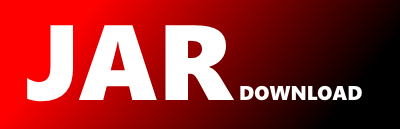
software.amazon.awssdk.services.sagemaker.model.ResourceConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the resources, including machine learning (ML) compute instances and ML storage volumes, to use for model
* training.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResourceConfig implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(ResourceConfig::instanceTypeAsString))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("InstanceCount").getter(getter(ResourceConfig::instanceCount)).setter(setter(Builder::instanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCount").build()).build();
private static final SdkField VOLUME_SIZE_IN_GB_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("VolumeSizeInGB").getter(getter(ResourceConfig::volumeSizeInGB)).setter(setter(Builder::volumeSizeInGB))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeSizeInGB").build()).build();
private static final SdkField VOLUME_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VolumeKmsKeyId").getter(getter(ResourceConfig::volumeKmsKeyId)).setter(setter(Builder::volumeKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeKmsKeyId").build()).build();
private static final SdkField KEEP_ALIVE_PERIOD_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("KeepAlivePeriodInSeconds")
.getter(getter(ResourceConfig::keepAlivePeriodInSeconds)).setter(setter(Builder::keepAlivePeriodInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeepAlivePeriodInSeconds").build())
.build();
private static final SdkField> INSTANCE_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InstanceGroups")
.getter(getter(ResourceConfig::instanceGroups))
.setter(setter(Builder::instanceGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InstanceGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TRAINING_PLAN_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TrainingPlanArn").getter(getter(ResourceConfig::trainingPlanArn))
.setter(setter(Builder::trainingPlanArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrainingPlanArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INSTANCE_TYPE_FIELD,
INSTANCE_COUNT_FIELD, VOLUME_SIZE_IN_GB_FIELD, VOLUME_KMS_KEY_ID_FIELD, KEEP_ALIVE_PERIOD_IN_SECONDS_FIELD,
INSTANCE_GROUPS_FIELD, TRAINING_PLAN_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String instanceType;
private final Integer instanceCount;
private final Integer volumeSizeInGB;
private final String volumeKmsKeyId;
private final Integer keepAlivePeriodInSeconds;
private final List instanceGroups;
private final String trainingPlanArn;
private ResourceConfig(BuilderImpl builder) {
this.instanceType = builder.instanceType;
this.instanceCount = builder.instanceCount;
this.volumeSizeInGB = builder.volumeSizeInGB;
this.volumeKmsKeyId = builder.volumeKmsKeyId;
this.keepAlivePeriodInSeconds = builder.keepAlivePeriodInSeconds;
this.instanceGroups = builder.instanceGroups;
this.trainingPlanArn = builder.trainingPlanArn;
}
/**
*
* The ML compute instance type.
*
*
*
* SageMaker Training on Amazon Elastic Compute Cloud (EC2) P4de instances is in preview release starting December
* 9th, 2022.
*
*
* Amazon EC2 P4de instances (currently in preview) are
* powered by 8 NVIDIA A100 GPUs with 80GB high-performance HBM2e GPU memory, which accelerate the speed of training
* ML models that need to be trained on large datasets of high-resolution data. In this preview release, Amazon
* SageMaker supports ML training jobs on P4de instances (ml.p4de.24xlarge
) to reduce model training
* time. The ml.p4de.24xlarge
instances are available in the following Amazon Web Services Regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
*
*
* To request quota limit increase and start using P4de instances, contact the SageMaker Training service team
* through your account team.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link TrainingInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #instanceTypeAsString}.
*
*
* @return The ML compute instance type.
*
* SageMaker Training on Amazon Elastic Compute Cloud (EC2) P4de instances is in preview release starting
* December 9th, 2022.
*
*
* Amazon EC2 P4de instances (currently in
* preview) are powered by 8 NVIDIA A100 GPUs with 80GB high-performance HBM2e GPU memory, which accelerate
* the speed of training ML models that need to be trained on large datasets of high-resolution data. In
* this preview release, Amazon SageMaker supports ML training jobs on P4de instances (
* ml.p4de.24xlarge
) to reduce model training time. The ml.p4de.24xlarge
instances
* are available in the following Amazon Web Services Regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
*
*
* To request quota limit increase and start using P4de instances, contact the SageMaker Training service
* team through your account team.
*
* @see TrainingInstanceType
*/
public final TrainingInstanceType instanceType() {
return TrainingInstanceType.fromValue(instanceType);
}
/**
*
* The ML compute instance type.
*
*
*
* SageMaker Training on Amazon Elastic Compute Cloud (EC2) P4de instances is in preview release starting December
* 9th, 2022.
*
*
* Amazon EC2 P4de instances (currently in preview) are
* powered by 8 NVIDIA A100 GPUs with 80GB high-performance HBM2e GPU memory, which accelerate the speed of training
* ML models that need to be trained on large datasets of high-resolution data. In this preview release, Amazon
* SageMaker supports ML training jobs on P4de instances (ml.p4de.24xlarge
) to reduce model training
* time. The ml.p4de.24xlarge
instances are available in the following Amazon Web Services Regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
*
*
* To request quota limit increase and start using P4de instances, contact the SageMaker Training service team
* through your account team.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link TrainingInstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #instanceTypeAsString}.
*
*
* @return The ML compute instance type.
*
* SageMaker Training on Amazon Elastic Compute Cloud (EC2) P4de instances is in preview release starting
* December 9th, 2022.
*
*
* Amazon EC2 P4de instances (currently in
* preview) are powered by 8 NVIDIA A100 GPUs with 80GB high-performance HBM2e GPU memory, which accelerate
* the speed of training ML models that need to be trained on large datasets of high-resolution data. In
* this preview release, Amazon SageMaker supports ML training jobs on P4de instances (
* ml.p4de.24xlarge
) to reduce model training time. The ml.p4de.24xlarge
instances
* are available in the following Amazon Web Services Regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
*
*
* To request quota limit increase and start using P4de instances, contact the SageMaker Training service
* team through your account team.
*
* @see TrainingInstanceType
*/
public final String instanceTypeAsString() {
return instanceType;
}
/**
*
* The number of ML compute instances to use. For distributed training, provide a value greater than 1.
*
*
* @return The number of ML compute instances to use. For distributed training, provide a value greater than 1.
*/
public final Integer instanceCount() {
return instanceCount;
}
/**
*
* The size of the ML storage volume that you want to provision.
*
*
* ML storage volumes store model artifacts and incremental states. Training algorithms might also use the ML
* storage volume for scratch space. If you want to store the training data in the ML storage volume, choose
* File
as the TrainingInputMode
in the algorithm specification.
*
*
* When using an ML instance with NVMe SSD
* volumes, SageMaker doesn't provision Amazon EBS General Purpose SSD (gp2) storage. Available storage is fixed
* to the NVMe-type instance's storage capacity. SageMaker configures storage paths for training datasets,
* checkpoints, model artifacts, and outputs to use the entire capacity of the instance storage. For example, ML
* instance families with the NVMe-type instance storage include ml.p4d
, ml.g4dn
, and
* ml.g5
.
*
*
* When using an ML instance with the EBS-only storage option and without instance storage, you must define the size
* of EBS volume through VolumeSizeInGB
in the ResourceConfig
API. For example, ML
* instance families that use EBS volumes include ml.c5
and ml.p2
.
*
*
* To look up instance types and their instance storage types and volumes, see Amazon EC2 Instance Types.
*
*
* To find the default local paths defined by the SageMaker training platform, see Amazon SageMaker Training Storage
* Folders for Training Datasets, Checkpoints, Model Artifacts, and Outputs.
*
*
* @return The size of the ML storage volume that you want to provision.
*
* ML storage volumes store model artifacts and incremental states. Training algorithms might also use the
* ML storage volume for scratch space. If you want to store the training data in the ML storage volume,
* choose File
as the TrainingInputMode
in the algorithm specification.
*
*
* When using an ML instance with NVMe
* SSD volumes, SageMaker doesn't provision Amazon EBS General Purpose SSD (gp2) storage. Available
* storage is fixed to the NVMe-type instance's storage capacity. SageMaker configures storage paths for
* training datasets, checkpoints, model artifacts, and outputs to use the entire capacity of the instance
* storage. For example, ML instance families with the NVMe-type instance storage include
* ml.p4d
, ml.g4dn
, and ml.g5
.
*
*
* When using an ML instance with the EBS-only storage option and without instance storage, you must define
* the size of EBS volume through VolumeSizeInGB
in the ResourceConfig
API. For
* example, ML instance families that use EBS volumes include ml.c5
and ml.p2
.
*
*
* To look up instance types and their instance storage types and volumes, see Amazon EC2 Instance Types.
*
*
* To find the default local paths defined by the SageMaker training platform, see Amazon SageMaker Training
* Storage Folders for Training Datasets, Checkpoints, Model Artifacts, and Outputs.
*/
public final Integer volumeSizeInGB() {
return volumeSizeInGB;
}
/**
*
* The Amazon Web Services KMS key that SageMaker uses to encrypt data on the storage volume attached to the ML
* compute instance(s) that run the training job.
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a VolumeKmsKeyId
when using an
* instance type with local storage.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* The VolumeKmsKeyId
can be in any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @return The Amazon Web Services KMS key that SageMaker uses to encrypt data on the storage volume attached to the
* ML compute instance(s) that run the training job.
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage
* volumes are encrypted using a hardware module on the instance. You can't request a
* VolumeKmsKeyId
when using an instance type with local storage.
*
*
* For a list of instance types that support local instance storage, see Instance Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* The VolumeKmsKeyId
can be in any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*/
public final String volumeKmsKeyId() {
return volumeKmsKeyId;
}
/**
*
* The duration of time in seconds to retain configured resources in a warm pool for subsequent training jobs.
*
*
* @return The duration of time in seconds to retain configured resources in a warm pool for subsequent training
* jobs.
*/
public final Integer keepAlivePeriodInSeconds() {
return keepAlivePeriodInSeconds;
}
/**
* For responses, this returns true if the service returned a value for the InstanceGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInstanceGroups() {
return instanceGroups != null && !(instanceGroups instanceof SdkAutoConstructList);
}
/**
*
* The configuration of a heterogeneous cluster in JSON format.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInstanceGroups} method.
*
*
* @return The configuration of a heterogeneous cluster in JSON format.
*/
public final List instanceGroups() {
return instanceGroups;
}
/**
*
* The Amazon Resource Name (ARN); of the training plan to use for this resource configuration.
*
*
* @return The Amazon Resource Name (ARN); of the training plan to use for this resource configuration.
*/
public final String trainingPlanArn() {
return trainingPlanArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(instanceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(instanceCount());
hashCode = 31 * hashCode + Objects.hashCode(volumeSizeInGB());
hashCode = 31 * hashCode + Objects.hashCode(volumeKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(keepAlivePeriodInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasInstanceGroups() ? instanceGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(trainingPlanArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResourceConfig)) {
return false;
}
ResourceConfig other = (ResourceConfig) obj;
return Objects.equals(instanceTypeAsString(), other.instanceTypeAsString())
&& Objects.equals(instanceCount(), other.instanceCount())
&& Objects.equals(volumeSizeInGB(), other.volumeSizeInGB())
&& Objects.equals(volumeKmsKeyId(), other.volumeKmsKeyId())
&& Objects.equals(keepAlivePeriodInSeconds(), other.keepAlivePeriodInSeconds())
&& hasInstanceGroups() == other.hasInstanceGroups() && Objects.equals(instanceGroups(), other.instanceGroups())
&& Objects.equals(trainingPlanArn(), other.trainingPlanArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ResourceConfig").add("InstanceType", instanceTypeAsString())
.add("InstanceCount", instanceCount()).add("VolumeSizeInGB", volumeSizeInGB())
.add("VolumeKmsKeyId", volumeKmsKeyId()).add("KeepAlivePeriodInSeconds", keepAlivePeriodInSeconds())
.add("InstanceGroups", hasInstanceGroups() ? instanceGroups() : null).add("TrainingPlanArn", trainingPlanArn())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceTypeAsString()));
case "InstanceCount":
return Optional.ofNullable(clazz.cast(instanceCount()));
case "VolumeSizeInGB":
return Optional.ofNullable(clazz.cast(volumeSizeInGB()));
case "VolumeKmsKeyId":
return Optional.ofNullable(clazz.cast(volumeKmsKeyId()));
case "KeepAlivePeriodInSeconds":
return Optional.ofNullable(clazz.cast(keepAlivePeriodInSeconds()));
case "InstanceGroups":
return Optional.ofNullable(clazz.cast(instanceGroups()));
case "TrainingPlanArn":
return Optional.ofNullable(clazz.cast(trainingPlanArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("InstanceType", INSTANCE_TYPE_FIELD);
map.put("InstanceCount", INSTANCE_COUNT_FIELD);
map.put("VolumeSizeInGB", VOLUME_SIZE_IN_GB_FIELD);
map.put("VolumeKmsKeyId", VOLUME_KMS_KEY_ID_FIELD);
map.put("KeepAlivePeriodInSeconds", KEEP_ALIVE_PERIOD_IN_SECONDS_FIELD);
map.put("InstanceGroups", INSTANCE_GROUPS_FIELD);
map.put("TrainingPlanArn", TRAINING_PLAN_ARN_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function