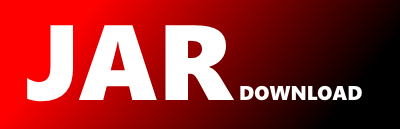
software.amazon.awssdk.services.sagemaker.model.CreateAutoMlJobRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateAutoMlJobRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField AUTO_ML_JOB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoMLJobName").getter(getter(CreateAutoMlJobRequest::autoMLJobName))
.setter(setter(Builder::autoMLJobName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobName").build()).build();
private static final SdkField> INPUT_DATA_CONFIG_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InputDataConfig")
.getter(getter(CreateAutoMlJobRequest::inputDataConfig))
.setter(setter(Builder::inputDataConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDataConfig").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoMLChannel::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OUTPUT_DATA_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OutputDataConfig")
.getter(getter(CreateAutoMlJobRequest::outputDataConfig)).setter(setter(Builder::outputDataConfig))
.constructor(AutoMLOutputDataConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputDataConfig").build()).build();
private static final SdkField PROBLEM_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProblemType").getter(getter(CreateAutoMlJobRequest::problemTypeAsString))
.setter(setter(Builder::problemType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProblemType").build()).build();
private static final SdkField AUTO_ML_JOB_OBJECTIVE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLJobObjective")
.getter(getter(CreateAutoMlJobRequest::autoMLJobObjective)).setter(setter(Builder::autoMLJobObjective))
.constructor(AutoMLJobObjective::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobObjective").build())
.build();
private static final SdkField AUTO_ML_JOB_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoMLJobConfig")
.getter(getter(CreateAutoMlJobRequest::autoMLJobConfig)).setter(setter(Builder::autoMLJobConfig))
.constructor(AutoMLJobConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMLJobConfig").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(CreateAutoMlJobRequest::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField GENERATE_CANDIDATE_DEFINITIONS_ONLY_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("GenerateCandidateDefinitionsOnly")
.getter(getter(CreateAutoMlJobRequest::generateCandidateDefinitionsOnly))
.setter(setter(Builder::generateCandidateDefinitionsOnly))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GenerateCandidateDefinitionsOnly")
.build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateAutoMlJobRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MODEL_DEPLOY_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ModelDeployConfig")
.getter(getter(CreateAutoMlJobRequest::modelDeployConfig)).setter(setter(Builder::modelDeployConfig))
.constructor(ModelDeployConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelDeployConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AUTO_ML_JOB_NAME_FIELD,
INPUT_DATA_CONFIG_FIELD, OUTPUT_DATA_CONFIG_FIELD, PROBLEM_TYPE_FIELD, AUTO_ML_JOB_OBJECTIVE_FIELD,
AUTO_ML_JOB_CONFIG_FIELD, ROLE_ARN_FIELD, GENERATE_CANDIDATE_DEFINITIONS_ONLY_FIELD, TAGS_FIELD,
MODEL_DEPLOY_CONFIG_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String autoMLJobName;
private final List inputDataConfig;
private final AutoMLOutputDataConfig outputDataConfig;
private final String problemType;
private final AutoMLJobObjective autoMLJobObjective;
private final AutoMLJobConfig autoMLJobConfig;
private final String roleArn;
private final Boolean generateCandidateDefinitionsOnly;
private final List tags;
private final ModelDeployConfig modelDeployConfig;
private CreateAutoMlJobRequest(BuilderImpl builder) {
super(builder);
this.autoMLJobName = builder.autoMLJobName;
this.inputDataConfig = builder.inputDataConfig;
this.outputDataConfig = builder.outputDataConfig;
this.problemType = builder.problemType;
this.autoMLJobObjective = builder.autoMLJobObjective;
this.autoMLJobConfig = builder.autoMLJobConfig;
this.roleArn = builder.roleArn;
this.generateCandidateDefinitionsOnly = builder.generateCandidateDefinitionsOnly;
this.tags = builder.tags;
this.modelDeployConfig = builder.modelDeployConfig;
}
/**
*
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*
*
* @return Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*/
public final String autoMLJobName() {
return autoMLJobName;
}
/**
* For responses, this returns true if the service returned a value for the InputDataConfig property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInputDataConfig() {
return inputDataConfig != null && !(inputDataConfig instanceof SdkAutoConstructList);
}
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInputDataConfig} method.
*
*
* @return An array of channel objects that describes the input data and its location. Each channel is a named input
* source. Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is
* required for the training dataset. There is not a minimum number of rows required for the validation
* dataset.
*/
public final List inputDataConfig() {
return inputDataConfig;
}
/**
*
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an AutoML job.
* Format(s) supported: CSV.
*
*
* @return Provides information about encryption and the Amazon S3 output path needed to store artifacts from an
* AutoML job. Format(s) supported: CSV.
*/
public final AutoMLOutputDataConfig outputDataConfig() {
return outputDataConfig;
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #problemType} will
* return {@link ProblemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #problemTypeAsString}.
*
*
* @return Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
* @see ProblemType
*/
public final ProblemType problemType() {
return ProblemType.fromValue(problemType);
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #problemType} will
* return {@link ProblemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #problemTypeAsString}.
*
*
* @return Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
* @see ProblemType
*/
public final String problemTypeAsString() {
return problemType;
}
/**
*
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default objective
* metric depends on the problem type. See AutoMLJobObjective for the default values.
*
*
* @return Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default
* objective metric depends on the problem type. See AutoMLJobObjective for the default values.
*/
public final AutoMLJobObjective autoMLJobObjective() {
return autoMLJobObjective;
}
/**
*
* A collection of settings used to configure an AutoML job.
*
*
* @return A collection of settings used to configure an AutoML job.
*/
public final AutoMLJobConfig autoMLJobConfig() {
return autoMLJobConfig;
}
/**
*
* The ARN of the role that is used to access the data.
*
*
* @return The ARN of the role that is used to access the data.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*
* @return Generates possible candidates without training the models. A candidate is a combination of data
* preprocessors, algorithms, and algorithm parameter settings.
*/
public final Boolean generateCandidateDefinitionsOnly() {
return generateCandidateDefinitionsOnly;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web
* ServicesResources. Tag keys must be unique per resource.
*/
public final List tags() {
return tags;
}
/**
*
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*
*
* @return Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*/
public final ModelDeployConfig modelDeployConfig() {
return modelDeployConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobName());
hashCode = 31 * hashCode + Objects.hashCode(hasInputDataConfig() ? inputDataConfig() : null);
hashCode = 31 * hashCode + Objects.hashCode(outputDataConfig());
hashCode = 31 * hashCode + Objects.hashCode(problemTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobObjective());
hashCode = 31 * hashCode + Objects.hashCode(autoMLJobConfig());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(generateCandidateDefinitionsOnly());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(modelDeployConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateAutoMlJobRequest)) {
return false;
}
CreateAutoMlJobRequest other = (CreateAutoMlJobRequest) obj;
return Objects.equals(autoMLJobName(), other.autoMLJobName()) && hasInputDataConfig() == other.hasInputDataConfig()
&& Objects.equals(inputDataConfig(), other.inputDataConfig())
&& Objects.equals(outputDataConfig(), other.outputDataConfig())
&& Objects.equals(problemTypeAsString(), other.problemTypeAsString())
&& Objects.equals(autoMLJobObjective(), other.autoMLJobObjective())
&& Objects.equals(autoMLJobConfig(), other.autoMLJobConfig()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(generateCandidateDefinitionsOnly(), other.generateCandidateDefinitionsOnly())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(modelDeployConfig(), other.modelDeployConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateAutoMlJobRequest").add("AutoMLJobName", autoMLJobName())
.add("InputDataConfig", hasInputDataConfig() ? inputDataConfig() : null)
.add("OutputDataConfig", outputDataConfig()).add("ProblemType", problemTypeAsString())
.add("AutoMLJobObjective", autoMLJobObjective()).add("AutoMLJobConfig", autoMLJobConfig())
.add("RoleArn", roleArn()).add("GenerateCandidateDefinitionsOnly", generateCandidateDefinitionsOnly())
.add("Tags", hasTags() ? tags() : null).add("ModelDeployConfig", modelDeployConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AutoMLJobName":
return Optional.ofNullable(clazz.cast(autoMLJobName()));
case "InputDataConfig":
return Optional.ofNullable(clazz.cast(inputDataConfig()));
case "OutputDataConfig":
return Optional.ofNullable(clazz.cast(outputDataConfig()));
case "ProblemType":
return Optional.ofNullable(clazz.cast(problemTypeAsString()));
case "AutoMLJobObjective":
return Optional.ofNullable(clazz.cast(autoMLJobObjective()));
case "AutoMLJobConfig":
return Optional.ofNullable(clazz.cast(autoMLJobConfig()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "GenerateCandidateDefinitionsOnly":
return Optional.ofNullable(clazz.cast(generateCandidateDefinitionsOnly()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ModelDeployConfig":
return Optional.ofNullable(clazz.cast(modelDeployConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("AutoMLJobName", AUTO_ML_JOB_NAME_FIELD);
map.put("InputDataConfig", INPUT_DATA_CONFIG_FIELD);
map.put("OutputDataConfig", OUTPUT_DATA_CONFIG_FIELD);
map.put("ProblemType", PROBLEM_TYPE_FIELD);
map.put("AutoMLJobObjective", AUTO_ML_JOB_OBJECTIVE_FIELD);
map.put("AutoMLJobConfig", AUTO_ML_JOB_CONFIG_FIELD);
map.put("RoleArn", ROLE_ARN_FIELD);
map.put("GenerateCandidateDefinitionsOnly", GENERATE_CANDIDATE_DEFINITIONS_ONLY_FIELD);
map.put("Tags", TAGS_FIELD);
map.put("ModelDeployConfig", MODEL_DEPLOY_CONFIG_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function