run on after the compilation job has completed.
* @see TargetDevice
*/
public final String compilationTargetDeviceAsString() {
return compilationTargetDevice;
}
/**
*
* The type of OS that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformOs} will return {@link TargetPlatformOs#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #compilationTargetPlatformOsAsString}.
*
*
* @return The type of OS that the model will run on after the compilation job has completed.
* @see TargetPlatformOs
*/
public final TargetPlatformOs compilationTargetPlatformOs() {
return TargetPlatformOs.fromValue(compilationTargetPlatformOs);
}
/**
*
* The type of OS that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformOs} will return {@link TargetPlatformOs#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #compilationTargetPlatformOsAsString}.
*
*
* @return The type of OS that the model will run on after the compilation job has completed.
* @see TargetPlatformOs
*/
public final String compilationTargetPlatformOsAsString() {
return compilationTargetPlatformOs;
}
/**
*
* The type of architecture that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformArch} will return {@link TargetPlatformArch#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #compilationTargetPlatformArchAsString}.
*
*
* @return The type of architecture that the model will run on after the compilation job has completed.
* @see TargetPlatformArch
*/
public final TargetPlatformArch compilationTargetPlatformArch() {
return TargetPlatformArch.fromValue(compilationTargetPlatformArch);
}
/**
*
* The type of architecture that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformArch} will return {@link TargetPlatformArch#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #compilationTargetPlatformArchAsString}.
*
*
* @return The type of architecture that the model will run on after the compilation job has completed.
* @see TargetPlatformArch
*/
public final String compilationTargetPlatformArchAsString() {
return compilationTargetPlatformArch;
}
/**
*
* The type of accelerator that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformAccelerator} will return
* {@link TargetPlatformAccelerator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compilationTargetPlatformAcceleratorAsString}.
*
*
* @return The type of accelerator that the model will run on after the compilation job has completed.
* @see TargetPlatformAccelerator
*/
public final TargetPlatformAccelerator compilationTargetPlatformAccelerator() {
return TargetPlatformAccelerator.fromValue(compilationTargetPlatformAccelerator);
}
/**
*
* The type of accelerator that the model will run on after the compilation job has completed.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationTargetPlatformAccelerator} will return
* {@link TargetPlatformAccelerator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compilationTargetPlatformAcceleratorAsString}.
*
*
* @return The type of accelerator that the model will run on after the compilation job has completed.
* @see TargetPlatformAccelerator
*/
public final String compilationTargetPlatformAcceleratorAsString() {
return compilationTargetPlatformAccelerator;
}
/**
*
* The time when the model compilation job was last modified.
*
*
* @return The time when the model compilation job was last modified.
*/
public final Instant lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* The status of the model compilation job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationJobStatus} will return {@link CompilationJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #compilationJobStatusAsString}.
*
*
* @return The status of the model compilation job.
* @see CompilationJobStatus
*/
public final CompilationJobStatus compilationJobStatus() {
return CompilationJobStatus.fromValue(compilationJobStatus);
}
/**
*
* The status of the model compilation job.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compilationJobStatus} will return {@link CompilationJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #compilationJobStatusAsString}.
*
*
* @return The status of the model compilation job.
* @see CompilationJobStatus
*/
public final String compilationJobStatusAsString() {
return compilationJobStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(compilationJobName());
hashCode = 31 * hashCode + Objects.hashCode(compilationJobArn());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(compilationStartTime());
hashCode = 31 * hashCode + Objects.hashCode(compilationEndTime());
hashCode = 31 * hashCode + Objects.hashCode(compilationTargetDeviceAsString());
hashCode = 31 * hashCode + Objects.hashCode(compilationTargetPlatformOsAsString());
hashCode = 31 * hashCode + Objects.hashCode(compilationTargetPlatformArchAsString());
hashCode = 31 * hashCode + Objects.hashCode(compilationTargetPlatformAcceleratorAsString());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(compilationJobStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CompilationJobSummary)) {
return false;
}
CompilationJobSummary other = (CompilationJobSummary) obj;
return Objects.equals(compilationJobName(), other.compilationJobName())
&& Objects.equals(compilationJobArn(), other.compilationJobArn())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(compilationStartTime(), other.compilationStartTime())
&& Objects.equals(compilationEndTime(), other.compilationEndTime())
&& Objects.equals(compilationTargetDeviceAsString(), other.compilationTargetDeviceAsString())
&& Objects.equals(compilationTargetPlatformOsAsString(), other.compilationTargetPlatformOsAsString())
&& Objects.equals(compilationTargetPlatformArchAsString(), other.compilationTargetPlatformArchAsString())
&& Objects.equals(compilationTargetPlatformAcceleratorAsString(),
other.compilationTargetPlatformAcceleratorAsString())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(compilationJobStatusAsString(), other.compilationJobStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CompilationJobSummary").add("CompilationJobName", compilationJobName())
.add("CompilationJobArn", compilationJobArn()).add("CreationTime", creationTime())
.add("CompilationStartTime", compilationStartTime()).add("CompilationEndTime", compilationEndTime())
.add("CompilationTargetDevice", compilationTargetDeviceAsString())
.add("CompilationTargetPlatformOs", compilationTargetPlatformOsAsString())
.add("CompilationTargetPlatformArch", compilationTargetPlatformArchAsString())
.add("CompilationTargetPlatformAccelerator", compilationTargetPlatformAcceleratorAsString())
.add("LastModifiedTime", lastModifiedTime()).add("CompilationJobStatus", compilationJobStatusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CompilationJobName":
return Optional.ofNullable(clazz.cast(compilationJobName()));
case "CompilationJobArn":
return Optional.ofNullable(clazz.cast(compilationJobArn()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "CompilationStartTime":
return Optional.ofNullable(clazz.cast(compilationStartTime()));
case "CompilationEndTime":
return Optional.ofNullable(clazz.cast(compilationEndTime()));
case "CompilationTargetDevice":
return Optional.ofNullable(clazz.cast(compilationTargetDeviceAsString()));
case "CompilationTargetPlatformOs":
return Optional.ofNullable(clazz.cast(compilationTargetPlatformOsAsString()));
case "CompilationTargetPlatformArch":
return Optional.ofNullable(clazz.cast(compilationTargetPlatformArchAsString()));
case "CompilationTargetPlatformAccelerator":
return Optional.ofNullable(clazz.cast(compilationTargetPlatformAcceleratorAsString()));
case "LastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "CompilationJobStatus":
return Optional.ofNullable(clazz.cast(compilationJobStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("CompilationJobName", COMPILATION_JOB_NAME_FIELD);
map.put("CompilationJobArn", COMPILATION_JOB_ARN_FIELD);
map.put("CreationTime", CREATION_TIME_FIELD);
map.put("CompilationStartTime", COMPILATION_START_TIME_FIELD);
map.put("CompilationEndTime", COMPILATION_END_TIME_FIELD);
map.put("CompilationTargetDevice", COMPILATION_TARGET_DEVICE_FIELD);
map.put("CompilationTargetPlatformOs", COMPILATION_TARGET_PLATFORM_OS_FIELD);
map.put("CompilationTargetPlatformArch", COMPILATION_TARGET_PLATFORM_ARCH_FIELD);
map.put("CompilationTargetPlatformAccelerator", COMPILATION_TARGET_PLATFORM_ACCELERATOR_FIELD);
map.put("LastModifiedTime", LAST_MODIFIED_TIME_FIELD);
map.put("CompilationJobStatus", COMPILATION_JOB_STATUS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function