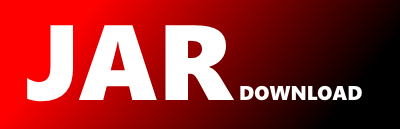
software.amazon.awssdk.services.sagemakergeospatial.model.Properties Maven / Gradle / Ivy
Show all versions of sagemakergeospatial Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakergeospatial.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Properties associated with the Item.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Properties implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField EO_CLOUD_COVER_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("EoCloudCover").getter(getter(Properties::eoCloudCover)).setter(setter(Builder::eoCloudCover))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EoCloudCover").build()).build();
private static final SdkField LANDSAT_CLOUD_COVER_LAND_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("LandsatCloudCoverLand").getter(getter(Properties::landsatCloudCoverLand))
.setter(setter(Builder::landsatCloudCoverLand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LandsatCloudCoverLand").build())
.build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(Properties::platform)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField VIEW_OFF_NADIR_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("ViewOffNadir").getter(getter(Properties::viewOffNadir)).setter(setter(Builder::viewOffNadir))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewOffNadir").build()).build();
private static final SdkField VIEW_SUN_AZIMUTH_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("ViewSunAzimuth").getter(getter(Properties::viewSunAzimuth)).setter(setter(Builder::viewSunAzimuth))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewSunAzimuth").build()).build();
private static final SdkField VIEW_SUN_ELEVATION_FIELD = SdkField. builder(MarshallingType.FLOAT)
.memberName("ViewSunElevation").getter(getter(Properties::viewSunElevation))
.setter(setter(Builder::viewSunElevation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewSunElevation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EO_CLOUD_COVER_FIELD,
LANDSAT_CLOUD_COVER_LAND_FIELD, PLATFORM_FIELD, VIEW_OFF_NADIR_FIELD, VIEW_SUN_AZIMUTH_FIELD,
VIEW_SUN_ELEVATION_FIELD));
private static final long serialVersionUID = 1L;
private final Float eoCloudCover;
private final Float landsatCloudCoverLand;
private final String platform;
private final Float viewOffNadir;
private final Float viewSunAzimuth;
private final Float viewSunElevation;
private Properties(BuilderImpl builder) {
this.eoCloudCover = builder.eoCloudCover;
this.landsatCloudCoverLand = builder.landsatCloudCoverLand;
this.platform = builder.platform;
this.viewOffNadir = builder.viewOffNadir;
this.viewSunAzimuth = builder.viewSunAzimuth;
this.viewSunElevation = builder.viewSunElevation;
}
/**
*
* Estimate of cloud cover.
*
*
* @return Estimate of cloud cover.
*/
public final Float eoCloudCover() {
return eoCloudCover;
}
/**
*
* Land cloud cover for Landsat Data Collection.
*
*
* @return Land cloud cover for Landsat Data Collection.
*/
public final Float landsatCloudCoverLand() {
return landsatCloudCoverLand;
}
/**
*
* Platform property. Platform refers to the unique name of the specific platform the instrument is attached to. For
* satellites it is the name of the satellite, eg. landsat-8 (Landsat-8), sentinel-2a.
*
*
* @return Platform property. Platform refers to the unique name of the specific platform the instrument is attached
* to. For satellites it is the name of the satellite, eg. landsat-8 (Landsat-8), sentinel-2a.
*/
public final String platform() {
return platform;
}
/**
*
* The angle from the sensor between nadir (straight down) and the scene center. Measured in degrees (0-90).
*
*
* @return The angle from the sensor between nadir (straight down) and the scene center. Measured in degrees (0-90).
*/
public final Float viewOffNadir() {
return viewOffNadir;
}
/**
*
* The sun azimuth angle. From the scene center point on the ground, this is the angle between truth north and the
* sun. Measured clockwise in degrees (0-360).
*
*
* @return The sun azimuth angle. From the scene center point on the ground, this is the angle between truth north
* and the sun. Measured clockwise in degrees (0-360).
*/
public final Float viewSunAzimuth() {
return viewSunAzimuth;
}
/**
*
* The sun elevation angle. The angle from the tangent of the scene center point to the sun. Measured from the
* horizon in degrees (-90-90). Negative values indicate the sun is below the horizon, e.g. sun elevation of -10°
* means the data was captured during nautical twilight.
*
*
* @return The sun elevation angle. The angle from the tangent of the scene center point to the sun. Measured from
* the horizon in degrees (-90-90). Negative values indicate the sun is below the horizon, e.g. sun
* elevation of -10° means the data was captured during nautical twilight.
*/
public final Float viewSunElevation() {
return viewSunElevation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eoCloudCover());
hashCode = 31 * hashCode + Objects.hashCode(landsatCloudCoverLand());
hashCode = 31 * hashCode + Objects.hashCode(platform());
hashCode = 31 * hashCode + Objects.hashCode(viewOffNadir());
hashCode = 31 * hashCode + Objects.hashCode(viewSunAzimuth());
hashCode = 31 * hashCode + Objects.hashCode(viewSunElevation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Properties)) {
return false;
}
Properties other = (Properties) obj;
return Objects.equals(eoCloudCover(), other.eoCloudCover())
&& Objects.equals(landsatCloudCoverLand(), other.landsatCloudCoverLand())
&& Objects.equals(platform(), other.platform()) && Objects.equals(viewOffNadir(), other.viewOffNadir())
&& Objects.equals(viewSunAzimuth(), other.viewSunAzimuth())
&& Objects.equals(viewSunElevation(), other.viewSunElevation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Properties").add("EoCloudCover", eoCloudCover())
.add("LandsatCloudCoverLand", landsatCloudCoverLand()).add("Platform", platform())
.add("ViewOffNadir", viewOffNadir()).add("ViewSunAzimuth", viewSunAzimuth())
.add("ViewSunElevation", viewSunElevation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EoCloudCover":
return Optional.ofNullable(clazz.cast(eoCloudCover()));
case "LandsatCloudCoverLand":
return Optional.ofNullable(clazz.cast(landsatCloudCoverLand()));
case "Platform":
return Optional.ofNullable(clazz.cast(platform()));
case "ViewOffNadir":
return Optional.ofNullable(clazz.cast(viewOffNadir()));
case "ViewSunAzimuth":
return Optional.ofNullable(clazz.cast(viewSunAzimuth()));
case "ViewSunElevation":
return Optional.ofNullable(clazz.cast(viewSunElevation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function