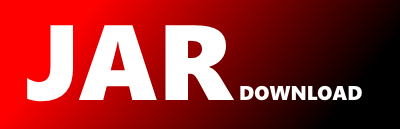
software.amazon.awssdk.services.sagemakerruntime.SageMakerRuntimeAsyncClient Maven / Gradle / Ivy
Show all versions of sagemakerruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerruntime;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointAsyncRequest;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointAsyncResponse;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointRequest;
import software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointResponse;
/**
* Service client for accessing Amazon SageMaker Runtime asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* The Amazon SageMaker runtime API.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SageMakerRuntimeAsyncClient extends AwsClient {
String SERVICE_NAME = "sagemaker";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "runtime.sagemaker";
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint.
*
*
* For an overview of Amazon SageMaker, see How It Works.
*
*
* Amazon SageMaker strips all POST headers except those supported by the API. Amazon SageMaker might add additional
* headers. You should not rely on the behavior of headers outside those enumerated in the request syntax.
*
*
* Calls to InvokeEndpoint
are authenticated by using Amazon Web Services Signature Version 4. For
* information, see Authenticating
* Requests (Amazon Web Services Signature Version 4) in the Amazon S3 API Reference.
*
*
* A customer's model containers must respond to requests within 60 seconds. The model itself can have a maximum
* processing time of 60 seconds before responding to invocations. If your model is going to take 50-60 seconds of
* processing time, the SDK socket timeout should be set to be 70 seconds.
*
*
*
* Endpoints are scoped to an individual account, and are not public. The URL does not contain the account ID, but
* Amazon SageMaker determines the account ID from the authentication token that is supplied by the caller.
*
*
*
* @param invokeEndpointRequest
* @return A Java Future containing the result of the InvokeEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalFailureException An internal failure occurred.
* - ServiceUnavailableException The service is unavailable. Try your call again.
* - ValidationErrorException Inspect your request and try again.
* - ModelErrorException Model (owned by the customer in the container) returned 4xx or 5xx error code.
* - InternalDependencyException Your request caused an exception with an internal dependency. Contact
* customer support.
* - ModelNotReadyException Either a serverless endpoint variant's resources are still being provisioned,
* or a multi-model endpoint is still downloading or loading the target model. Wait and try your request
* again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample SageMakerRuntimeAsyncClient.InvokeEndpoint
* @see AWS API Documentation
*/
default CompletableFuture invokeEndpoint(InvokeEndpointRequest invokeEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint.
*
*
* For an overview of Amazon SageMaker, see How It Works.
*
*
* Amazon SageMaker strips all POST headers except those supported by the API. Amazon SageMaker might add additional
* headers. You should not rely on the behavior of headers outside those enumerated in the request syntax.
*
*
* Calls to InvokeEndpoint
are authenticated by using Amazon Web Services Signature Version 4. For
* information, see Authenticating
* Requests (Amazon Web Services Signature Version 4) in the Amazon S3 API Reference.
*
*
* A customer's model containers must respond to requests within 60 seconds. The model itself can have a maximum
* processing time of 60 seconds before responding to invocations. If your model is going to take 50-60 seconds of
* processing time, the SDK socket timeout should be set to be 70 seconds.
*
*
*
* Endpoints are scoped to an individual account, and are not public. The URL does not contain the account ID, but
* Amazon SageMaker determines the account ID from the authentication token that is supplied by the caller.
*
*
*
* This is a convenience which creates an instance of the {@link InvokeEndpointRequest.Builder} avoiding the need to
* create one manually via {@link InvokeEndpointRequest#builder()}
*
*
* @param invokeEndpointRequest
* A {@link Consumer} that will call methods on {@link InvokeEndpointInput.Builder} to create a request.
* @return A Java Future containing the result of the InvokeEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalFailureException An internal failure occurred.
* - ServiceUnavailableException The service is unavailable. Try your call again.
* - ValidationErrorException Inspect your request and try again.
* - ModelErrorException Model (owned by the customer in the container) returned 4xx or 5xx error code.
* - InternalDependencyException Your request caused an exception with an internal dependency. Contact
* customer support.
* - ModelNotReadyException Either a serverless endpoint variant's resources are still being provisioned,
* or a multi-model endpoint is still downloading or loading the target model. Wait and try your request
* again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample SageMakerRuntimeAsyncClient.InvokeEndpoint
* @see AWS API Documentation
*/
default CompletableFuture invokeEndpoint(Consumer invokeEndpointRequest) {
return invokeEndpoint(InvokeEndpointRequest.builder().applyMutation(invokeEndpointRequest).build());
}
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint in an asynchronous manner.
*
*
* Inference requests sent to this API are enqueued for asynchronous processing. The processing of the inference
* request may or may not complete before you receive a response from this API. The response from this API will not
* contain the result of the inference request but contain information about where you can locate it.
*
*
* Amazon SageMaker strips all POST
headers except those supported by the API. Amazon SageMaker might
* add additional headers. You should not rely on the behavior of headers outside those enumerated in the request
* syntax.
*
*
* Calls to InvokeEndpointAsync
are authenticated by using Amazon Web Services Signature Version 4. For
* information, see Authenticating
* Requests (Amazon Web Services Signature Version 4) in the Amazon S3 API Reference.
*
*
* @param invokeEndpointAsyncRequest
* @return A Java Future containing the result of the InvokeEndpointAsync operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalFailureException An internal failure occurred.
* - ServiceUnavailableException The service is unavailable. Try your call again.
* - ValidationErrorException Inspect your request and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample SageMakerRuntimeAsyncClient.InvokeEndpointAsync
* @see AWS API Documentation
*/
default CompletableFuture invokeEndpointAsync(
InvokeEndpointAsyncRequest invokeEndpointAsyncRequest) {
throw new UnsupportedOperationException();
}
/**
*
* After you deploy a model into production using Amazon SageMaker hosting services, your client applications use
* this API to get inferences from the model hosted at the specified endpoint in an asynchronous manner.
*
*
* Inference requests sent to this API are enqueued for asynchronous processing. The processing of the inference
* request may or may not complete before you receive a response from this API. The response from this API will not
* contain the result of the inference request but contain information about where you can locate it.
*
*
* Amazon SageMaker strips all POST
headers except those supported by the API. Amazon SageMaker might
* add additional headers. You should not rely on the behavior of headers outside those enumerated in the request
* syntax.
*
*
* Calls to InvokeEndpointAsync
are authenticated by using Amazon Web Services Signature Version 4. For
* information, see Authenticating
* Requests (Amazon Web Services Signature Version 4) in the Amazon S3 API Reference.
*
*
*
* This is a convenience which creates an instance of the {@link InvokeEndpointAsyncRequest.Builder} avoiding the
* need to create one manually via {@link InvokeEndpointAsyncRequest#builder()}
*
*
* @param invokeEndpointAsyncRequest
* A {@link Consumer} that will call methods on {@link InvokeEndpointAsyncInput.Builder} to create a request.
* @return A Java Future containing the result of the InvokeEndpointAsync operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalFailureException An internal failure occurred.
* - ServiceUnavailableException The service is unavailable. Try your call again.
* - ValidationErrorException Inspect your request and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SageMakerRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample SageMakerRuntimeAsyncClient.InvokeEndpointAsync
* @see AWS API Documentation
*/
default CompletableFuture invokeEndpointAsync(
Consumer invokeEndpointAsyncRequest) {
return invokeEndpointAsync(InvokeEndpointAsyncRequest.builder().applyMutation(invokeEndpointAsyncRequest).build());
}
@Override
default SageMakerRuntimeServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link SageMakerRuntimeAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SageMakerRuntimeAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SageMakerRuntimeAsyncClient}.
*/
static SageMakerRuntimeAsyncClientBuilder builder() {
return new DefaultSageMakerRuntimeAsyncClientBuilder();
}
}