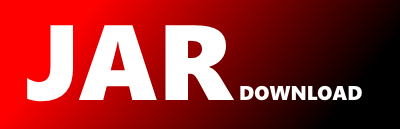
software.amazon.awssdk.services.sagemakeredge.DefaultSagemakerEdgeClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemakeredge Show documentation
Show all versions of sagemakeredge Show documentation
The AWS Java SDK for Sagemaker Edge module holds the client classes that are used for
communicating with Sagemaker Edge.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakeredge;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.sagemakeredge.internal.SagemakerEdgeServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeploymentsRequest;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeploymentsResponse;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeviceRegistrationRequest;
import software.amazon.awssdk.services.sagemakeredge.model.GetDeviceRegistrationResponse;
import software.amazon.awssdk.services.sagemakeredge.model.InternalServiceException;
import software.amazon.awssdk.services.sagemakeredge.model.SagemakerEdgeException;
import software.amazon.awssdk.services.sagemakeredge.model.SendHeartbeatRequest;
import software.amazon.awssdk.services.sagemakeredge.model.SendHeartbeatResponse;
import software.amazon.awssdk.services.sagemakeredge.transform.GetDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.sagemakeredge.transform.GetDeviceRegistrationRequestMarshaller;
import software.amazon.awssdk.services.sagemakeredge.transform.SendHeartbeatRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link SagemakerEdgeClient}.
*
* @see SagemakerEdgeClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSagemakerEdgeClient implements SagemakerEdgeClient {
private static final Logger log = Logger.loggerFor(DefaultSagemakerEdgeClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultSagemakerEdgeClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Use to get the active deployments from a device.
*
*
* @param getDeploymentsRequest
* @return Result of the GetDeployments operation returned by the service.
* @throws InternalServiceException
* An internal failure occurred. Try your request again. If the problem persists, contact Amazon Web
* Services customer support.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SagemakerEdgeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SagemakerEdgeClient.GetDeployments
* @see AWS
* API Documentation
*/
@Override
public GetDeploymentsResponse getDeployments(GetDeploymentsRequest getDeploymentsRequest) throws InternalServiceException,
AwsServiceException, SdkClientException, SagemakerEdgeException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Sagemaker Edge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeployments");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeployments").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeploymentsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeploymentsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Use to check if a device is registered with SageMaker Edge Manager.
*
*
* @param getDeviceRegistrationRequest
* @return Result of the GetDeviceRegistration operation returned by the service.
* @throws InternalServiceException
* An internal failure occurred. Try your request again. If the problem persists, contact Amazon Web
* Services customer support.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SagemakerEdgeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SagemakerEdgeClient.GetDeviceRegistration
* @see AWS API Documentation
*/
@Override
public GetDeviceRegistrationResponse getDeviceRegistration(GetDeviceRegistrationRequest getDeviceRegistrationRequest)
throws InternalServiceException, AwsServiceException, SdkClientException, SagemakerEdgeException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeviceRegistrationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeviceRegistrationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeviceRegistrationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Sagemaker Edge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeviceRegistration");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeviceRegistration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeviceRegistrationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeviceRegistrationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Use to get the current status of devices registered on SageMaker Edge Manager.
*
*
* @param sendHeartbeatRequest
* @return Result of the SendHeartbeat operation returned by the service.
* @throws InternalServiceException
* An internal failure occurred. Try your request again. If the problem persists, contact Amazon Web
* Services customer support.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SagemakerEdgeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SagemakerEdgeClient.SendHeartbeat
* @see AWS
* API Documentation
*/
@Override
public SendHeartbeatResponse sendHeartbeat(SendHeartbeatRequest sendHeartbeatRequest) throws InternalServiceException,
AwsServiceException, SdkClientException, SagemakerEdgeException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SendHeartbeatResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendHeartbeatRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendHeartbeatRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Sagemaker Edge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendHeartbeat");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SendHeartbeat").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(sendHeartbeatRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SendHeartbeatRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
SagemakerEdgeServiceClientConfigurationBuilder serviceConfigBuilder = new SagemakerEdgeServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SagemakerEdgeException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceException")
.exceptionBuilderSupplier(InternalServiceException::builder).build());
}
@Override
public final SagemakerEdgeServiceClientConfiguration serviceClientConfiguration() {
return new SagemakerEdgeServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy