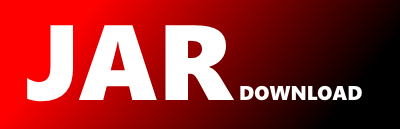
software.amazon.awssdk.services.sagemaker.model.TrainingJobStatusCounters Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The numbers of training jobs launched by a hyperparameter tuning job, categorized by status.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TrainingJobStatusCounters implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField COMPLETED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Completed").getter(getter(TrainingJobStatusCounters::completed)).setter(setter(Builder::completed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Completed").build()).build();
private static final SdkField IN_PROGRESS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("InProgress").getter(getter(TrainingJobStatusCounters::inProgress)).setter(setter(Builder::inProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InProgress").build()).build();
private static final SdkField RETRYABLE_ERROR_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RetryableError").getter(getter(TrainingJobStatusCounters::retryableError))
.setter(setter(Builder::retryableError))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetryableError").build()).build();
private static final SdkField NON_RETRYABLE_ERROR_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NonRetryableError").getter(getter(TrainingJobStatusCounters::nonRetryableError))
.setter(setter(Builder::nonRetryableError))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NonRetryableError").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Stopped").getter(getter(TrainingJobStatusCounters::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Stopped").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPLETED_FIELD,
IN_PROGRESS_FIELD, RETRYABLE_ERROR_FIELD, NON_RETRYABLE_ERROR_FIELD, STOPPED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Integer completed;
private final Integer inProgress;
private final Integer retryableError;
private final Integer nonRetryableError;
private final Integer stopped;
private TrainingJobStatusCounters(BuilderImpl builder) {
this.completed = builder.completed;
this.inProgress = builder.inProgress;
this.retryableError = builder.retryableError;
this.nonRetryableError = builder.nonRetryableError;
this.stopped = builder.stopped;
}
/**
*
* The number of completed training jobs launched by the hyperparameter tuning job.
*
*
* @return The number of completed training jobs launched by the hyperparameter tuning job.
*/
public final Integer completed() {
return completed;
}
/**
*
* The number of in-progress training jobs launched by a hyperparameter tuning job.
*
*
* @return The number of in-progress training jobs launched by a hyperparameter tuning job.
*/
public final Integer inProgress() {
return inProgress;
}
/**
*
* The number of training jobs that failed, but can be retried. A failed training job can be retried only if it
* failed because an internal service error occurred.
*
*
* @return The number of training jobs that failed, but can be retried. A failed training job can be retried only if
* it failed because an internal service error occurred.
*/
public final Integer retryableError() {
return retryableError;
}
/**
*
* The number of training jobs that failed and can't be retried. A failed training job can't be retried if it failed
* because a client error occurred.
*
*
* @return The number of training jobs that failed and can't be retried. A failed training job can't be retried if
* it failed because a client error occurred.
*/
public final Integer nonRetryableError() {
return nonRetryableError;
}
/**
*
* The number of training jobs launched by a hyperparameter tuning job that were manually stopped.
*
*
* @return The number of training jobs launched by a hyperparameter tuning job that were manually stopped.
*/
public final Integer stopped() {
return stopped;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(completed());
hashCode = 31 * hashCode + Objects.hashCode(inProgress());
hashCode = 31 * hashCode + Objects.hashCode(retryableError());
hashCode = 31 * hashCode + Objects.hashCode(nonRetryableError());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TrainingJobStatusCounters)) {
return false;
}
TrainingJobStatusCounters other = (TrainingJobStatusCounters) obj;
return Objects.equals(completed(), other.completed()) && Objects.equals(inProgress(), other.inProgress())
&& Objects.equals(retryableError(), other.retryableError())
&& Objects.equals(nonRetryableError(), other.nonRetryableError()) && Objects.equals(stopped(), other.stopped());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TrainingJobStatusCounters").add("Completed", completed()).add("InProgress", inProgress())
.add("RetryableError", retryableError()).add("NonRetryableError", nonRetryableError()).add("Stopped", stopped())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Completed":
return Optional.ofNullable(clazz.cast(completed()));
case "InProgress":
return Optional.ofNullable(clazz.cast(inProgress()));
case "RetryableError":
return Optional.ofNullable(clazz.cast(retryableError()));
case "NonRetryableError":
return Optional.ofNullable(clazz.cast(nonRetryableError()));
case "Stopped":
return Optional.ofNullable(clazz.cast(stopped()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Completed", COMPLETED_FIELD);
map.put("InProgress", IN_PROGRESS_FIELD);
map.put("RetryableError", RETRYABLE_ERROR_FIELD);
map.put("NonRetryableError", NON_RETRYABLE_ERROR_FIELD);
map.put("Stopped", STOPPED_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function