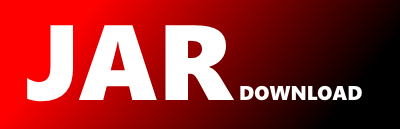
software.amazon.awssdk.services.sagemakerfeaturestoreruntime.SageMakerFeatureStoreRuntimeClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerfeaturestoreruntime;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.AccessForbiddenException;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.BatchGetRecordRequest;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.BatchGetRecordResponse;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.DeleteRecordRequest;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.DeleteRecordResponse;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.GetRecordRequest;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.GetRecordResponse;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.InternalFailureException;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.PutRecordRequest;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.PutRecordResponse;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.ResourceNotFoundException;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.SageMakerFeatureStoreRuntimeException;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.ServiceUnavailableException;
import software.amazon.awssdk.services.sagemakerfeaturestoreruntime.model.ValidationErrorException;
/**
* Service client for accessing Amazon SageMaker Feature Store Runtime. This can be created using the static
* {@link #builder()} method.
*
*
* Contains all data plane API operations and data types for the Amazon SageMaker Feature Store. Use this API to put,
* delete, and retrieve (get) features from a feature store.
*
*
* Use the following operations to configure your OnlineStore
and OfflineStore
features, and
* to create and manage feature groups:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SageMakerFeatureStoreRuntimeClient extends SdkClient {
String SERVICE_NAME = "sagemaker";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "featurestore-runtime.sagemaker";
/**
* Create a {@link SageMakerFeatureStoreRuntimeClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SageMakerFeatureStoreRuntimeClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SageMakerFeatureStoreRuntimeClient}.
*/
static SageMakerFeatureStoreRuntimeClientBuilder builder() {
return new DefaultSageMakerFeatureStoreRuntimeClientBuilder();
}
/**
*
* Retrieves a batch of Records
from a FeatureGroup
.
*
*
* @param batchGetRecordRequest
* @return Result of the BatchGetRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.BatchGetRecord
* @see AWS API Documentation
*/
default BatchGetRecordResponse batchGetRecord(BatchGetRecordRequest batchGetRecordRequest) throws ValidationErrorException,
InternalFailureException, ServiceUnavailableException, AccessForbiddenException, AwsServiceException,
SdkClientException, SageMakerFeatureStoreRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a batch of Records
from a FeatureGroup
.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetRecordRequest.Builder} avoiding the need to
* create one manually via {@link BatchGetRecordRequest#builder()}
*
*
* @param batchGetRecordRequest
* A {@link Consumer} that will call methods on {@link BatchGetRecordRequest.Builder} to create a request.
* @return Result of the BatchGetRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.BatchGetRecord
* @see AWS API Documentation
*/
default BatchGetRecordResponse batchGetRecord(Consumer batchGetRecordRequest)
throws ValidationErrorException, InternalFailureException, ServiceUnavailableException, AccessForbiddenException,
AwsServiceException, SdkClientException, SageMakerFeatureStoreRuntimeException {
return batchGetRecord(BatchGetRecordRequest.builder().applyMutation(batchGetRecordRequest).build());
}
/**
*
* Deletes a Record
from a FeatureGroup
. A new record will show up in the
* OfflineStore
when the DeleteRecord
API is called. This record will have a value of
* True
in the is_deleted
column.
*
*
* @param deleteRecordRequest
* @return Result of the DeleteRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.DeleteRecord
* @see AWS API Documentation
*/
default DeleteRecordResponse deleteRecord(DeleteRecordRequest deleteRecordRequest) throws ValidationErrorException,
InternalFailureException, ServiceUnavailableException, AccessForbiddenException, AwsServiceException,
SdkClientException, SageMakerFeatureStoreRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Record
from a FeatureGroup
. A new record will show up in the
* OfflineStore
when the DeleteRecord
API is called. This record will have a value of
* True
in the is_deleted
column.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRecordRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRecordRequest#builder()}
*
*
* @param deleteRecordRequest
* A {@link Consumer} that will call methods on {@link DeleteRecordRequest.Builder} to create a request.
* @return Result of the DeleteRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.DeleteRecord
* @see AWS API Documentation
*/
default DeleteRecordResponse deleteRecord(Consumer deleteRecordRequest)
throws ValidationErrorException, InternalFailureException, ServiceUnavailableException, AccessForbiddenException,
AwsServiceException, SdkClientException, SageMakerFeatureStoreRuntimeException {
return deleteRecord(DeleteRecordRequest.builder().applyMutation(deleteRecordRequest).build());
}
/**
*
* Use for OnlineStore
serving from a FeatureStore
. Only the latest records stored in the
* OnlineStore
can be retrieved. If no Record with RecordIdentifierValue
is found, then an
* empty result is returned.
*
*
* @param getRecordRequest
* @return Result of the GetRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws ResourceNotFoundException
* A resource that is required to perform an action was not found.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.GetRecord
* @see AWS API Documentation
*/
default GetRecordResponse getRecord(GetRecordRequest getRecordRequest) throws ValidationErrorException,
ResourceNotFoundException, InternalFailureException, ServiceUnavailableException, AccessForbiddenException,
AwsServiceException, SdkClientException, SageMakerFeatureStoreRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Use for OnlineStore
serving from a FeatureStore
. Only the latest records stored in the
* OnlineStore
can be retrieved. If no Record with RecordIdentifierValue
is found, then an
* empty result is returned.
*
*
*
* This is a convenience which creates an instance of the {@link GetRecordRequest.Builder} avoiding the need to
* create one manually via {@link GetRecordRequest#builder()}
*
*
* @param getRecordRequest
* A {@link Consumer} that will call methods on {@link GetRecordRequest.Builder} to create a request.
* @return Result of the GetRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws ResourceNotFoundException
* A resource that is required to perform an action was not found.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.GetRecord
* @see AWS API Documentation
*/
default GetRecordResponse getRecord(Consumer getRecordRequest) throws ValidationErrorException,
ResourceNotFoundException, InternalFailureException, ServiceUnavailableException, AccessForbiddenException,
AwsServiceException, SdkClientException, SageMakerFeatureStoreRuntimeException {
return getRecord(GetRecordRequest.builder().applyMutation(getRecordRequest).build());
}
/**
*
* Used for data ingestion into the FeatureStore
. The PutRecord
API writes to both the
* OnlineStore
and OfflineStore
. If the record is the latest record for the
* recordIdentifier
, the record is written to both the OnlineStore
and
* OfflineStore
. If the record is a historic record, it is written only to the
* OfflineStore
.
*
*
* @param putRecordRequest
* @return Result of the PutRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.PutRecord
* @see AWS API Documentation
*/
default PutRecordResponse putRecord(PutRecordRequest putRecordRequest) throws ValidationErrorException,
InternalFailureException, ServiceUnavailableException, AccessForbiddenException, AwsServiceException,
SdkClientException, SageMakerFeatureStoreRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Used for data ingestion into the FeatureStore
. The PutRecord
API writes to both the
* OnlineStore
and OfflineStore
. If the record is the latest record for the
* recordIdentifier
, the record is written to both the OnlineStore
and
* OfflineStore
. If the record is a historic record, it is written only to the
* OfflineStore
.
*
*
*
* This is a convenience which creates an instance of the {@link PutRecordRequest.Builder} avoiding the need to
* create one manually via {@link PutRecordRequest#builder()}
*
*
* @param putRecordRequest
* A {@link Consumer} that will call methods on {@link PutRecordRequest.Builder} to create a request.
* @return Result of the PutRecord operation returned by the service.
* @throws ValidationErrorException
* There was an error validating your request.
* @throws InternalFailureException
* An internal failure occurred. Try your request again. If the problem persists, contact AWS customer
* support.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws AccessForbiddenException
* You do not have permission to perform an action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerFeatureStoreRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerFeatureStoreRuntimeClient.PutRecord
* @see AWS API Documentation
*/
default PutRecordResponse putRecord(Consumer putRecordRequest) throws ValidationErrorException,
InternalFailureException, ServiceUnavailableException, AccessForbiddenException, AwsServiceException,
SdkClientException, SageMakerFeatureStoreRuntimeException {
return putRecord(PutRecordRequest.builder().applyMutation(putRecordRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}