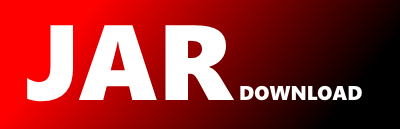
software.amazon.awssdk.services.sagemaker.model.UpdateImageVersionRequest Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateImageVersionRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField IMAGE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageName").getter(getter(UpdateImageVersionRequest::imageName)).setter(setter(Builder::imageName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageName").build()).build();
private static final SdkField ALIAS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Alias")
.getter(getter(UpdateImageVersionRequest::alias)).setter(setter(Builder::alias))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Alias").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Version").getter(getter(UpdateImageVersionRequest::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Version").build()).build();
private static final SdkField> ALIASES_TO_ADD_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AliasesToAdd")
.getter(getter(UpdateImageVersionRequest::aliasesToAdd))
.setter(setter(Builder::aliasesToAdd))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AliasesToAdd").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ALIASES_TO_DELETE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AliasesToDelete")
.getter(getter(UpdateImageVersionRequest::aliasesToDelete))
.setter(setter(Builder::aliasesToDelete))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AliasesToDelete").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VENDOR_GUIDANCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VendorGuidance").getter(getter(UpdateImageVersionRequest::vendorGuidanceAsString))
.setter(setter(Builder::vendorGuidance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VendorGuidance").build()).build();
private static final SdkField JOB_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobType").getter(getter(UpdateImageVersionRequest::jobTypeAsString)).setter(setter(Builder::jobType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobType").build()).build();
private static final SdkField ML_FRAMEWORK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MLFramework").getter(getter(UpdateImageVersionRequest::mlFramework))
.setter(setter(Builder::mlFramework))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MLFramework").build()).build();
private static final SdkField PROGRAMMING_LANG_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProgrammingLang").getter(getter(UpdateImageVersionRequest::programmingLang))
.setter(setter(Builder::programmingLang))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgrammingLang").build()).build();
private static final SdkField PROCESSOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Processor").getter(getter(UpdateImageVersionRequest::processorAsString))
.setter(setter(Builder::processor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Processor").build()).build();
private static final SdkField HOROVOD_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Horovod").getter(getter(UpdateImageVersionRequest::horovod)).setter(setter(Builder::horovod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Horovod").build()).build();
private static final SdkField RELEASE_NOTES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReleaseNotes").getter(getter(UpdateImageVersionRequest::releaseNotes))
.setter(setter(Builder::releaseNotes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReleaseNotes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IMAGE_NAME_FIELD, ALIAS_FIELD,
VERSION_FIELD, ALIASES_TO_ADD_FIELD, ALIASES_TO_DELETE_FIELD, VENDOR_GUIDANCE_FIELD, JOB_TYPE_FIELD,
ML_FRAMEWORK_FIELD, PROGRAMMING_LANG_FIELD, PROCESSOR_FIELD, HOROVOD_FIELD, RELEASE_NOTES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String imageName;
private final String alias;
private final Integer version;
private final List aliasesToAdd;
private final List aliasesToDelete;
private final String vendorGuidance;
private final String jobType;
private final String mlFramework;
private final String programmingLang;
private final String processor;
private final Boolean horovod;
private final String releaseNotes;
private UpdateImageVersionRequest(BuilderImpl builder) {
super(builder);
this.imageName = builder.imageName;
this.alias = builder.alias;
this.version = builder.version;
this.aliasesToAdd = builder.aliasesToAdd;
this.aliasesToDelete = builder.aliasesToDelete;
this.vendorGuidance = builder.vendorGuidance;
this.jobType = builder.jobType;
this.mlFramework = builder.mlFramework;
this.programmingLang = builder.programmingLang;
this.processor = builder.processor;
this.horovod = builder.horovod;
this.releaseNotes = builder.releaseNotes;
}
/**
*
* The name of the image.
*
*
* @return The name of the image.
*/
public final String imageName() {
return imageName;
}
/**
*
* The alias of the image version.
*
*
* @return The alias of the image version.
*/
public final String alias() {
return alias;
}
/**
*
* The version of the image.
*
*
* @return The version of the image.
*/
public final Integer version() {
return version;
}
/**
* For responses, this returns true if the service returned a value for the AliasesToAdd property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAliasesToAdd() {
return aliasesToAdd != null && !(aliasesToAdd instanceof SdkAutoConstructList);
}
/**
*
* A list of aliases to add.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAliasesToAdd} method.
*
*
* @return A list of aliases to add.
*/
public final List aliasesToAdd() {
return aliasesToAdd;
}
/**
* For responses, this returns true if the service returned a value for the AliasesToDelete property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAliasesToDelete() {
return aliasesToDelete != null && !(aliasesToDelete instanceof SdkAutoConstructList);
}
/**
*
* A list of aliases to delete.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAliasesToDelete} method.
*
*
* @return A list of aliases to delete.
*/
public final List aliasesToDelete() {
return aliasesToDelete;
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vendorGuidance}
* will return {@link VendorGuidance#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #vendorGuidanceAsString}.
*
*
* @return The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @see VendorGuidance
*/
public final VendorGuidance vendorGuidance() {
return VendorGuidance.fromValue(vendorGuidance);
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vendorGuidance}
* will return {@link VendorGuidance#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #vendorGuidanceAsString}.
*
*
* @return The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @see VendorGuidance
*/
public final String vendorGuidanceAsString() {
return vendorGuidance;
}
/**
*
* Indicates SageMaker AI job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker AI training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker AI inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker AI notebook kernels.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link JobType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobTypeAsString}.
*
*
* @return Indicates SageMaker AI job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker AI training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker AI inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker AI notebook kernels.
*
*
* @see JobType
*/
public final JobType jobType() {
return JobType.fromValue(jobType);
}
/**
*
* Indicates SageMaker AI job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker AI training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker AI inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker AI notebook kernels.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #jobType} will
* return {@link JobType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #jobTypeAsString}.
*
*
* @return Indicates SageMaker AI job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker AI training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker AI inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker AI notebook kernels.
*
*
* @see JobType
*/
public final String jobTypeAsString() {
return jobType;
}
/**
*
* The machine learning framework vended in the image version.
*
*
* @return The machine learning framework vended in the image version.
*/
public final String mlFramework() {
return mlFramework;
}
/**
*
* The supported programming language and its version.
*
*
* @return The supported programming language and its version.
*/
public final String programmingLang() {
return programmingLang;
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #processor} will
* return {@link Processor#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #processorAsString}.
*
*
* @return Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @see Processor
*/
public final Processor processor() {
return Processor.fromValue(processor);
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #processor} will
* return {@link Processor#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #processorAsString}.
*
*
* @return Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @see Processor
*/
public final String processorAsString() {
return processor;
}
/**
*
* Indicates Horovod compatibility.
*
*
* @return Indicates Horovod compatibility.
*/
public final Boolean horovod() {
return horovod;
}
/**
*
* The maintainer description of the image version.
*
*
* @return The maintainer description of the image version.
*/
public final String releaseNotes() {
return releaseNotes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(imageName());
hashCode = 31 * hashCode + Objects.hashCode(alias());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(hasAliasesToAdd() ? aliasesToAdd() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAliasesToDelete() ? aliasesToDelete() : null);
hashCode = 31 * hashCode + Objects.hashCode(vendorGuidanceAsString());
hashCode = 31 * hashCode + Objects.hashCode(jobTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mlFramework());
hashCode = 31 * hashCode + Objects.hashCode(programmingLang());
hashCode = 31 * hashCode + Objects.hashCode(processorAsString());
hashCode = 31 * hashCode + Objects.hashCode(horovod());
hashCode = 31 * hashCode + Objects.hashCode(releaseNotes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateImageVersionRequest)) {
return false;
}
UpdateImageVersionRequest other = (UpdateImageVersionRequest) obj;
return Objects.equals(imageName(), other.imageName()) && Objects.equals(alias(), other.alias())
&& Objects.equals(version(), other.version()) && hasAliasesToAdd() == other.hasAliasesToAdd()
&& Objects.equals(aliasesToAdd(), other.aliasesToAdd()) && hasAliasesToDelete() == other.hasAliasesToDelete()
&& Objects.equals(aliasesToDelete(), other.aliasesToDelete())
&& Objects.equals(vendorGuidanceAsString(), other.vendorGuidanceAsString())
&& Objects.equals(jobTypeAsString(), other.jobTypeAsString())
&& Objects.equals(mlFramework(), other.mlFramework())
&& Objects.equals(programmingLang(), other.programmingLang())
&& Objects.equals(processorAsString(), other.processorAsString()) && Objects.equals(horovod(), other.horovod())
&& Objects.equals(releaseNotes(), other.releaseNotes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateImageVersionRequest").add("ImageName", imageName()).add("Alias", alias())
.add("Version", version()).add("AliasesToAdd", hasAliasesToAdd() ? aliasesToAdd() : null)
.add("AliasesToDelete", hasAliasesToDelete() ? aliasesToDelete() : null)
.add("VendorGuidance", vendorGuidanceAsString()).add("JobType", jobTypeAsString())
.add("MLFramework", mlFramework()).add("ProgrammingLang", programmingLang())
.add("Processor", processorAsString()).add("Horovod", horovod()).add("ReleaseNotes", releaseNotes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ImageName":
return Optional.ofNullable(clazz.cast(imageName()));
case "Alias":
return Optional.ofNullable(clazz.cast(alias()));
case "Version":
return Optional.ofNullable(clazz.cast(version()));
case "AliasesToAdd":
return Optional.ofNullable(clazz.cast(aliasesToAdd()));
case "AliasesToDelete":
return Optional.ofNullable(clazz.cast(aliasesToDelete()));
case "VendorGuidance":
return Optional.ofNullable(clazz.cast(vendorGuidanceAsString()));
case "JobType":
return Optional.ofNullable(clazz.cast(jobTypeAsString()));
case "MLFramework":
return Optional.ofNullable(clazz.cast(mlFramework()));
case "ProgrammingLang":
return Optional.ofNullable(clazz.cast(programmingLang()));
case "Processor":
return Optional.ofNullable(clazz.cast(processorAsString()));
case "Horovod":
return Optional.ofNullable(clazz.cast(horovod()));
case "ReleaseNotes":
return Optional.ofNullable(clazz.cast(releaseNotes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ImageName", IMAGE_NAME_FIELD);
map.put("Alias", ALIAS_FIELD);
map.put("Version", VERSION_FIELD);
map.put("AliasesToAdd", ALIASES_TO_ADD_FIELD);
map.put("AliasesToDelete", ALIASES_TO_DELETE_FIELD);
map.put("VendorGuidance", VENDOR_GUIDANCE_FIELD);
map.put("JobType", JOB_TYPE_FIELD);
map.put("MLFramework", ML_FRAMEWORK_FIELD);
map.put("ProgrammingLang", PROGRAMMING_LANG_FIELD);
map.put("Processor", PROCESSOR_FIELD);
map.put("Horovod", HOROVOD_FIELD);
map.put("ReleaseNotes", RELEASE_NOTES_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function