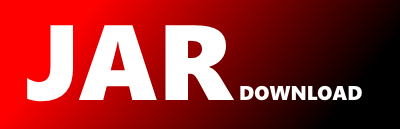
software.amazon.awssdk.services.sagemaker.model.UpdateWorkforceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemaker Show documentation
Show all versions of sagemaker Show documentation
The AWS Java SDK for Amazon SageMaker module holds the client classes that are used for communicating
with Amazon SageMaker Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemaker.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateWorkforceRequest extends SageMakerRequest implements
ToCopyableBuilder {
private static final SdkField WORKFORCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkforceName").getter(getter(UpdateWorkforceRequest::workforceName))
.setter(setter(Builder::workforceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkforceName").build()).build();
private static final SdkField SOURCE_IP_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SourceIpConfig")
.getter(getter(UpdateWorkforceRequest::sourceIpConfig)).setter(setter(Builder::sourceIpConfig))
.constructor(SourceIpConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceIpConfig").build()).build();
private static final SdkField OIDC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("OidcConfig").getter(getter(UpdateWorkforceRequest::oidcConfig)).setter(setter(Builder::oidcConfig))
.constructor(OidcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OidcConfig").build()).build();
private static final SdkField WORKFORCE_VPC_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("WorkforceVpcConfig")
.getter(getter(UpdateWorkforceRequest::workforceVpcConfig)).setter(setter(Builder::workforceVpcConfig))
.constructor(WorkforceVpcConfigRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkforceVpcConfig").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKFORCE_NAME_FIELD,
SOURCE_IP_CONFIG_FIELD, OIDC_CONFIG_FIELD, WORKFORCE_VPC_CONFIG_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String workforceName;
private final SourceIpConfig sourceIpConfig;
private final OidcConfig oidcConfig;
private final WorkforceVpcConfigRequest workforceVpcConfig;
private UpdateWorkforceRequest(BuilderImpl builder) {
super(builder);
this.workforceName = builder.workforceName;
this.sourceIpConfig = builder.sourceIpConfig;
this.oidcConfig = builder.oidcConfig;
this.workforceVpcConfig = builder.workforceVpcConfig;
}
/**
*
* The name of the private workforce that you want to update. You can find your workforce name by using the ListWorkforces
* operation.
*
*
* @return The name of the private workforce that you want to update. You can find your workforce name by using the
* ListWorkforces
* operation.
*/
public final String workforceName() {
return workforceName;
}
/**
*
* A list of one to ten worker IP address ranges (CIDRs) that can be used to access
* tasks assigned to this workforce.
*
*
* Maximum: Ten CIDR values
*
*
* @return A list of one to ten worker IP address ranges (CIDRs) that can be used to
* access tasks assigned to this workforce.
*
* Maximum: Ten CIDR values
*/
public final SourceIpConfig sourceIpConfig() {
return sourceIpConfig;
}
/**
*
* Use this parameter to update your OIDC Identity Provider (IdP) configuration for a workforce made using your own
* IdP.
*
*
* @return Use this parameter to update your OIDC Identity Provider (IdP) configuration for a workforce made using
* your own IdP.
*/
public final OidcConfig oidcConfig() {
return oidcConfig;
}
/**
*
* Use this parameter to update your VPC configuration for a workforce.
*
*
* @return Use this parameter to update your VPC configuration for a workforce.
*/
public final WorkforceVpcConfigRequest workforceVpcConfig() {
return workforceVpcConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(workforceName());
hashCode = 31 * hashCode + Objects.hashCode(sourceIpConfig());
hashCode = 31 * hashCode + Objects.hashCode(oidcConfig());
hashCode = 31 * hashCode + Objects.hashCode(workforceVpcConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateWorkforceRequest)) {
return false;
}
UpdateWorkforceRequest other = (UpdateWorkforceRequest) obj;
return Objects.equals(workforceName(), other.workforceName()) && Objects.equals(sourceIpConfig(), other.sourceIpConfig())
&& Objects.equals(oidcConfig(), other.oidcConfig())
&& Objects.equals(workforceVpcConfig(), other.workforceVpcConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateWorkforceRequest").add("WorkforceName", workforceName())
.add("SourceIpConfig", sourceIpConfig()).add("OidcConfig", oidcConfig())
.add("WorkforceVpcConfig", workforceVpcConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "WorkforceName":
return Optional.ofNullable(clazz.cast(workforceName()));
case "SourceIpConfig":
return Optional.ofNullable(clazz.cast(sourceIpConfig()));
case "OidcConfig":
return Optional.ofNullable(clazz.cast(oidcConfig()));
case "WorkforceVpcConfig":
return Optional.ofNullable(clazz.cast(workforceVpcConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("WorkforceName", WORKFORCE_NAME_FIELD);
map.put("SourceIpConfig", SOURCE_IP_CONFIG_FIELD);
map.put("OidcConfig", OIDC_CONFIG_FIELD);
map.put("WorkforceVpcConfig", WORKFORCE_VPC_CONFIG_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy