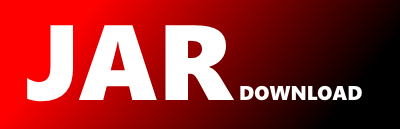
software.amazon.awssdk.services.sagemakergeospatial.SageMakerGeospatialAsyncClient Maven / Gradle / Ivy
Show all versions of sagemakergeospatial Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakergeospatial;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetTileResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceRequest;
import software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceResponse;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsPublisher;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsPublisher;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsPublisher;
import software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionPublisher;
/**
* Service client for accessing Amazon SageMaker geospatial capabilities asynchronously. This can be created using the
* static {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Provides APIs for creating and managing SageMaker geospatial resources.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SageMakerGeospatialAsyncClient extends AwsClient {
String SERVICE_NAME = "sagemaker-geospatial";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "sagemaker-geospatial";
/**
*
* Use this operation to delete an Earth Observation job.
*
*
* @param deleteEarthObservationJobRequest
* @return A Java Future containing the result of the DeleteEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.DeleteEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture deleteEarthObservationJob(
DeleteEarthObservationJobRequest deleteEarthObservationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to delete an Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link DeleteEarthObservationJobRequest#builder()}
*
*
* @param deleteEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.DeleteEarthObservationJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DeleteEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.DeleteEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture deleteEarthObservationJob(
Consumer deleteEarthObservationJobRequest) {
return deleteEarthObservationJob(DeleteEarthObservationJobRequest.builder()
.applyMutation(deleteEarthObservationJobRequest).build());
}
/**
*
* Use this operation to delete a Vector Enrichment job.
*
*
* @param deleteVectorEnrichmentJobRequest
* @return A Java Future containing the result of the DeleteVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.DeleteVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture deleteVectorEnrichmentJob(
DeleteVectorEnrichmentJobRequest deleteVectorEnrichmentJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to delete a Vector Enrichment job.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link DeleteVectorEnrichmentJobRequest#builder()}
*
*
* @param deleteVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.DeleteVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DeleteVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.DeleteVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture deleteVectorEnrichmentJob(
Consumer deleteVectorEnrichmentJobRequest) {
return deleteVectorEnrichmentJob(DeleteVectorEnrichmentJobRequest.builder()
.applyMutation(deleteVectorEnrichmentJobRequest).build());
}
/**
*
* Use this operation to export results of an Earth Observation job and optionally source images used as input to
* the EOJ to an Amazon S3 location.
*
*
* @param exportEarthObservationJobRequest
* @return A Java Future containing the result of the ExportEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ExportEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture exportEarthObservationJob(
ExportEarthObservationJobRequest exportEarthObservationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to export results of an Earth Observation job and optionally source images used as input to
* the EOJ to an Amazon S3 location.
*
*
*
* This is a convenience which creates an instance of the {@link ExportEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link ExportEarthObservationJobRequest#builder()}
*
*
* @param exportEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ExportEarthObservationJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ExportEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ExportEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture exportEarthObservationJob(
Consumer exportEarthObservationJobRequest) {
return exportEarthObservationJob(ExportEarthObservationJobRequest.builder()
.applyMutation(exportEarthObservationJobRequest).build());
}
/**
*
* Use this operation to copy results of a Vector Enrichment job to an Amazon S3 location.
*
*
* @param exportVectorEnrichmentJobRequest
* @return A Java Future containing the result of the ExportVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ExportVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture exportVectorEnrichmentJob(
ExportVectorEnrichmentJobRequest exportVectorEnrichmentJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to copy results of a Vector Enrichment job to an Amazon S3 location.
*
*
*
* This is a convenience which creates an instance of the {@link ExportVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link ExportVectorEnrichmentJobRequest#builder()}
*
*
* @param exportVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ExportVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ExportVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ExportVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture exportVectorEnrichmentJob(
Consumer exportVectorEnrichmentJobRequest) {
return exportVectorEnrichmentJob(ExportVectorEnrichmentJobRequest.builder()
.applyMutation(exportVectorEnrichmentJobRequest).build());
}
/**
*
* Get the details for a previously initiated Earth Observation job.
*
*
* @param getEarthObservationJobRequest
* @return A Java Future containing the result of the GetEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture getEarthObservationJob(
GetEarthObservationJobRequest getEarthObservationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get the details for a previously initiated Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetEarthObservationJobRequest.Builder} avoiding the
* need to create one manually via {@link GetEarthObservationJobRequest#builder()}
*
*
* @param getEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetEarthObservationJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture getEarthObservationJob(
Consumer getEarthObservationJobRequest) {
return getEarthObservationJob(GetEarthObservationJobRequest.builder().applyMutation(getEarthObservationJobRequest)
.build());
}
/**
*
* Use this operation to get details of a specific raster data collection.
*
*
* @param getRasterDataCollectionRequest
* @return A Java Future containing the result of the GetRasterDataCollection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetRasterDataCollection
* @see AWS API Documentation
*/
default CompletableFuture getRasterDataCollection(
GetRasterDataCollectionRequest getRasterDataCollectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get details of a specific raster data collection.
*
*
*
* This is a convenience which creates an instance of the {@link GetRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link GetRasterDataCollectionRequest#builder()}
*
*
* @param getRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetRasterDataCollectionRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetRasterDataCollection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetRasterDataCollection
* @see AWS API Documentation
*/
default CompletableFuture getRasterDataCollection(
Consumer getRasterDataCollectionRequest) {
return getRasterDataCollection(GetRasterDataCollectionRequest.builder().applyMutation(getRasterDataCollectionRequest)
.build());
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The output binary file.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetTile
* @see AWS
* API Documentation
*/
default CompletableFuture getTile(GetTileRequest getTileRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The output binary file.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetTile
* @see AWS
* API Documentation
*/
default CompletableFuture getTile(Consumer getTileRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getTile(GetTileRequest.builder().applyMutation(getTileRequest).build(), asyncResponseTransformer);
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
* @param getTileRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetTile
* @see AWS
* API Documentation
*/
default CompletableFuture getTile(GetTileRequest getTileRequest, Path destinationPath) {
return getTile(getTileRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Gets a web mercator tile for the given Earth Observation job.
*
*
*
* This is a convenience which creates an instance of the {@link GetTileRequest.Builder} avoiding the need to create
* one manually via {@link GetTileRequest#builder()}
*
*
* @param getTileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetTileRequest.Builder} to create a
* request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The output binary file.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetTile
* @see AWS
* API Documentation
*/
default CompletableFuture getTile(Consumer getTileRequest, Path destinationPath) {
return getTile(GetTileRequest.builder().applyMutation(getTileRequest).build(), destinationPath);
}
/**
*
* Retrieves details of a Vector Enrichment Job for a given job Amazon Resource Name (ARN).
*
*
* @param getVectorEnrichmentJobRequest
* @return A Java Future containing the result of the GetVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture getVectorEnrichmentJob(
GetVectorEnrichmentJobRequest getVectorEnrichmentJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details of a Vector Enrichment Job for a given job Amazon Resource Name (ARN).
*
*
*
* This is a convenience which creates an instance of the {@link GetVectorEnrichmentJobRequest.Builder} avoiding the
* need to create one manually via {@link GetVectorEnrichmentJobRequest#builder()}
*
*
* @param getVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.GetVectorEnrichmentJobRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.GetVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture getVectorEnrichmentJob(
Consumer getVectorEnrichmentJobRequest) {
return getVectorEnrichmentJob(GetVectorEnrichmentJobRequest.builder().applyMutation(getVectorEnrichmentJobRequest)
.build());
}
/**
*
* Use this operation to get a list of the Earth Observation jobs associated with the calling Amazon Web Services
* account.
*
*
* @param listEarthObservationJobsRequest
* @return A Java Future containing the result of the ListEarthObservationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default CompletableFuture listEarthObservationJobs(
ListEarthObservationJobsRequest listEarthObservationJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get a list of the Earth Observation jobs associated with the calling Amazon Web Services
* account.
*
*
*
* This is a convenience which creates an instance of the {@link ListEarthObservationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListEarthObservationJobsRequest#builder()}
*
*
* @param listEarthObservationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListEarthObservationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default CompletableFuture listEarthObservationJobs(
Consumer listEarthObservationJobsRequest) {
return listEarthObservationJobs(ListEarthObservationJobsRequest.builder().applyMutation(listEarthObservationJobsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsPublisher publisher = client.listEarthObservationJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsPublisher publisher = client.listEarthObservationJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation.
*
*
* @param listEarthObservationJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsPublisher listEarthObservationJobsPaginator(
ListEarthObservationJobsRequest listEarthObservationJobsRequest) {
return new ListEarthObservationJobsPublisher(this, listEarthObservationJobsRequest);
}
/**
*
* This is a variant of
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsPublisher publisher = client.listEarthObservationJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListEarthObservationJobsPublisher publisher = client.listEarthObservationJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEarthObservationJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListEarthObservationJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListEarthObservationJobsRequest#builder()}
*
*
* @param listEarthObservationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListEarthObservationJobsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListEarthObservationJobs
* @see AWS API Documentation
*/
default ListEarthObservationJobsPublisher listEarthObservationJobsPaginator(
Consumer listEarthObservationJobsRequest) {
return listEarthObservationJobsPaginator(ListEarthObservationJobsRequest.builder()
.applyMutation(listEarthObservationJobsRequest).build());
}
/**
*
* Use this operation to get raster data collections.
*
*
* @param listRasterDataCollectionsRequest
* @return A Java Future containing the result of the ListRasterDataCollections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default CompletableFuture listRasterDataCollections(
ListRasterDataCollectionsRequest listRasterDataCollectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to get raster data collections.
*
*
*
* This is a convenience which creates an instance of the {@link ListRasterDataCollectionsRequest.Builder} avoiding
* the need to create one manually via {@link ListRasterDataCollectionsRequest#builder()}
*
*
* @param listRasterDataCollectionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListRasterDataCollections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default CompletableFuture listRasterDataCollections(
Consumer listRasterDataCollectionsRequest) {
return listRasterDataCollections(ListRasterDataCollectionsRequest.builder()
.applyMutation(listRasterDataCollectionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsPublisher publisher = client.listRasterDataCollectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsPublisher publisher = client.listRasterDataCollectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation.
*
*
* @param listRasterDataCollectionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsPublisher listRasterDataCollectionsPaginator(
ListRasterDataCollectionsRequest listRasterDataCollectionsRequest) {
return new ListRasterDataCollectionsPublisher(this, listRasterDataCollectionsRequest);
}
/**
*
* This is a variant of
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsPublisher publisher = client.listRasterDataCollectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListRasterDataCollectionsPublisher publisher = client.listRasterDataCollectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRasterDataCollections(software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRasterDataCollectionsRequest.Builder} avoiding
* the need to create one manually via {@link ListRasterDataCollectionsRequest#builder()}
*
*
* @param listRasterDataCollectionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListRasterDataCollectionsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListRasterDataCollections
* @see AWS API Documentation
*/
default ListRasterDataCollectionsPublisher listRasterDataCollectionsPaginator(
Consumer listRasterDataCollectionsRequest) {
return listRasterDataCollectionsPaginator(ListRasterDataCollectionsRequest.builder()
.applyMutation(listRasterDataCollectionsRequest).build());
}
/**
*
* Lists the tags attached to the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags attached to the resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListTagsForResourceRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Retrieves a list of vector enrichment jobs.
*
*
* @param listVectorEnrichmentJobsRequest
* @return A Java Future containing the result of the ListVectorEnrichmentJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default CompletableFuture listVectorEnrichmentJobs(
ListVectorEnrichmentJobsRequest listVectorEnrichmentJobsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of vector enrichment jobs.
*
*
*
* This is a convenience which creates an instance of the {@link ListVectorEnrichmentJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListVectorEnrichmentJobsRequest#builder()}
*
*
* @param listVectorEnrichmentJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListVectorEnrichmentJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default CompletableFuture listVectorEnrichmentJobs(
Consumer listVectorEnrichmentJobsRequest) {
return listVectorEnrichmentJobs(ListVectorEnrichmentJobsRequest.builder().applyMutation(listVectorEnrichmentJobsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsPublisher publisher = client.listVectorEnrichmentJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsPublisher publisher = client.listVectorEnrichmentJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation.
*
*
* @param listVectorEnrichmentJobsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsPublisher listVectorEnrichmentJobsPaginator(
ListVectorEnrichmentJobsRequest listVectorEnrichmentJobsRequest) {
return new ListVectorEnrichmentJobsPublisher(this, listVectorEnrichmentJobsRequest);
}
/**
*
* This is a variant of
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsPublisher publisher = client.listVectorEnrichmentJobsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.ListVectorEnrichmentJobsPublisher publisher = client.listVectorEnrichmentJobsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVectorEnrichmentJobs(software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListVectorEnrichmentJobsRequest.Builder} avoiding
* the need to create one manually via {@link ListVectorEnrichmentJobsRequest#builder()}
*
*
* @param listVectorEnrichmentJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.ListVectorEnrichmentJobsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.ListVectorEnrichmentJobs
* @see AWS API Documentation
*/
default ListVectorEnrichmentJobsPublisher listVectorEnrichmentJobsPaginator(
Consumer listVectorEnrichmentJobsRequest) {
return listVectorEnrichmentJobsPaginator(ListVectorEnrichmentJobsRequest.builder()
.applyMutation(listVectorEnrichmentJobsRequest).build());
}
/**
*
* Allows you run image query on a specific raster data collection to get a list of the satellite imagery matching
* the selected filters.
*
*
* @param searchRasterDataCollectionRequest
* @return A Java Future containing the result of the SearchRasterDataCollection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default CompletableFuture searchRasterDataCollection(
SearchRasterDataCollectionRequest searchRasterDataCollectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows you run image query on a specific raster data collection to get a list of the satellite imagery matching
* the selected filters.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link SearchRasterDataCollectionRequest#builder()}
*
*
* @param searchRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the SearchRasterDataCollection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default CompletableFuture searchRasterDataCollection(
Consumer searchRasterDataCollectionRequest) {
return searchRasterDataCollection(SearchRasterDataCollectionRequest.builder()
.applyMutation(searchRasterDataCollectionRequest).build());
}
/**
*
* This is a variant of
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionPublisher publisher = client.searchRasterDataCollectionPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionPublisher publisher = client.searchRasterDataCollectionPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation.
*
*
* @param searchRasterDataCollectionRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionPublisher searchRasterDataCollectionPaginator(
SearchRasterDataCollectionRequest searchRasterDataCollectionRequest) {
return new SearchRasterDataCollectionPublisher(this, searchRasterDataCollectionRequest);
}
/**
*
* This is a variant of
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionPublisher publisher = client.searchRasterDataCollectionPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sagemakergeospatial.paginators.SearchRasterDataCollectionPublisher publisher = client.searchRasterDataCollectionPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchRasterDataCollection(software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRasterDataCollectionRequest.Builder} avoiding
* the need to create one manually via {@link SearchRasterDataCollectionRequest#builder()}
*
*
* @param searchRasterDataCollectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.SearchRasterDataCollectionRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.SearchRasterDataCollection
* @see AWS API Documentation
*/
default SearchRasterDataCollectionPublisher searchRasterDataCollectionPaginator(
Consumer searchRasterDataCollectionRequest) {
return searchRasterDataCollectionPaginator(SearchRasterDataCollectionRequest.builder()
.applyMutation(searchRasterDataCollectionRequest).build());
}
/**
*
* Use this operation to create an Earth observation job.
*
*
* @param startEarthObservationJobRequest
* @return A Java Future containing the result of the StartEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StartEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture startEarthObservationJob(
StartEarthObservationJobRequest startEarthObservationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to create an Earth observation job.
*
*
*
* This is a convenience which creates an instance of the {@link StartEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link StartEarthObservationJobRequest#builder()}
*
*
* @param startEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StartEarthObservationJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StartEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StartEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture startEarthObservationJob(
Consumer startEarthObservationJobRequest) {
return startEarthObservationJob(StartEarthObservationJobRequest.builder().applyMutation(startEarthObservationJobRequest)
.build());
}
/**
*
* Creates a Vector Enrichment job for the supplied job type. Currently, there are two supported job types: reverse
* geocoding and map matching.
*
*
* @param startVectorEnrichmentJobRequest
* @return A Java Future containing the result of the StartVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StartVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture startVectorEnrichmentJob(
StartVectorEnrichmentJobRequest startVectorEnrichmentJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Vector Enrichment job for the supplied job type. Currently, there are two supported job types: reverse
* geocoding and map matching.
*
*
*
* This is a convenience which creates an instance of the {@link StartVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link StartVectorEnrichmentJobRequest#builder()}
*
*
* @param startVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StartVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StartVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException You have exceeded the service quota.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StartVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture startVectorEnrichmentJob(
Consumer startVectorEnrichmentJobRequest) {
return startVectorEnrichmentJob(StartVectorEnrichmentJobRequest.builder().applyMutation(startVectorEnrichmentJobRequest)
.build());
}
/**
*
* Use this operation to stop an existing earth observation job.
*
*
* @param stopEarthObservationJobRequest
* @return A Java Future containing the result of the StopEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StopEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture stopEarthObservationJob(
StopEarthObservationJobRequest stopEarthObservationJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use this operation to stop an existing earth observation job.
*
*
*
* This is a convenience which creates an instance of the {@link StopEarthObservationJobRequest.Builder} avoiding
* the need to create one manually via {@link StopEarthObservationJobRequest#builder()}
*
*
* @param stopEarthObservationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StopEarthObservationJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StopEarthObservationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StopEarthObservationJob
* @see AWS API Documentation
*/
default CompletableFuture stopEarthObservationJob(
Consumer stopEarthObservationJobRequest) {
return stopEarthObservationJob(StopEarthObservationJobRequest.builder().applyMutation(stopEarthObservationJobRequest)
.build());
}
/**
*
* Stops the Vector Enrichment job for a given job ARN.
*
*
* @param stopVectorEnrichmentJobRequest
* @return A Java Future containing the result of the StopVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StopVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture stopVectorEnrichmentJob(
StopVectorEnrichmentJobRequest stopVectorEnrichmentJobRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops the Vector Enrichment job for a given job ARN.
*
*
*
* This is a convenience which creates an instance of the {@link StopVectorEnrichmentJobRequest.Builder} avoiding
* the need to create one manually via {@link StopVectorEnrichmentJobRequest#builder()}
*
*
* @param stopVectorEnrichmentJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.StopVectorEnrichmentJobRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StopVectorEnrichmentJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - ConflictException Updating or deleting a resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.StopVectorEnrichmentJob
* @see AWS API Documentation
*/
default CompletableFuture stopVectorEnrichmentJob(
Consumer stopVectorEnrichmentJobRequest) {
return stopVectorEnrichmentJob(StopVectorEnrichmentJobRequest.builder().applyMutation(stopVectorEnrichmentJobRequest)
.build());
}
/**
*
* The resource you want to tag.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The resource you want to tag.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* The resource you want to untag.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The resource you want to untag.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sagemakergeospatial.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request processing has failed because of an unknown error, exception, or
* failure.
* - ResourceNotFoundException The request references a resource which does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerGeospatialException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample SageMakerGeospatialAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
@Override
default SageMakerGeospatialServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link SageMakerGeospatialAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SageMakerGeospatialAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SageMakerGeospatialAsyncClient}.
*/
static SageMakerGeospatialAsyncClientBuilder builder() {
return new DefaultSageMakerGeospatialAsyncClientBuilder();
}
}