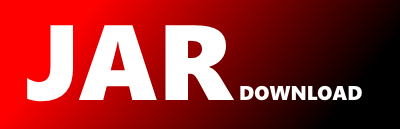
software.amazon.awssdk.services.sagemakergeospatial.model.RasterDataCollectionQueryOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemakergeospatial Show documentation
Show all versions of sagemakergeospatial Show documentation
The AWS Java SDK for Sage Maker Geospatial module holds the client classes that are used for
communicating with Sage Maker Geospatial.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakergeospatial.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The output structure contains the Raster Data Collection Query input along with some additional metadata.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RasterDataCollectionQueryOutput implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AREA_OF_INTEREST_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AreaOfInterest")
.getter(getter(RasterDataCollectionQueryOutput::areaOfInterest)).setter(setter(Builder::areaOfInterest))
.constructor(AreaOfInterest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AreaOfInterest").build()).build();
private static final SdkField PROPERTY_FILTERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PropertyFilters")
.getter(getter(RasterDataCollectionQueryOutput::propertyFilters)).setter(setter(Builder::propertyFilters))
.constructor(PropertyFilters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PropertyFilters").build()).build();
private static final SdkField RASTER_DATA_COLLECTION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RasterDataCollectionArn").getter(getter(RasterDataCollectionQueryOutput::rasterDataCollectionArn))
.setter(setter(Builder::rasterDataCollectionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RasterDataCollectionArn").build())
.build();
private static final SdkField RASTER_DATA_COLLECTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RasterDataCollectionName").getter(getter(RasterDataCollectionQueryOutput::rasterDataCollectionName))
.setter(setter(Builder::rasterDataCollectionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RasterDataCollectionName").build())
.build();
private static final SdkField TIME_RANGE_FILTER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TimeRangeFilter")
.getter(getter(RasterDataCollectionQueryOutput::timeRangeFilter)).setter(setter(Builder::timeRangeFilter))
.constructor(TimeRangeFilterOutput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeRangeFilter").build()).build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(AREA_OF_INTEREST_FIELD, PROPERTY_FILTERS_FIELD, RASTER_DATA_COLLECTION_ARN_FIELD,
RASTER_DATA_COLLECTION_NAME_FIELD, TIME_RANGE_FILTER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final AreaOfInterest areaOfInterest;
private final PropertyFilters propertyFilters;
private final String rasterDataCollectionArn;
private final String rasterDataCollectionName;
private final TimeRangeFilterOutput timeRangeFilter;
private RasterDataCollectionQueryOutput(BuilderImpl builder) {
this.areaOfInterest = builder.areaOfInterest;
this.propertyFilters = builder.propertyFilters;
this.rasterDataCollectionArn = builder.rasterDataCollectionArn;
this.rasterDataCollectionName = builder.rasterDataCollectionName;
this.timeRangeFilter = builder.timeRangeFilter;
}
/**
*
* The Area of Interest used in the search.
*
*
* @return The Area of Interest used in the search.
*/
public final AreaOfInterest areaOfInterest() {
return areaOfInterest;
}
/**
*
* Property filters used in the search.
*
*
* @return Property filters used in the search.
*/
public final PropertyFilters propertyFilters() {
return propertyFilters;
}
/**
*
* The ARN of the Raster Data Collection against which the search is done.
*
*
* @return The ARN of the Raster Data Collection against which the search is done.
*/
public final String rasterDataCollectionArn() {
return rasterDataCollectionArn;
}
/**
*
* The name of the raster data collection.
*
*
* @return The name of the raster data collection.
*/
public final String rasterDataCollectionName() {
return rasterDataCollectionName;
}
/**
*
* The TimeRange filter used in the search.
*
*
* @return The TimeRange filter used in the search.
*/
public final TimeRangeFilterOutput timeRangeFilter() {
return timeRangeFilter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(areaOfInterest());
hashCode = 31 * hashCode + Objects.hashCode(propertyFilters());
hashCode = 31 * hashCode + Objects.hashCode(rasterDataCollectionArn());
hashCode = 31 * hashCode + Objects.hashCode(rasterDataCollectionName());
hashCode = 31 * hashCode + Objects.hashCode(timeRangeFilter());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RasterDataCollectionQueryOutput)) {
return false;
}
RasterDataCollectionQueryOutput other = (RasterDataCollectionQueryOutput) obj;
return Objects.equals(areaOfInterest(), other.areaOfInterest())
&& Objects.equals(propertyFilters(), other.propertyFilters())
&& Objects.equals(rasterDataCollectionArn(), other.rasterDataCollectionArn())
&& Objects.equals(rasterDataCollectionName(), other.rasterDataCollectionName())
&& Objects.equals(timeRangeFilter(), other.timeRangeFilter());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RasterDataCollectionQueryOutput").add("AreaOfInterest", areaOfInterest())
.add("PropertyFilters", propertyFilters()).add("RasterDataCollectionArn", rasterDataCollectionArn())
.add("RasterDataCollectionName", rasterDataCollectionName())
.add("TimeRangeFilter", timeRangeFilter() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AreaOfInterest":
return Optional.ofNullable(clazz.cast(areaOfInterest()));
case "PropertyFilters":
return Optional.ofNullable(clazz.cast(propertyFilters()));
case "RasterDataCollectionArn":
return Optional.ofNullable(clazz.cast(rasterDataCollectionArn()));
case "RasterDataCollectionName":
return Optional.ofNullable(clazz.cast(rasterDataCollectionName()));
case "TimeRangeFilter":
return Optional.ofNullable(clazz.cast(timeRangeFilter()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("AreaOfInterest", AREA_OF_INTEREST_FIELD);
map.put("PropertyFilters", PROPERTY_FILTERS_FIELD);
map.put("RasterDataCollectionArn", RASTER_DATA_COLLECTION_ARN_FIELD);
map.put("RasterDataCollectionName", RASTER_DATA_COLLECTION_NAME_FIELD);
map.put("TimeRangeFilter", TIME_RANGE_FILTER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy