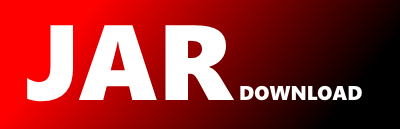
software.amazon.awssdk.services.sagemakerruntime.model.InvokeEndpointRequest Maven / Gradle / Ivy
Show all versions of sagemakerruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerruntime.model;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class InvokeEndpointRequest extends SageMakerRuntimeRequest implements
ToCopyableBuilder {
private static final SdkField ENDPOINT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointName").getter(getter(InvokeEndpointRequest::endpointName)).setter(setter(Builder::endpointName))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("EndpointName").build()).build();
private static final SdkField BODY_FIELD = SdkField
. builder(MarshallingType.SDK_BYTES)
.memberName("Body")
.getter(getter(InvokeEndpointRequest::body))
.setter(setter(Builder::body))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Body").build(),
PayloadTrait.create()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ContentType").getter(getter(InvokeEndpointRequest::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField ACCEPT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Accept")
.getter(getter(InvokeEndpointRequest::accept)).setter(setter(Builder::accept))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Accept").build()).build();
private static final SdkField CUSTOM_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CustomAttributes")
.getter(getter(InvokeEndpointRequest::customAttributes))
.setter(setter(Builder::customAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-SageMaker-Custom-Attributes")
.build()).build();
private static final SdkField TARGET_MODEL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TargetModel")
.getter(getter(InvokeEndpointRequest::targetModel))
.setter(setter(Builder::targetModel))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-SageMaker-Target-Model")
.build()).build();
private static final SdkField TARGET_VARIANT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TargetVariant")
.getter(getter(InvokeEndpointRequest::targetVariant))
.setter(setter(Builder::targetVariant))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-SageMaker-Target-Variant")
.build()).build();
private static final SdkField TARGET_CONTAINER_HOSTNAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TargetContainerHostname")
.getter(getter(InvokeEndpointRequest::targetContainerHostname))
.setter(setter(Builder::targetContainerHostname))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("X-Amzn-SageMaker-Target-Container-Hostname").build()).build();
private static final SdkField INFERENCE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InferenceId")
.getter(getter(InvokeEndpointRequest::inferenceId))
.setter(setter(Builder::inferenceId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-SageMaker-Inference-Id")
.build()).build();
private static final SdkField ENABLE_EXPLANATIONS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EnableExplanations")
.getter(getter(InvokeEndpointRequest::enableExplanations))
.setter(setter(Builder::enableExplanations))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("X-Amzn-SageMaker-Enable-Explanations").build()).build();
private static final SdkField INFERENCE_COMPONENT_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InferenceComponentName")
.getter(getter(InvokeEndpointRequest::inferenceComponentName))
.setter(setter(Builder::inferenceComponentName))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER)
.locationName("X-Amzn-SageMaker-Inference-Component").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENDPOINT_NAME_FIELD,
BODY_FIELD, CONTENT_TYPE_FIELD, ACCEPT_FIELD, CUSTOM_ATTRIBUTES_FIELD, TARGET_MODEL_FIELD, TARGET_VARIANT_FIELD,
TARGET_CONTAINER_HOSTNAME_FIELD, INFERENCE_ID_FIELD, ENABLE_EXPLANATIONS_FIELD, INFERENCE_COMPONENT_NAME_FIELD));
private final String endpointName;
private final SdkBytes body;
private final String contentType;
private final String accept;
private final String customAttributes;
private final String targetModel;
private final String targetVariant;
private final String targetContainerHostname;
private final String inferenceId;
private final String enableExplanations;
private final String inferenceComponentName;
private InvokeEndpointRequest(BuilderImpl builder) {
super(builder);
this.endpointName = builder.endpointName;
this.body = builder.body;
this.contentType = builder.contentType;
this.accept = builder.accept;
this.customAttributes = builder.customAttributes;
this.targetModel = builder.targetModel;
this.targetVariant = builder.targetVariant;
this.targetContainerHostname = builder.targetContainerHostname;
this.inferenceId = builder.inferenceId;
this.enableExplanations = builder.enableExplanations;
this.inferenceComponentName = builder.inferenceComponentName;
}
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @return The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*/
public final String endpointName() {
return endpointName;
}
/**
*
* Provides input data, in the format specified in the ContentType
request header. Amazon SageMaker
* passes all of the data in the body to the model.
*
*
* For information about the format of the request body, see Common Data Formats-Inference.
*
*
* @return Provides input data, in the format specified in the ContentType
request header. Amazon
* SageMaker passes all of the data in the body to the model.
*
* For information about the format of the request body, see Common Data
* Formats-Inference.
*/
public final SdkBytes body() {
return body;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @return The MIME type of the input data in the request body.
*/
public final String contentType() {
return contentType;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @return The desired MIME type of the inference response from the model container.
*/
public final String accept() {
return accept;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @return Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata
* that a service endpoint was programmed to process. The value must consist of no more than 1024 visible
* US-ASCII characters as specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with
* Trace ID:
in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker
* Python SDK.
*/
public final String customAttributes() {
return customAttributes;
}
/**
*
* The model to request for inference when invoking a multi-model endpoint.
*
*
* @return The model to request for inference when invoking a multi-model endpoint.
*/
public final String targetModel() {
return targetModel;
}
/**
*
* Specify the production variant to send the inference request to when invoking an endpoint that is running two or
* more variants. Note that this parameter overrides the default behavior for the endpoint, which is to distribute
* the invocation traffic based on the variant weights.
*
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*
*
* @return Specify the production variant to send the inference request to when invoking an endpoint that is running
* two or more variants. Note that this parameter overrides the default behavior for the endpoint, which is
* to distribute the invocation traffic based on the variant weights.
*
* For information about how to use variant targeting to perform a/b testing, see Test models in
* production
*/
public final String targetVariant() {
return targetVariant;
}
/**
*
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter specifies
* the host name of the container to invoke.
*
*
* @return If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter
* specifies the host name of the container to invoke.
*/
public final String targetContainerHostname() {
return targetContainerHostname;
}
/**
*
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*
*
* @return If you provide a value, it is added to the captured data when you enable data capture on the endpoint.
* For information about data capture, see Capture Data.
*/
public final String inferenceId() {
return inferenceId;
}
/**
*
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*
*
* @return An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*/
public final String enableExplanations() {
return enableExplanations;
}
/**
*
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference component
* to invoke.
*
*
* @return If the endpoint hosts one or more inference components, this parameter specifies the name of inference
* component to invoke.
*/
public final String inferenceComponentName() {
return inferenceComponentName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(endpointName());
hashCode = 31 * hashCode + Objects.hashCode(body());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(accept());
hashCode = 31 * hashCode + Objects.hashCode(customAttributes());
hashCode = 31 * hashCode + Objects.hashCode(targetModel());
hashCode = 31 * hashCode + Objects.hashCode(targetVariant());
hashCode = 31 * hashCode + Objects.hashCode(targetContainerHostname());
hashCode = 31 * hashCode + Objects.hashCode(inferenceId());
hashCode = 31 * hashCode + Objects.hashCode(enableExplanations());
hashCode = 31 * hashCode + Objects.hashCode(inferenceComponentName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InvokeEndpointRequest)) {
return false;
}
InvokeEndpointRequest other = (InvokeEndpointRequest) obj;
return Objects.equals(endpointName(), other.endpointName()) && Objects.equals(body(), other.body())
&& Objects.equals(contentType(), other.contentType()) && Objects.equals(accept(), other.accept())
&& Objects.equals(customAttributes(), other.customAttributes())
&& Objects.equals(targetModel(), other.targetModel()) && Objects.equals(targetVariant(), other.targetVariant())
&& Objects.equals(targetContainerHostname(), other.targetContainerHostname())
&& Objects.equals(inferenceId(), other.inferenceId())
&& Objects.equals(enableExplanations(), other.enableExplanations())
&& Objects.equals(inferenceComponentName(), other.inferenceComponentName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InvokeEndpointRequest").add("EndpointName", endpointName())
.add("Body", body() == null ? null : "*** Sensitive Data Redacted ***").add("ContentType", contentType())
.add("Accept", accept())
.add("CustomAttributes", customAttributes() == null ? null : "*** Sensitive Data Redacted ***")
.add("TargetModel", targetModel()).add("TargetVariant", targetVariant())
.add("TargetContainerHostname", targetContainerHostname()).add("InferenceId", inferenceId())
.add("EnableExplanations", enableExplanations()).add("InferenceComponentName", inferenceComponentName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EndpointName":
return Optional.ofNullable(clazz.cast(endpointName()));
case "Body":
return Optional.ofNullable(clazz.cast(body()));
case "ContentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "Accept":
return Optional.ofNullable(clazz.cast(accept()));
case "CustomAttributes":
return Optional.ofNullable(clazz.cast(customAttributes()));
case "TargetModel":
return Optional.ofNullable(clazz.cast(targetModel()));
case "TargetVariant":
return Optional.ofNullable(clazz.cast(targetVariant()));
case "TargetContainerHostname":
return Optional.ofNullable(clazz.cast(targetContainerHostname()));
case "InferenceId":
return Optional.ofNullable(clazz.cast(inferenceId()));
case "EnableExplanations":
return Optional.ofNullable(clazz.cast(enableExplanations()));
case "InferenceComponentName":
return Optional.ofNullable(clazz.cast(inferenceComponentName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function