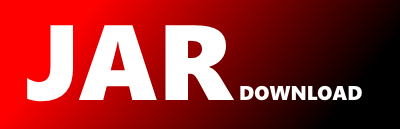
software.amazon.awssdk.services.sagemaker.model.RecommendationJobContainerConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies mandatory fields for running an Inference Recommender job directly in the CreateInferenceRecommendationsJob API. The fields specified in ContainerConfig
override the
* corresponding fields in the model package. Use ContainerConfig
if you want to specify these fields for
* the recommendation job but don't want to edit them in your model package.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RecommendationJobContainerConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Domain")
.getter(getter(RecommendationJobContainerConfig::domain)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Domain").build()).build();
private static final SdkField TASK_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Task")
.getter(getter(RecommendationJobContainerConfig::task)).setter(setter(Builder::task))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Task").build()).build();
private static final SdkField FRAMEWORK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Framework").getter(getter(RecommendationJobContainerConfig::framework))
.setter(setter(Builder::framework))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Framework").build()).build();
private static final SdkField FRAMEWORK_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FrameworkVersion").getter(getter(RecommendationJobContainerConfig::frameworkVersion))
.setter(setter(Builder::frameworkVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FrameworkVersion").build()).build();
private static final SdkField PAYLOAD_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PayloadConfig")
.getter(getter(RecommendationJobContainerConfig::payloadConfig)).setter(setter(Builder::payloadConfig))
.constructor(RecommendationJobPayloadConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PayloadConfig").build()).build();
private static final SdkField NEAREST_MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NearestModelName").getter(getter(RecommendationJobContainerConfig::nearestModelName))
.setter(setter(Builder::nearestModelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NearestModelName").build()).build();
private static final SdkField> SUPPORTED_INSTANCE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedInstanceTypes")
.getter(getter(RecommendationJobContainerConfig::supportedInstanceTypes))
.setter(setter(Builder::supportedInstanceTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedInstanceTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SUPPORTED_ENDPOINT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SupportedEndpointType").getter(getter(RecommendationJobContainerConfig::supportedEndpointTypeAsString))
.setter(setter(Builder::supportedEndpointType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedEndpointType").build())
.build();
private static final SdkField DATA_INPUT_CONFIG_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataInputConfig").getter(getter(RecommendationJobContainerConfig::dataInputConfig))
.setter(setter(Builder::dataInputConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataInputConfig").build()).build();
private static final SdkField> SUPPORTED_RESPONSE_MIME_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedResponseMIMETypes")
.getter(getter(RecommendationJobContainerConfig::supportedResponseMIMETypes))
.setter(setter(Builder::supportedResponseMIMETypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedResponseMIMETypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_FIELD, TASK_FIELD,
FRAMEWORK_FIELD, FRAMEWORK_VERSION_FIELD, PAYLOAD_CONFIG_FIELD, NEAREST_MODEL_NAME_FIELD,
SUPPORTED_INSTANCE_TYPES_FIELD, SUPPORTED_ENDPOINT_TYPE_FIELD, DATA_INPUT_CONFIG_FIELD,
SUPPORTED_RESPONSE_MIME_TYPES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String domain;
private final String task;
private final String framework;
private final String frameworkVersion;
private final RecommendationJobPayloadConfig payloadConfig;
private final String nearestModelName;
private final List supportedInstanceTypes;
private final String supportedEndpointType;
private final String dataInputConfig;
private final List supportedResponseMIMETypes;
private RecommendationJobContainerConfig(BuilderImpl builder) {
this.domain = builder.domain;
this.task = builder.task;
this.framework = builder.framework;
this.frameworkVersion = builder.frameworkVersion;
this.payloadConfig = builder.payloadConfig;
this.nearestModelName = builder.nearestModelName;
this.supportedInstanceTypes = builder.supportedInstanceTypes;
this.supportedEndpointType = builder.supportedEndpointType;
this.dataInputConfig = builder.dataInputConfig;
this.supportedResponseMIMETypes = builder.supportedResponseMIMETypes;
}
/**
*
* The machine learning domain of the model and its components.
*
*
* Valid Values: COMPUTER_VISION | NATURAL_LANGUAGE_PROCESSING | MACHINE_LEARNING
*
*
* @return The machine learning domain of the model and its components.
*
* Valid Values: COMPUTER_VISION | NATURAL_LANGUAGE_PROCESSING | MACHINE_LEARNING
*/
public final String domain() {
return domain;
}
/**
*
* The machine learning task that the model accomplishes.
*
*
* Valid Values:
* IMAGE_CLASSIFICATION | OBJECT_DETECTION | TEXT_GENERATION | IMAGE_SEGMENTATION | FILL_MASK | CLASSIFICATION | REGRESSION | OTHER
*
*
* @return The machine learning task that the model accomplishes.
*
* Valid Values:
* IMAGE_CLASSIFICATION | OBJECT_DETECTION | TEXT_GENERATION | IMAGE_SEGMENTATION | FILL_MASK | CLASSIFICATION | REGRESSION | OTHER
*/
public final String task() {
return task;
}
/**
*
* The machine learning framework of the container image.
*
*
* Valid Values: TENSORFLOW | PYTORCH | XGBOOST | SAGEMAKER-SCIKIT-LEARN
*
*
* @return The machine learning framework of the container image.
*
* Valid Values: TENSORFLOW | PYTORCH | XGBOOST | SAGEMAKER-SCIKIT-LEARN
*/
public final String framework() {
return framework;
}
/**
*
* The framework version of the container image.
*
*
* @return The framework version of the container image.
*/
public final String frameworkVersion() {
return frameworkVersion;
}
/**
*
* Specifies the SamplePayloadUrl
and all other sample payload-related fields.
*
*
* @return Specifies the SamplePayloadUrl
and all other sample payload-related fields.
*/
public final RecommendationJobPayloadConfig payloadConfig() {
return payloadConfig;
}
/**
*
* The name of a pre-trained machine learning model benchmarked by Amazon SageMaker Inference Recommender that
* matches your model.
*
*
* Valid Values:
* efficientnetb7 | unet | xgboost | faster-rcnn-resnet101 | nasnetlarge | vgg16 | inception-v3 | mask-rcnn | sagemaker-scikit-learn | densenet201-gluon | resnet18v2-gluon | xception | densenet201 | yolov4 | resnet152 | bert-base-cased | xceptionV1-keras | resnet50 | retinanet
*
*
* @return The name of a pre-trained machine learning model benchmarked by Amazon SageMaker Inference Recommender
* that matches your model.
*
* Valid Values:
* efficientnetb7 | unet | xgboost | faster-rcnn-resnet101 | nasnetlarge | vgg16 | inception-v3 | mask-rcnn | sagemaker-scikit-learn | densenet201-gluon | resnet18v2-gluon | xception | densenet201 | yolov4 | resnet152 | bert-base-cased | xceptionV1-keras | resnet50 | retinanet
*/
public final String nearestModelName() {
return nearestModelName;
}
/**
* For responses, this returns true if the service returned a value for the SupportedInstanceTypes property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSupportedInstanceTypes() {
return supportedInstanceTypes != null && !(supportedInstanceTypes instanceof SdkAutoConstructList);
}
/**
*
* A list of the instance types that are used to generate inferences in real-time.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedInstanceTypes} method.
*
*
* @return A list of the instance types that are used to generate inferences in real-time.
*/
public final List supportedInstanceTypes() {
return supportedInstanceTypes;
}
/**
*
* The endpoint type to receive recommendations for. By default this is null, and the results of the inference
* recommendation job return a combined list of both real-time and serverless benchmarks. By specifying a value for
* this field, you can receive a longer list of benchmarks for the desired endpoint type.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #supportedEndpointType} will return {@link RecommendationJobSupportedEndpointType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #supportedEndpointTypeAsString}.
*
*
* @return The endpoint type to receive recommendations for. By default this is null, and the results of the
* inference recommendation job return a combined list of both real-time and serverless benchmarks. By
* specifying a value for this field, you can receive a longer list of benchmarks for the desired endpoint
* type.
* @see RecommendationJobSupportedEndpointType
*/
public final RecommendationJobSupportedEndpointType supportedEndpointType() {
return RecommendationJobSupportedEndpointType.fromValue(supportedEndpointType);
}
/**
*
* The endpoint type to receive recommendations for. By default this is null, and the results of the inference
* recommendation job return a combined list of both real-time and serverless benchmarks. By specifying a value for
* this field, you can receive a longer list of benchmarks for the desired endpoint type.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #supportedEndpointType} will return {@link RecommendationJobSupportedEndpointType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #supportedEndpointTypeAsString}.
*
*
* @return The endpoint type to receive recommendations for. By default this is null, and the results of the
* inference recommendation job return a combined list of both real-time and serverless benchmarks. By
* specifying a value for this field, you can receive a longer list of benchmarks for the desired endpoint
* type.
* @see RecommendationJobSupportedEndpointType
*/
public final String supportedEndpointTypeAsString() {
return supportedEndpointType;
}
/**
*
* Specifies the name and shape of the expected data inputs for your trained model with a JSON dictionary form. This
* field is used for optimizing your model using SageMaker Neo. For more information, see DataInputConfig.
*
*
* @return Specifies the name and shape of the expected data inputs for your trained model with a JSON dictionary
* form. This field is used for optimizing your model using SageMaker Neo. For more information, see DataInputConfig.
*/
public final String dataInputConfig() {
return dataInputConfig;
}
/**
* For responses, this returns true if the service returned a value for the SupportedResponseMIMETypes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSupportedResponseMIMETypes() {
return supportedResponseMIMETypes != null && !(supportedResponseMIMETypes instanceof SdkAutoConstructList);
}
/**
*
* The supported MIME types for the output data.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedResponseMIMETypes} method.
*
*
* @return The supported MIME types for the output data.
*/
public final List supportedResponseMIMETypes() {
return supportedResponseMIMETypes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(domain());
hashCode = 31 * hashCode + Objects.hashCode(task());
hashCode = 31 * hashCode + Objects.hashCode(framework());
hashCode = 31 * hashCode + Objects.hashCode(frameworkVersion());
hashCode = 31 * hashCode + Objects.hashCode(payloadConfig());
hashCode = 31 * hashCode + Objects.hashCode(nearestModelName());
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedInstanceTypes() ? supportedInstanceTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(supportedEndpointTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(dataInputConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedResponseMIMETypes() ? supportedResponseMIMETypes() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RecommendationJobContainerConfig)) {
return false;
}
RecommendationJobContainerConfig other = (RecommendationJobContainerConfig) obj;
return Objects.equals(domain(), other.domain()) && Objects.equals(task(), other.task())
&& Objects.equals(framework(), other.framework()) && Objects.equals(frameworkVersion(), other.frameworkVersion())
&& Objects.equals(payloadConfig(), other.payloadConfig())
&& Objects.equals(nearestModelName(), other.nearestModelName())
&& hasSupportedInstanceTypes() == other.hasSupportedInstanceTypes()
&& Objects.equals(supportedInstanceTypes(), other.supportedInstanceTypes())
&& Objects.equals(supportedEndpointTypeAsString(), other.supportedEndpointTypeAsString())
&& Objects.equals(dataInputConfig(), other.dataInputConfig())
&& hasSupportedResponseMIMETypes() == other.hasSupportedResponseMIMETypes()
&& Objects.equals(supportedResponseMIMETypes(), other.supportedResponseMIMETypes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RecommendationJobContainerConfig").add("Domain", domain()).add("Task", task())
.add("Framework", framework()).add("FrameworkVersion", frameworkVersion()).add("PayloadConfig", payloadConfig())
.add("NearestModelName", nearestModelName())
.add("SupportedInstanceTypes", hasSupportedInstanceTypes() ? supportedInstanceTypes() : null)
.add("SupportedEndpointType", supportedEndpointTypeAsString()).add("DataInputConfig", dataInputConfig())
.add("SupportedResponseMIMETypes", hasSupportedResponseMIMETypes() ? supportedResponseMIMETypes() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Domain":
return Optional.ofNullable(clazz.cast(domain()));
case "Task":
return Optional.ofNullable(clazz.cast(task()));
case "Framework":
return Optional.ofNullable(clazz.cast(framework()));
case "FrameworkVersion":
return Optional.ofNullable(clazz.cast(frameworkVersion()));
case "PayloadConfig":
return Optional.ofNullable(clazz.cast(payloadConfig()));
case "NearestModelName":
return Optional.ofNullable(clazz.cast(nearestModelName()));
case "SupportedInstanceTypes":
return Optional.ofNullable(clazz.cast(supportedInstanceTypes()));
case "SupportedEndpointType":
return Optional.ofNullable(clazz.cast(supportedEndpointTypeAsString()));
case "DataInputConfig":
return Optional.ofNullable(clazz.cast(dataInputConfig()));
case "SupportedResponseMIMETypes":
return Optional.ofNullable(clazz.cast(supportedResponseMIMETypes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Domain", DOMAIN_FIELD);
map.put("Task", TASK_FIELD);
map.put("Framework", FRAMEWORK_FIELD);
map.put("FrameworkVersion", FRAMEWORK_VERSION_FIELD);
map.put("PayloadConfig", PAYLOAD_CONFIG_FIELD);
map.put("NearestModelName", NEAREST_MODEL_NAME_FIELD);
map.put("SupportedInstanceTypes", SUPPORTED_INSTANCE_TYPES_FIELD);
map.put("SupportedEndpointType", SUPPORTED_ENDPOINT_TYPE_FIELD);
map.put("DataInputConfig", DATA_INPUT_CONFIG_FIELD);
map.put("SupportedResponseMIMETypes", SUPPORTED_RESPONSE_MIME_TYPES_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function