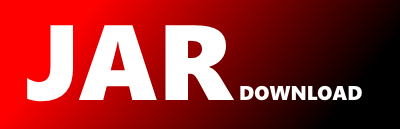
software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointResponse Maven / Gradle / Ivy
Show all versions of sagemakerruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerruntime.model;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class InvokeEndpointResponse extends SageMakerRuntimeResponse implements
ToCopyableBuilder {
private static final SdkField BODY_FIELD = SdkField
. builder(MarshallingType.SDK_BYTES)
.memberName("Body")
.getter(getter(InvokeEndpointResponse::body))
.setter(setter(Builder::body))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Body").build(),
PayloadTrait.create()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ContentType").getter(getter(InvokeEndpointResponse::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField INVOKED_PRODUCTION_VARIANT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InvokedProductionVariant")
.getter(getter(InvokeEndpointResponse::invokedProductionVariant))
.setter(setter(Builder::invokedProductionVariant))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-Amzn-Invoked-Production-Variant")
.build()).build();
private static final SdkField CUSTOM_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CustomAttributes")
.getter(getter(InvokeEndpointResponse::customAttributes))
.setter(setter(Builder::customAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-SageMaker-Custom-Attributes")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BODY_FIELD,
CONTENT_TYPE_FIELD, INVOKED_PRODUCTION_VARIANT_FIELD, CUSTOM_ATTRIBUTES_FIELD));
private final SdkBytes body;
private final String contentType;
private final String invokedProductionVariant;
private final String customAttributes;
private InvokeEndpointResponse(BuilderImpl builder) {
super(builder);
this.body = builder.body;
this.contentType = builder.contentType;
this.invokedProductionVariant = builder.invokedProductionVariant;
this.customAttributes = builder.customAttributes;
}
/**
*
* Includes the inference provided by the model.
*
*
* For information about the format of the response body, see Common Data Formats-Inference.
*
*
* If the explainer is activated, the body includes the explanations provided by the model. For more information,
* see the Response section under Invoke the Endpoint in the Developer Guide.
*
*
* @return Includes the inference provided by the model.
*
* For information about the format of the response body, see Common Data
* Formats-Inference.
*
*
* If the explainer is activated, the body includes the explanations provided by the model. For more
* information, see the Response section under Invoke the Endpoint in the Developer Guide.
*/
public final SdkBytes body() {
return body;
}
/**
*
* The MIME type of the inference returned from the model container.
*
*
* @return The MIME type of the inference returned from the model container.
*/
public final String contentType() {
return contentType;
}
/**
*
* Identifies the production variant that was invoked.
*
*
* @return Identifies the production variant that was invoked.
*/
public final String invokedProductionVariant() {
return invokedProductionVariant;
}
/**
*
* Provides additional information in the response about the inference returned by a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to return an ID received in the CustomAttributes
header of a request or other metadata that
* a service endpoint was programmed to produce. The value must consist of no more than 1024 visible US-ASCII
* characters as specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1). If the customer wants the custom attribute
* returned, the model must set the custom attribute to be included on the way back.
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @return Provides additional information in the response about the inference returned by a model hosted at an
* Amazon SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use
* this value, for example, to return an ID received in the CustomAttributes
header of a
* request or other metadata that a service endpoint was programmed to produce. The value must consist of no
* more than 1024 visible US-ASCII characters as specified in Section 3.3.6. Field Value Components of the
* Hypertext Transfer Protocol (HTTP/1.1). If the customer wants the custom attribute returned, the model
* must set the custom attribute to be included on the way back.
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with
* Trace ID:
in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker
* Python SDK.
*/
public final String customAttributes() {
return customAttributes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(body());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(invokedProductionVariant());
hashCode = 31 * hashCode + Objects.hashCode(customAttributes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InvokeEndpointResponse)) {
return false;
}
InvokeEndpointResponse other = (InvokeEndpointResponse) obj;
return Objects.equals(body(), other.body()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(invokedProductionVariant(), other.invokedProductionVariant())
&& Objects.equals(customAttributes(), other.customAttributes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InvokeEndpointResponse").add("Body", body() == null ? null : "*** Sensitive Data Redacted ***")
.add("ContentType", contentType()).add("InvokedProductionVariant", invokedProductionVariant())
.add("CustomAttributes", customAttributes() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Body":
return Optional.ofNullable(clazz.cast(body()));
case "ContentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "InvokedProductionVariant":
return Optional.ofNullable(clazz.cast(invokedProductionVariant()));
case "CustomAttributes":
return Optional.ofNullable(clazz.cast(customAttributes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For information about the format of the response body, see Common Data
* Formats-Inference.
*
*
* If the explainer is activated, the body includes the explanations provided by the model. For more
* information, see the Response section under Invoke the Endpoint in the Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder body(SdkBytes body);
/**
*
* The MIME type of the inference returned from the model container.
*
*
* @param contentType
* The MIME type of the inference returned from the model container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder contentType(String contentType);
/**
*
* Identifies the production variant that was invoked.
*
*
* @param invokedProductionVariant
* Identifies the production variant that was invoked.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder invokedProductionVariant(String invokedProductionVariant);
/**
*
* Provides additional information in the response about the inference returned by a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value,
* for example, to return an ID received in the CustomAttributes
header of a request or other
* metadata that a service endpoint was programmed to produce. The value must consist of no more than 1024
* visible US-ASCII characters as specified in Section 3.3.6. Field Value Components of the
* Hypertext Transfer Protocol (HTTP/1.1). If the customer wants the custom attribute returned, the model must
* set the custom attribute to be included on the way back.
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your
* code does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python
* SDK.
*
*
* @param customAttributes
* Provides additional information in the response about the inference returned by a model hosted at an
* Amazon SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could
* use this value, for example, to return an ID received in the CustomAttributes
header of a
* request or other metadata that a service endpoint was programmed to produce. The value must consist of
* no more than 1024 visible US-ASCII characters as specified in Section 3.3.6. Field Value Components of
* the Hypertext Transfer Protocol (HTTP/1.1). If the customer wants the custom attribute returned, the
* model must set the custom attribute to be included on the way back.
*
* The code in your model is responsible for setting or updating any custom attributes in the response.
* If your code does not set this value in the response, an empty value is returned. For example, if a
* custom attribute represents the trace ID, your model can prepend the custom attribute with
* Trace ID:
in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker
* Python SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder customAttributes(String customAttributes);
}
static final class BuilderImpl extends SageMakerRuntimeResponse.BuilderImpl implements Builder {
private SdkBytes body;
private String contentType;
private String invokedProductionVariant;
private String customAttributes;
private BuilderImpl() {
}
private BuilderImpl(InvokeEndpointResponse model) {
super(model);
body(model.body);
contentType(model.contentType);
invokedProductionVariant(model.invokedProductionVariant);
customAttributes(model.customAttributes);
}
public final ByteBuffer getBody() {
return body == null ? null : body.asByteBuffer();
}
public final void setBody(ByteBuffer body) {
body(body == null ? null : SdkBytes.fromByteBuffer(body));
}
@Override
public final Builder body(SdkBytes body) {
this.body = body;
return this;
}
public final String getContentType() {
return contentType;
}
public final void setContentType(String contentType) {
this.contentType = contentType;
}
@Override
public final Builder contentType(String contentType) {
this.contentType = contentType;
return this;
}
public final String getInvokedProductionVariant() {
return invokedProductionVariant;
}
public final void setInvokedProductionVariant(String invokedProductionVariant) {
this.invokedProductionVariant = invokedProductionVariant;
}
@Override
public final Builder invokedProductionVariant(String invokedProductionVariant) {
this.invokedProductionVariant = invokedProductionVariant;
return this;
}
public final String getCustomAttributes() {
return customAttributes;
}
public final void setCustomAttributes(String customAttributes) {
this.customAttributes = customAttributes;
}
@Override
public final Builder customAttributes(String customAttributes) {
this.customAttributes = customAttributes;
return this;
}
@Override
public InvokeEndpointResponse build() {
return new InvokeEndpointResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}