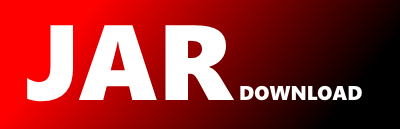
software.amazon.awssdk.services.sagemakerruntime.SageMakerRuntimeClient Maven / Gradle / Ivy
Show all versions of sagemakerruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerruntime;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.sagemakerruntime.model.InternalFailureException;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointRequest;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointResponse;
import software.amazon.awssdk.services.sagemakerruntime.model.ModelErrorException;
import software.amazon.awssdk.services.sagemakerruntime.model.SageMakerRuntimeException;
import software.amazon.awssdk.services.sagemakerruntime.model.ServiceUnavailableException;
import software.amazon.awssdk.services.sagemakerruntime.model.ValidationErrorException;
/**
* Service client for accessing Amazon SageMaker Runtime. This can be created using the static {@link #builder()}
* method.
*
*
* The Amazon SageMaker runtime API.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SageMakerRuntimeClient extends SdkClient {
String SERVICE_NAME = "sagemaker";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "runtime.sagemaker";
/**
* Create a {@link SageMakerRuntimeClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SageMakerRuntimeClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SageMakerRuntimeClient}.
*/
static SageMakerRuntimeClientBuilder builder() {
return new DefaultSageMakerRuntimeClientBuilder();
}
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint.
*
*
* For an overview of Amazon SageMaker, see How It Works.
*
*
* Amazon SageMaker strips all POST headers except those supported by the API. Amazon SageMaker might add additional
* headers. You should not rely on the behavior of headers outside those enumerated in the request syntax.
*
*
* Calls to InvokeEndpoint
are authenticated by using AWS Signature Version 4. For information, see Authenticating
* Requests (AWS Signature Version 4) in the Amazon S3 API Reference.
*
*
* A customer's model containers must respond to requests within 60 seconds. The model itself can have a maximum
* processing time of 60 seconds before responding to invocations. If your model is going to take 50-60 seconds of
* processing time, the SDK socket timeout should be set to be 70 seconds.
*
*
*
* Endpoints are scoped to an individual account, and are not public. The URL does not contain the account ID, but
* Amazon SageMaker determines the account ID from the authentication token that is supplied by the caller.
*
*
*
* @param invokeEndpointRequest
* @return Result of the InvokeEndpoint operation returned by the service.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is unavailable. Try your call again.
* @throws ValidationErrorException
* Inspect your request and try again.
* @throws ModelErrorException
* Model (owned by the customer in the container) returned 4xx or 5xx error code.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerRuntimeClient.InvokeEndpoint
* @see AWS API Documentation
*/
default InvokeEndpointResponse invokeEndpoint(InvokeEndpointRequest invokeEndpointRequest) throws InternalFailureException,
ServiceUnavailableException, ValidationErrorException, ModelErrorException, AwsServiceException, SdkClientException,
SageMakerRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint.
*
*
* For an overview of Amazon SageMaker, see How It Works.
*
*
* Amazon SageMaker strips all POST headers except those supported by the API. Amazon SageMaker might add additional
* headers. You should not rely on the behavior of headers outside those enumerated in the request syntax.
*
*
* Calls to InvokeEndpoint
are authenticated by using AWS Signature Version 4. For information, see Authenticating
* Requests (AWS Signature Version 4) in the Amazon S3 API Reference.
*
*
* A customer's model containers must respond to requests within 60 seconds. The model itself can have a maximum
* processing time of 60 seconds before responding to invocations. If your model is going to take 50-60 seconds of
* processing time, the SDK socket timeout should be set to be 70 seconds.
*
*
*
* Endpoints are scoped to an individual account, and are not public. The URL does not contain the account ID, but
* Amazon SageMaker determines the account ID from the authentication token that is supplied by the caller.
*
*
*
* This is a convenience which creates an instance of the {@link InvokeEndpointRequest.Builder} avoiding the need to
* create one manually via {@link InvokeEndpointRequest#builder()}
*
*
* @param invokeEndpointRequest
* A {@link Consumer} that will call methods on {@link InvokeEndpointInput.Builder} to create a request.
* @return Result of the InvokeEndpoint operation returned by the service.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is unavailable. Try your call again.
* @throws ValidationErrorException
* Inspect your request and try again.
* @throws ModelErrorException
* Model (owned by the customer in the container) returned 4xx or 5xx error code.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SageMakerRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SageMakerRuntimeClient.InvokeEndpoint
* @see AWS API Documentation
*/
default InvokeEndpointResponse invokeEndpoint(Consumer invokeEndpointRequest)
throws InternalFailureException, ServiceUnavailableException, ValidationErrorException, ModelErrorException,
AwsServiceException, SdkClientException, SageMakerRuntimeException {
return invokeEndpoint(InvokeEndpointRequest.builder().applyMutation(invokeEndpointRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}