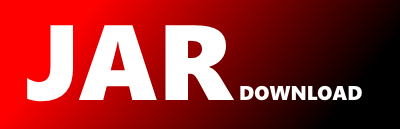
software.amazon.awssdk.services.sagemakergeospatial.SageMakerGeospatialClient Maven / Gradle / Ivy
Show all versions of sagemakergeospatial Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakergeospatial;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ResponseBytes;
import software.amazon.awssdk.core.ResponseInputStream;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.sagemakergeospatial.model.AccessDeniedException;
import software.amazon.awssdk.services.sagemakergeospatial.model.ConflictException;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetTileResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.InternalServerException;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ResourceNotFoundException;
import software.amazon.awssdk.services.sagemakergeospatial.model.SageMakerGeospatialException;
import software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ThrottlingException;
import software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ValidationException;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable;
/**
* Service client for accessing Amazon SageMaker geospatial capabilities. This can be created using the static
* {@link #builder()} method.
*
*
* Provides APIs for creating and managing SageMaker geospatial resources.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SageMakerGeospatialClient extends AwsClient {
String SERVICE_NAME = "sagemaker-geospatial";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "sagemaker-geospatial";
/**
*
* Use this operation to delete an Earth Observation job.
*
*
* @param deleteEarthObservationJobRequest
* @return Result of the DeleteEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.DeleteEarthObservationJob
* @see AWS API Documentation
*/
default DeleteEarthObservationJobResponse deleteEarthObservationJob(
DeleteEarthObservationJobRequest deleteEarthObservationJobRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to delete an Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link DeleteEarthObservationJobRequest#builder()}
*
*
* @param deleteEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobRequest.Builder}
* to create a request.
* @return Result of the DeleteEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.DeleteEarthObservationJob
* @see AWS API Documentation
*/
default DeleteEarthObservationJobResponse deleteEarthObservationJob(
Consumer deleteEarthObservationJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException,
AwsServiceException, SdkClientException, SageMakerGeospatialException {
return deleteEarthObservationJob(DeleteEarthObservationJobRequest.builder()
.applyMutation(deleteEarthObservationJobRequest).build());
}
/**
*
* Use this operation to delete a Vector Enrichment job.
*
*
* @param deleteVectorEnrichmentJobRequest
* @return Result of the DeleteVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.DeleteVectorEnrichmentJob
* @see AWS API Documentation
*/
default DeleteVectorEnrichmentJobResponse deleteVectorEnrichmentJob(
DeleteVectorEnrichmentJobRequest deleteVectorEnrichmentJobRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to delete a Vector Enrichment job.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link DeleteVectorEnrichmentJobRequest#builder()}
*
*
* @param deleteVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return Result of the DeleteVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.DeleteVectorEnrichmentJob
* @see AWS API Documentation
*/
default DeleteVectorEnrichmentJobResponse deleteVectorEnrichmentJob(
Consumer deleteVectorEnrichmentJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException,
AwsServiceException, SdkClientException, SageMakerGeospatialException {
return deleteVectorEnrichmentJob(DeleteVectorEnrichmentJobRequest.builder()
.applyMutation(deleteVectorEnrichmentJobRequest).build());
}
/**
*
* Use this operation to export results of an Earth Observation job and optionally source images used as input to
* the EOJ to an Amazon S3 location.
*
*
* @param exportEarthObservationJobRequest
* @return Result of the ExportEarthObservationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ExportEarthObservationJob
* @see AWS API Documentation
*/
default ExportEarthObservationJobResponse exportEarthObservationJob(
ExportEarthObservationJobRequest exportEarthObservationJobRequest) throws ServiceQuotaExceededException,
AccessDeniedException, ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException,
ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to export results of an Earth Observation job and optionally source images used as input to
* the EOJ to an Amazon S3 location.
*
*
*
* This is a convenience which creates an instance of the {@link ExportEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link ExportEarthObservationJobRequest#builder()}
*
*
* @param exportEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobRequest.Builder}
* to create a request.
* @return Result of the ExportEarthObservationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ExportEarthObservationJob
* @see AWS API Documentation
*/
default ExportEarthObservationJobResponse exportEarthObservationJob(
Consumer exportEarthObservationJobRequest)
throws ServiceQuotaExceededException, AccessDeniedException, ValidationException, ThrottlingException,
InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return exportEarthObservationJob(ExportEarthObservationJobRequest.builder()
.applyMutation(exportEarthObservationJobRequest).build());
}
/**
*
* Use this operation to copy results of a Vector Enrichment job to an Amazon S3 location.
*
*
* @param exportVectorEnrichmentJobRequest
* @return Result of the ExportVectorEnrichmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ExportVectorEnrichmentJob
* @see AWS API Documentation
*/
default ExportVectorEnrichmentJobResponse exportVectorEnrichmentJob(
ExportVectorEnrichmentJobRequest exportVectorEnrichmentJobRequest) throws ServiceQuotaExceededException,
AccessDeniedException, ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException,
ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to copy results of a Vector Enrichment job to an Amazon S3 location.
*
*
*
* This is a convenience which creates an instance of the {@link ExportVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link ExportVectorEnrichmentJobRequest#builder()}
*
*
* @param exportVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return Result of the ExportVectorEnrichmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ExportVectorEnrichmentJob
* @see AWS API Documentation
*/
default ExportVectorEnrichmentJobResponse exportVectorEnrichmentJob(
Consumer exportVectorEnrichmentJobRequest)
throws ServiceQuotaExceededException, AccessDeniedException, ValidationException, ThrottlingException,
InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return exportVectorEnrichmentJob(ExportVectorEnrichmentJobRequest.builder()
.applyMutation(exportVectorEnrichmentJobRequest).build());
}
/**
*
* Get the details for a previously initiated Earth Observation job.
*
*
* @param getEarthObservationJobRequest
* @return Result of the GetEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetEarthObservationJob
* @see AWS API Documentation
*/
default GetEarthObservationJobResponse getEarthObservationJob(GetEarthObservationJobRequest getEarthObservationJobRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Get the details for a previously initiated Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetEarthObservationJobRequest.Builder} avoiding the
* need to create one manually via {@link GetEarthObservationJobRequest#builder()}
*
*
* @param getEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobRequest.Builder} to
* create a request.
* @return Result of the GetEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetEarthObservationJob
* @see AWS API Documentation
*/
default GetEarthObservationJobResponse getEarthObservationJob(
Consumer getEarthObservationJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getEarthObservationJob(GetEarthObservationJobRequest.builder().applyMutation(getEarthObservationJobRequest)
.build());
}
/**
*
* Use this operation to get details of a specific raster data collection.
*
*
* @param getRasterDataCollectionRequest
* @return Result of the GetRasterDataCollection operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetRasterDataCollection
* @see AWS API Documentation
*/
default GetRasterDataCollectionResponse getRasterDataCollection(GetRasterDataCollectionRequest getRasterDataCollectionRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get details of a specific raster data collection.
*
*
*
* This is a convenience which creates an instance of the {@link GetRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link GetRasterDataCollectionRequest#builder()}
*
*
* @param getRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionRequest.Builder}
* to create a request.
* @return Result of the GetRasterDataCollection operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetRasterDataCollection
* @see AWS API Documentation
*/
default GetRasterDataCollectionResponse getRasterDataCollection(
Consumer getRasterDataCollectionRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getRasterDataCollection(GetRasterDataCollectionRequest.builder().applyMutation(getRasterDataCollectionRequest)
.build());
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetTileResponse and an
* InputStream to the response content are provided as parameters to the callback. The callback may return a
* transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see AWS
* API Documentation
*/
default ReturnT getTile(GetTileRequest getTileRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled GetTileResponse and an
* InputStream to the response content are provided as parameters to the callback. The callback may return a
* transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see AWS
* API Documentation
*/
default ReturnT getTile(Consumer getTileRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return getTile(GetTileRequest.builder().applyMutation(getTileRequest).build(), responseTransformer);
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getTile(GetTileRequest, ResponseTransformer)
* @see AWS
* API Documentation
*/
default GetTileResponse getTile(GetTileRequest getTileRequest, Path destinationPath) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getTile(getTileRequest, ResponseTransformer.toFile(destinationPath));
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getTile(GetTileRequest, ResponseTransformer)
* @see AWS
* API Documentation
*/
default GetTileResponse getTile(Consumer getTileRequest, Path destinationPath)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
return getTile(GetTileRequest.builder().applyMutation(getTileRequest).build(), destinationPath);
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The output binary file.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getObject(getTile, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseInputStream getTile(GetTileRequest getTileRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getTile(getTileRequest, ResponseTransformer.toInputStream());
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The output binary file.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getObject(getTile, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseInputStream getTile(Consumer getTileRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
return getTile(GetTileRequest.builder().applyMutation(getTileRequest).build());
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The output binary file.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getObject(getTile, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseBytes getTileAsBytes(GetTileRequest getTileRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getTile(getTileRequest, ResponseTransformer.toBytes());
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The output binary file.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetTile
* @see #getObject(getTile, ResponseTransformer)
* @see AWS
* API Documentation
*/
default ResponseBytes getTileAsBytes(Consumer getTileRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
return getTileAsBytes(GetTileRequest.builder().applyMutation(getTileRequest).build());
}
/**
*
* Retrieves details of a Vector Enrichment Job for a given job Amazon Resource Name (ARN).
*
*
* @param getVectorEnrichmentJobRequest
* @return Result of the GetVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetVectorEnrichmentJob
* @see AWS API Documentation
*/
default GetVectorEnrichmentJobResponse getVectorEnrichmentJob(GetVectorEnrichmentJobRequest getVectorEnrichmentJobRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of a Vector Enrichment Job for a given job Amazon Resource Name (ARN).
*
*
*
* This is a convenience which creates an instance of the {@link GetVectorEnrichmentJobRequest.Builder} avoiding the
* need to create one manually via {@link GetVectorEnrichmentJobRequest#builder()}
*
*
* @param getVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobRequest.Builder} to
* create a request.
* @return Result of the GetVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.GetVectorEnrichmentJob
* @see AWS API Documentation
*/
default GetVectorEnrichmentJobResponse getVectorEnrichmentJob(
Consumer getVectorEnrichmentJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return getVectorEnrichmentJob(GetVectorEnrichmentJobRequest.builder().applyMutation(getVectorEnrichmentJobRequest)
.build());
}
/**
*
* Use this operation to get a list of the Earth Observation jobs associated with the calling Amazon Web Services
* account.
*
*
* @param listEarthObservationJobsRequest
* @return Result of the ListEarthObservationJobs operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsResponse listEarthObservationJobs(
ListEarthObservationJobsRequest listEarthObservationJobsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get a list of the Earth Observation jobs associated with the calling Amazon Web Services
* account.
*
*
*
* This is a convenience which creates an instance of the {@link ListEarthObservationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListEarthObservationJobsRequest#builder()}
*
*
* @param listEarthObservationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest.Builder}
* to create a request.
* @return Result of the ListEarthObservationJobs operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsResponse listEarthObservationJobs(
Consumer listEarthObservationJobsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listEarthObservationJobs(ListEarthObservationJobsRequest.builder().applyMutation(listEarthObservationJobsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client.listEarthObservationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client
* .listEarthObservationJobsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client.listEarthObservationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation.
*
*
* @param listEarthObservationJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsIterable listEarthObservationJobsPaginator(
ListEarthObservationJobsRequest listEarthObservationJobsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return new ListEarthObservationJobsIterable(this, listEarthObservationJobsRequest);
}
/**
*
* This is a variant of
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client.listEarthObservationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client
* .listEarthObservationJobsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsIterable responses = client.listEarthObservationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListEarthObservationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListEarthObservationJobsRequest#builder()}
*
*
* @param listEarthObservationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest.Builder}
* to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsIterable listEarthObservationJobsPaginator(
Consumer listEarthObservationJobsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listEarthObservationJobsPaginator(ListEarthObservationJobsRequest.builder()
.applyMutation(listEarthObservationJobsRequest).build());
}
/**
*
* Use this operation to get raster data collections.
*
*
* @param listRasterDataCollectionsRequest
* @return Result of the ListRasterDataCollections operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsResponse listRasterDataCollections(
ListRasterDataCollectionsRequest listRasterDataCollectionsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get raster data collections.
*
*
*
* This is a convenience which creates an instance of the {@link ListRasterDataCollectionsRequest.Builder} avoiding
* the need to create one manually via {@link ListRasterDataCollectionsRequest#builder()}
*
*
* @param listRasterDataCollectionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest.Builder}
* to create a request.
* @return Result of the ListRasterDataCollections operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsResponse listRasterDataCollections(
Consumer listRasterDataCollectionsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listRasterDataCollections(ListRasterDataCollectionsRequest.builder()
.applyMutation(listRasterDataCollectionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client.listRasterDataCollectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client
* .listRasterDataCollectionsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client.listRasterDataCollectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation.
*
*
* @param listRasterDataCollectionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsIterable listRasterDataCollectionsPaginator(
ListRasterDataCollectionsRequest listRasterDataCollectionsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return new ListRasterDataCollectionsIterable(this, listRasterDataCollectionsRequest);
}
/**
*
* This is a variant of
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client.listRasterDataCollectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client
* .listRasterDataCollectionsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsIterable responses = client.listRasterDataCollectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRasterDataCollectionsRequest.Builder} avoiding
* the need to create one manually via {@link ListRasterDataCollectionsRequest#builder()}
*
*
* @param listRasterDataCollectionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest.Builder}
* to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsIterable listRasterDataCollectionsPaginator(
Consumer listRasterDataCollectionsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listRasterDataCollectionsPaginator(ListRasterDataCollectionsRequest.builder()
.applyMutation(listRasterDataCollectionsRequest).build());
}
/**
*
* Lists the tags attached to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags attached to the resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceRequest.Builder} to
* create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Retrieves a list of vector enrichment jobs.
*
*
* @param listVectorEnrichmentJobsRequest
* @return Result of the ListVectorEnrichmentJobs operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsResponse listVectorEnrichmentJobs(
ListVectorEnrichmentJobsRequest listVectorEnrichmentJobsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of vector enrichment jobs.
*
*
*
* This is a convenience which creates an instance of the {@link ListVectorEnrichmentJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListVectorEnrichmentJobsRequest#builder()}
*
*
* @param listVectorEnrichmentJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest.Builder}
* to create a request.
* @return Result of the ListVectorEnrichmentJobs operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsResponse listVectorEnrichmentJobs(
Consumer listVectorEnrichmentJobsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listVectorEnrichmentJobs(ListVectorEnrichmentJobsRequest.builder().applyMutation(listVectorEnrichmentJobsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client.listVectorEnrichmentJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client
* .listVectorEnrichmentJobsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client.listVectorEnrichmentJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation.
*
*
* @param listVectorEnrichmentJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsIterable listVectorEnrichmentJobsPaginator(
ListVectorEnrichmentJobsRequest listVectorEnrichmentJobsRequest) throws AccessDeniedException, ValidationException,
ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return new ListVectorEnrichmentJobsIterable(this, listVectorEnrichmentJobsRequest);
}
/**
*
* This is a variant of
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client.listVectorEnrichmentJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client
* .listVectorEnrichmentJobsPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsIterable responses = client.listVectorEnrichmentJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVectorEnrichmentJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListVectorEnrichmentJobsRequest#builder()}
*
*
* @param listVectorEnrichmentJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest.Builder}
* to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsIterable listVectorEnrichmentJobsPaginator(
Consumer listVectorEnrichmentJobsRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return listVectorEnrichmentJobsPaginator(ListVectorEnrichmentJobsRequest.builder()
.applyMutation(listVectorEnrichmentJobsRequest).build());
}
/**
*
* Allows you run image query on a specific raster data collection to get a list of the satellite imagery matching
* the selected filters.
*
*
* @param searchRasterDataCollectionRequest
* @return Result of the SearchRasterDataCollection operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionResponse searchRasterDataCollection(
SearchRasterDataCollectionRequest searchRasterDataCollectionRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Allows you run image query on a specific raster data collection to get a list of the satellite imagery matching
* the selected filters.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link SearchRasterDataCollectionRequest#builder()}
*
*
* @param searchRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest.Builder}
* to create a request.
* @return Result of the SearchRasterDataCollection operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionResponse searchRasterDataCollection(
Consumer searchRasterDataCollectionRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return searchRasterDataCollection(SearchRasterDataCollectionRequest.builder()
.applyMutation(searchRasterDataCollectionRequest).build());
}
/**
*
* This is a variant of
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client.searchRasterDataCollectionPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client
* .searchRasterDataCollectionPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client.searchRasterDataCollectionPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation.
*
*
* @param searchRasterDataCollectionRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionIterable searchRasterDataCollectionPaginator(
SearchRasterDataCollectionRequest searchRasterDataCollectionRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return new SearchRasterDataCollectionIterable(this, searchRasterDataCollectionRequest);
}
/**
*
* This is a variant of
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client.searchRasterDataCollectionPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client
* .searchRasterDataCollectionPaginator(request);
* for (software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionIterable responses = client.searchRasterDataCollectionPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link SearchRasterDataCollectionRequest#builder()}
*
*
* @param searchRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest.Builder}
* to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionIterable searchRasterDataCollectionPaginator(
Consumer searchRasterDataCollectionRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
return searchRasterDataCollectionPaginator(SearchRasterDataCollectionRequest.builder()
.applyMutation(searchRasterDataCollectionRequest).build());
}
/**
*
* Use this operation to create an Earth observation job.
*
*
* @param startEarthObservationJobRequest
* @return Result of the StartEarthObservationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StartEarthObservationJob
* @see AWS API Documentation
*/
default StartEarthObservationJobResponse startEarthObservationJob(
StartEarthObservationJobRequest startEarthObservationJobRequest) throws ServiceQuotaExceededException,
AccessDeniedException, ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException,
ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to create an Earth observation job.
*
*
*
* This is a convenience which creates an instance of the {@link StartEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link StartEarthObservationJobRequest#builder()}
*
*
* @param startEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobRequest.Builder}
* to create a request.
* @return Result of the StartEarthObservationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StartEarthObservationJob
* @see AWS API Documentation
*/
default StartEarthObservationJobResponse startEarthObservationJob(
Consumer startEarthObservationJobRequest)
throws ServiceQuotaExceededException, AccessDeniedException, ValidationException, ThrottlingException,
InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return startEarthObservationJob(StartEarthObservationJobRequest.builder().applyMutation(startEarthObservationJobRequest)
.build());
}
/**
*
* Creates a Vector Enrichment job for the supplied job type. Currently, there are two supported job types: reverse
* geocoding and map matching.
*
*
* @param startVectorEnrichmentJobRequest
* @return Result of the StartVectorEnrichmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StartVectorEnrichmentJob
* @see AWS API Documentation
*/
default StartVectorEnrichmentJobResponse startVectorEnrichmentJob(
StartVectorEnrichmentJobRequest startVectorEnrichmentJobRequest) throws ServiceQuotaExceededException,
AccessDeniedException, ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException,
ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Vector Enrichment job for the supplied job type. Currently, there are two supported job types: reverse
* geocoding and map matching.
*
*
*
* This is a convenience which creates an instance of the {@link StartVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link StartVectorEnrichmentJobRequest#builder()}
*
*
* @param startVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return Result of the StartVectorEnrichmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* You have exceeded the service quota.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StartVectorEnrichmentJob
* @see AWS API Documentation
*/
default StartVectorEnrichmentJobResponse startVectorEnrichmentJob(
Consumer startVectorEnrichmentJobRequest)
throws ServiceQuotaExceededException, AccessDeniedException, ValidationException, ThrottlingException,
InternalServerException, ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException,
SageMakerGeospatialException {
return startVectorEnrichmentJob(StartVectorEnrichmentJobRequest.builder().applyMutation(startVectorEnrichmentJobRequest)
.build());
}
/**
*
* Use this operation to stop an existing earth observation job.
*
*
* @param stopEarthObservationJobRequest
* @return Result of the StopEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StopEarthObservationJob
* @see AWS API Documentation
*/
default StopEarthObservationJobResponse stopEarthObservationJob(StopEarthObservationJobRequest stopEarthObservationJobRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to stop an existing earth observation job.
*
*
*
* This is a convenience which creates an instance of the {@link StopEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link StopEarthObservationJobRequest#builder()}
*
*
* @param stopEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobRequest.Builder}
* to create a request.
* @return Result of the StopEarthObservationJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StopEarthObservationJob
* @see AWS API Documentation
*/
default StopEarthObservationJobResponse stopEarthObservationJob(
Consumer stopEarthObservationJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException,
AwsServiceException, SdkClientException, SageMakerGeospatialException {
return stopEarthObservationJob(StopEarthObservationJobRequest.builder().applyMutation(stopEarthObservationJobRequest)
.build());
}
/**
*
* Stops the Vector Enrichment job for a given job ARN.
*
*
* @param stopVectorEnrichmentJobRequest
* @return Result of the StopVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StopVectorEnrichmentJob
* @see AWS API Documentation
*/
default StopVectorEnrichmentJobResponse stopVectorEnrichmentJob(StopVectorEnrichmentJobRequest stopVectorEnrichmentJobRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, ConflictException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* Stops the Vector Enrichment job for a given job ARN.
*
*
*
* This is a convenience which creates an instance of the {@link StopVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link StopVectorEnrichmentJobRequest#builder()}
*
*
* @param stopVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return Result of the StopVectorEnrichmentJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.StopVectorEnrichmentJob
* @see AWS API Documentation
*/
default StopVectorEnrichmentJobResponse stopVectorEnrichmentJob(
Consumer stopVectorEnrichmentJobRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, ConflictException,
AwsServiceException, SdkClientException, SageMakerGeospatialException {
return stopVectorEnrichmentJob(StopVectorEnrichmentJobRequest.builder().applyMutation(stopVectorEnrichmentJobRequest)
.build());
}
/**
*
* The resource you want to tag.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.TagResource
* @see AWS API Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* The resource you want to tag.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceRequest.Builder} to create a
* request.
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.TagResource
* @see AWS API Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* The resource you want to untag.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.UntagResource
* @see AWS API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws AccessDeniedException,
ValidationException, ThrottlingException, InternalServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, SageMakerGeospatialException {
throw new UnsupportedOperationException();
}
/**
*
* The resource you want to untag.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceRequest.Builder} to create a
* request.
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ResourceNotFoundException
* The request references a resource which does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerGeospatialException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerGeospatialClient.UntagResource
* @see AWS API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws AccessDeniedException, ValidationException, ThrottlingException, InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, SageMakerGeospatialException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
* Create a {@link SageMakerGeospatialClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SageMakerGeospatialClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SageMakerGeospatialClient}.
*/
static SageMakerGeospatialClientBuilder builder() {
return new DefaultSageMakerGeospatialClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default SageMakerGeospatialServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}