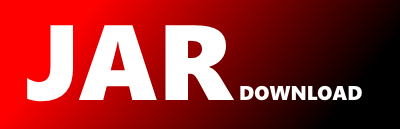
software.amazon.awssdk.services.sagemakeredge.SagemakerEdgeAsyncClient Maven / Gradle / Ivy
Show all versions of sagemakeredge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakeredge;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeploymentsRequest;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeploymentsResponse;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeviceRegistrationRequest;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeviceRegistrationResponse;
import software.amazon.awssdk.services.sagemakeredge.model.SendHeartbeatRequest;
import software.amazon.awssdk.services.sagemakeredge.model.SendHeartbeatResponse;
/**
* Service client for accessing Amazon Sagemaker Edge Manager asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* SageMaker Edge Manager dataplane service for communicating with active agents.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SagemakerEdgeAsyncClient extends AwsClient {
String SERVICE_NAME = "sagemaker";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "edge.sagemaker";
/**
*
* Use to get the active deployments from a device.
*
*
* @param getDeploymentsRequest
* @return A Java Future containing the result of the GetDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.GetDeployments
* @see AWS
* API Documentation
*/
default CompletableFuture getDeployments(GetDeploymentsRequest getDeploymentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use to get the active deployments from a device.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeploymentsRequest.Builder} avoiding the need to
* create one manually via {@link GetDeploymentsRequest#builder()}
*
*
* @param getDeploymentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakeredge.model.GetDeploymentsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.GetDeployments
* @see AWS
* API Documentation
*/
default CompletableFuture getDeployments(Consumer getDeploymentsRequest) {
return getDeployments(GetDeploymentsRequest.builder().applyMutation(getDeploymentsRequest).build());
}
/**
*
* Use to check if a device is registered with SageMaker Edge Manager.
*
*
* @param getDeviceRegistrationRequest
* @return A Java Future containing the result of the GetDeviceRegistration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.GetDeviceRegistration
* @see AWS API Documentation
*/
default CompletableFuture getDeviceRegistration(
GetDeviceRegistrationRequest getDeviceRegistrationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use to check if a device is registered with SageMaker Edge Manager.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeviceRegistrationRequest.Builder} avoiding the
* need to create one manually via {@link GetDeviceRegistrationRequest#builder()}
*
*
* @param getDeviceRegistrationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakeredge.model.GetDeviceRegistrationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetDeviceRegistration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.GetDeviceRegistration
* @see AWS API Documentation
*/
default CompletableFuture getDeviceRegistration(
Consumer getDeviceRegistrationRequest) {
return getDeviceRegistration(GetDeviceRegistrationRequest.builder().applyMutation(getDeviceRegistrationRequest).build());
}
/**
*
* Use to get the current status of devices registered on SageMaker Edge Manager.
*
*
* @param sendHeartbeatRequest
* @return A Java Future containing the result of the SendHeartbeat operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.SendHeartbeat
* @see AWS
* API Documentation
*/
default CompletableFuture sendHeartbeat(SendHeartbeatRequest sendHeartbeatRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use to get the current status of devices registered on SageMaker Edge Manager.
*
*
*
* This is a convenience which creates an instance of the {@link SendHeartbeatRequest.Builder} avoiding the need to
* create one manually via {@link SendHeartbeatRequest#builder()}
*
*
* @param sendHeartbeatRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakeredge.model.SendHeartbeatRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SendHeartbeat operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceException An internal failure occurred. Try your request again. If the problem
* persists, contact Amazon Web Services customer support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SagemakerEdgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SagemakerEdgeAsyncClient.SendHeartbeat
* @see AWS
* API Documentation
*/
default CompletableFuture sendHeartbeat(Consumer sendHeartbeatRequest) {
return sendHeartbeat(SendHeartbeatRequest.builder().applyMutation(sendHeartbeatRequest).build());
}
@Override
default SagemakerEdgeServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link SagemakerEdgeAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SagemakerEdgeAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SagemakerEdgeAsyncClient}.
*/
static SagemakerEdgeAsyncClientBuilder builder() {
return new DefaultSagemakerEdgeAsyncClientBuilder();
}
}