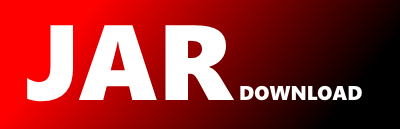
software.amazon.awssdk.services.sagemaker.model.ThroughputConfig Maven / Gradle / Ivy
Show all versions of sagemaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Used to set feature group throughput configuration. There are two modes: ON_DEMAND
and
* PROVISIONED
. With on-demand mode, you are charged for data reads and writes that your application
* performs on your feature group. You do not need to specify read and write throughput because Feature Store
* accommodates your workloads as they ramp up and down. You can switch a feature group to on-demand only once in a 24
* hour period. With provisioned throughput mode, you specify the read and write capacity per second that you expect
* your application to require, and you are billed based on those limits. Exceeding provisioned throughput will result
* in your requests being throttled.
*
*
* Note: PROVISIONED
throughput mode is supported only for feature groups that are offline-only, or use the
* Standard
tier online store.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ThroughputConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField THROUGHPUT_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ThroughputMode").getter(getter(ThroughputConfig::throughputModeAsString))
.setter(setter(Builder::throughputMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ThroughputMode").build()).build();
private static final SdkField PROVISIONED_READ_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ProvisionedReadCapacityUnits")
.getter(getter(ThroughputConfig::provisionedReadCapacityUnits))
.setter(setter(Builder::provisionedReadCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProvisionedReadCapacityUnits")
.build()).build();
private static final SdkField PROVISIONED_WRITE_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ProvisionedWriteCapacityUnits")
.getter(getter(ThroughputConfig::provisionedWriteCapacityUnits))
.setter(setter(Builder::provisionedWriteCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProvisionedWriteCapacityUnits")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(THROUGHPUT_MODE_FIELD,
PROVISIONED_READ_CAPACITY_UNITS_FIELD, PROVISIONED_WRITE_CAPACITY_UNITS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String throughputMode;
private final Integer provisionedReadCapacityUnits;
private final Integer provisionedWriteCapacityUnits;
private ThroughputConfig(BuilderImpl builder) {
this.throughputMode = builder.throughputMode;
this.provisionedReadCapacityUnits = builder.provisionedReadCapacityUnits;
this.provisionedWriteCapacityUnits = builder.provisionedWriteCapacityUnits;
}
/**
*
* The mode used for your feature group throughput: ON_DEMAND
or PROVISIONED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #throughputMode}
* will return {@link ThroughputMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #throughputModeAsString}.
*
*
* @return The mode used for your feature group throughput: ON_DEMAND
or PROVISIONED
.
* @see ThroughputMode
*/
public final ThroughputMode throughputMode() {
return ThroughputMode.fromValue(throughputMode);
}
/**
*
* The mode used for your feature group throughput: ON_DEMAND
or PROVISIONED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #throughputMode}
* will return {@link ThroughputMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #throughputModeAsString}.
*
*
* @return The mode used for your feature group throughput: ON_DEMAND
or PROVISIONED
.
* @see ThroughputMode
*/
public final String throughputModeAsString() {
return throughputMode;
}
/**
*
* For provisioned feature groups with online store enabled, this indicates the read throughput you are billed for
* and can consume without throttling.
*
*
* This field is not applicable for on-demand feature groups.
*
*
* @return For provisioned feature groups with online store enabled, this indicates the read throughput you are
* billed for and can consume without throttling.
*
* This field is not applicable for on-demand feature groups.
*/
public final Integer provisionedReadCapacityUnits() {
return provisionedReadCapacityUnits;
}
/**
*
* For provisioned feature groups, this indicates the write throughput you are billed for and can consume without
* throttling.
*
*
* This field is not applicable for on-demand feature groups.
*
*
* @return For provisioned feature groups, this indicates the write throughput you are billed for and can consume
* without throttling.
*
* This field is not applicable for on-demand feature groups.
*/
public final Integer provisionedWriteCapacityUnits() {
return provisionedWriteCapacityUnits;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(throughputModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(provisionedReadCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(provisionedWriteCapacityUnits());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ThroughputConfig)) {
return false;
}
ThroughputConfig other = (ThroughputConfig) obj;
return Objects.equals(throughputModeAsString(), other.throughputModeAsString())
&& Objects.equals(provisionedReadCapacityUnits(), other.provisionedReadCapacityUnits())
&& Objects.equals(provisionedWriteCapacityUnits(), other.provisionedWriteCapacityUnits());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ThroughputConfig").add("ThroughputMode", throughputModeAsString())
.add("ProvisionedReadCapacityUnits", provisionedReadCapacityUnits())
.add("ProvisionedWriteCapacityUnits", provisionedWriteCapacityUnits()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ThroughputMode":
return Optional.ofNullable(clazz.cast(throughputModeAsString()));
case "ProvisionedReadCapacityUnits":
return Optional.ofNullable(clazz.cast(provisionedReadCapacityUnits()));
case "ProvisionedWriteCapacityUnits":
return Optional.ofNullable(clazz.cast(provisionedWriteCapacityUnits()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ThroughputMode", THROUGHPUT_MODE_FIELD);
map.put("ProvisionedReadCapacityUnits", PROVISIONED_READ_CAPACITY_UNITS_FIELD);
map.put("ProvisionedWriteCapacityUnits", PROVISIONED_WRITE_CAPACITY_UNITS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* This field is not applicable for on-demand feature groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder provisionedReadCapacityUnits(Integer provisionedReadCapacityUnits);
/**
*
* For provisioned feature groups, this indicates the write throughput you are billed for and can consume
* without throttling.
*
*
* This field is not applicable for on-demand feature groups.
*
*
* @param provisionedWriteCapacityUnits
* For provisioned feature groups, this indicates the write throughput you are billed for and can consume
* without throttling.
*
* This field is not applicable for on-demand feature groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder provisionedWriteCapacityUnits(Integer provisionedWriteCapacityUnits);
}
static final class BuilderImpl implements Builder {
private String throughputMode;
private Integer provisionedReadCapacityUnits;
private Integer provisionedWriteCapacityUnits;
private BuilderImpl() {
}
private BuilderImpl(ThroughputConfig model) {
throughputMode(model.throughputMode);
provisionedReadCapacityUnits(model.provisionedReadCapacityUnits);
provisionedWriteCapacityUnits(model.provisionedWriteCapacityUnits);
}
public final String getThroughputMode() {
return throughputMode;
}
public final void setThroughputMode(String throughputMode) {
this.throughputMode = throughputMode;
}
@Override
public final Builder throughputMode(String throughputMode) {
this.throughputMode = throughputMode;
return this;
}
@Override
public final Builder throughputMode(ThroughputMode throughputMode) {
this.throughputMode(throughputMode == null ? null : throughputMode.toString());
return this;
}
public final Integer getProvisionedReadCapacityUnits() {
return provisionedReadCapacityUnits;
}
public final void setProvisionedReadCapacityUnits(Integer provisionedReadCapacityUnits) {
this.provisionedReadCapacityUnits = provisionedReadCapacityUnits;
}
@Override
public final Builder provisionedReadCapacityUnits(Integer provisionedReadCapacityUnits) {
this.provisionedReadCapacityUnits = provisionedReadCapacityUnits;
return this;
}
public final Integer getProvisionedWriteCapacityUnits() {
return provisionedWriteCapacityUnits;
}
public final void setProvisionedWriteCapacityUnits(Integer provisionedWriteCapacityUnits) {
this.provisionedWriteCapacityUnits = provisionedWriteCapacityUnits;
}
@Override
public final Builder provisionedWriteCapacityUnits(Integer provisionedWriteCapacityUnits) {
this.provisionedWriteCapacityUnits = provisionedWriteCapacityUnits;
return this;
}
@Override
public ThroughputConfig build() {
return new ThroughputConfig(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}