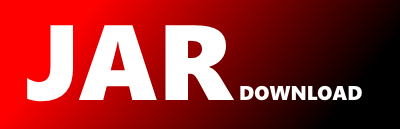
software.amazon.awssdk.services.sagemakeredge.model.DeploymentModel Maven / Gradle / Ivy
Show all versions of sagemakeredge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakeredge.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentModel implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField MODEL_HANDLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelHandle").getter(getter(DeploymentModel::modelHandle)).setter(setter(Builder::modelHandle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelHandle").build()).build();
private static final SdkField MODEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelName").getter(getter(DeploymentModel::modelName)).setter(setter(Builder::modelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelName").build()).build();
private static final SdkField MODEL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelVersion").getter(getter(DeploymentModel::modelVersion)).setter(setter(Builder::modelVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelVersion").build()).build();
private static final SdkField DESIRED_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DesiredState").getter(getter(DeploymentModel::desiredStateAsString))
.setter(setter(Builder::desiredState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DesiredState").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(DeploymentModel::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DeploymentModel::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusReason").getter(getter(DeploymentModel::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusReason").build()).build();
private static final SdkField ROLLBACK_FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RollbackFailureReason").getter(getter(DeploymentModel::rollbackFailureReason))
.setter(setter(Builder::rollbackFailureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RollbackFailureReason").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MODEL_HANDLE_FIELD,
MODEL_NAME_FIELD, MODEL_VERSION_FIELD, DESIRED_STATE_FIELD, STATE_FIELD, STATUS_FIELD, STATUS_REASON_FIELD,
ROLLBACK_FAILURE_REASON_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ModelHandle", MODEL_HANDLE_FIELD);
put("ModelName", MODEL_NAME_FIELD);
put("ModelVersion", MODEL_VERSION_FIELD);
put("DesiredState", DESIRED_STATE_FIELD);
put("State", STATE_FIELD);
put("Status", STATUS_FIELD);
put("StatusReason", STATUS_REASON_FIELD);
put("RollbackFailureReason", ROLLBACK_FAILURE_REASON_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String modelHandle;
private final String modelName;
private final String modelVersion;
private final String desiredState;
private final String state;
private final String status;
private final String statusReason;
private final String rollbackFailureReason;
private DeploymentModel(BuilderImpl builder) {
this.modelHandle = builder.modelHandle;
this.modelName = builder.modelName;
this.modelVersion = builder.modelVersion;
this.desiredState = builder.desiredState;
this.state = builder.state;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.rollbackFailureReason = builder.rollbackFailureReason;
}
/**
*
* The unique handle of the model.
*
*
* @return The unique handle of the model.
*/
public final String modelHandle() {
return modelHandle;
}
/**
*
* The name of the model.
*
*
* @return The name of the model.
*/
public final String modelName() {
return modelName;
}
/**
*
* The version of the model.
*
*
* @return The version of the model.
*/
public final String modelVersion() {
return modelVersion;
}
/**
*
* The desired state of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #desiredState} will
* return {@link ModelState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #desiredStateAsString}.
*
*
* @return The desired state of the model.
* @see ModelState
*/
public final ModelState desiredState() {
return ModelState.fromValue(desiredState);
}
/**
*
* The desired state of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #desiredState} will
* return {@link ModelState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #desiredStateAsString}.
*
*
* @return The desired state of the model.
* @see ModelState
*/
public final String desiredStateAsString() {
return desiredState;
}
/**
*
* Returns the current state of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ModelState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return Returns the current state of the model.
* @see ModelState
*/
public final ModelState state() {
return ModelState.fromValue(state);
}
/**
*
* Returns the current state of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ModelState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return Returns the current state of the model.
* @see ModelState
*/
public final String stateAsString() {
return state;
}
/**
*
* Returns the deployment status of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link DeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Returns the deployment status of the model.
* @see DeploymentStatus
*/
public final DeploymentStatus status() {
return DeploymentStatus.fromValue(status);
}
/**
*
* Returns the deployment status of the model.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link DeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Returns the deployment status of the model.
* @see DeploymentStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* Returns the error message for the deployment status result.
*
*
* @return Returns the error message for the deployment status result.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* Returns the error message if there is a rollback.
*
*
* @return Returns the error message if there is a rollback.
*/
public final String rollbackFailureReason() {
return rollbackFailureReason;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(modelHandle());
hashCode = 31 * hashCode + Objects.hashCode(modelName());
hashCode = 31 * hashCode + Objects.hashCode(modelVersion());
hashCode = 31 * hashCode + Objects.hashCode(desiredStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(rollbackFailureReason());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentModel)) {
return false;
}
DeploymentModel other = (DeploymentModel) obj;
return Objects.equals(modelHandle(), other.modelHandle()) && Objects.equals(modelName(), other.modelName())
&& Objects.equals(modelVersion(), other.modelVersion())
&& Objects.equals(desiredStateAsString(), other.desiredStateAsString())
&& Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusReason(), other.statusReason())
&& Objects.equals(rollbackFailureReason(), other.rollbackFailureReason());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeploymentModel").add("ModelHandle", modelHandle()).add("ModelName", modelName())
.add("ModelVersion", modelVersion()).add("DesiredState", desiredStateAsString()).add("State", stateAsString())
.add("Status", statusAsString()).add("StatusReason", statusReason())
.add("RollbackFailureReason", rollbackFailureReason()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ModelHandle":
return Optional.ofNullable(clazz.cast(modelHandle()));
case "ModelName":
return Optional.ofNullable(clazz.cast(modelName()));
case "ModelVersion":
return Optional.ofNullable(clazz.cast(modelVersion()));
case "DesiredState":
return Optional.ofNullable(clazz.cast(desiredStateAsString()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "RollbackFailureReason":
return Optional.ofNullable(clazz.cast(rollbackFailureReason()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function