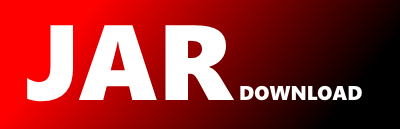
software.amazon.awssdk.services.sagemakergeospatial.model.Property Maven / Gradle / Ivy
Show all versions of sagemakergeospatial Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakergeospatial.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a single searchable property to search on.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Property implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField EO_CLOUD_COVER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("EoCloudCover")
.getter(getter(Property::eoCloudCover)).setter(setter(Builder::eoCloudCover)).constructor(EoCloudCoverInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EoCloudCover").build()).build();
private static final SdkField LANDSAT_CLOUD_COVER_LAND_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LandsatCloudCoverLand")
.getter(getter(Property::landsatCloudCoverLand)).setter(setter(Builder::landsatCloudCoverLand))
.constructor(LandsatCloudCoverLandInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LandsatCloudCoverLand").build())
.build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Platform").getter(getter(Property::platform)).setter(setter(Builder::platform))
.constructor(PlatformInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField VIEW_OFF_NADIR_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ViewOffNadir")
.getter(getter(Property::viewOffNadir)).setter(setter(Builder::viewOffNadir)).constructor(ViewOffNadirInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewOffNadir").build()).build();
private static final SdkField VIEW_SUN_AZIMUTH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ViewSunAzimuth")
.getter(getter(Property::viewSunAzimuth)).setter(setter(Builder::viewSunAzimuth))
.constructor(ViewSunAzimuthInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewSunAzimuth").build()).build();
private static final SdkField VIEW_SUN_ELEVATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ViewSunElevation")
.getter(getter(Property::viewSunElevation)).setter(setter(Builder::viewSunElevation))
.constructor(ViewSunElevationInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViewSunElevation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EO_CLOUD_COVER_FIELD,
LANDSAT_CLOUD_COVER_LAND_FIELD, PLATFORM_FIELD, VIEW_OFF_NADIR_FIELD, VIEW_SUN_AZIMUTH_FIELD,
VIEW_SUN_ELEVATION_FIELD));
private static final long serialVersionUID = 1L;
private final EoCloudCoverInput eoCloudCover;
private final LandsatCloudCoverLandInput landsatCloudCoverLand;
private final PlatformInput platform;
private final ViewOffNadirInput viewOffNadir;
private final ViewSunAzimuthInput viewSunAzimuth;
private final ViewSunElevationInput viewSunElevation;
private final Type type;
private Property(BuilderImpl builder) {
this.eoCloudCover = builder.eoCloudCover;
this.landsatCloudCoverLand = builder.landsatCloudCoverLand;
this.platform = builder.platform;
this.viewOffNadir = builder.viewOffNadir;
this.viewSunAzimuth = builder.viewSunAzimuth;
this.viewSunElevation = builder.viewSunElevation;
this.type = builder.type;
}
/**
*
* The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*
*
* @return The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*/
public final EoCloudCoverInput eoCloudCover() {
return eoCloudCover;
}
/**
*
* The structure representing Land Cloud Cover property filter for Landsat collection containing a lower bound and
* upper bound.
*
*
* @return The structure representing Land Cloud Cover property filter for Landsat collection containing a lower
* bound and upper bound.
*/
public final LandsatCloudCoverLandInput landsatCloudCoverLand() {
return landsatCloudCoverLand;
}
/**
*
* The structure representing Platform property filter consisting of value and comparison operator.
*
*
* @return The structure representing Platform property filter consisting of value and comparison operator.
*/
public final PlatformInput platform() {
return platform;
}
/**
*
* The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*
*
* @return The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*/
public final ViewOffNadirInput viewOffNadir() {
return viewOffNadir;
}
/**
*
* The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*
*
* @return The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*/
public final ViewSunAzimuthInput viewSunAzimuth() {
return viewSunAzimuth;
}
/**
*
* The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*
*
* @return The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*/
public final ViewSunElevationInput viewSunElevation() {
return viewSunElevation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eoCloudCover());
hashCode = 31 * hashCode + Objects.hashCode(landsatCloudCoverLand());
hashCode = 31 * hashCode + Objects.hashCode(platform());
hashCode = 31 * hashCode + Objects.hashCode(viewOffNadir());
hashCode = 31 * hashCode + Objects.hashCode(viewSunAzimuth());
hashCode = 31 * hashCode + Objects.hashCode(viewSunElevation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Property)) {
return false;
}
Property other = (Property) obj;
return Objects.equals(eoCloudCover(), other.eoCloudCover())
&& Objects.equals(landsatCloudCoverLand(), other.landsatCloudCoverLand())
&& Objects.equals(platform(), other.platform()) && Objects.equals(viewOffNadir(), other.viewOffNadir())
&& Objects.equals(viewSunAzimuth(), other.viewSunAzimuth())
&& Objects.equals(viewSunElevation(), other.viewSunElevation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Property").add("EoCloudCover", eoCloudCover())
.add("LandsatCloudCoverLand", landsatCloudCoverLand()).add("Platform", platform())
.add("ViewOffNadir", viewOffNadir()).add("ViewSunAzimuth", viewSunAzimuth())
.add("ViewSunElevation", viewSunElevation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EoCloudCover":
return Optional.ofNullable(clazz.cast(eoCloudCover()));
case "LandsatCloudCoverLand":
return Optional.ofNullable(clazz.cast(landsatCloudCoverLand()));
case "Platform":
return Optional.ofNullable(clazz.cast(platform()));
case "ViewOffNadir":
return Optional.ofNullable(clazz.cast(viewOffNadir()));
case "ViewSunAzimuth":
return Optional.ofNullable(clazz.cast(viewSunAzimuth()));
case "ViewSunElevation":
return Optional.ofNullable(clazz.cast(viewSunElevation()));
default:
return Optional.empty();
}
}
/**
* Create an instance of this class with {@link #eoCloudCover()} initialized to the given value.
*
*
* The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*
*
* @param eoCloudCover
* The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*/
public static Property fromEoCloudCover(EoCloudCoverInput eoCloudCover) {
return builder().eoCloudCover(eoCloudCover).build();
}
/**
* Create an instance of this class with {@link #eoCloudCover()} initialized to the given value.
*
*
* The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*
*
* @param eoCloudCover
* The structure representing EoCloudCover property filter containing a lower bound and upper bound.
*/
public static Property fromEoCloudCover(Consumer eoCloudCover) {
EoCloudCoverInput.Builder builder = EoCloudCoverInput.builder();
eoCloudCover.accept(builder);
return fromEoCloudCover(builder.build());
}
/**
* Create an instance of this class with {@link #landsatCloudCoverLand()} initialized to the given value.
*
*
* The structure representing Land Cloud Cover property filter for Landsat collection containing a lower bound and
* upper bound.
*
*
* @param landsatCloudCoverLand
* The structure representing Land Cloud Cover property filter for Landsat collection containing a lower
* bound and upper bound.
*/
public static Property fromLandsatCloudCoverLand(LandsatCloudCoverLandInput landsatCloudCoverLand) {
return builder().landsatCloudCoverLand(landsatCloudCoverLand).build();
}
/**
* Create an instance of this class with {@link #landsatCloudCoverLand()} initialized to the given value.
*
*
* The structure representing Land Cloud Cover property filter for Landsat collection containing a lower bound and
* upper bound.
*
*
* @param landsatCloudCoverLand
* The structure representing Land Cloud Cover property filter for Landsat collection containing a lower
* bound and upper bound.
*/
public static Property fromLandsatCloudCoverLand(Consumer landsatCloudCoverLand) {
LandsatCloudCoverLandInput.Builder builder = LandsatCloudCoverLandInput.builder();
landsatCloudCoverLand.accept(builder);
return fromLandsatCloudCoverLand(builder.build());
}
/**
* Create an instance of this class with {@link #platform()} initialized to the given value.
*
*
* The structure representing Platform property filter consisting of value and comparison operator.
*
*
* @param platform
* The structure representing Platform property filter consisting of value and comparison operator.
*/
public static Property fromPlatform(PlatformInput platform) {
return builder().platform(platform).build();
}
/**
* Create an instance of this class with {@link #platform()} initialized to the given value.
*
*
* The structure representing Platform property filter consisting of value and comparison operator.
*
*
* @param platform
* The structure representing Platform property filter consisting of value and comparison operator.
*/
public static Property fromPlatform(Consumer platform) {
PlatformInput.Builder builder = PlatformInput.builder();
platform.accept(builder);
return fromPlatform(builder.build());
}
/**
* Create an instance of this class with {@link #viewOffNadir()} initialized to the given value.
*
*
* The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*
*
* @param viewOffNadir
* The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*/
public static Property fromViewOffNadir(ViewOffNadirInput viewOffNadir) {
return builder().viewOffNadir(viewOffNadir).build();
}
/**
* Create an instance of this class with {@link #viewOffNadir()} initialized to the given value.
*
*
* The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*
*
* @param viewOffNadir
* The structure representing ViewOffNadir property filter containing a lower bound and upper bound.
*/
public static Property fromViewOffNadir(Consumer viewOffNadir) {
ViewOffNadirInput.Builder builder = ViewOffNadirInput.builder();
viewOffNadir.accept(builder);
return fromViewOffNadir(builder.build());
}
/**
* Create an instance of this class with {@link #viewSunAzimuth()} initialized to the given value.
*
*
* The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*
*
* @param viewSunAzimuth
* The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*/
public static Property fromViewSunAzimuth(ViewSunAzimuthInput viewSunAzimuth) {
return builder().viewSunAzimuth(viewSunAzimuth).build();
}
/**
* Create an instance of this class with {@link #viewSunAzimuth()} initialized to the given value.
*
*
* The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*
*
* @param viewSunAzimuth
* The structure representing ViewSunAzimuth property filter containing a lower bound and upper bound.
*/
public static Property fromViewSunAzimuth(Consumer viewSunAzimuth) {
ViewSunAzimuthInput.Builder builder = ViewSunAzimuthInput.builder();
viewSunAzimuth.accept(builder);
return fromViewSunAzimuth(builder.build());
}
/**
* Create an instance of this class with {@link #viewSunElevation()} initialized to the given value.
*
*
* The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*
*
* @param viewSunElevation
* The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*/
public static Property fromViewSunElevation(ViewSunElevationInput viewSunElevation) {
return builder().viewSunElevation(viewSunElevation).build();
}
/**
* Create an instance of this class with {@link #viewSunElevation()} initialized to the given value.
*
*
* The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*
*
* @param viewSunElevation
* The structure representing ViewSunElevation property filter containing a lower bound and upper bound.
*/
public static Property fromViewSunElevation(Consumer viewSunElevation) {
ViewSunElevationInput.Builder builder = ViewSunElevationInput.builder();
viewSunElevation.accept(builder);
return fromViewSunElevation(builder.build());
}
/**
* Retrieve an enum value representing which member of this object is populated.
*
* When this class is returned in a service response, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if the
* service returned a member that is only known to a newer SDK version.
*
* When this class is created directly in your code, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if zero
* members are set, and {@code null} if more than one member is set.
*/
public Type type() {
return type;
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function